How to Use String.indent() to Left Indent Lines in Java.
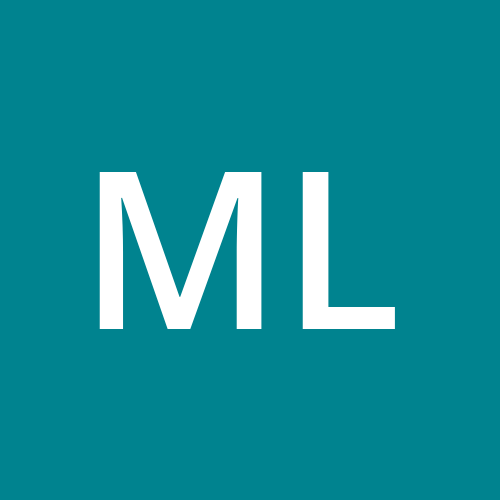
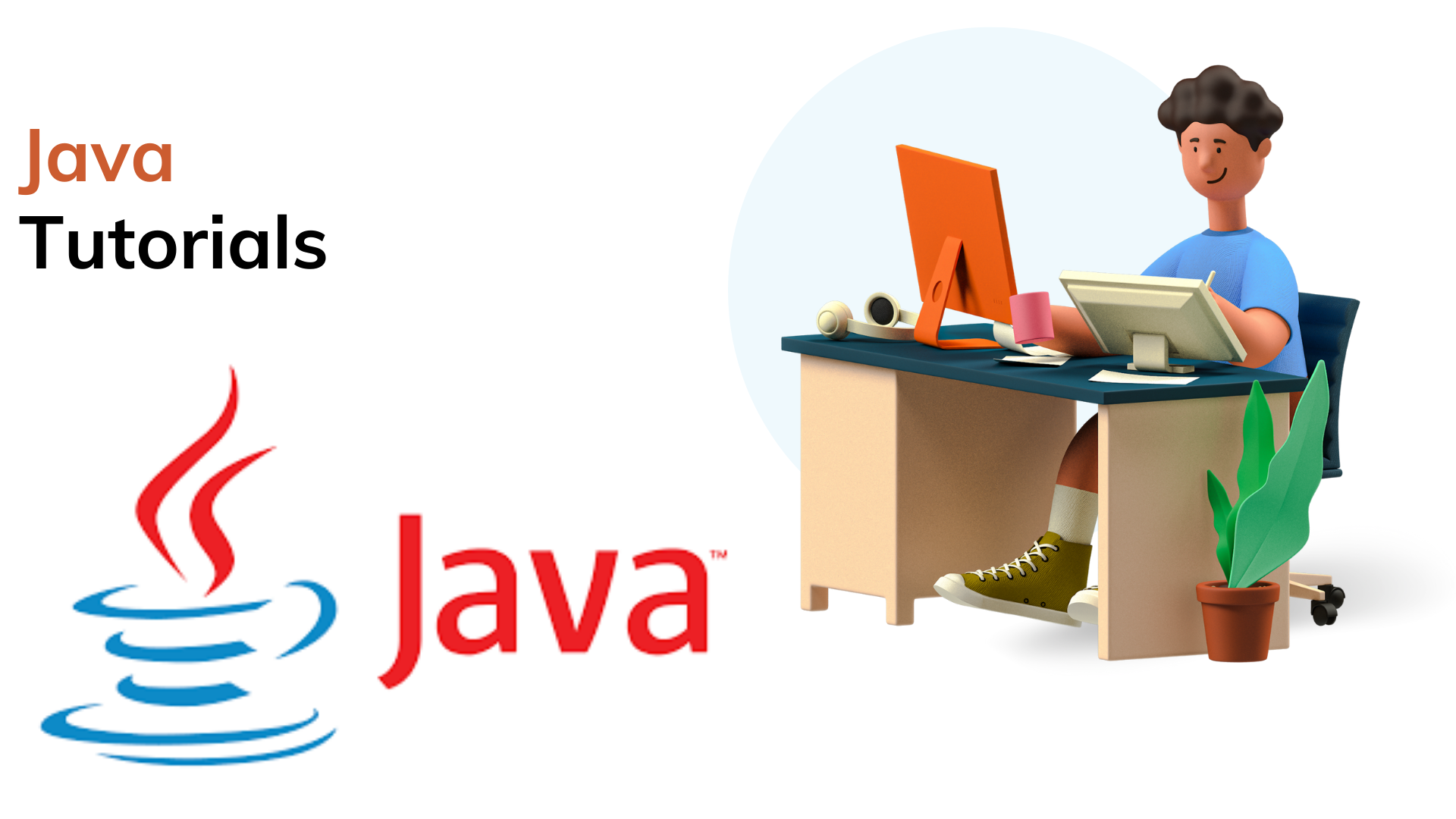
Java 12 introduced the String.indent()
method, a simple and convenient way to add or remove leading spaces from lines in a string. This method allows you to add left indentation to individual lines or entire blocks of text, making it especially useful for formatting output for logs, reports, and any multi-line string that requires consistent indentation.
In this article, we’ll cover how to use String.indent()
for left-indenting lines, its parameters, and practical examples to showcase its versatility.
What is String.indent()
?
The String.indent(int n)
method in Java takes an integer value n
as a parameter and adds n
spaces to the start of each line in a string. If n
is positive, it indents (or left-indents) each line with n
spaces. If n
is negative, it removes up to n
leading spaces from each line. If n
is zero, it keeps the original string unchanged.
