Mastering setState in Flutter: When to Use It and When to Avoid It

Table of contents
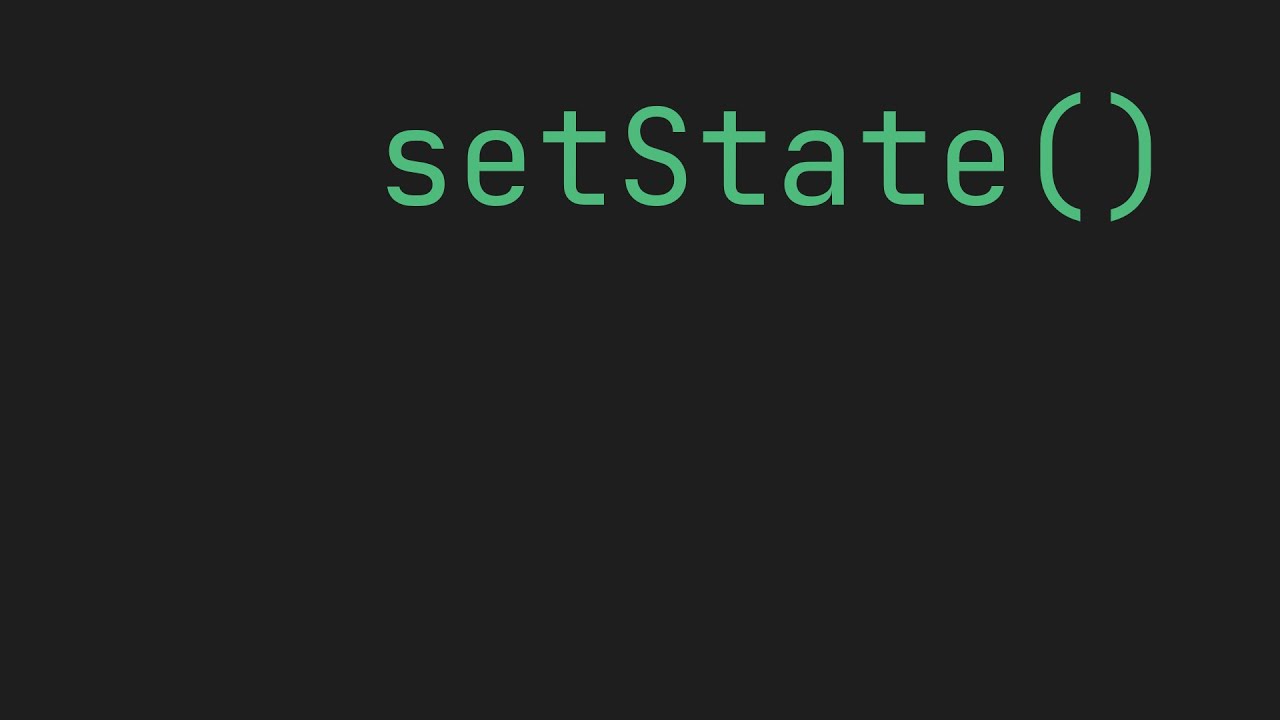
Understanding when to use setState
in Flutter is crucial for managing our app’s state effectively. Here’s a detailed guide:
When to use setState
?
- Updating the UI: Use
setState
when we need to update the UI in response to changes in the internal state of aStatefulWidget
. For example, if we have a counter that increments when a button is pressed, we will usesetState
to update the counter and refresh the UI.
void _incrementCounter() {
setState(() {
_counter++;
});
}
- User Interactions: It’s common to use
setState
in response to user interactions, such as button presses, form submissions, or other input events.
onPressed: () {
setState(() {
_isButtonPressed=true;
});
}
- State Changes: When the state of our widget changes and we need the UI to reflect these changes,
setState
is necessary. This includes changes in variables that are used in the build method.
void _toggleVisibility() {
setState(() {
_isVisible = !_isVisible;
});
}
When not to use setState
?
- Initialization: Do not use
setState
in theinitState
method. TheinitState
method is called only once when the widget is inserted into widget tree, and the state is already considered dirty at this point.
@override
void initState() {
super.initState();
// No need for setState here
_initializeData();
}
- Asynchronous Operations: Avoid using
setState
directly in asynchronous operations. Instead, complete the async operation first and then callsetState
to update the state.
void _fetchData() async {
final data = await fetchDataFromApi();
setState(() {
_data = data;
});
}
Performance Considerations: Be mindful of performance. Avoid calling
setState
in tight loops or frequently within a short period, as it can lead to performance issues. Only callsetState
when necessary to update the UI.Disposed Widgets: Never call
setState
after the widget has been disposed. This can lead to errors and unexpected behavior. Always check if the widget is still mounted before callingsetState
.
if (mounted) {
setState(() {
_data = newData;
});
}
Best Practices:
- Minimal Updates: Only wrap the state changes that require a UI update within
setState
. Avoid wrapping computational logic or asynchronous calls insidesetState
.
void _updateCounter() {
_counter++;
setState(() {}); // Only the state change is wrapped
}
- State Management: For complex state management, consider using state management solutions like Provider, Riverpod or Bloc instead of relying solely on
setState
.
By following the above guidelines, we can ensure that our Flutter app remains efficient and responsive. Happy Coding 🐦.
Subscribe to my newsletter
Read articles from Harish Kunchala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
