Unlocking the Vault: Mastering Encapsulation in JavaScript!
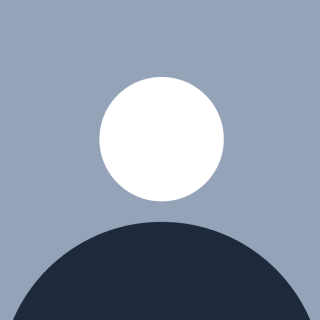
Table of contents
- What is Encapsulation in JavaScript?
- Encapsulation with Getters and Setters
- Using Private Fields in Modern JavaScript
- Flowchart: Encapsulation in Action
- The Benefits of Encapsulation
- Challenge – Build Your Own Secure Vault
- Advanced Encapsulation – Keeping Methods Private
- Encapsulation Best Practices
- Real-World Examples of Encapsulation
- Challenge – Build a Secure Login System
- Unlocking the Power of Encapsulation
- Let’s tackle Advanced Classes Next!
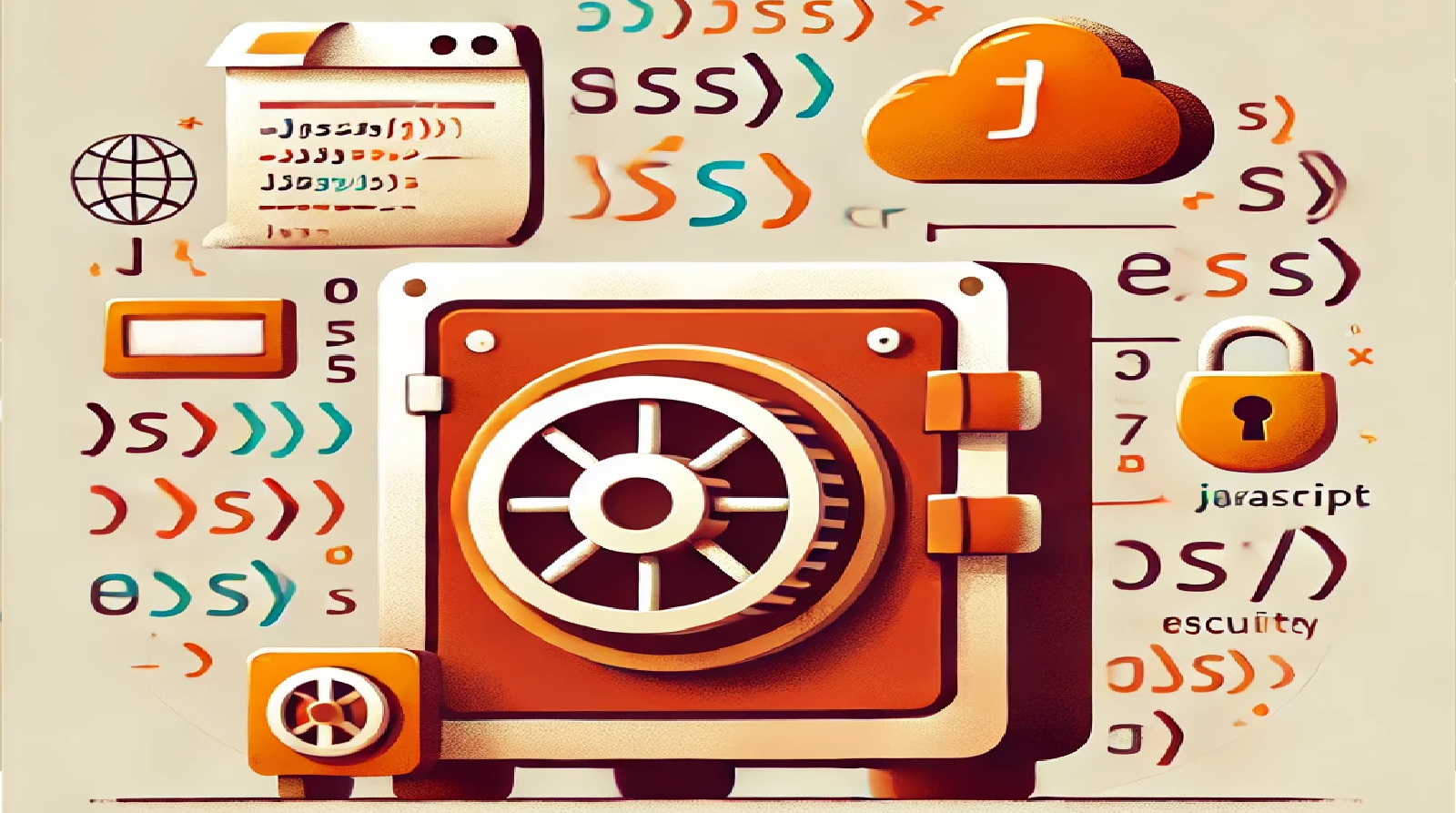
Imagine you have a vault where you keep all your valuable belongings. Only you have the key to open it, and nobody can see or access its contents unless you allow it. In JavaScript, encapsulation works in a similar way—it’s a technique that allows you to hide the internal details of an object (the valuables in the vault) while exposing only the necessary parts to the outside world.
