Enhance Azure Traffic Manager with Terraform: Leveraging Data Sources

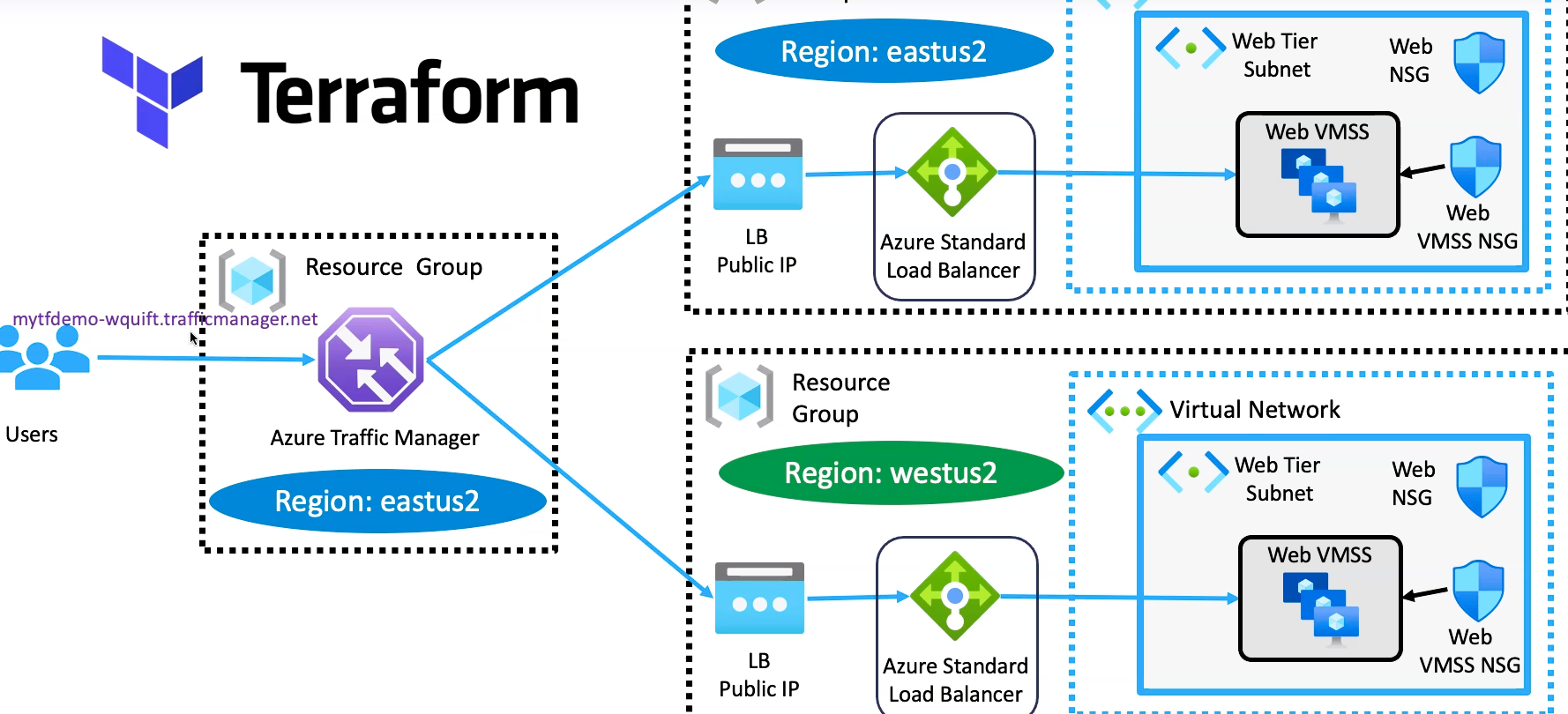
Azure Traffic Manager is a robust DNS-based traffic load balancer that enables you to distribute network traffic efficiently across multiple regions. Imagine a scenario where you've deployed both web and application servers in various regions to ensure high availability and disaster recovery. Each of these servers is fronted by Azure Load Balancers with public IPs exposed to handle incoming traffic. To optimize the distribution of traffic among these multiple regions and ensure seamless failover in case of regional outages, configuring Azure Traffic Manager is essential.
