How to Uncover Test Coverage and Understand Code in Elixir by Crashing Your App
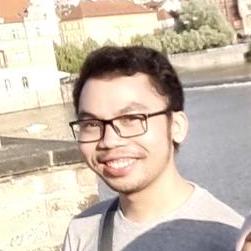
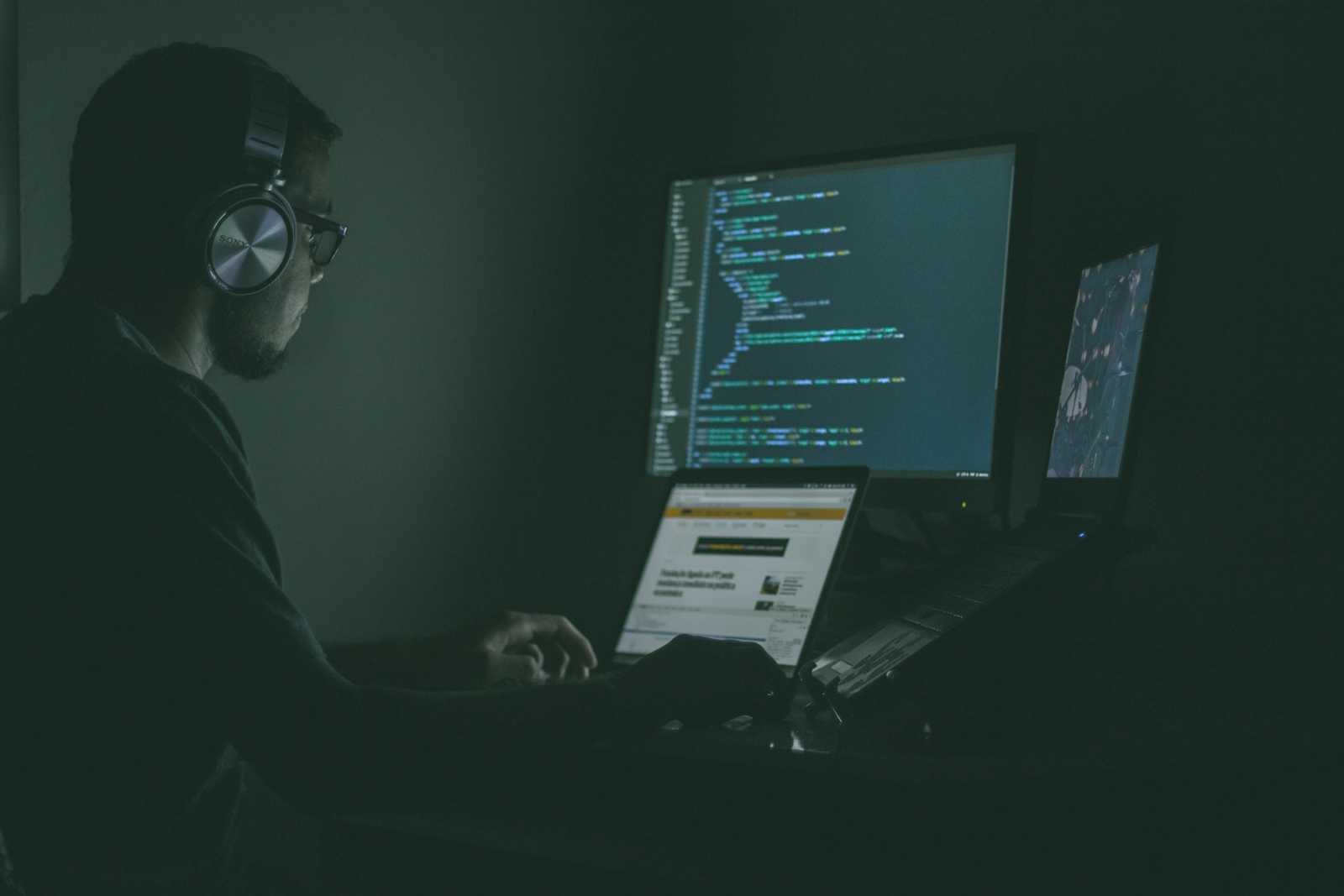
Elixir library such as excoveralls
allow us to generated an HTML report that summarise whether each line of code in an elixir project has been covered by our unit test suite or not. The HTML report can be easily generated by running mix coveralls.html
command.
In this article, I’d like to introduce a simpler, yet (in my opinion) clever, method to quickly check if a line of code is covered and explain how this approach can provide valuable insights into the code.
Using raise
to Verify Code Coverage and Gain Quick Insights
Let say we have a calculator module with a single function to add two numbers
defmodule Calculator do
def add(num1, num2) do
num1 + num2
end
end
and we want to know quickly if any of our unit tests has passed through or covered the function Calculator.add/2
. Instead of doing:
Running
mix coveralls.html
command.Open the generated html file.
Check if the function
Calculator.add/2
has been covered (by looking at the color of the line of code. I.e. green or red).
we could find it out quicker by putting a raise
before the line of code that we want to check.
defmodule Calculator do
def add(num1, num2) do
# added raise to intentionally crash the app
# when the function is invoked
raise "BOOM!"
num1 + num2
end
end
If our unit test covers the line of code we intend it to, running mix test
should cause one or more tests to fail. However, if the unit test does not cover the code, running mix test
should not result in any failures. Additionally, we should be able to confirm that the failure is caused by our intentional raise by reviewing the unit test error message.
Conclusion
By using the raise
technique, you can not only quickly verify whether a specific function or line of code is covered by a unit test but also identify the test cases associated with a particular piece of code. This can be especially valuable when joining a new project and trying to understand how a function is used and why it exists by examining the corresponding test cases—assuming, of course, that the project is well-covered by unit tests.
The next time you dive into a new Elixir project, you can intentionally trigger an application crash with raise
to quickly explore the use cases of specific functions or code. By reviewing the failing tests and their descriptions, you can gain insights into how the code is intended to work.
Subscribe to my newsletter
Read articles from Nyoman Abiwinanda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
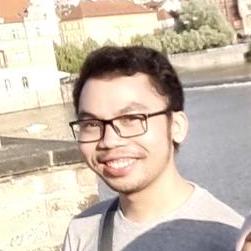
Nyoman Abiwinanda
Nyoman Abiwinanda
Passionate Elixir developer with a multidisciplinary background in backend development and software infrastructure. I enjoy sharing programming insights online to empower developers in building maintainable and scalable software.