Encapsulation is one of the core principles of Object-Oriented Programming (OOP). It ensures that the internal state of an object is protected and can only be accessed or modified through well-defined interfaces (like methods). This makes your code more secure, organized, and easy to maintain.
What is Encapsulation in JavaScript?
In programming, encapsulation is the process of restricting direct access to certain properties of an object and controlling how they are accessed or modified. The main idea is to bundle the object’s data and the methods that operate on that data together, while keeping the data hidden (or private) from outside interference.
In our vault analogy, the vault represents the object, the valuables inside are the private properties, and the key represents the methods that allow controlled access to those valuables.
Encapsulation with Getters and Setters
One of the most common ways to implement encapsulation in JavaScript is by using getters and setters. These are special methods that allow you to control how the properties of an object are accessed and updated.
Let’s create a vault that holds a valuable item (a secret), but we don’t want anyone to directly access or change that secret. We’ll use getters and setters to control access to the vault.
Code Snippet: Encapsulation with Getters and Setters
class Vault {
constructor(secret) {
this._secret = secret; // The secret is a "private" property (convention uses _)
}
// Getter to access the secret
getSecret() {
return this._secret;
}
// Setter to modify the secret, with some validation
setSecret(newSecret) {
if (newSecret && newSecret.length > 5) {
this._secret = newSecret;
} else {
console.log("The new secret must be longer than 5 characters.");
}
}
}
// Create a new Vault instance
const myVault = new Vault("mySecretKey123");
// Access the secret
console.log(myVault.getSecret()); // Output: mySecretKey123
// Try to update the secret
myVault.setSecret("newKey"); // Output: The new secret must be longer than 5 characters.
myVault.setSecret("newSecretKey456");
console.log(myVault.getSecret()); // Output: newSecretKey456
Explanation:
Private Property (
_secret
): We use the convention of adding an underscore (_
) to signal that the property is private, meaning it shouldn’t be directly accessed or modified.Getter (
getSecret()
): This method allows controlled access to the private property_secret
.Setter (
setSecret()
): This method controls how the private property can be updated, with validation (in this case, requiring the new secret to be at least 6 characters long).
Just like how only the owner can unlock the vault and see its contents, the Vault
class uses getters and setters to control access to the secret inside.
Using Private Fields in Modern JavaScript
In the latest versions of JavaScript, there’s an even better way to implement encapsulation using private fields. These fields are truly private and cannot be accessed from outside the class, not even accidentally. To define a private field, you use the #
symbol before the property name.
Let’s improve our vault by making the secret truly private using private fields.
Code Snippet: Encapsulation with Private Fields
class Vault {
// Private field
#secret;
constructor(secret) {
this.#secret = secret; // The secret is truly private
}
// Getter to access the secret
getSecret() {
return this.#secret;
}
// Setter to modify the secret with validation
setSecret(newSecret) {
if (newSecret && newSecret.length > 5) {
this.#secret = newSecret;
} else {
console.log("The new secret must be longer than 5 characters.");
}
}
}
// Create a new Vault instance
const myVault = new Vault("superSecret123");
// Access the secret
console.log(myVault.getSecret()); // Output: superSecret123
// Try to access the secret directly (this will fail)
console.log(myVault.#secret); // Error: Private field '#secret' must be declared in an enclosing class
// Update the secret using the setter
myVault.setSecret("newSecretKey789");
console.log(myVault.getSecret()); // Output: newSecretKey789
Explanation:
Private Field (
#secret
): Unlike the_secret
convention, using#secret
makes the property truly private. It cannot be accessed or modified from outside the class, ensuring stronger encapsulation.Getter and Setter: Even with private fields, we can still provide controlled access through getter and setter methods.
