Understanding the 'This' Keyword in JavaScript: A Beginner's Guide
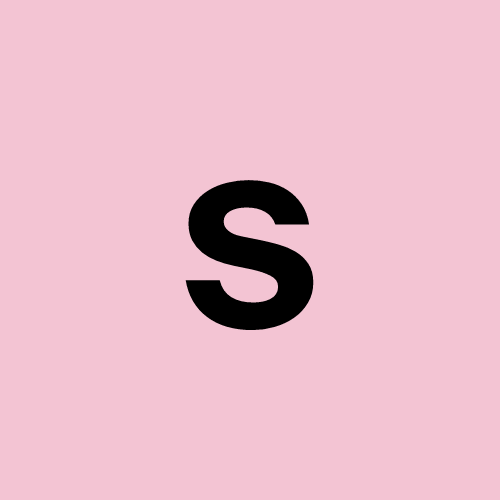
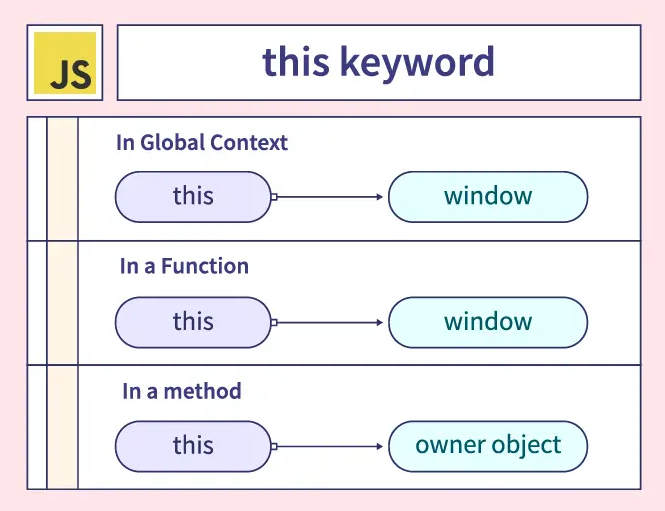
In JavaScript, the this
keyword is a crucial concept that refers to the object that is currently executing or calling a function. Its value is determined by the context in which the function is invoked, making it dynamic and versatile.
Contexts of this
Global Context: When
this
is used in the global scope (outside any function), it refers to the global object. In a web browser, this is typically thewindow
object. For example:console.log(this); // Outputs: Window {...}
Function Context: In a regular function,
this
refers to the global object when not in strict mode. However, in strict mode,this
isundefined
. For instance:function showThis() { console.log(this); } showThis(); // Outputs: Window {...} (non-strict mode)
Object Method: When a function is called as a method of an object,
this
refers to that object. For example:const obj = { name: 'Alice', greet() { console.log(`Hello, ${this.name}`); } }; obj.greet(); // Outputs: Hello, Alice
Event Handlers: In event handlers,
this
refers to the element that triggered the event:document.getElementById('myButton').addEventListener('click', function() { console.log(this); // Refers to the button element });
Explicit Binding: JavaScript provides methods like
call()
,apply()
, andbind()
to explicitly set the value ofthis
. For example:function greet() { console.log(`Hello, ${this.name}`); } const user = { name: 'Bob' }; greet.call(user); // Outputs: Hello, Bob
Conclusion
Understanding how this
works in different contexts is essential for effective JavaScript programming. It allows developers to manipulate objects dynamically and access their properties and methods efficiently. The principles of using the this
keyword are foundational in building complex applications like the Hexahome platform, where context-driven behavior is vital for user interactions and functionality.
Subscribe to my newsletter
Read articles from seo2 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
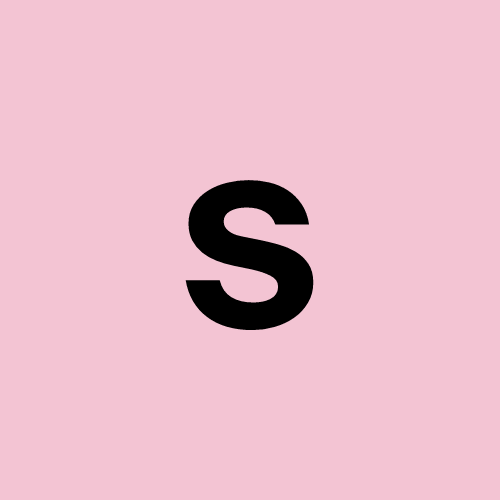