JavaScript in the Real World: Node and Beyond!
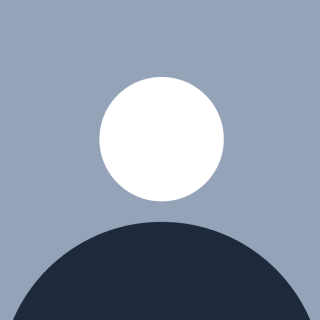
Table of contents
- What is Node.js? – The Engine That Powers Your Space Station
- npm – Your Space Station's Supply Ship
- Setting Up npm – Launching Your Space Station
- Creating a Fun Package – Your Space Mission Generator!
- Publishing Your Package – Sending Supplies to the npm Universe
- Bringing Everything Together – Installing npm Packages
- Understanding npm by Building Your Own Fun Package
- Building the Command Center of Your Space Station
- Setting Up a Basic HTTP Server – Powering Up Your Control Center
- Understanding the Event Loop – Managing Requests Efficiently
- Adding npm Packages – Equipping Your Space Station
- Handling Dynamic Data – Setting Up a Simple JSON Response
- Basic Server Request Flow
- Powering Your Space Station with Node.js
- Upgrading the Space Station with Advanced Node.js Capabilities
- Adding Dynamic Routes with Express.js
- Parsing Incoming Data – Using Body-Parser for Incoming Supplies
- Real-Time Updates – Simulating a Live Data Feed with SetInterval
- The Upgraded Server Workflow
- Challenge – Build Your Own Space Station Dashboard
- Elevating Your Node.js Server Beyond Basics
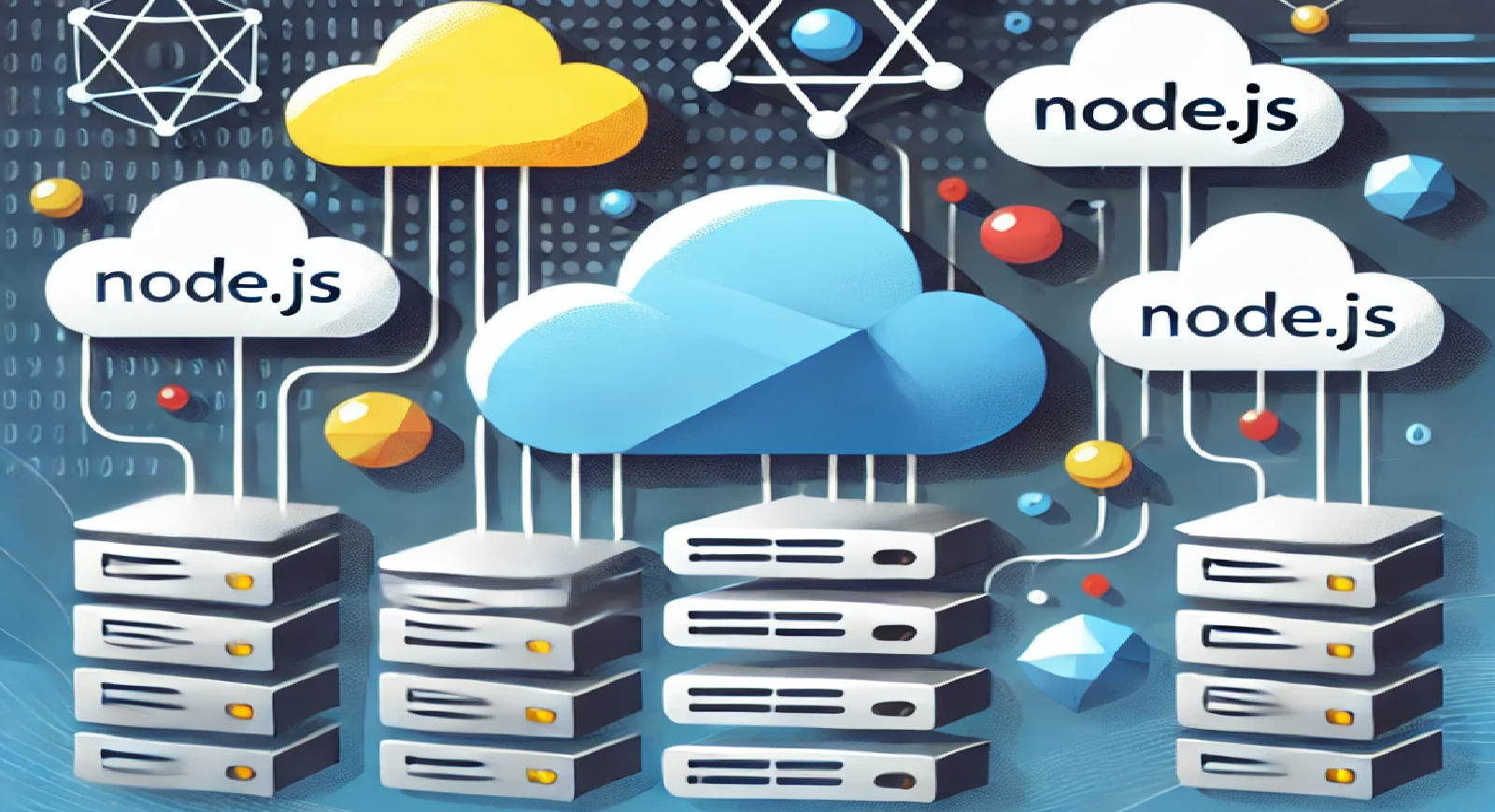
Welcome aboard your very own space station, powered by the versatile Node.js engine. But just like any space station, you need supplies to function. That's where npm (Node Package Manager) comes in—it’s the supply ship that delivers the tools and packages you need to keep your station running smoothly. In this article, we’ll dive into the basics of Node.js and npm and even create our own fun package to publish to npm. Think of it as sending out your own space supplies to other stations in the npm universe!
What is Node.js? – The Engine That Powers Your Space Station
Before we board the npm supply ship, let’s understand the heart of the station: Node.js. Traditionally, JavaScript runs inside the browser to control web pages, but Node.js takes JavaScript beyond the browser and powers backend applications, servers, and more.
Think of Node.js as the control center of your space station, responsible for:
