Local Storage in JavaScript: Why Do We Need JSON.stringify()? 🤔

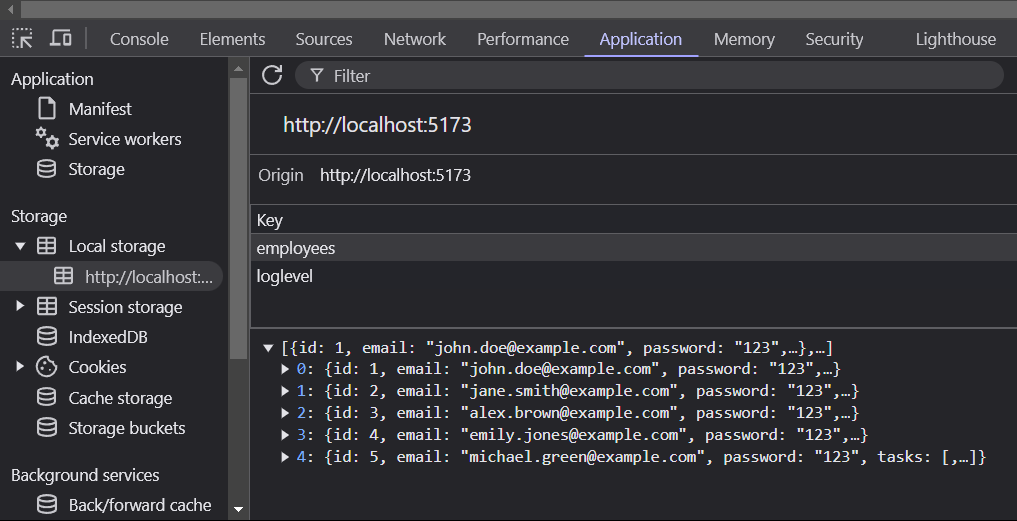
In the world of client-side storage, localStorage is like the superhero of web development. It lets you store data directly in the browser, so even if the user closes the browser or refreshes the page, the data stays safe. 🙌
But, here's the catch—localStorage only understands strings. So, if you want to store complex data like JavaScript objects (for example, an employee's details), you can’t just drop it directly in there. You need to convert it into a string first! Enter the magic of JSON.stringify()
. 🎩✨
What is Local Storage? 🧐
Local Storage is a key-value storage system that lives in the browser. You can store data in it, and that data will stick around until you manually delete it. Unlike cookies, the data doesn’t expire after a session ends, so it’s perfect for things like saving user preferences, session info, or even form data.
But here's the thing—localStorage can only store strings. No objects, no arrays, and definitely no functions. 😤 So when you need to store an object (say, an employee's details), you have to turn it into a string first. And that’s where JSON.stringify()
comes into play. 💥
Why Do We Need JSON.stringify()
? 🤨
Since localStorage only accepts strings, any complex data (objects, arrays, etc.) needs to be converted into a string format before it can be saved. That’s what JSON.stringify()
does: it converts your JavaScript object into a JSON string that localStorage can understand.
Let’s Break It Down with an Example:
Let’s say you have an employee object like this:
const employee = {
name: "John Doe",
position: "Software Engineer",
id: "EMP1234"
};
Now, if you try to directly store this object in localStorage, you’re going to get an error. 🤯 localStorage doesn’t know what to do with an object. That’s why we need to use JSON.stringify()
to convert it into a string:
localStorage.setItem('employee', JSON.stringify(employee));
Now the employee data is safely stored as a string in localStorage, like this:
"{"name":"John Doe","position":"Software Engineer","id":"EMP1234"}"
How Does JSON.stringify()
Work?
JSON.stringify()
turns your JavaScript object into a string that looks like valid JSON (even though it’s just a string). It’s like taking your complex object and converting it into a format that localStorage can easily store. 🔐
So, after we store the data as a string, we can later retrieve it and use JSON.parse()
to turn it back into a JavaScript object.
Retrieving the Data:
Once the data is stored as a string, retrieving it is easy:
const employeeData = localStorage.getItem('employee');
But since it’s still a string, we need to parse it back into an object:
const employeeObject = JSON.parse(employeeData);
console.log(employeeObject); // { name: "John Doe", position: "Software Engineer", id: "EMP1234" }
Why Is This Conversion Important?
localStorage Only Supports Strings: Since localStorage can only handle strings, we have to use
JSON.stringify()
to convert objects into strings that localStorage can store. Without this, you'd just get an error when trying to save objects.Maintaining Data Integrity: Using
JSON.stringify()
ensures that your object’s data remains intact. The properties likename
,position
, andid
are preserved when the object is converted to a string, so you don’t lose any data in the process.Cross-Browser Compatibility: JSON is a universally supported format, meaning any browser that supports localStorage can handle the JSON string you store. So, whether the user is on Chrome, Firefox, or Safari, your data will be safe and accessible.
Handling Complex Data: If your object has nested structures or arrays inside it,
JSON.stringify()
will ensure the entire structure is correctly serialized and stored, so you can easily retrieve and use it later.
Real-World Example: Employee Management System (EMS)
Let’s say you’re building an Employee Management System (EMS). You can use localStorage to store employee data temporarily. For example:
When an admin adds a new employee, you can store their data (name, position, ID, etc.) in localStorage.
Later, when the admin views the employee details, the data can be retrieved from localStorage without needing to make another server request, improving performance.
Final Thoughts 🏁
To wrap things up:
localStorage is a great tool for storing data in the browser, but it only accepts strings.
Use
JSON.stringify()
to convert objects (or arrays) into strings so that they can be stored in localStorage.Use
JSON.parse()
to retrieve the data and convert it back into a usable JavaScript object.
So the next time you need to store an object in localStorage, remember: JSON.stringify()
is your friend! Without it, you'd be stuck with errors and no data. 😅
Subscribe to my newsletter
Read articles from Abhishek Raut directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Raut
Abhishek Raut
🔭 I’m currently working on Full Stack Project 👯 I’m looking to collaborate on Animated Websites 🌱 I’m currently learning Data Science 💬 Ask me about React GSAP ⚡ Fun fact I am Working on Project and learning tech stack used in That