Understanding Modern Development Frameworks: A Guide for Developers and Technical Decision-makers

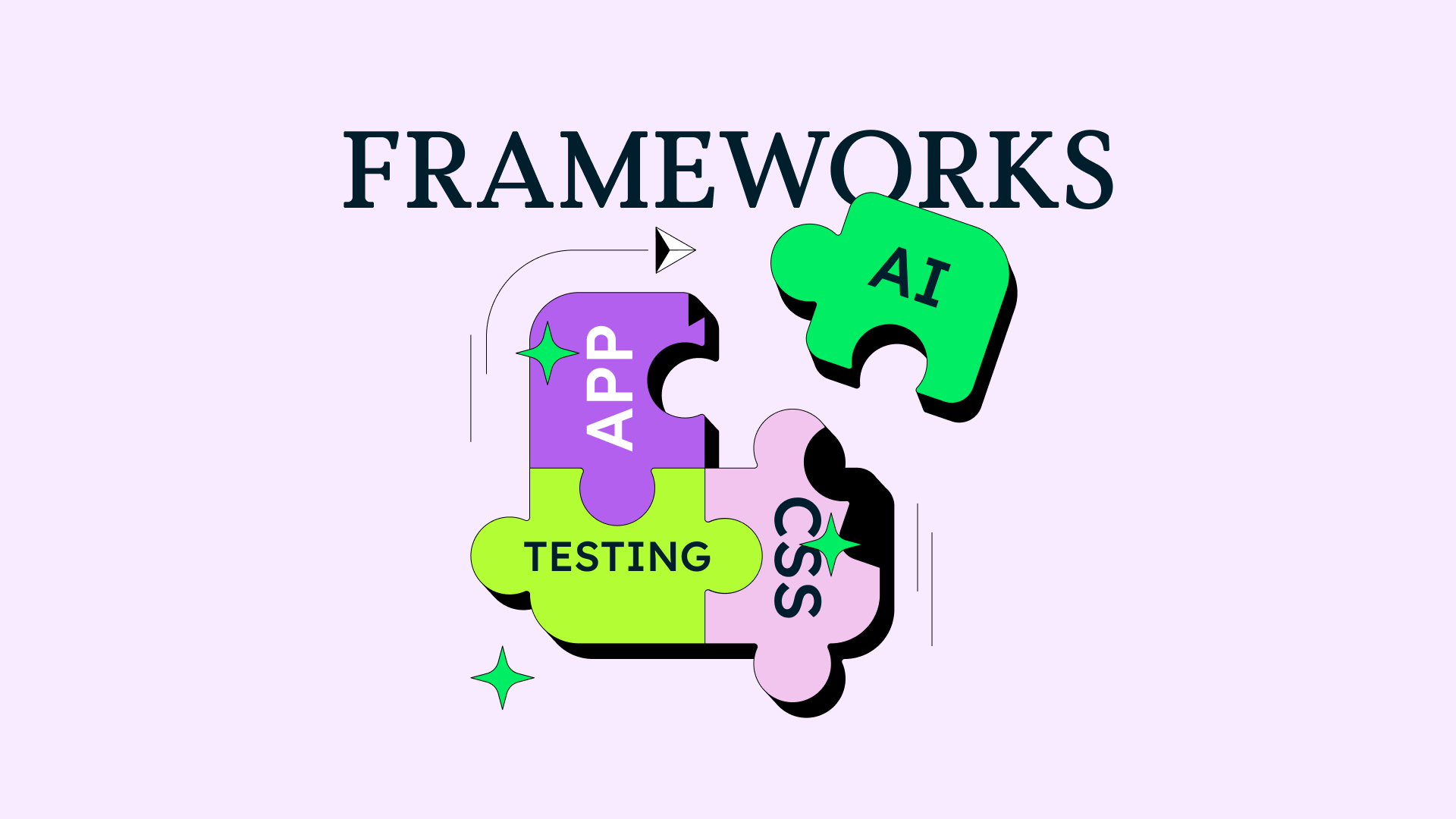
As a developer for over 20 years, I've seen firsthand how choosing the right framework can make or break a project. The term "framework" has become so broad that it's often misunderstood. Let's clear up the confusion and help you make better technical decisions.
What is a Framework?
In terms of software development, a framework is a structured set of tools, libraries, and conventions that provides a foundation for building applications more efficiently by handling common functionalities so developers can focus on unique features.
A modern web application can combine multiple frameworks to handle different aspects of development efficiently:
React with Tailwind CSS manages the user interface and styling.