Running web servers,
Communicating with databases,
Handling real-time applications (like chats or multiplayer games),
Managing files, networks, and more.
Node.js uses Google’s V8 engine (the same engine that powers Chrome) to execute JavaScript at blazing speeds, and its non-blocking, event-driven architecture allows it to handle thousands of requests simultaneously—perfect for large-scale applications.
npm – Your Space Station's Supply Ship
In our space station, we need supplies to get things done. These supplies come in the form of packages, which are reusable blocks of code that perform specific tasks. Want to build a web server? Install a package like Express.js. Need a tool to format dates? There’s a package for that, too!
Enter npm—the Node Package Manager. npm is like your trusty supply ship, delivering packages to your space station to help you build better, faster, and smarter applications. It:
Installs packages you need,
Manages dependencies so your project has everything it needs,
Publishes your own packages for other developers to use.
What is a Package?
A package is simply a reusable module of code. Whether it’s a tool, a framework, or even just a function, a package can help you solve a specific problem. Every package includes:
JavaScript code: The actual functionality of the package.
A
package.json
file: This is the blueprint of the package, describing its name, version, dependencies, and more.
By using npm, you can quickly install and use these packages to speed up development, rather than writing everything from scratch.
Setting Up npm – Launching Your Space Station
Before we create and publish our fun package, let’s set up npm to manage our project. When you initialize a new project, npm creates a package.json
file, which is like the blueprint of your space station. This file keeps track of all the packages (dependencies) your project needs.
Step 1: Initialize npm
To start, open your terminal and navigate to the folder where you want to create your project. Then, run:
npm init -y
This command creates a package.json
file with some default settings.
