A Beginner's Guide to Version Control and Collaboration

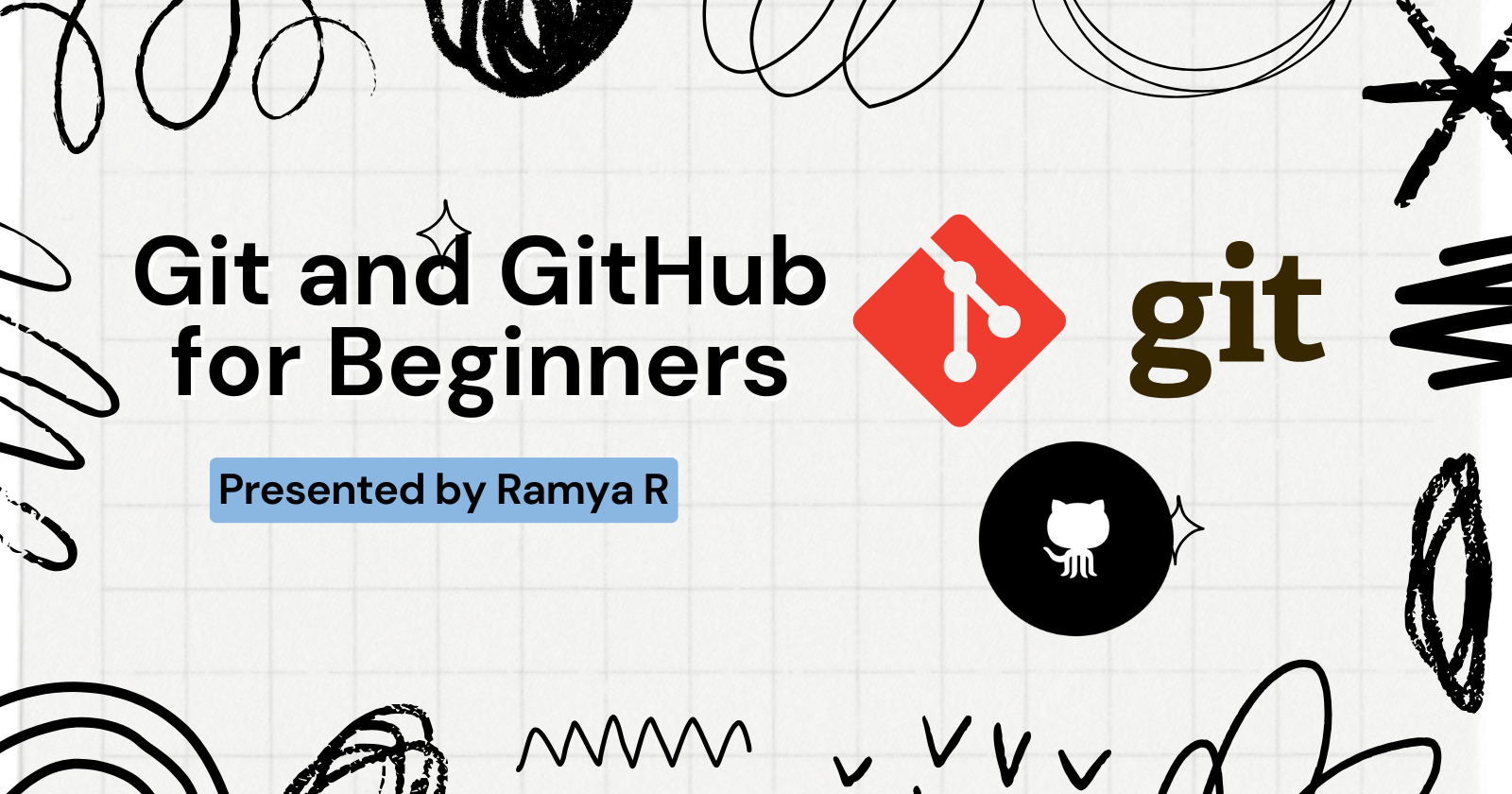
Welcome to my journey as a new DevOps engineer! One of the first things I learned as I started diving into DevOps practices was version control, particularly using Git and GitHub. Git is an essential tool for managing changes to your codebase, while GitHub provides a collaborative platform for sharing and versioning your projects.
In this post, I’ll walk you through the basic Git commands needed to create repositories on GitHub, manage documents, and collaborate with others. I'll also provide examples so you can follow along!
1. Setting Up Git
Before you can start using Git, you need to install it on your machine. You can download it from the official Git website. After installation, you need to configure your name and email address to ensure your commits are correctly attributed to you.
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Now you're ready to start using Git!
2. Creating a Local Git Repository
You can create a new local repository in two ways:
Option 1: Initialize a New Repository
Navigate to the folder where you want your repository and run:
git init
This command creates a new .git
directory, making it a Git repository. Now you can start tracking changes within this folder.
Option 2: Clone an Existing Repository
If you want to clone a repository from GitHub (or any other remote), you can use the git clone
command:
git clone https://github.com/username/repository-name.git
This will create a copy of the remote repository on your local machine.
3. Connecting to GitHub
Now that you have a local repository, let's connect it to GitHub. If you don’t already have a GitHub account, go ahead and create one here.
To link your local repository to a GitHub repository:
Create a new repository on GitHub by clicking the "New" button on your GitHub dashboard.
