React Error Handling: Built-in ErrorBoundary vs react-error-boundary

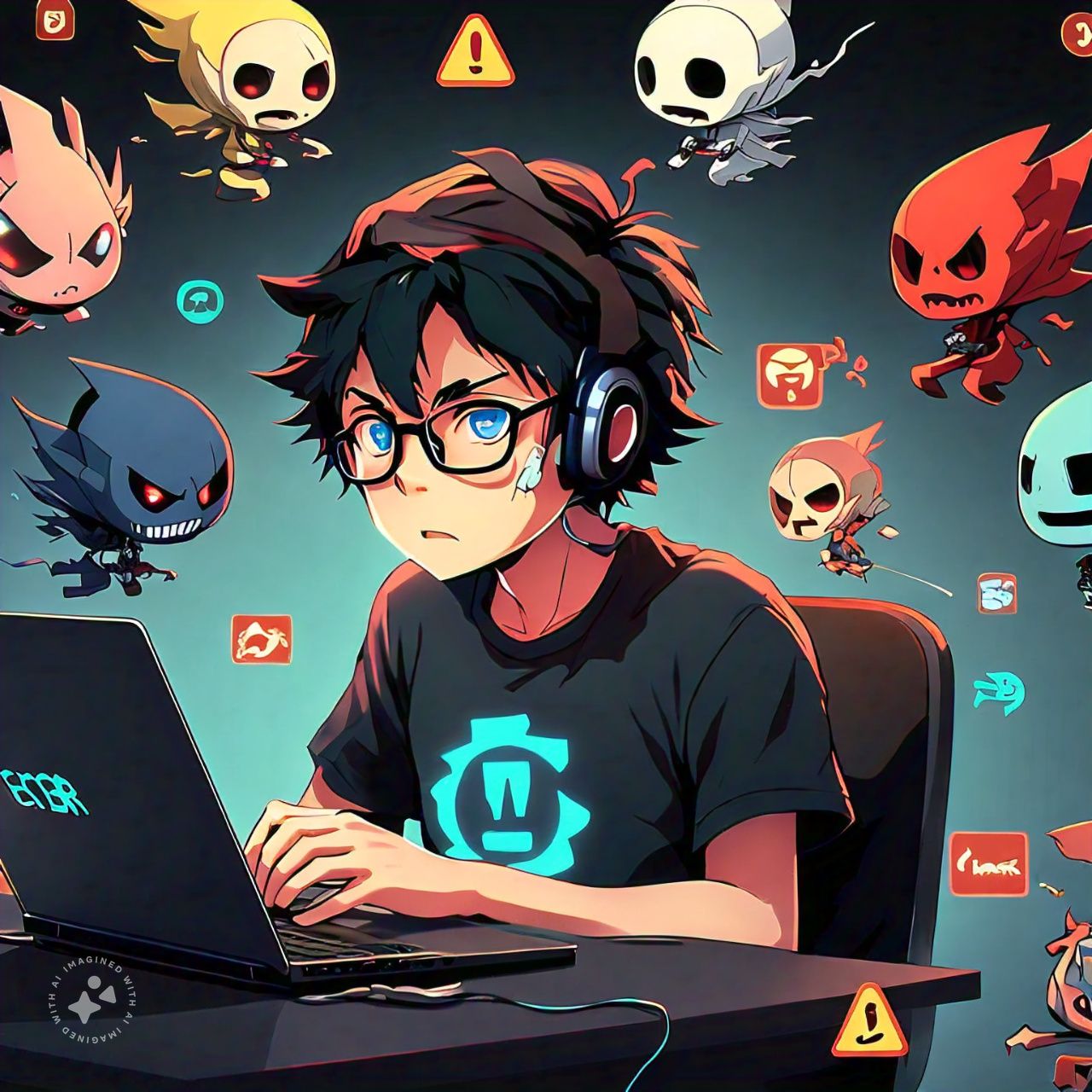
Errors are common in any app. React offers Error Boundaries to handle them gracefully, so your app doesn’t crash completely when something goes wrong. But which should you use: React's built-in ErrorBoundary or the react-error-boundary
library? Let’s find out.
