How to Address Common Accessibility Challenges in iOS Mobile Apps Using SwiftUI

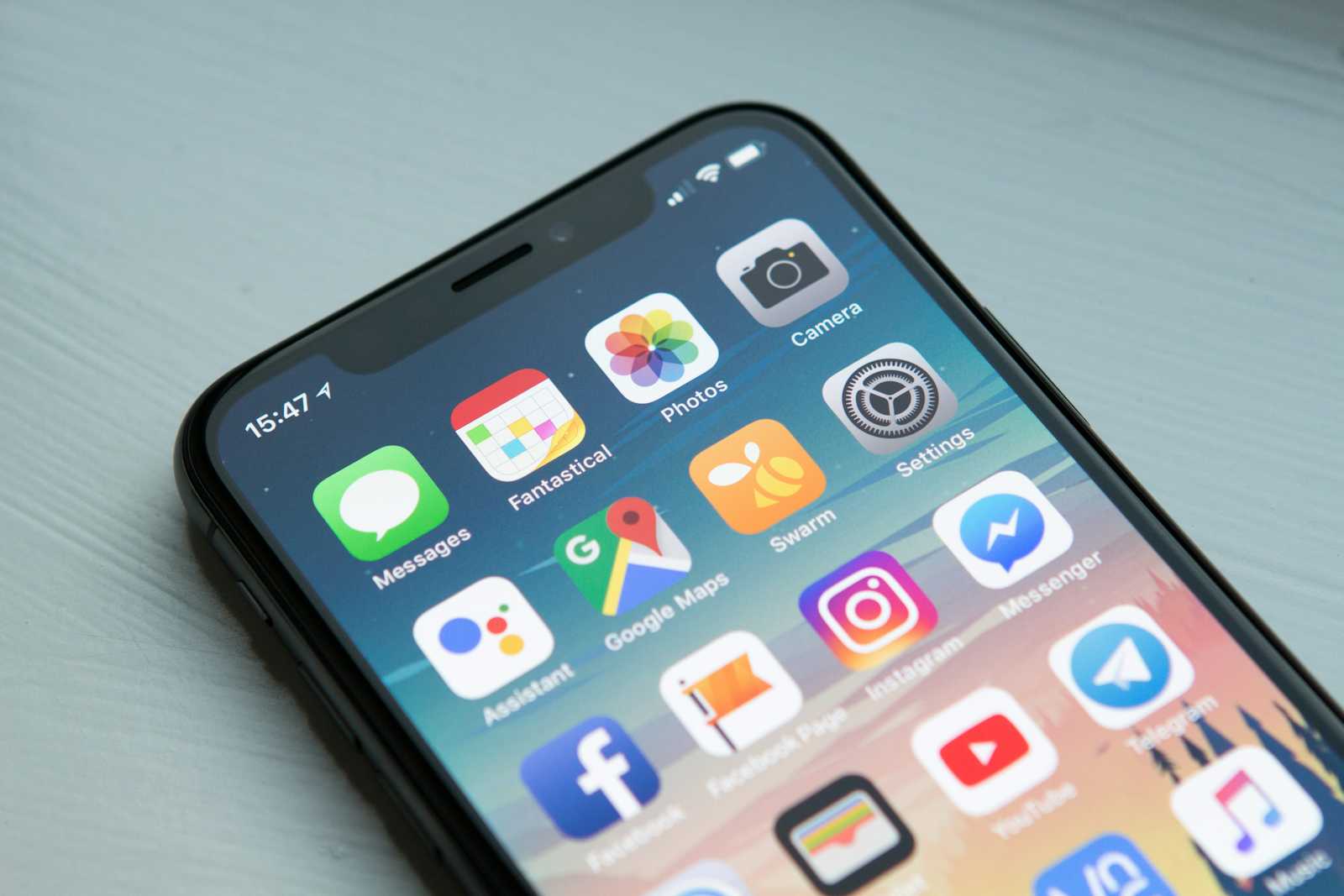
Mobile apps are essential tools in daily life, making accessibility a top priority. However, many apps still do not provide inclusive experiences for people with disabilities.
This article highlights nine common accessibility challenges in mobile apps and demonstrates how SwiftUI features can help developers address these issues effectively.
Each challenge is paired with a SwiftUI solution, sample code, and testing tips to guide developers in creating accessible and user-friendly apps.
Table of Contents
Mobile Apps Accessibility Issues and SwiftUI Solutions
Missing Labels and Descriptions
Challenge: Many apps lack appropriate labels or descriptions for buttons, images, and other interactive elements, making it difficult for screen readers to communicate their purpose to visually impaired users. Without these labels, users might struggle to understand the app’s functionality.
SwiftUI Solution: SwiftUI’s
.accessibilityLabel(_:)
modifier allows developers to assign clear, descriptive labels to interactive elements. These labels improve navigation and understanding by giving screen readers the necessary context.Example:
Label("Shop", systemImage: "cart") .accessibilityLabel("Go to Shop")
Testing: Enable VoiceOver on an iOS device, navigate through the app, and ensure each element has an accurate label. VoiceOver should read labels clearly to help users understand each element’s purpose without needing additional explanation.
Insufficient Color Contrast
Challenge: Low contrast between text and background colors can make it difficult for users with visual impairments to read the content, especially for those with color vision deficiencies or low vision.
SwiftUI Solution: Use SwiftUI’s dynamic system colors (
.primary
and.secondary
), which automatically adapt to the light or dark mode setting on the device, ensuring good readability.Example:
Text("Shop") .foregroundColor(.primary) // Adapts to light or dark mode automatically
If custom colors are necessary, test them against WCAG standards for color contrast, using tools like Color Contrast Analyzer.