What is package.json
?
The package.json
file is the mission log for your project. It lists all the packages your project depends on, along with other metadata like the project’s name, version, and description. Here's an example of a package.json
file:
{
"name": "fun-space-missions",
"version": "1.0.0",
"description": "A package that generates random space missions",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"dependencies": {},
"devDependencies": {}
}
name: The name of your project.
version: The version of your project.
description: A short description of what your project does.
scripts: Commands you can run (like starting the app with
npm start
).dependencies: Lists the packages your project needs.
devDependencies: Lists the development tools your project relies on (e.g., testing frameworks).
This file ensures that your project’s dependencies (supplies) are tracked and can be easily shared with other developers.
Creating a Fun Package – Your Space Mission Generator!
Let’s make things fun. Instead of something simple like temperature conversions, we’re going to create a package that generates random space missions! These missions will give you an objective and a destination in space. Think of it as sending space missions from your station to others!
Step 1: Write the Code
In your project folder, create a new file called index.js
. Add the following code:
// Space mission generator
// Array of mission objectives
const objectives = [
"Explore a new planet",
"Collect asteroid samples",
"Repair the space station",
"Study a black hole",
"Rescue a stranded spaceship"
];
// Array of destinations
const destinations = [
"Mars",
"Jupiter",
"Alpha Centauri",
"The Andromeda Galaxy",
"The Moon"
];
// Function to generate a random mission
function generateMission() {
const objective = objectives[Math.floor(Math.random() * objectives.length)];
const destination = destinations[Math.floor(Math.random() * destinations.length)];
return `Mission: ${objective} at ${destination}`;
}
// Export the function
module.exports = { generateMission };
This code generates a random space mission, combining a random objective (e.g., “Explore a new planet”) with a random destination (e.g., “Mars”).
Step 2: Test the Package Locally
Before publishing your package to npm, let’s test it locally to make sure it works. Create another file in the same folder called test.js
:
// Import the space mission generator
const missionGenerator = require('./index');
// Test the mission generator
console.log(missionGenerator.generateMission());
Run the test using Node.js:
node test.js
You should see a random space mission printed to the console, like:
Mission: Explore a new planet at Mars
If everything works, you’re ready to publish your package to npm!
Publishing Your Package – Sending Supplies to the npm Universe
Now that your package is working, let’s publish it to npm so that other developers can install and use it! First, make sure you have an npm account. If you don’t have one yet, you can create one here.
Step 1: Login to npm
Log into your npm account from the terminal:
enpm login
Step 2: Publish Your Package
Once logged in, run the following command to publish your package:
npm publish
Your package is now live on npm! Other developers can install it by running:
npm install <your-package-name>
Congratulations! You’ve just published your own package to the npm universe, allowing other developers to install and use your space mission generator.
Bringing Everything Together – Installing npm Packages
Now that we’ve successfully created and published a package, let’s talk about how npm helps you manage packages in your projects. When you install a package using npm, it does the following:
Downloads the package from the npm registry.
Stores it in your project’s
node_modules
folder.Records the package in the
package.json
file so future developers know what packages your project depends on.
You can install any package from npm using:
npm install <package-name>
npm also lets you update, remove, and manage dependencies easily, making sure your space station always has the right supplies.
Understanding npm by Building Your Own Fun Package
In this part, we learned about Node.js and npm, and by creating our own space mission generator, we explored how packages are created and published to the npm registry. You now understand how npm works as a supply ship, delivering essential tools and packages to your projects and managing dependencies efficiently.
In the next part, we’ll take this knowledge further by setting up a Node.js server and creating real-world applications.
Building the Command Center of Your Space Station
Now that we’ve sent out our own space mission package into the npm universe, it’s time to focus on building the command center of our space station: a Node.js server. In this part, we’ll create a basic HTTP server to handle incoming requests, send responses, and explore some key features that make Node.js ideal for backend programming. Think of this server as the control panel that coordinates data and functionality across our space station.