Now, the secret inside the vault is completely hidden from outside access. Only the vault’s owner (through the defined methods) can unlock and modify the secret.
Flowchart: Encapsulation in Action
Here’s a simple flowchart to illustrate how encapsulation works:
Vault Object
------------------
| #secret | <-- Private Field (hidden from outside)
------------------
| getSecret() | <-- Getter to access the secret
| setSecret() | <-- Setter to modify the secret
------------------
The private field is hidden inside the vault, and only the getter and setter provide controlled access. Without these methods, the outside world cannot directly interact with the private field.
The Benefits of Encapsulation
Encapsulation offers several key benefits for organizing and protecting your code:
1. Data Protection
Just like a vault keeps your valuables safe, encapsulation protects sensitive data inside objects. Private fields and methods ensure that your code can’t be accidentally or maliciously modified from outside the object.
2. Simplified Interface
Encapsulation hides unnecessary details from the outside world and exposes only the essential methods. This makes your code easier to understand and interact with.
3. Code Flexibility
By controlling how data is accessed and modified, encapsulation makes it easier to update or change the internal workings of a class without affecting the rest of your code. You can modify how a private property works without changing the interface exposed to the outside world.
Challenge – Build Your Own Secure Vault
Now that you understand the basics of encapsulation, it’s time to build your own secure vault! Create a class that holds private data, uses getters and setters to control access, and implements validation for modifying the data.
Challenge Instructions:
Create a
BankAccount
class: This class should have private fields forbalance
andaccountNumber
.Use getters and setters: Provide a getter to check the balance and a setter to deposit or withdraw funds, ensuring that withdrawals don’t exceed the available balance.
Implement validation: Ensure that deposits are positive numbers and that withdrawals don’t cause the balance to go negative.
Example:
class BankAccount {
#balance;
#accountNumber;
constructor(accountNumber, initialBalance) {
this.#accountNumber = accountNumber;
this.#balance = initialBalance;
}
// Getter for balance
getBalance() {
return this.#balance;
}
// Setter for deposit
deposit(amount) {
if (amount > 0) {
this.#balance += amount;
console.log(`Deposited: $${amount}. New balance: $${this.#balance}.`);
} else {
console.log("Deposit amount must be positive.");
}
}
// Setter for withdrawal
withdraw(amount) {
if (amount > 0 && amount <= this.#balance) {
this.#balance -= amount;
console.log(`Withdrew: $${amount}. New balance: $${this.#balance}.`);
} else {
console.log("Insufficient balance or invalid amount.");
}
}
}
// Example usage
const account = new BankAccount("12345", 1000);
account.deposit(200); // Deposited: $200. New balance: $1200.
account.withdraw(500); // Withdrew: $500. New balance: $700.
console.log(account.getBalance()); // Output: $700
Advanced Encapsulation – Keeping Methods Private
Encapsulation isn't just about hiding properties; you can also hide methods within your class. Sometimes, you may want certain helper functions or logic to stay hidden, only to be used internally within the class itself. JavaScript allows us to make methods private in modern versions by using the #
symbol, just like with private fields.
Let’s add a private method to our Vault
class that checks if the secret is in a valid format before allowing it to be updated.
Code Snippet: Private Methods in JavaScript
class Vault {
#secret;
constructor(secret) {
this.#secret = secret;
}
// Private method to validate the secret
#isValidSecret(newSecret) {
return newSecret && newSecret.length > 5;
}
// Public setter method to update the secret
setSecret(newSecret) {
if (this.#isValidSecret(newSecret)) {
this.#secret = newSecret;
console.log("Secret updated successfully.");
} else {
console.log("Invalid secret. Must be longer than 5 characters.");
}
}
// Public getter method to access the secret
getSecret() {
return this.#secret;
}
}
const vault = new Vault("mySecret123");
// Try to update with an invalid secret
vault.setSecret("123"); // Output: Invalid secret. Must be longer than 5 characters.
// Update with a valid secret
vault.setSecret("newSecret456"); // Output: Secret updated successfully.
console.log(vault.getSecret()); // Output: newSecret456
Explanation:
Private Method (
#isValidSecret()
): This method is hidden from the outside world and is only accessible inside the class. It checks whether the new secret meets the validation criteria.Public Methods (
setSecret()
andgetSecret()
): These methods control how the private property is accessed and updated. The setter method uses the private method to validate new inputs before updating the secret.