By integrating Azure Traffic Manager with your Terraform Infrastructure as Code (IaC) setup, you can automate the deployment and management of traffic routing policies, enhancing both the performance and resilience of your applications. This approach not only simplifies the configuration process but also ensures consistency and repeatability across your infrastructure deployments.
Terraform Remote State Datasource
Terraform remote state data source retrieves the output values of the root module from some other terraform configuration using the latest state snapshot from the remote backend.
Use Case: Terraform Remote State Data Source in Azure Traffic Manager Setup
In this project, we are deploying web servers and app servers in two different Azure regions, each fronted by its own Load Balancer with a publicly exposed IP address. To ensure seamless load balancing across these regions, we will use Azure Traffic Manager, which will reside in a separate Terraform project.
How Remote State Data Source is Used
Regional Load Balancers: Each region’s Load Balancer is provisioned within its respective Terraform configuration.
Azure Traffic Manager Configuration: Azure Traffic Manager, in a separate Terraform configuration, retrieves the public IPs of these Load Balancers using the Terraform Remote State Data Source.
Traffic Balancing: Azure Traffic Manager distributes traffic between the regions based on its configured traffic-routing method (e.g., performance, priority, or geographic).
Key Benefits of this Approach
Seamless Integration: The remote state data source eliminates manual intervention by dynamically fetching the required values.
Decoupled Configurations: Load Balancers and Azure Traffic Manager are managed in separate configurations, enhancing modularity and maintainability.
Efficient Failover and Load Balancing: With Azure Traffic Manager, traffic is intelligently routed to the most suitable region based on configuration, improving application availability and performance.
we manage two distinct Terraform projects, each responsible for deploying infrastructure in a separate Azure region. These projects generate independent state files to represent the deployed resources in their respective regions i.e. eastus2 and westus2
As we have provisioned 2 identical infrastructures in their respective regions. Now the Remote State Datastore will refer to both the state files present in the storage container when and wherever required.
Configure Remote State Datasource
The Remote State Data Source enables seamless integration across multiple Terraform projects. In this case, it will be used while creating the Azure Traffic Manager to establish connectivity between servers deployed in two different regions.
Project-1 Data Source (EASTUS2)
Retrieves the Terraform state for resources deployed in the EASTUS2 region.
Key configuration parameters include the storage account, resource group, and state file path.
# Project-1 Datasource(EASTUS2)
data "terraform_remote_state" "project1_eastus" {
backend = "azurerm"
config = {
storage_account_name = "terraformstatestore0"
resource_group_name = "terraform-storageAcc-rg"
container_name = "tfstatefiles"
key = "project-1-eastus2-terraform.tfstate"
subscription_id = "xxxxxxx-xxxxxxxx-xxxxx"
}
}
# Project-2 Datasource(WESTUS)
data "terraform_remote_state" "project2_westus" {
backend = "azurerm"
config = {
storage_account_name = "terraformstatestore0"
resource_group_name = "terraform-storageAcc-rg"
container_name = "tfstatefiles"
key = "project-2-westus2-terraform.tfstate"
subscription_id = "xxxx-xxxxxxxxxxx-xxxxxxx"
}
}
Project-2 Data Source (WESTUS)
Retrieves the Terraform state for resources deployed in the WESTUS region.
Similar parameters are defined to connect to the remote backend.
These data sources allow the Azure Traffic Manager to fetch public IPs from regional Load Balancers dynamically. Projects are managed separately, reducing complexity and enhancing modularity.
Important Consideration Before Implementing Azure Traffic Manager
Before implementing Azure Traffic Manager with the azurerm_traffic_manager_azure_endpoint
resource, ensure that the domain_name_label
attribute is defined in the Load Balancer Public IP. This is a necessary step for creating the Fully Qualified Domain Name (FQDN). Without this, Traffic Manager will not be able to resolve the FQDN correctly.
If the domain_name_label
is not set or cannot be used, you will need to proceed with the azurerm_traffic_manager_external_endpoint
resource, which allows you to use external endpoints with direct IP addresses instead of relying on the FQDN.