Testing: Use Xcode’s Accessibility Inspector to verify contrast, and ensure that text remains readable in both light and dark modes. WCAG guidelines recommend a minimum contrast ratio of 4.5:1 for normal text.
Small Touch Targets
Challenge: Small buttons or other touch areas can be difficult for users with motor impairments to interact with accurately. Elements that are too small may require more precision than some users can provide.
SwiftUI Solution: Set minimum touch sizes by adding padding or using
.frame(minWidth:minHeight:)
to ensure a comfortable touch target size.Example:
Button(action: { /* Action */ }) { Text("Tap Me") .frame(minWidth: 44, minHeight: 44) }.padding()
Testing: Manually interact with touch elements in the app on an iOS device. Ensure they are easily tappable without precise effort. Verify touch target size with the Accessibility Inspector to confirm they meet recommended minimums (44x44 points).
Inaccessible Navigation
Challenge: Apps with limited navigability can cause frustration for users who rely on screen readers or keyboards. Without a clear reading order, navigating through the interface becomes challenging.
SwiftUI Techniques for Accessible Navigation:
Group Elements with
.accessibilityElement(children:)
: Combine related elements into a single accessible unit for more streamlined navigation.VStack { Text("Profile") Image("profile_picture") } .accessibilityElement(children: .combine)
Set Focus with
.accessibilityFocused
: Programmatically control focus on specific elements.Text("Special Announcement") .accessibilityFocused($isFocused)
Custom Actions with
.accessibilityAction
: Add specific actions for interactive controls like sliders or steppers.Slider(value: $value) .accessibilityAction(named: "Increase") { value += 10 }
Hide Decorative Elements with
.accessibilityHidden
: Exclude non-essential visuals from screen readers.Image("decorative_image") .accessibilityHidden(true)
Testing: Enable VoiceOver and use swipe gestures to confirm the intended focus order. Also, use a connected keyboard or switch control to test smooth transitions and confirm navigability.
Lack of Feedback for Actions
Challenge: Without feedback, users with visual or hearing impairments may struggle to confirm if an action has completed. Feedback like haptic, auditory, or visual cues can enhance usability.
SwiftUI Solution: Use
.accessibilityHint
to provide additional information about the action that will occur.Example:
Button("Submit") { // Submit action }.accessibilityHint("Submits the form")
Testing: Use VoiceOver to ensure that hints are read immediately after labels. Check that users can understand what each button does without extra explanation.
Complex or Confusing User Interfaces
Challenge: Cluttered interfaces can be overwhelming, particularly for users with cognitive impairments, who may struggle to navigate or process information effectively.
SwiftUI Solution: Simplify layouts and use
.accessibilitySortPriority
to organize the reading order logically.Example:
VStack { Text("Main Content") .accessibilitySortPriority(1) Button("Secondary Action") .accessibilitySortPriority(2) }
Testing: Use VoiceOver to verify the logical reading order and ensure only relevant elements are accessible. Use
.accessibilityHidden
to hide decorative elements that do not add meaningful information.
Lack of Support for Assistive Technologies
Challenge: Inadequate support for screen readers or other assistive technologies can make apps unusable for some users.
SwiftUI Solution: Group elements with
.accessibilityElement(children: .combine)
for cohesive navigation. This improves readability and usability for screen reader users.Example:
VStack { Text("Profile") Image("profile_picture") } .accessibilityElement(children: .combine)
Testing: Check with VoiceOver that grouped elements are announced as a single unit, improving navigation flow for visually impaired users.
Poorly Implemented Accessibility Features
Challenge: Without regular testing and updates, accessibility features can degrade over time, negatively impacting the user experience.
SwiftUI Solution: Regular testing with VoiceOver and Xcode’s Accessibility Inspector helps maintain effective functionality.
Testing: Conduct regular testing to detect regressions or improvements needed for accessibility. Recheck VoiceOver usability after UI updates to confirm features remain effective.
Insufficient Customization Options
Challenge: Limited customization options, such as font size or color schemes, restrict usability for users with specific visual needs.
SwiftUI Solution: Use
.dynamicTypeSize()
to allow text scaling based on the user’s preferred settings.Example:
Text("Adjustable Text") .dynamicTypeSize(.xxxLarge)
Testing: Adjust text size in iOS Accessibility settings, and ensure the app’s text scales correctly without truncating or overlapping, preserving readability.
References
Apple Developer Documentation: SwiftUI Accessibility
Comprehensive guide to accessibility in SwiftUI, covering accessibility properties like
.accessibilityLabel
,.accessibilityHint
,.accessibilityElement
, and more.
Apple Human Interface Guidelines: Accessibility
Apple's best practices for designing accessible apps, including color contrast and touch target size recommendations.
Color Contrast Analyzer
A tool for testing contrast ratios to ensure color accessibility compliance with WCAG standards.
Color Contrast Analyzer
VoiceOver and Accessibility Inspector
Tools for testing accessibility features, available in iOS and Xcode for simulating screen reader usage and checking accessibility properties.
Chandarana, N., & Gada, T. (2024). Accessibility Challenges in Current Mobile Applications: A Comprehensive Overview.
This journal paper provides an in-depth analysis of common accessibility challenges faced in mobile applications, discussing real-world examples and potential solutions for developers.
International Journal of Innovative Research in Computer and Communication Engineering.
Subscribe to my newsletter
Read articles from Namaswi Chandarana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
