Spring Boot Essentials: Basics

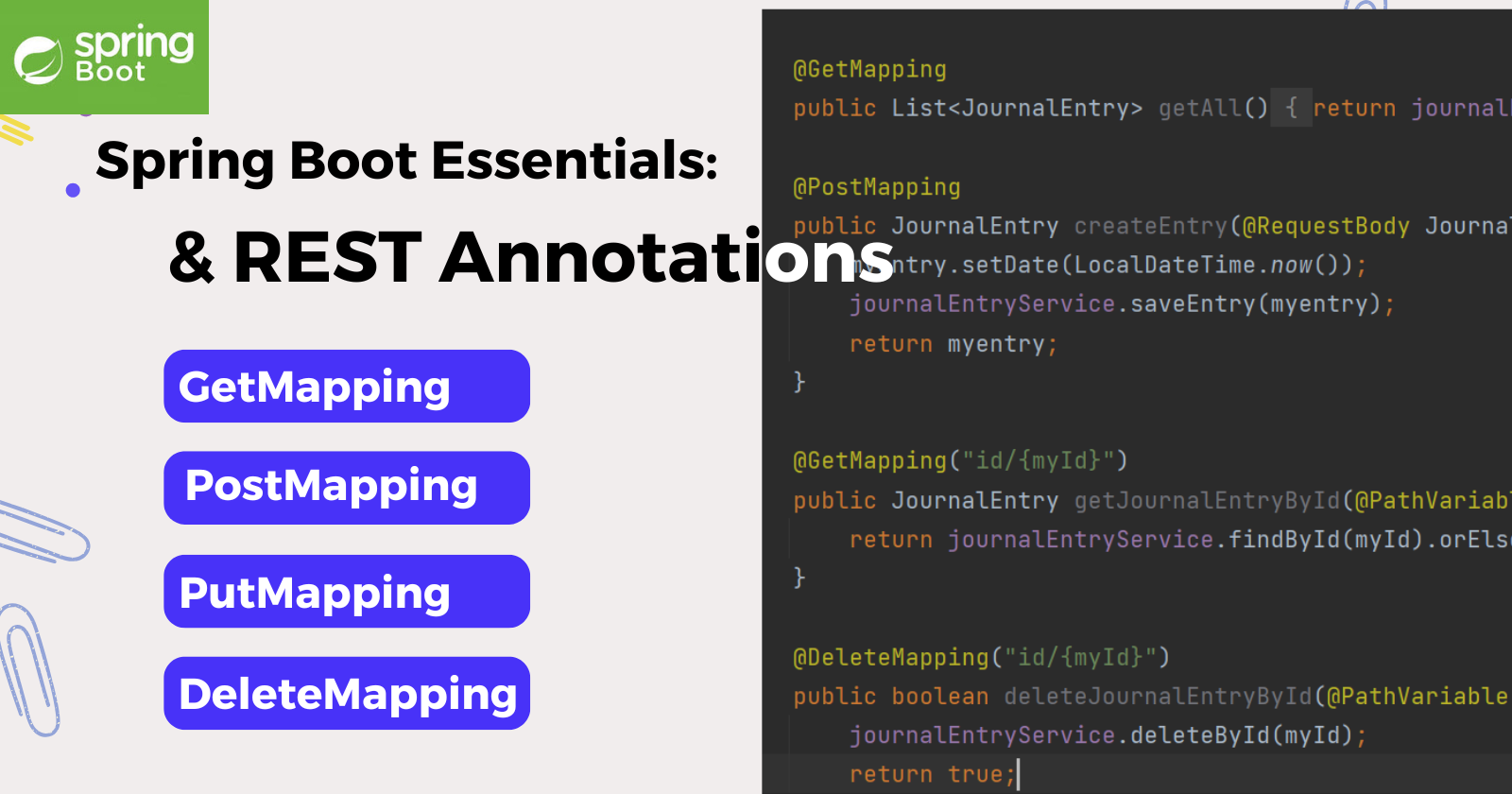
In this post, we will learn about various annotations used in Spring Boot. These annotations help developers efficiently create APIs without worrying about server management or handling different HTTP requests. Spring Boot annotations (like @GetMapping, @PostMapping, etc.) are directly linked to specific HTTP methods (such as GET, POST, DELETE, and PUT). These methods are the fundamental operations used in RESTful APIs to perform Create, Read, Update, and Delete (CRUD) actions.
Here’s how it works:
@GetMapping: Maps to the HTTP GET method, used to retrieve data from the server.
@PostMapping: Maps to the HTTP POST method, used to create new resources.
@PutMapping: Maps to the HTTP PUT method to update existing resources.
@DeleteMapping: Maps to the HTTP DELETE method, used to delete resources.
Detailed overview
@GetMapping
@GetMapping is an annotation in Spring Boot used to map HTTP GET requests to a specific method in your controller. It’s typically used to retrieve or fetch data from the server.
When a GET request is sent to a specific URL, the method marked with @GetMapping will handle that request and return the appropriate response.
To test the given example you need to install postman or other way by which you can request to server.
Example of @GetMapping
Let’s say you're building an API to manage users in your application, and you want to create an endpoint that retrieves the details of a user.
package com.example.demo.controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { // Example: Get user by ID @GetMapping("/users/{id}") public String getUserById(@PathVariable("id") Long id) { // For this example, we'll just return a simple message return "User with ID: " + id; } }
What is Path variable : In Spring Boot, @PathVariable is an annotation used to bind the dynamic values from the URL path to a method parameter in your controller. This allows you to capture specific values from the URL and use them in your business logic.