Setting Up a Basic HTTP Server – Powering Up Your Control Center
An HTTP server is like the main console of your space station, processing incoming signals (requests) and sending back responses. With Node.js, setting up a server is straightforward and lightweight, allowing us to handle data, serve files, and even process real-time information.
Step 1: Import the HTTP Module
Node.js comes with several built-in modules, and one of the most important for server development is the HTTP module. This module allows us to create servers, handle requests, and send responses.
To start, let’s import the HTTP module. In your project directory, create a new file called server.js
and add the following line:
// Import the built-in 'http' module
const http = require('http');
The http
module is our communication bridge. It allows our server to receive requests and send back responses.
Step 2: Create a Server and Define Response Logic
Now that we’ve imported the HTTP module, let’s use it to create a server. The http.createServer()
function creates an HTTP server that listens for incoming requests and processes them based on our defined logic.
Add the following code to server.js
:
// Import the HTTP module
const http = require('http');
// Create a server object
const server = http.createServer((req, res) => {
// Check the URL of the request
if (req.url === "/") {
// Send a response for the home page
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end("Welcome to the Space Station Control Center!");
} else if (req.url === "/status") {
// Send a status message
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end("All systems are operational.");
} else {
// Handle unknown routes
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end("404 Not Found - The requested route does not exist.");
}
});
This code creates a server that listens for different URLs and responds with different messages. Let’s break down each part:
req.url: This property checks the URL of the incoming request to determine which route (page) the user is requesting.
res.writeHead(): Sets the status code (200 for success, 404 for not found) and the content type (plain text).
res.end(): Sends the response back to the client and closes the connection.
Step 3: Make the Server Listen on a Port
After creating the server, we need to assign it a port to listen on. Ports are like docking bays—they allow incoming traffic to access the server. In this case, we’ll make our server listen on port 3000.
Add the following code to start the server:
// The server listens on port 3000
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Now, if you run the server with node server.js
and go to http://localhost:3000 in your browser, you should see the message "Welcome to the Space Station Control Center!" displayed. If you visit http://localhost:3000/status, you’ll see "All systems are operational."
Understanding the Event Loop – Managing Requests Efficiently
The event loop is at the heart of how Node.js handles incoming requests. Think of it as the traffic control center of your space station. The event loop ensures that requests are processed efficiently without blocking other tasks, even if one task takes longer than usual.
How the Event Loop Works
In traditional server environments, each request might be processed sequentially or by creating a new thread for each request, which can slow down performance. But Node.js is single-threaded and uses an event-driven model. This means it can handle multiple requests by queuing them and processing each one as soon as resources are available.
Here’s a simple breakdown of how the event loop manages tasks:
Request Queue: All incoming requests are added to the queue.
Asynchronous Operations: Node.js can start processing some requests while waiting for others (like reading files or accessing databases).
Callback Execution: When an operation is complete, its callback is added to the event loop, which ensures it’s processed without blocking other requests.
The event loop makes Node.js highly efficient, especially for applications that require real-time data handling, like online gaming or live chat systems.
Adding npm Packages – Equipping Your Space Station
Now that our server is up and running, let’s add a popular package from npm to enhance our space station. This time, we’ll install nodemon, a tool that automatically restarts the server whenever changes are made. Think of nodemon as a maintenance bot—it watches for updates, allowing us to develop faster without manually restarting the server.
Step 1: Install nodemon
To install nodemon, use npm by running the following command in your terminal:
npm install -g nodemon
The -g
flag installs nodemon globally, so it’s available for all projects on your machine.
Step 2: Run the Server with nodemon
To run your server with nodemon, use the following command:
nodemon server.js
Now, whenever you make changes to server.js, nodemon will automatically restart the server. This speeds up development and lets you see changes in real-time.