FastAPI or Django with LangChain handle backend operations and AI functionality, while MongoDB acts as a memory store.
These frameworks communicate through APIs and defined interfaces to work together—for example, a user interaction in the React front end can trigger an AI process through LangChain in the Python back end, which uses MongoDB Atlas Vector Search to retrieve relevant data and then display it back to the user with Tailwind CSS styles.
Table of Contents:
The Framework Landscape in 2024
Think of frameworks as tools in a technical toolbox. Understanding what each tool is designed to do—and what it isn't—ensures you use the right solution for the task at hand.
The Evolution of Development Frameworks
The framework landscape has transformed dramatically over the past decade. What started as simple libraries for rendering web pages has evolved into sophisticated ecosystems that can:
Handle complex state management.
Process real-time data streams.
Integrate AI capabilities.
Scale automatically based on demand.
Deploy across multiple platforms from a single codebase.
Why Framework Choice Matters
Frameworks are puzzle pieces: each has a unique shape and function. When chosen wisely, they fit together seamlessly to create a cohesive application. But forcing incompatible pieces together can lead to inefficiencies and broken functionality.
Here's why your choice matters:
Technical impact
Performance: Different frameworks have different performance characteristics. Instagram chose React for its virtual DOM, which handles frequent updates efficiently.
Scalability: Uber's back end uses Node.js because it excels at handling numerous concurrent connections.
Maintenance: Shopify standardized on React Native to maintain a single codebase for mobile applications.
Business impact
Development speed: The right framework can accelerate development by 2-3x.
Team productivity: Familiar frameworks reduce onboarding time from months to weeks.
Cost efficiency: Proper framework selection can significantly reduce hosting and maintenance costs.
Common pitfalls
Over-engineering: Using Next.js when a simple HTML page would suffice
Under-engineering: Using vanilla JavaScript for a complex, state-heavy application
Misaligned tools: Using Electron (a desktop app framework) to build a simple website
Trend-chasing: Adopting the newest framework without considering maintenance implications
Let’s dive deeper into the various categories of development frameworks and how they can help you build better applications.
Note: I give framework examples throughout this article, but these are not exhaustive lists and new frameworks are created daily|weekly|monthly
.
Application Frameworks
These are your Swiss Army knives of development—comprehensive toolkits that handle the entire application lifecycle. While each framework has its specialties, they typically provide:
Database integration and ORM support.
Authentication and authorization.
API routing and middleware.
Template engines or component systems.
Asset management.
Security features.
Development tools and debugging support.
What is an application? An application is the combination of individual components working together, including a user interface and backend services, to perform specific functions or a set of functions for users. It’s designed to be fully deployable and operate in production environments.
You'll encounter them in three main flavors:
Full-Stack Web Application Frameworks
Django and Rails: Perfect for data-heavy applications with complex business logic. They follow the "batteries included" philosophy, providing everything you need out of the box.
Next.js and Nuxt.js: Modern full-stack frameworks optimized for React and Vue.js, respectively. They excel at building performant applications with capabilities like server-side rendering, static site generation, and API integration.
Spring Boot: Enterprise-grade framework favored for large-scale Java applications, particularly in financial and banking sectors.
Mobile Application Frameworks
Flutter: Google's toolkit for building natively compiled apps for mobile, web, and desktop from a single codebase. Known for smooth animations and native performance.
React Native: Ideal when you want to leverage your team's React knowledge for mobile development. Great for apps that need to feel native while maintaining code sharing between platforms.
SwiftUI and Jetpack Compose: Native frameworks for iOS and Android, respectively. Ideal when platform-specific features and optimal performance are crucial.
Desktop Application Frameworks
Electron: Powers apps like VS Code and Slack. Ideal for leveraging existing web development knowledge without having to learn OS-specific languages to build cross-platform desktop applications.
Tauri: A modern alternative to Electron with smaller bundle sizes and better performance. Uses native system webviews to run apps more efficiently, with less memory and faster startup times. Developers can use their existing web development skills without learning new programming languages for each OS.
PyQt: A good option for building GUI applications in Python, especially for data science projects. It has a rich set of libraries and widgets, suitable for both small tools and complex apps.
Gaming Frameworks
Unity: A popular framework used for creating 2D and 3D games. It supports multiple platforms, making it a versatile choice for game developers. Unity is known for its user-friendly interface and extensive asset store.
Unreal Engine: Known for its high-quality graphics, Unreal Engine is a powerful framework used for both AAA games and indie projects. It provides advanced visual tools and real-time rendering capabilities.