This method is particularly useful for multi-line strings, such as formatted text blocks and logs, where you want consistent indentation across each line.
Syntax of String.indent()
The syntax for the indent
method is:
public String indent(int n)
Parameters:
n
: The number of spaces to add or remove. A positiven
adds spaces; a negativen
removes spaces.
Returns: A new string with the modified indentation for each line.
Basic Usage Examples
Example 1: Adding Left Indentation
Let’s add 4 spaces to each line of a multi-line string.
public class IndentExample {
public static void main(String[] args) {
String text = "Hello,\nThis is a sample text block.\nEnjoy learning Java!";
String indentedText = text.indent(4);
System.out.println(indentedText);
}
}
Output:
Hello,
This is a sample text block.
Enjoy learning Java!
Here, indent(4)
adds 4 spaces to each line in text
.
Example 2: Removing Indentation
You can also remove spaces by passing a negative value. For instance, if we have a text block already indented by 4 spaces, we can remove those spaces using indent(-4)
.
public class RemoveIndentExample {
public static void main(String[] args) {
String text = " Already indented line.\n Second indented line.";
String unindentedText = text.indent(-4);
System.out.println(unindentedText);
}
}
Output:
Already indented line.
Second indented line.
By using indent(-4)
, we strip away 4 leading spaces from each line.
Practical Applications of String.indent()
Application 1: Formatting Output in Logs
Suppose you’re generating logs with structured data, and you want to add indentation for readability. Using String.indent()
simplifies this process.
public class LogIndentExample {
public static void main(String[] args) {
String logMessage = "Error Code: 500\nMessage: Internal Server Error\nDetails: NullPointerException at line 42";
String indentedLog = logMessage.indent(4);
System.out.println("Log Output:\n" + indentedLog);
}
}
Output:
Log Output:
Error Code: 500
Message: Internal Server Error
Details: NullPointerException at line 42
This indentation helps distinguish log entries in a log file.
Application 2: Indenting Text Blocks for Console Output
In some applications, such as CLI (Command Line Interface) tools, it’s helpful to indent text for specific sections of output.
public class ConsoleOutputExample {
public static void main(String[] args) {
String instructions = "Usage:\n java MyApp [options]\n\nOptions:\n -h, --help Show help message\n -v, --version Show version information";
System.out.println("Application Instructions:\n" + instructions.indent(2));
}
}
Output:
Application Instructions:
Usage:
java MyApp [options]
Options:
-h, --help Show help message
-v, --version Show version information
This example shows how indent(2)
is used to make the CLI output more organized.
How Negative Indentation Works
If a line has fewer leading spaces than the number specified in a negative indent()
call, Java removes only as many spaces as available, leaving the line unaffected if there are no leading spaces.
public class NegativeIndentExample {
public static void main(String[] args) {
String text = " Line with two spaces\n Line with four spaces\nNo leading spaces";
String result = text.indent(-3);
System.out.println(result);
}
}
Output:
Line with two spaces
Line with four spaces
No leading spaces
In this example:
The first line has 2 spaces, so
indent(-3)
removes only those 2.The second line has 4 spaces, so
indent(-3)
removes 3, leaving 1.The third line is unaffected because it has no leading spaces.
Working with Multiline Strings
String.indent()
works well with multiline strings, including Java 13’s text blocks, which are useful for defining long, formatted strings.
public class TextBlockIndentExample {
public static void main(String[] args) {
String textBlock = """
This is line one.
This is line two.
This is line three.
""";
System.out.println("Indented Text Block:\n" + textBlock.indent(4));
}
}
Output:
Indented Text Block:
This is line one.
This is line two.
This is line three.
With text blocks, String.indent()
can help keep consistent indentation, especially when displaying structured text.
Limitations of String.indent()
Line-by-Line Basis:
String.indent()
operates on a line-by-line basis, so each line receives the specified indentation independently.No Complex Formatting: For complex formatting, such as varying indent levels across lines,
String.indent()
may not be suitable. Instead, you’d need a custom solution.Java 12+ Only:
String.indent()
is only available in Java 12 and later versions.
Conclusion
The String.indent()
method in Java provides a straightforward way to manage left indentation in multi-line strings. Whether you need to format log messages, console outputs, or large text blocks, this method makes it easy to add or remove spaces consistently. By using String.indent()
, you can significantly improve the readability and presentation of your Java program’s text output, ensuring that information is clear and well-organized.
More such articles:
https://www.youtube.com/@maheshwarligade
Subscribe to my newsletter
Read articles from Maheshwar Ligade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
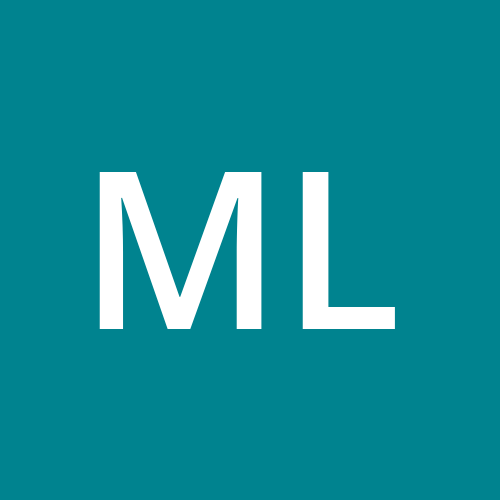
Maheshwar Ligade
Maheshwar Ligade
Learner, Love to make things simple, Full Stack Developer, StackOverflower, Passionate about using machine learning, deep learning and AI