Understanding Insertion Sort: A Simple Yet Powerful Sorting Algorithm
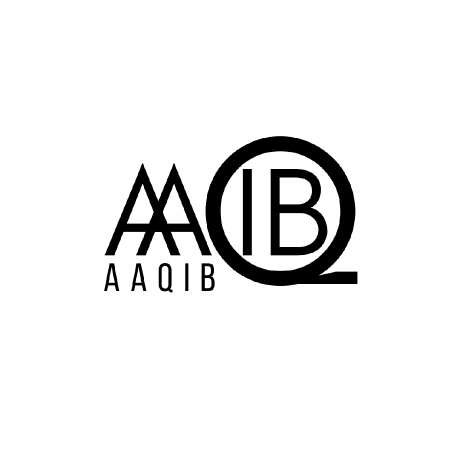
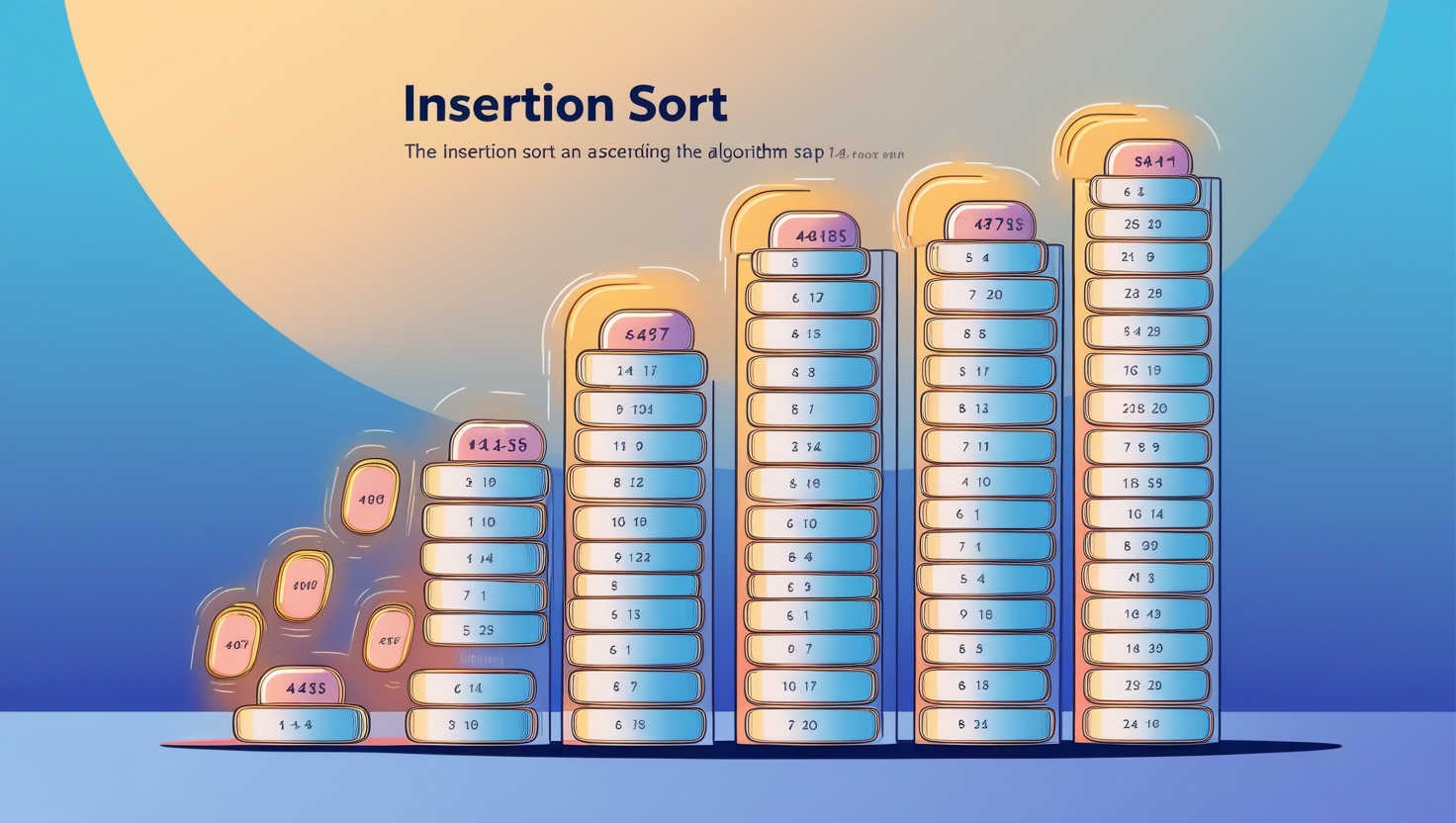
Sorting is an essential part of computer science. Among the various sorting algorithms, Insertion Sort stands out due to its simplicity and effectiveness for small datasets. This blog will walk you through the mechanics of Insertion Sort and its implementation.