Godot: An open-source game engine that's lightweight and flexible. Godot is often used for smaller or indie game projects and features an intuitive scene system that makes development straightforward.
AI Frameworks
Here's a crucial point many miss: AI frameworks like TensorFlow or LangChain are powerful, but they're not standalone solutions. These frameworks require integration with other tools and frameworks for data handling, user interface, and deployment to create a complete, production-ready application. These are just another piece of the puzzle.
The exception? Data scientists can use these tools directly in Jupyter notebooks for research and prototyping. But for production applications, you'll need more.
Let's look at the major players and their sweet spots:
AI Model Development Frameworks
TensorFlow: Google's powerhouse for deep learning, TensorFlow is perfect for applications involving computer vision, neural networks, and production-grade ML. Pinterest uses it for image recognition and recommendations, highlighting its strength in processing large amounts of image data efficiently.
PyTorch: Developed by Facebook, PyTorch is a flexible framework ideal for research, natural language processing, and quick prototyping. Tesla has utilized PyTorch for various machine learning tasks, including research related to autonomous driving vision systems, demonstrating its versatility in cutting-edge applications.
JAX: A high-performance numerical computing framework by Google, JAX is well-suited for scientific computing and large-scale transformers. DeepMind has used JAX for advanced AI research, including projects like AlphaFold for protein structure prediction, showcasing its effectiveness in large-scale computational problems.
AI Model Deployment and Serving Frameworks
TensorFlow Serving: A deployment tool for TensorFlow models, perfect for serving high-performance ML models in production environments. For example, it has been used to serve image classification models for e-commerce platforms, ensuring rapid and scalable response times.
TorchServe: A robust tool for deploying PyTorch models, perfect for scalable PyTorch model serving. It has been used for deploying chatbot models for real-time customer support, providing flexibility and efficiency in managing conversational AI.
NVIDIA Triton Inference Server: A multi-framework model serving tool, NVIDIA Triton Inference Server is capable of handling models from TensorFlow, PyTorch, ONNX, and more. It is ideal for managing inference requests for multi-modal AI applications, making it highly suitable for complex AI deployments.
LLM Integration Frameworks
LangChain: The Swiss Army knife for LLM applications. Ideal for building chatbots and document Q&A systems. It has been employed to create customer service bots capable of accessing company knowledge bases, demonstrating its usefulness in enhancing customer interactions.
LlamaIndex: Specializing in data connection, LlamaIndex is perfect for building search engines over private data. It has been used to create semantic search systems for internal documents, facilitating more effective data retrieval and organizational knowledge management.
Hugging Face Transformers: A pre-trained model hub, Hugging Face Transformers allows for the quick deployment of state-of-the-art models. It has been used to add sentiment analysis to customer feedback systems, highlighting its capability in natural language understanding.
AI Data Processing Frameworks
Apache Spark (MLlib): Ideal for large-scale data transformation and ML, used for processing millions of user interactions in recommendation systems. Its scalability and efficiency make it a popular choice for big data ML tasks.
Pandas: A widely-used data manipulation and analysis tool that is perfect for data cleaning, analysis, and feature engineering. It is often employed in preparing customer data for churn prediction models, owing to its intuitive data handling capabilities.
Polars: A high-performance data manipulation framework, used when Pandas is not fast enough for processing requirements. It has been applied in real-time financial data analysis, delivering faster data processing and efficiency.
AI Automation and Orchestration Frameworks
CrewAI: Used to orchestrate multiple AI agents, making it perfect for complex workflows requiring multiple AI models. It has been used to create a content creation pipeline that plans, writes, and edits, showcasing its ability to automate creative processes.
Auto-GPT: Develops autonomous AI agents, ideal for self-directed task completion. It has been utilized for automated research and data gathering, highlighting its potential for automating repetitive knowledge tasks.
Microsoft Semantic Kernel: An AI orchestration tool perfect for integrating AI into .NET applications. It has been used to add AI capabilities to existing enterprise applications, providing a seamless integration of AI functions into established workflows.
AI Data Ingestion and Document Processing Frameworks
Apache NiFi: A powerful tool for data flow automation, well-suited for real-time data ingestion and processing. It has been used to extract and transform log data before analysis, ensuring efficient data flow for various use cases.
Haystack: Specialized in document processing for search and QA systems. It has been utilized to create pipelines for indexing documents and answering questions, making it ideal for building internal knowledge base searches.
