Create Your API
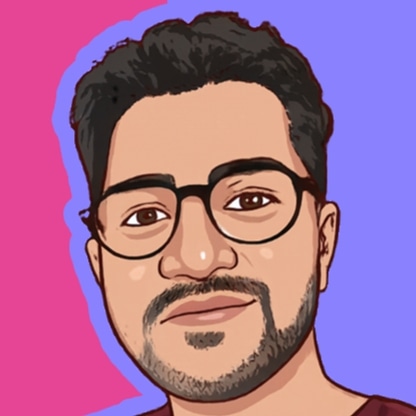
APIs (Application Programming Interfaces) are the backbone of modern software development, enabling applications to communicate and share data efficiently. Whether you're building a project or looking to level up your development skills, creating your API is a fundamental milestone.
In this blog, we'll explore how to create a simple RESTful API from scratch using Node.js and Express.js, two popular tools for backend development.
Step 1: Initialize Your Project
Create a new project folder and navigate into it:
mkdir my-api cd my-api
Initialize a new Node.js project:
npm init -y
This creates a
package.json
file to manage your project dependencies.
Step 2: Install Dependencies
We'll use Express
for building the API and nodemon
for easier development.
npm install express
npm install --save-dev nodemon
Update the package.json
file to use nodemon
for running the project. Add this to the scripts
section:
"scripts": {
"start": "nodemon index.js"
}
Step 3: Set Up the Basic API Structure
Create a file named
index.js
:touch index.js
Add the following code to
index.js
to set up a basic Express server:const express = require("express"); const app = express(); app.use(express.json()); // For parsing JSON requests const PORT = 3000; app.listen(PORT, () => { console.log(`Server is running on http://localhost:${PORT}`); });
Run your server:
npm start
Visit http://localhost:3000 in your browser or a tool like Postman to verify that the server is running.
Step 4: Create Your First API Endpoint
Let’s create a simple GET
endpoint:
Modify
index.js
to include the following route:app.get("/", (req, res) => { res.send("Welcome to My API!"); });
Restart the server (
nodemon
should auto-restart if running). Open http://localhost:3000 in your browser, and you should see the message "Welcome to My API!".
Step 5: Build a CRUD API
To demonstrate API capabilities, let’s build a CRUD (Create, Read, Update, Delete) API for managing "users."
Create an in-memory data store (array):
const users = [ { id: 1, name: "Alice" }, { id: 2, name: "Bob" }, ];
Add routes for each CRUD operation:
// Get all users app.get("/users", (req, res) => { res.json(users); }); // Get a user by ID app.get("/users/:id", (req, res) => { const user = users.find(u => u.id === parseInt(req.params.id)); if (!user) return res.status(404).send("User not found."); res.json(user); }); // Add a new user app.post("/users", (req, res) => { const user = { id: users.length + 1, name: req.body.name }; users.push(user); res.status(201).json(user); }); // Update a user app.put("/users/:id", (req, res) => { const user = users.find(u => u.id === parseInt(req.params.id)); if (!user) return res.status(404).send("User not found."); user.name = req.body.name; res.json(user); }); // Delete a user app.delete("/users/:id", (req, res) => { const index = users.findIndex(u => u.id === parseInt(req.params.id)); if (index === -1) return res.status(404).send("User not found."); const deletedUser = users.splice(index, 1); res.json(deletedUser); });
Test the API using Postman or a similar tool. Try the following requests:
GET /users
to fetch all users.GET /users/:id
to fetch a specific user.POST /users
with a JSON body like{"name": "Charlie"}
to add a user.PUT /users/:id
with a JSON body like{"name": "David"}
to update a user.DELETE /users/:id
to delete a user.
Step 6: Error Handling and Validation
Improve the API by adding error handling and input validation:
Install the
joi
library:npm install joi
Update the
POST
andPUT
routes to validate input:const Joi = require("joi"); const validateUser = (user) => { const schema = Joi.object({ name: Joi.string().min(3).required(), }); return schema.validate(user); }; app.post("/users", (req, res) => { const { error } = validateUser(req.body); if (error) return res.status(400).send(error.details[0].message); const user = { id: users.length + 1, name: req.body.name }; users.push(user); res.status(201).json(user); }); app.put("/users/:id", (req, res) => { const user = users.find(u => u.id === parseInt(req.params.id)); if (!user) return res.status(404).send("User not found."); const { error } = validateUser(req.body); if (error) return res.status(400).send(error.details[0].message); user.name = req.body.name; res.json(user); });
Step 7: Deploy Your API
Once your API is ready, deploy it to a platform like Render, Vercel, or Heroku.
Push your code to a GitHub repository.
Follow the deployment instructions for your chosen platform.
You’ve built and deployed a fully functional RESTful API. This API can now serve as the backend for your web or mobile applications!
Happy coding 🚀
Do you have questions or feedback? Drop a comment below, or connect with me on LinkedIn and GitHub
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
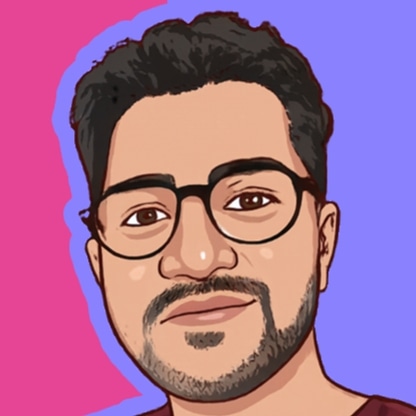
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