Copy the URL of the repository (it should look something like
https://github.com/username/repository-name.git
).Run the following command in your local Git repository to add the remote:
git remote add origin https://github.com/username/repository-name.git
This tells Git where to push and pull updates for your project.
4. Basic Git Commands
Now let's cover the basic Git commands you'll need to manage your project:
git init
As mentioned earlier, git init
is used to initialize a new Git repository in your project folder.
git init
git add
After you make changes to your files, you need to "stage" them before committing. You can add specific files or all files in the directory.
git add filename.txt # Add a specific file
git add . # Add all changed files
git commit
Once you've staged your changes, commit them to your local repository. A commit is like a snapshot of your project at a certain point in time.
git commit -m "Commit message describing changes"
Make sure your commit message is descriptive and concise.
git push
To upload your changes to the remote repository on GitHub, use git push
. If you’re pushing for the first time, you may need to specify the branch (usually main
or master
):
git push origin main
git pull
To pull the latest changes from a remote repository (useful when collaborating with others), use:
git pull origin main
This command fetches the updates from GitHub and merges them into your local repository.
git clone
If you want to copy an existing GitHub repository to your local machine, use git clone
:
git clone https://github.com/username/repository-name.git
1. What is Git Branching?
At its core, Git branching allows you to diverge from the main line of development and work on a different version of your code in parallel. Think of it like creating a new path on a project while keeping the original path intact. Branching helps you isolate changes to specific features, bug fixes, or experiments without affecting the main project.
Main branch (often called
main
ormaster
): This is the default branch that contains the production-ready code.Feature branches: These branches are created to work on new features or changes to the project.
Bugfix branches: These branches are dedicated to fixing issues or bugs in the codebase.
Example Scenario:
Imagine you’re working on a project and want to add a new feature. Instead of directly modifying the main
branch, you create a new branch where you can make changes without disrupting the main project.
2. How to Create and Work with Branches
Here’s how you can create and manage branches in Git:
Creating a Branch
To create a new branch, use the git branch
command:
git branch new-feature
This command creates a branch named new-feature
but doesn't switch to it. To both create and switch to the branch, use:
git checkout -b new-feature
Switching Between Branches
To switch to an existing branch, use the git checkout
command:
git checkout new-feature
After switching, any changes you make will be isolated to this branch.
Deleting a Branch
Once you’re done with a branch (e.g., after merging your changes), you may want to delete it. Use the following command to delete a branch locally:
git branch -d new-feature
If the branch hasn’t been merged, Git will warn you. If you want to force the deletion, you can use -D
.
3. What is Git Merging?
Once you’ve finished working on a feature or bug fix in a branch, you’ll want to bring those changes back into the main codebase. Git merging is the process of taking the changes from one branch and integrating them into another. Merging helps to bring together all of the work done in separate branches, so the project is up-to-date with the latest changes.
In most cases, merging is done from a feature branch into the main
branch.
4. How to Merge Branches
Now, let’s walk through how to merge a branch into the main
branch.
Step 1: Switch to the main
Branch
Before merging, make sure you’re on the branch that you want to merge changes into (typically main
):
git checkout main
Step 2: Merge the Feature Branch
To merge the new-feature
branch into main
, use:
git merge new-feature
This will bring the changes from the new-feature
branch into your main
branch. If there are no conflicts, the merge will happen automatically, and your working directory will be updated with the changes from both branches.
5. Resolving Merge Conflicts
Sometimes, Git cannot automatically merge changes, especially if the same lines in a file were modified in both branches. This is called a merge conflict. When this happens, Git will pause the merge and ask you to resolve the conflict manually.
How to Resolve Merge Conflicts:
Git will mark the conflict in the file by adding conflict markers:
<<<<<<< HEAD // changes from the current branch (e.g., main) ======= // changes from the branch being merged (e.g., new-feature) >>>>>>> new-feature
Open the file and edit the content, choosing which changes to keep or how to combine them.
After resolving the conflict, remove the conflict markers and save the file.
Add the resolved file to the staging area:
git add resolved-file.txt
Complete the merge with a commit:
git commit
This will finalize the merge, and the conflict will be resolved.
6. Best Practices for Git Branching and Merging
To work efficiently with Git, especially in team environments, it’s important to follow some best practices for branching and merging:
Use descriptive names for branches: Name your branches based on the feature or fix you’re working on (e.g.,
feature/login-page
,bugfix/fix-navbar
).Merge often: Regularly merge the latest changes from the
main
branch into your feature branches to avoid conflicts.Create pull requests (PRs): If you’re working in a team, use pull requests to review code before merging it into the
main
branch. This ensures that everyone is on the same page and that the code is reviewed before being integrated.Keep commits small and focused: Make sure each commit is focused on a single task or change. This makes reviewing and understanding changes much easier.
Thank you for reading :)
Ramya R
Subscribe to my newsletter
Read articles from Ramya R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ramya R
Ramya R
Hello, I'm Ramya! 👩💻 I'm a passionate tech enthusiast on a journey to build a thriving career in Cloud Computing and DevOps. 🌩️⚙️ I strongly believe in learning through mistakes and growing together as a community. 🌱 By documenting my tech journey, I aim to track my personal and professional growth while inspiring others to embrace their learning paths. Let’s connect, share, and grow in this ever-evolving world of technology! 🚀