Understanding the Filter Method in JavaScript: A Simple Guide
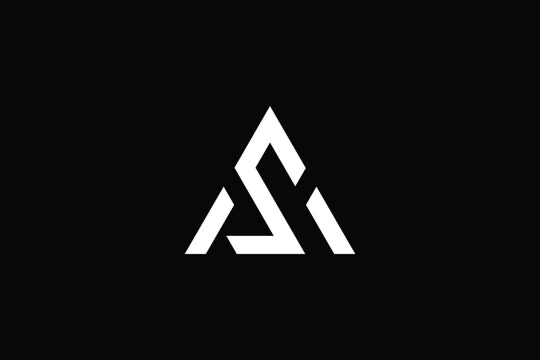
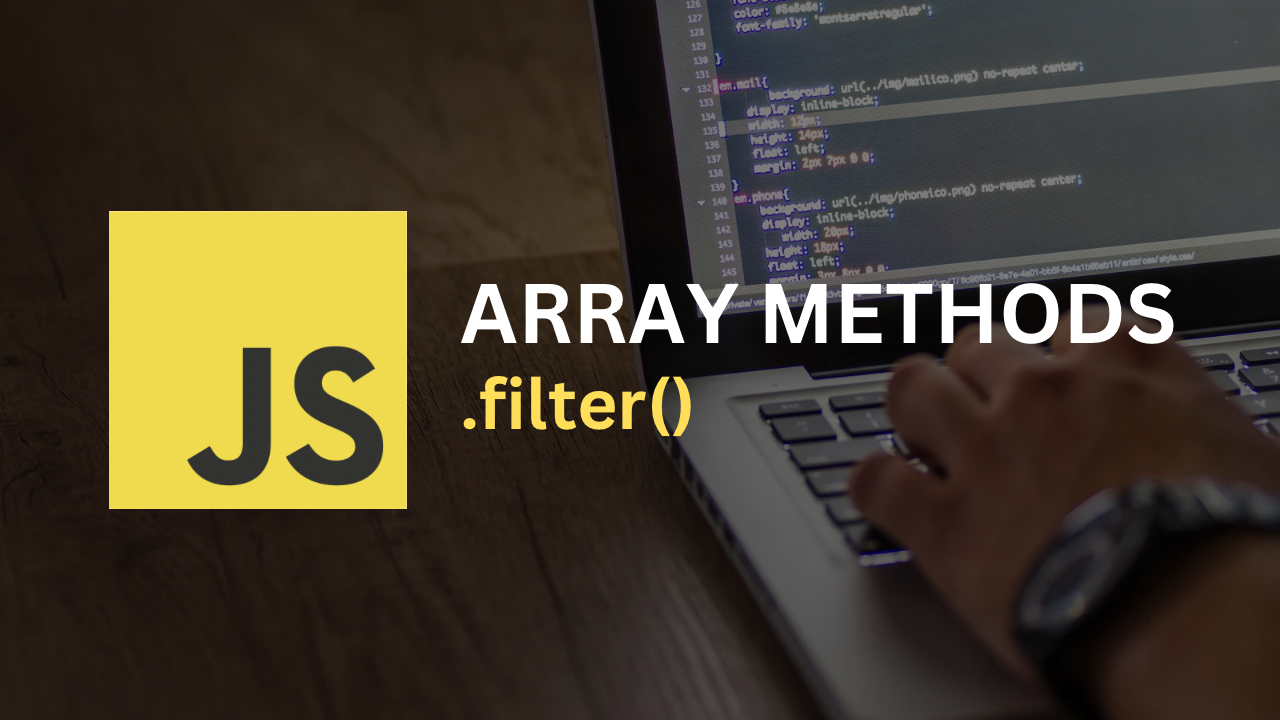
Hello everyone,
Welcome to code invasion,
In this tutorial we will learn how the filter method in JavaScript works,
The filter method in JavaScript is an array method that creates a sub array of elements that satisfies a given condition from an original array.