Unstructured: Designed for extracting data from a variety of formats, including PDFs, HTML, and images. It’s perfect for handling unstructured content in document processing workflows and has been used to extract relevant information from scanned documents for data analysis.
Airbyte: An open-source data integration tool that is ideal for connecting and syncing data across multiple sources. It has been used to ingest data from third-party APIs into analytics systems, enabling effective data consolidation.
Interactive AI Web Frameworks
Gradio: Simplifies the process of building web UIs for machine learning models, making it perfect for creating quick demos for ML models. It has been used to create interactive image classifiers for end users, providing an accessible interface for testing ML capabilities.
Streamlit: A Python-based web app framework for ML and data science, ideal for turning data scripts into shareable web apps. It has been used to build user-friendly dashboards for exploring model predictions, enhancing the accessibility of ML models.
MLOps and AI Monitoring Frameworks
MLflow: Used for experiment tracking and model lifecycle management, perfect for keeping track of experiments and model versions. It has been applied to manage multiple iterations of predictive models, supporting organized development workflows.
Kubeflow: A Kubernetes-native platform for MLOps, ideal for deploying, scaling, and managing machine learning models on Kubernetes. It has been used to run end-to-end ML workflows in production environments, ensuring scalability and consistency.
Prometheus & Grafana: Monitoring and alerting tools for ML infrastructure, perfect for tracking model inference performance and system metrics. They have been used to monitor latency and resource usage of deployed ML services, ensuring optimal operational performance.
Web frameworks
Web development has evolved into two distinct camps—think of them as the front-of-house and kitchen staff in a restaurant. Both essential, both specialized, but with very different responsibilities.
Note: We already discussed full-stack web application frameworks above, which marry these two together.
Frontend Frameworks
Frontend frameworks handle what users see and interact with, managing everything from data display to user interactions, user input, and how the overall user experience is structured. These frameworks ensure that the interface is visually appealing, intuitive, and responsive to user actions.
They play a critical role in how smoothly data is presented, processed, and updated in real time, providing dynamic elements like animations, form validation, and client-side routing to enhance usability.
By using frontend frameworks, developers can create highly interactive and cohesive user experiences that feel natural and engaging.
React: Ideal for large-scale applications with complex state management. It features a virtual DOM for optimal performance, making it suitable for highly interactive user interfaces.
Vue.js: Perfect for both small projects and enterprise applications. It has a gentle learning curve combined with powerful scalability, making it an approachable yet robust frontend solution.
Svelte: Ideal for when you have performance-critical applications and want smaller bundles. It compiles away framework code for lighter applications, providing better performance and a smaller footprint.
Backend Frameworks
Backend frameworks manage server-side logic, data processing, and system integration, handling everything from receiving and processing client requests to interacting with databases and external APIs. These frameworks ensure that the server processes are efficient, scalable, and secure, supporting high concurrency and maintaining consistent data flows.
They provide the essential tools for developers to build, maintain, and optimize the server-side of applications, including tasks such as handling authentication, managing business logic, and ensuring data consistency.
A well-chosen backend framework allows developers to focus more on creating features rather than dealing with low-level server management.
Express.js: Perfect for APIs and microservices, offering minimal structure with maximum flexibility. It is highly popular in Node.js environments for building efficient server-side logic.
FastAPI: Designed for high-performance APIs, with automatic API documentation and type checking. It is commonly used for fast and secure backend implementations in Python.
NestJS: For large-scale Node.js applications, featuring an Angular-inspired architecture that enhances scalability. It provides a well-structured framework for enterprise-level backends.
CSS/UI Frameworks
Modern CSS frameworks have evolved beyond simple styling to become complete design systems. Gone are the days when CSS frameworks just provided basic grid systems and button styles.
Today's frameworks are sophisticated tools that enable consistent design at scale. They offer features such as responsive layouts, dark mode support, accessibility enhancements, and interactive components.
They've become essential for maintaining design consistency across large applications and teams, while significantly reducing development time and technical debt. Some frameworks even include built-in performance optimization, design tokens for brand customization, and tools for managing design systems across multiple platforms.
They fall into two main categories:
Utility-first Frameworks
Tailwind CSS: Custom designs with consistent systems. It features rapid prototyping capabilities, highly customizable design tokens, and small production bundles through PurgeCSS.
UnoCSS: A customizable, efficient, utility-first CSS solution. It provides on-demand CSS for optimal performance and minimal bundle size, making it a modern tool for design system management.