This approach ensures that internal logic, like validation, stays hidden and cannot be misused from outside the class.
Encapsulation Best Practices
Now that we've covered the basics of encapsulation, let's go over some best practices to ensure you're using it effectively in your JavaScript projects.
1. Use Private Fields for Sensitive Data
Whenever you're working with sensitive or important data (like passwords, account balances, or private keys), make sure to store them in private fields. This ensures that the data can’t be accessed or tampered with directly.
2. Expose Only What’s Necessary
Limit the number of methods and properties that are publicly accessible. Exposing too much data can make your code more difficult to maintain and may allow unintended access to sensitive parts of your application.
3. Use Getters and Setters Wisely
Getters and setters are powerful tools for encapsulation, but they should be used carefully. Always validate input before modifying private properties, and avoid using setters for complex logic that may confuse users of your class.
4. Protect Helper Methods
If a method is only needed within the class itself (such as a helper function), make it private. This reduces the complexity of your public interface and ensures that outside code doesn’t rely on internal logic that may change.
Real-World Examples of Encapsulation
In real-world applications, encapsulation is essential for keeping your code clean, secure, and maintainable. Let’s explore some real-world scenarios where encapsulation plays a vital role:
1. Form Validation
When working with user input (like in a form), you don’t want the raw input data to be accessible or modifiable without validation. Encapsulation allows you to validate form fields before storing them in a database.
Example: Encapsulation in Form Validation
class UserForm {
#username;
#email;
// Setters with validation
setUsername(username) {
if (username.length >= 5) {
this.#username = username;
} else {
console.log("Username must be at least 5 characters long.");
}
}
setEmail(email) {
if (email.includes("@")) {
this.#email = email;
} else {
console.log("Invalid email address.");
}
}
// Getters to access validated fields
getUsername() {
return this.#username;
}
getEmail() {
return this.#email;
}
}
const form = new UserForm();
form.setUsername("john123"); // Valid username
form.setEmail("john@example.com"); // Valid email
console.log(form.getUsername()); // Output: john123
console.log(form.getEmail()); // Output: john@example.com
Explanation:
The
UserForm
class uses private fields (#username
and#email
) and controls how they are set through setters that validate the input.This ensures that only valid usernames and emails are stored inside the object, protecting your application from incorrect or harmful data.
Challenge – Build a Secure Login System
Now it’s your turn to apply what you’ve learned about encapsulation. Create a secure login system where a user’s password is stored in a private field, and the system can only validate the password through controlled methods.
Challenge Instructions:
Create a
User
class: This class should have private fields for theusername
andpassword
.Use a method to set the password: The password should be validated before it’s stored (e.g., it must be at least 8 characters long).
Add a method to validate the password: This method should check if the provided password matches the stored password.
Example:
class User {
#username;
#password;
constructor(username, password) {
this.#username = username;
this.setPassword(password); // Validate password during initialization
}
// Private method to validate password
#isValidPassword(password) {
return password.length >= 8;
}
// Setter with password validation
setPassword(password) {
if (this.#isValidPassword(password)) {
this.#password = password;
console.log("Password set successfully.");
} else {
console.log("Password must be at least 8 characters long.");
}
}
// Method to validate provided password
validatePassword(inputPassword) {
return inputPassword === this.#password;
}
}
const user = new User("johnDoe", "mySecretPassword123");
// Validate password
console.log(user.validatePassword("wrongPassword")); // Output: false
console.log(user.validatePassword("mySecretPassword123")); // Output: true
Explanation:
The
User
class uses a private field for the password and validates it before storing it.The
validatePassword()
method allows the user to check if the input matches the stored password, but the actual password remains hidden.
Unlocking the Power of Encapsulation
In this article, we’ve explored how encapsulation acts like a vault, protecting the internal state of objects and controlling how that state is accessed or modified. You’ve learned how to:
Use getters and setters to control access to private fields.
Implement private fields and private methods to ensure that sensitive data stays hidden.
Apply encapsulation principles to real-world scenarios like form validation and login systems.
Encapsulation helps you write cleaner, more secure, and more maintainable code by keeping important details hidden and only exposing what’s necessary.
Let’s tackle Advanced Classes Next!
In the next article, we’ll dive deeper into Advanced Classes, exploring topics like class hierarchies, method chaining, and other advanced features that will take your JavaScript skills to the next level.
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by