What is Insertion Sort?
Insertion Sort is a straightforward comparison-based algorithm. It works by dividing the array into two parts:
A sorted section (built element by element).
An unsorted section.
The algorithm takes elements from the unsorted part one at a time and places them in the correct position in the sorted part.
How Does Insertion Sort Work?
Here’s a step-by-step breakdown:
Start with the second element (since a single element is already "sorted").
Compare this element with those before it in the sorted section.
Shift the larger elements in the sorted section to the right to make room for the current element.
Insert the current element into its correct position.
Repeat this process for all elements in the unsorted section.
Visual Representation of Insertion Sort
Let’s sort the array [5, 3, 8, 6]
using Insertion Sort:
Initial Array:
[5, 3, 8, 6]
The first element,5
, is already sorted.Step 1: Compare
3
with5
.
Since3 < 5
, shift5
to the right and place3
in the first position.
Array after Step 1:[3, 5, 8, 6]
Step 2: Compare
8
with5
.
Since8 > 5
, no shifting is needed.
Array after Step 2:[3, 5, 8, 6]
Step 3: Compare
6
with8
.
Since6 < 8
, shift8
to the right.
Compare6
with5
. Since6 > 5
, place6
in its correct position.
Array after Step 3:[3, 5, 6, 8]
Sorted Array:
[3, 5, 6, 8]
InsertionSort(array)
for i from 1 to array.length - 1
key = array[i]
j = i - 1
while j >= 0 and array[j] > key
array[j + 1] = array[j]
j = j - 1
array[j + 1] = key
Time and Space Complexity
Best Case: O(n) – When the array is already sorted.
Worst Case: O(n²) – When the array is sorted in reverse order.
Space Complexity: O(1) – No additional memory is required.
Why Use Insertion Sort?
Simple to Implement: Ideal for educational purposes.
Efficient for Small Datasets: Works well when the array size is small.
Adaptive: Performs well on nearly sorted data.
When to Avoid Insertion Sort?
For large datasets or when performance is critical, more advanced algorithms like Merge Sort, Quick Sort, or Heap Sort are preferred due to their better time complexity.
Conclusion
Insertion Sort is a simple yet effective sorting algorithm, especially for small datasets or nearly sorted arrays. By understanding its working, you’ll not only appreciate its simplicity but also lay a strong foundation for learning more complex algorithms.
Subscribe to my newsletter
Read articles from Aaqib Bashir Mir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
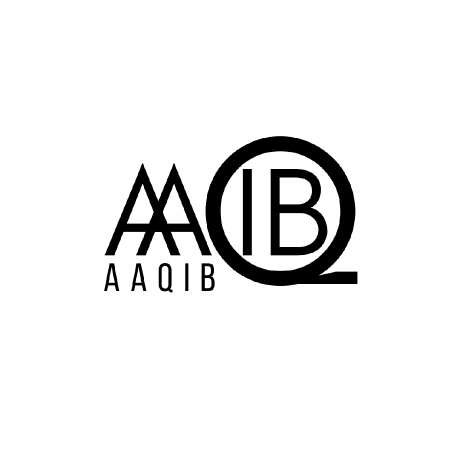