Component-based Frameworks
Bootstrap: Rapid prototyping and traditional web applications. It offers an extensive library of pre-built components, a responsive grid system, and a rich ecosystem of themes and plugins.
Material UI: For applications following Material Design guidelines. It includes a comprehensive component library, a theming system, and accessibility compliance features.
Testing and Infrastructure
These frameworks form the backbone of reliable, scalable applications. While they might not get as much attention as flashy frontend frameworks or AI tools, they're the critical infrastructure that keeps modern applications running smoothly at scale.
Testing frameworks ensure code quality and prevent regressions, while containerization and orchestration frameworks handle the increasingly complex world of cloud deployment and scaling.
In an era where a single code change can affect millions of users instantly, or where an application might need to scale from hundreds to millions of requests in minutes, these frameworks aren't just nice-to-have—they're essential for survival. Major tech companies like Netflix, Amazon, and Google rely on these tools to maintain their rapid development pace while ensuring reliability and performance at massive scale.
Testing Frameworks
Jest: Features snapshot testing, code coverage, and mocking capabilities, making it a popular choice for ensuring the quality of JavaScript codebases.
Cypress: Provides real browser testing and time-travel debugging, ideal for end-to-end testing of web applications.
PyTest: Known for its simple syntax, powerful extensions, and easy fixture management, making it a go-to framework for testing Python applications.
Containerization and Orchestration
Docker: Used for application containerization, providing consistent development and deployment environments with isolated dependencies and efficient resource usage.
Kubernetes: Orchestration of large-scale distributed applications. It provides automated scaling, self-healing deployments, and rolling updates, ensuring that containerized applications can grow as needed.
Making the Right Choice
Selecting the right framework can determine the success of your entire project. Consider these critical points to guide your decision-making process:
1. Application Type and Scale
Small site? Consider React or Vue.js.
Large application? Next.js or Django might be better.
Need SEO? Look for SSR capabilities.
2. Team Capabilities
Strong JavaScript? Consider Node.js ecosystem.
Python experts? Django or FastAPI might be better.
Need quick ramp-up? Combine key frameworks like Vue.js and Bootstrap.
3. Technical Requirements
High-performance computing? Consider Rust frameworks.
Real-time updates? Look at WebSocket support.
AI requirements? Integration with platforms like Hugging Face and frameworks like LangChain might be essential.
4. Scaling Strategy
Vertical scaling? Simpler frameworks might suffice.
Horizontal scaling? You need frameworks with microservices support.
Global distribution? Consider edge computing capabilities.
5. Long-term Maintenance
Community size and activity
Available talent pool
Corporate backing and stability
Documentation quality
Upgrade path complexity
The Bottom Line
Framework selection isn't just a technical decision—it's a strategic one that affects your project's success, team productivity, and maintenance costs. So take the time to understand your options.
Now you should have all of the puzzle pieces, but it's up to you to put them together thoughtfully to create the perfect technology stack that meets both your current and future needs.
Additional Resources
If you'd like to learn how MongoDB integrates seamlessly with many of the frameworks mentioned in this guide, check out the resources below. You'll find that it offers a flexible and scalable database solution that adapts to different use cases.
Frequently Asked Questions (FAQ)
What is a development framework?
A development framework is a set of tools, libraries, and conventions that provide a foundation for building applications efficiently. Frameworks handle common tasks so developers can focus on building unique features.
How do AI and development frameworks work together?
AI frameworks can be combined with traditional development frameworks to create intelligent, data-driven applications. For instance, a backend framework like FastAPI can handle incoming requests, while an AI framework like LangChain processes natural language data, providing users with enhanced functionality like chatbots or recommendation systems.
Why is choosing the right framework important?
Choosing the right framework can impact your project's performance, scalability, and maintenance. It ensures that your application meets user expectations, is easy to manage, and can grow as needed.
How do I decide which framework to use for my project?
Consider factors like application type, team expertise, performance needs, scalability, and long-term maintenance. Each of these can guide you in selecting a framework that matches your specific requirements.
Can I use multiple frameworks in one project?
Yes, combining multiple frameworks can be very effective. You might use one framework for the front end, another for backend services, and a third for AI integrations, ensuring that each part of your application is handled by the best-suited tool.
How does MongoDB fit into development frameworks?
MongoDB integrates with many frameworks, acting as the data layer for your applications. It provides a flexible, scalable solution that supports full-stack, backend, and AI frameworks to store and retrieve data efficiently.
Subscribe to my newsletter
Read articles from Jesse Hall directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