@PathVariable("id"): The @PathVariable annotation tells Spring to take the value from the path in the URL (in this case, the id part of /users/{id}) and assign it to the id method parameter.
How to request:
When a client (like a browser or Postman) sends a GET request to http://localhost:8080/users/1, it will trigger the getUserById method. The value 1 in the URL will be captured as the id variable and passed into the method. The method returns a simple string message, "User with ID: 1" in this case.
@PostMapping
@PostMapping is an annotation in Spring Boot used to handle HTTP POST requests. It’s typically used to create new resources on the server.
When a POST request is sent to a specific URL, the method marked with @PostMapping will handle that request and return the appropriate response. This is often used to send data to the server for creation purposes, such as creating a new user or adding a new item to a database.
Example of @PostMapping in Spring Boot: Let’s say you want to create an endpoint for adding a new user to your system. Here's an example where we send user data using a POST request to create a user.
package com.example.demo.controller; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { // Example: Create a new user @PostMapping("/users") public String createUser(@RequestBody User user) { // For this example, we'll just return a simple message return "User created: " + user.getName(); } }
Explanation of @RequestBody:
@RequestBody is used to bind the body of the HTTP POST request to a method parameter. In this case, we are sending a User object as JSON data in the request body, and Spring Boot will automatically convert it into a Java object.
The User class might look like this:
public class User { private String name; private String email; private int age; // Getters and Setters public String getName() { return name; } public void setName(String name) { this.name = name; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
In this example, when you send a POST request, the @RequestBody annotation binds the incoming JSON to the User object.
How to Test the API with Postman: Open Postman.
(a) Set the HTTP method to POST from the dropdown next to the URL field.
(b) Enter the URL for the POST request:
(c) In the Body tab in Postman, select the raw option and choose JSON from the dropdown.
(d) Enter the JSON data in the request body. For example:
{ "name": "John Doe", "email": "john.doe@example.com", "age": 30 }
(e) Send the request by clicking the Send button.
What Happens When You Send the POST Request?
When you send a POST request with the above JSON data, Spring Boot will automatically map the JSON fields (name, email, and age) to the User object in the controller. The method createUser will be invoked, and the user.getName() will return the value John Doe.
The response will be something like:
User created: John Doe
@PutMapping
@PutMapping is an annotation in Spring Boot used to handle HTTP PUT requests. It's primarily used to update existing resources on the server. The PUT method typically requires the client to send the updated data for the resource in the request body.
Example of @PutMapping in Spring Boot: Let’s say you want to create an endpoint to update an existing user’s information. Here's how you can handle this with @PutMapping.
package com.example.demo.controller; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { // Example: Update user details @PutMapping("/users/{id}") public String updateUser(@PathVariable("id") Long id, @RequestBody User user) { // For this example, we'll assume the user details are updated and return a simple message. return "User with ID " + id + " updated: " + user.getName(); } }
How to Test the API with Postman:
Set the HTTP method to PUT from the dropdown next to the URL field.
Open Postman.
Enter the URL for the PUT request:
write this json
{ "name": "Jane Doe", "email": "
jane.doe@example.com
", "age": 32 }
Explanation:
@PutMapping("/users/{id}"): This maps the HTTP PUT request to the updateUser method. The {id} part is a dynamic path variable that allows you to specify which user's information you want to update.
@PathVariable("id"): The @PathVariable annotation is used to capture the value of the dynamic {id} from the URL path. For example, if the request URL is /users/1, the value 1 will be passed to the method parameter id.
@RequestBody: The @RequestBody annotation is used to bind the JSON data in the request body to the User object. This data will contain the updated user details, such as name, email, or age.
The method updateUser returns a message with the updated user name, but in a real-world application, you would typically update the data in a database and then return a success response.
@DeleteMapping
@DeleteMapping
is an annotation in Spring Boot used to handle HTTP DELETE requests. It is typically used to remove a resource from the server, such as deleting a user, product, or any other resource in your application.Example:
Let’s say you want to create an endpoint to delete an existing user. Here's how you can handle this with @DeleteMapping.
package com.example.demo.controller; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { // Example: Delete user by ID @DeleteMapping("/users/{id}") public String deleteUser(@PathVariable("id") Long id) { // For this example, we'll assume the user is deleted and return a simple message. return "User with ID " + id + " has been deleted."; } }
How to Test the API with Postman:
Open Postman.
Set the HTTP method to DELETE from the dropdown next to the URL field.
Enter the URL for the DELETE request:
Here, 1 is the ID of the user you want to delete.
Send the request by clicking the Send button.
//this message will appear in postman User with ID 1 has been deleted.
Explanation:
@DeleteMapping("/users/{id}"): This maps the HTTP DELETE request to the deleteUser method. The {id} part is a dynamic path variable that allows you to specify which user you want to delete.
@PathVariable("id"): The @PathVariable annotation captures the value of the {id} from the URL path. For example, if the request URL is /users/1, the value 1 will be passed to the method as the id parameter.
The method deleteUser simply returns a message saying that the user with the given ID has been deleted. In a real application, you would typically delete the resource from the database.
This content has been created with the help of AI and my own learning. If you find any errors or discrepancies, please let me know, and I will gladly correct them.
Subscribe to my newsletter
Read articles from Niraj Sahani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Niraj Sahani
Niraj Sahani
I’m Niraj Sahani, a passionate software developer with a keen interest in creating Android apps. My expertise includes Java programming, Data Structures and Algorithms (DSA), and MySQL. I am dedicated to building efficient and user-friendly applications, while continually expanding my knowledge of new technologies. As a quick learner and problem solver, I am eager to contribute to innovative projects and grow in the tech industry.