Handling Dynamic Data – Setting Up a Simple JSON Response
Now that our server is operational, let’s make it more interactive by serving JSON data. JSON (JavaScript Object Notation) is a popular format for sending and receiving data, commonly used in APIs. In our space station, JSON is like the data log, where information is structured in a format that’s easy for both machines and humans to read.
Step 1: Create a Dynamic Route for Space Data
Let’s modify our server to serve some JSON data about our space station’s systems. Add this new route to server.js
:
// Create a JSON response for system status
const server = http.createServer((req, res) => {
if (req.url === "/") {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end("Welcome to the Space Station Control Center!");
} else if (req.url === "/status") {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end("All systems are operational.");
} else if (req.url === "/systems") {
// JSON response with space station data
const systemsData = {
lifeSupport: "Operational",
power: "Optimal",
navigation: "Online",
communication: "Stable"
};
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify(systemsData));
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end("404 Not Found - The requested route does not exist.");
}
});
Explanation:
JSON Response: The
/systems
route responds with a JSON object representing the status of our space station’s systems.JSON.stringify(): This method converts the JavaScript object
systemsData
into a JSON-formatted string, which we send as a response.
Now, if you go to http://localhost:3000/systems in your browser, you’ll see:
{
"lifeSupport": "Operational",
"power": "Optimal",
"navigation": "Online",
"communication": "Stable"
}
This feature allows our server to serve data dynamically in JSON format, which is especially useful for building APIs and applications that need to share structured data.
Basic Server Request Flow
Here’s a flowchart to visualize how our Node.js server processes requests:
[ Client Request ]
|
+-------------|-----------------+
| Event Loop |
| - Handles incoming requests |
| - Manages asynchronous ops |
+-------------|-----------------+
|
[ Server Response ]
- Text for "/"
- Text for "/status"
- JSON for "/systems"
This flowchart shows the event-driven nature of our server, where requests are handled efficiently and responses are sent back based on the request URL.
Powering Your Space Station with Node.js
In Part 2, we set up a Node.js server that acts as the control panel of our space station, responding to different requests with text and JSON data. We explored the event loop and installed nodemon, our maintenance bot that automates server restarts during development. You now have a basic server running and are ready to expand its capabilities further.
In the next part, we’ll take things a step further by adding more dynamic content and additional packages to enhance our space station’s control panel.
Upgrading the Space Station with Advanced Node.js Capabilities
Our space station server is up and running, handling requests and delivering information. But now, it’s time to upgrade by adding more functionality using additional npm packages. Imagine this as installing new modules to our station’s control center to handle more complex tasks, like parsing incoming data and managing routes more efficiently.
In this final part, we’ll enhance our server with more dynamic routes, enable data parsing, and even simulate real-time data updates for a live view of our station’s operations!
Adding Dynamic Routes with Express.js
Managing routes manually in a large application can get complicated. This is where Express.js, a lightweight framework, comes in. Think of Express as additional command modules for your space station, making it easier to handle different routes and requests with minimal setup.
Step 1: Install Express
To add Express to our project, run the following npm command:
npm install express
Step 2: Setting Up Express
Let’s modify our server to use Express for routing. In server.js, replace the previous setup with Express:
// Import the express module
const express = require('express');
const app = express();
// Define routes using Express
app.get("/", (req, res) => {
res.send("Welcome to the upgraded Space Station Control Center!");
});
app.get("/status", (req, res) => {
res.send("All systems are operational.");
});
app.get("/systems", (req, res) => {
const systemsData = {
lifeSupport: "Operational",
power: "Optimal",
navigation: "Online",
communication: "Stable"
};
res.json(systemsData);
});
// Start the server on port 3000
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
With Express, routing becomes more straightforward:
app.get(): Defines routes that respond to HTTP GET requests.
res.send(): Sends plain text responses.
res.json(): Sends JSON responses.
Now, with Express, adding new routes is as simple as defining additional app.get() calls, making it easier to expand our space station’s capabilities.