What is an Error Boundary?
An Error Boundary catches JavaScript errors in a part of your app and shows a fallback UI (like an error message) instead of crashing the whole app.
Example: If your app fetches data from an API and the API fails, an Error Boundary can show an error message instead of a blank screen.
React's Built-in ErrorBoundary
React's built-in Error Boundaries are class components with special methods like:
getDerivedStateFromError
: Updates the state when an error happens.componentDidCatch
: Logs the error.
class ErrorBoundary extends React.Component {
state = { hasError: false };
static getDerivedStateFromError() {
return { hasError: true };
}
componentDidCatch(error, info) {
console.error("Error:", error, info);
}
render() {
if (this.state.hasError) return <h1>Something went wrong.</h1>;
return this.props.children;
}
}
Limitations of Built-in ErrorBoundary
Only Works with Class Components: No support for functional components.
No Async Error Handling: Doesn’t catch errors in
async/await
or Promises.No Event Handler Support: Errors in
onClick
or other event handlers aren’t caught.No Retry Feature: If you want to let users retry after an error, you must code it yourself.
react-error-boundary: The Modern Way
The react-error-boundary
library is a powerful alternative. It supports functional components, retries, logging, and even async error handling.
import { ErrorBoundary } from "react-error-boundary";
function ErrorFallback({ error, resetErrorBoundary }) {
return (
<div>
<p>Error: {error.message}</p>
<button onClick={resetErrorBoundary}>Try Again</button>
</div>
);
}
function App() {
return (
<ErrorBoundary
FallbackComponent={ErrorFallback}
onError={(error, errorInfo) => {
console.error("Logged Error:", error, errorInfo); // Handles error logging
}}
>
<MyComponent />
</ErrorBoundary>
);
}
Why react-error-boundary is Better
Works with Functional Components: Supports hooks like
useErrorBoundary
.Handles Async Errors: You can catch
fetch
orasync/await
errors.Event Handler Support: Wrap errors with
showBoundary
.Retry Made Easy: Automatically adds a "Try Again" button via
resetErrorBoundary
.Easy Error Logging: Use the
onError
prop to log errors to the console or services like Sentry.
Advanced Example: Handling Async and Event Errors
import { useErrorBoundary } from "react-error-boundary";
function MyComponent() {
const { showBoundary } = useErrorBoundary();
async function fetchData() {
try {
const response = await fetch("https://api.example.com");
if (!response.ok) throw new Error("Failed to fetch");
} catch (error) {
showBoundary(error);
}
}
return (
<div>
<button onClick={fetchData}>Fetch Data</button>
<button
onClick={() => {
try {
throw new Error("Manual error");
} catch (error) {
showBoundary(error); // Handle event handler errors
}
}}
>
Throw Error
</button>
</div>
);
}
Comparison: Built-in vs. react-error-boundary
Feature | Built-in ErrorBoundary | react-error-boundary |
Functional Components | ❌ Not supported | ✅ Supported |
Async Errors | ❌ Not handled | ✅ Handled with hooks |
Event Handler Errors | ❌ Not handled | ✅ Handled with showBoundary |
Retry or Reset | ❌ Manual implementation | ✅ Built-in "Try Again" |
Error Logging | ❌ Custom logic needed | ✅ Simple onError prop |
Which One Should You Use?
Use React's Built-in ErrorBoundary if:
You’re using class components.
Your error handling needs are simple.
You want to avoid adding dependencies.
Use react-error-boundary if:
You’re using functional components.
You need to handle async or event handler errors.
You want retry/reset functionality and easy error logging.
Conclusion
React’s built-in ErrorBoundary works but has limitations with modern use cases like async functions or functional components. react-error-boundary
makes error handling simpler, more powerful, and more user-friendly. It’s also great for logging errors with its onError
feature.
Choose the right tool for your project, and keep your app safe from unexpected crashes! 🚀
Subscribe to my newsletter
Read articles from Junais Babu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