Provision Azure Traffic Manager
For provisioning Azure Traffic Manager via Terraform we will require the following terraform resource, respective input variable, and most importantly above defined Data Sources.
azurerm_traffic_manager_profile
azurerm_traffic_manager_azure_endpoint
# Resorce1: Traffic Manager Profile
resource "azurerm_traffic_manager_profile" "tm_profile" {
name = "tmprofile-${random_string.random_name.id}" # Name has to be unique across azure cloud
resource_group_name = azurerm_resource_group.rg.name
traffic_routing_method = "Weighted"
dns_config {
relative_name = "tmprofile-${random_string.random_name.id}"
ttl = 100
}
monitor_config {
protocol = "http"
port = 80
path = "/"
interval_in_seconds = 30
timeout_in_seconds = 9
tolerated_number_of_failures = 3
}
tags = local.common_tags
}
# Traffic Manager Endpoint - Project-1-EastUs2
resource "azurerm_traffic_manager_azure_endpoint" "tm_endoint_project1_eastus2" {
name = "tm-project1-eastus2"
profile_id = azurerm_traffic_manager_profile.tm_profile.id
weight = 50
target_resource_id = data.terraform_remote_state.project1_eastus.outputs.web_lb_public_ip_id # This is being refered from Remote datasource
}
# Traffic Manager Endpoint - Project-2-WestUs2
resource "azurerm_traffic_manager_azure_endpoint" "tm_endoint_project1_westus2" {
name = "tm-project1-westus2"
profile_id = azurerm_traffic_manager_profile.tm_profile.id
weight = 50
target_resource_id = data.terraform_remote_state.project2_westus.outputs.web_lb_public_ip_id # This is being refered from Remote datasource
}
1. Traffic Manager Profile
The azurerm_traffic_manager_profile
resource defines the Traffic Manager instance. The profile manages traffic routing across regions using the Weighted Routing method, which distributes traffic based on assigned weights.
Key Configurations:
Name: A unique name for the profile (uses
random_string
to ensure uniqueness globally).Traffic Routing Method: Defines the logic for routing traffic; in this case, Weighted is used to balance traffic based on specified weights.
DNS Config: Sets up the DNS entry for Traffic Manager with a time-to-live (TTL) value of 100 seconds.
Monitor Config: Configures health monitoring for endpoints, including the HTTP protocol, port, health check path, and failure tolerances.
2. Traffic Manager Endpoints
The endpoints in Traffic Manager represent the target resources (e.g., Load Balancers) that handle traffic in specific regions. Terraform’s azurerm_traffic_manager_azure_endpoint
resource creates these connections.
Endpoint for Project-1 (EastUS2)
Represents the Load Balancer in the EastUS2 region.
Uses Terraform’s Remote State Datasource to retrieve the public IP of the Load Balancer (
web_lb_public_ip_id
) dynamically from the state file of the respective project.Assigned a weight of 50, which defines the proportion of traffic routed to this endpoint.
Endpoint for Project-2 (WestUS)
Represents the Load Balancer in the WestUS region.
Similarly, uses Remote State Datasource to fetch the public IP (
web_lb_public_ip_id
) from the state file of Project-2.Assigned an equal weight of 50 for traffic distribution.
How It Works
Traffic Manager Profile:
Acts as a global entry point for incoming traffic.
DNS configuration ensures that the Traffic Manager routes requests efficiently based on health and weight.
Remote State Integration:
Fetches real-time public IPs of Load Balancers deployed in different projects (EastUS2 and WestUS).
Enables seamless integration of regional resources without hardcoding IP addresses.
Load Balancing Logic:
The Weighted Routing method splits traffic evenly (50:50) between the two regions, ensuring high availability.
Health monitoring ensures only healthy endpoints receive traffic.
Traffic Manager Deployment Verification
The Azure Traffic Manager has been successfully deployed, and its Fully Qualified Domain Name (FQDN) is now available. The FQDN of the Traffic Manager profile can be accessed as shown below:
Additionally, we have verified the accessibility of the Traffic Manager's FQDN, confirming that traffic is being routed properly across the configured endpoints. The successful access test is shown below:
This verifies that the Traffic Manager is working as expected, balancing the traffic between the specified endpoints based on the configuration.
Verification on Azure Portal
Subscribe to my newsletter
Read articles from Ritesh Kumar Nayak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ritesh Kumar Nayak
Ritesh Kumar Nayak
Passionate about helping organizations build scalable infrastructure and DevOps solutions with cloud technologies. Experienced in designing robust systems, automating processes, and driving efficiency through innovative cloud solutions. Advocate for best practices in DevOps and cloud computing, committed to enabling teams to achieve their full potential.