Parsing Incoming Data – Using Body-Parser for Incoming Supplies
Sometimes, our server will need to handle incoming data from clients, such as form submissions or JSON payloads. This is like receiving supply crates that need to be unpacked and processed. Body-Parser is a middleware package that allows us to parse incoming data easily, making it accessible within our routes.
Step 1: Install Body-Parser
To add body-parsing functionality, let’s install the body-parser package:
npm install body-parser
Step 2: Integrate Body-Parser with Express
In your server.js file, import and set up Body-Parser. This will allow your server to parse both URL-encoded and JSON data sent by clients.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
// Middleware to parse JSON and URL-encoded data
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: true }));
// Example route to handle incoming data
app.post("/report", (req, res) => {
const report = req.body;
console.log("New Report Received:", report);
res.send("Report received and logged.");
});
Explanation:
app.use(bodyParser.json()): Tells Express to parse JSON payloads.
app.use(bodyParser.urlencoded({ extended: true })): Tells Express to parse URL-encoded data (like form data).
app.post("/report"): Creates a POST route where incoming reports can be submitted. This data can now be accessed via
req.body
.
Real-Time Updates – Simulating a Live Data Feed with SetInterval
Imagine your space station needs to constantly monitor the status of systems and update the command center with live data. To simulate this, we can use setInterval to send periodic status updates.
Step 1: Add a Real-Time Endpoint
In server.js, add a new route that simulates a real-time data feed by generating random status updates every few seconds.
// Real-time system status simulation
app.get("/live-status", (req, res) => {
const getStatusUpdate = () => {
const systemsData = {
lifeSupport: Math.random() > 0.1 ? "Operational" : "Maintenance",
power: Math.random() > 0.2 ? "Optimal" : "Low",
navigation: "Online",
communication: Math.random() > 0.15 ? "Stable" : "Interference"
};
return systemsData;
};
const updateInterval = setInterval(() => {
const data = getStatusUpdate();
res.write(`data: ${JSON.stringify(data)}\n\n`);
}, 3000);
// End response when connection is closed
req.on('close', () => clearInterval(updateInterval));
res.setHeader("Content-Type", "text/event-stream");
res.setHeader("Cache-Control", "no-cache");
res.setHeader("Connection", "keep-alive");
res.flushHeaders();
});
Explanation:
setInterval(): Generates random system statuses every 3 seconds.
res.write(): Sends data to the client each time the interval runs.
req.on('close'): Clears the interval when the client disconnects, freeing up resources.
Now, if you access http://localhost:3000/live-status, you’ll see a real-time stream of status updates from your space station’s systems.
The Upgraded Server Workflow
Here’s a flowchart illustrating how our upgraded server with Express, Body-Parser, and real-time updates operates:
[ Incoming Request ]
|
+----------|----------+
| Express Routes |
| - GET, POST, etc. |
+----------|----------+
|
+--------------|--------------+
| Body-Parser Middleware |
| - Parses incoming data |
+--------------|--------------+
|
[ Real-Time Updates ]
- Sends data stream
This flowchart shows how incoming requests are routed, processed with Body-Parser, and connected to real-time updates.
Challenge – Build Your Own Space Station Dashboard
To reinforce your understanding, here’s a challenge: build an interactive dashboard for your space station by adding routes that allow users to:
Check System Status: A route that shows the real-time status of systems.
Submit Reports: A POST route where users can submit maintenance or status reports.
View All Reports: A route to retrieve all reports submitted so far.
Elevating Your Node.js Server Beyond Basics
In this final part, we transformed our Node.js server into a fully functional control center using Express for routing, Body-Parser for handling incoming data, and simulated real-time updates. With these new tools, your server can handle more complex tasks, manage dynamic data, and simulate live information streams—all essential for building modern, responsive applications.
Next up, we'll dive into building RESTful APIs, where you’ll learn to structure data-driven endpoints for full-stack applications!"
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by