Let’s take a few examples to understand it better,
let numbers = [1,2,3,4,5,6];
If we have an array of numbers and we want only the even numbers in this array. We can achieve this by using the array.filter()
method to filter the even numbers.
To do this,
We’ll start by calling the filter method on our array by typing numbers.filter()
.
It is very important to keep in mind that the filter method returns an array. We can store the array returned by the filter method in a variable. In this case we will call our variable evenNumbers.
const evenNumbers = numbers.filter()
The filter method accepts a test function which it executes on each element in the array. If an element passes the test it is returned in by the filter method. If an element fails the test it is ignored by the filter method.
Let’s declare this function and call it test
const multiples = numbers.filter()
function test () {}
Under the hood, when we call the filter method on an array, it loops over the array and executes the function on every element in the array. During the loop the filter method automatically provides the current element, the index of the current element and the array as parameters to the test function passed into it. In most instances we would use only the element.
We can receive the element as a parameter and in our function body we would check to see if the element satisfies the condition.
const evenNumbers = numbers.filter()
function test (element) {
return element % 2 === 0
}
Now all that’s left is to pass the test function into our filter method.
After that we will log our evenNumbers variable to the console to see the results
const evenNumbers = numbers.filter(test)
function test (element) {
return element % 2 === 0
}
console.log(evenNumbers)
If we run our file in the terminal using node, we’ll see the array [2,4,6] logged to the console. You can run your file in the terminal using node by typing ‘node’ followed by your file name.
Alternatively, we could move our function declaration into the filter method and this would still work perfectly. Let’s try it and see the results…
numbers.filter(function test(element) {
return element % 2 === 0
})
let’s run our file with node index.js
to see the results… and there we have it!
we have our sub array which satisfy the given condition logged to the console.
Using arrow functions
NB: The more modern way of writing a the test function in the filter method is to use arrow functions.
An arrow function is a concise way to write functions in JavaScript without the function keyword.
To do this we’ll simply write our function without the function statement like this….
const evenNumbers = numbers.filter(() => {})
Recall that the filter method automatically provides the current element, the index of the current element and the array as parameters to the function passed into it. In this case we would use only the element.
So in our arrow function we’ll accept the current element the filter method provides as a parameter. In our function, we’ll check if the element satisfies the condition.
const evenNumbers = numbers.filter((element) => {
return element % 2 === 0
})
console.log(evenNumbers)
And if we run our code in the terminal we have our sub array which satisfy the given condition logged to the console.
Example 2
Now another use case could be when we have an array of objects describing books and their prices.
let books = [
{ name titlt
If we want to filter out the books that cost below $50 we could simply do this using the filter method.
We’ll start by calling our filter method on the array.
We’ll then define the function which we’ll use to check if a book satisfies the criteria. We will use the element value provided by the filter method.
Using the dot notation we will check if our element prices are greater than 50.
const books = books.filter((book) => {
return book.price > 50
})
Let’s run our file with node to see the results.
Boom 💥 there we have it, the sub array of books that satisfy our condition.
The filter method in JavaScript is an array method that filters an array based on a specific criteria.
If you enjoyed this tutorial hit the like button, follow the page for more javascript and web development tutorial.
Subscribe to my newsletter
Read articles from Salawu Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
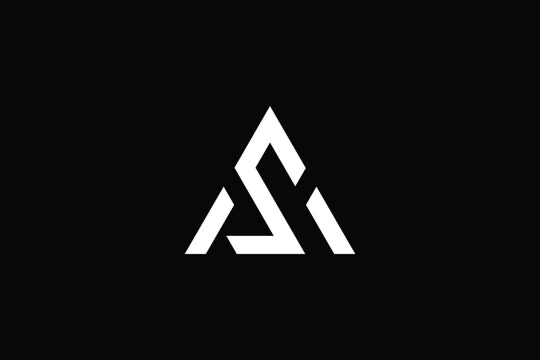