Mastering Go's Comma-Ok Syntax: A Beginner's Guide to Error Handling

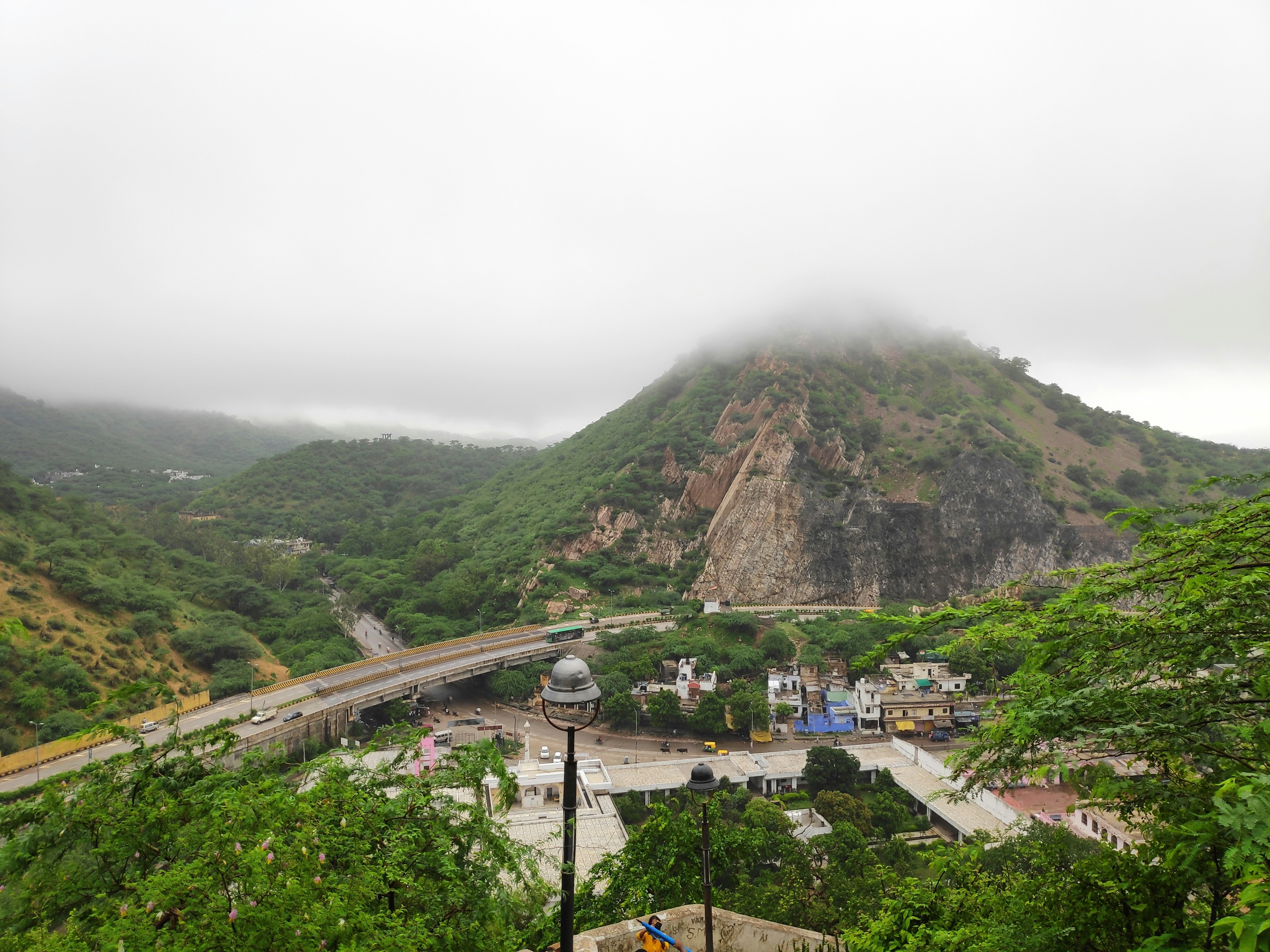
The comma-ok syntax in Go is a practical way to handle operations that might succeed or fail. It helps you check the outcome of an operation, ensuring your program doesn't crash unexpectedly.
In this article, we’ll explore comma-ok step by step using simple examples and concepts we’ve covered so far, like functions, loops, and conditional statements. We'll use err
when the error is important and _
when it can be ignored.
What is the Comma-Ok Syntax?
In Go, some operations return multiple values, often including:
A result (the actual value).
An error or status indicating success or failure.
The comma-ok syntax is written as:
result, err := someOperation()
Here:
result
stores the outcome of the operation.err
stores an error if the operation failed, ornil
if it succeeded.
When the error is irrelevant, you can replace err
with _
like this:
result, _ := someOperation() // Ignoring the error
Using Comma-Ok Syntax
Example 1: Validating User Input
Let’s validate user input where errors matter.
package main
import (
"fmt"
"strconv"
)
func main() {
// Simulating user input
userInput := "123abc"
// Attempt to convert user input to an integer
num, err := strconv.Atoi(userInput)
// Check if the conversion succeeded
if err == nil {
fmt.Printf("The valid number is: %d\n", num)
} else {
fmt.Println("Invalid number! Please enter a proper numeric value.")
}
}
Explanation
Simulating Input: The
userInput
variable represents input from the user.Conversion: We attempt to convert the string into an integer using
strconv.Atoi
.Comma-Ok:
num
: Stores the converted number if successful.err
: Stores the error if the conversion fails.
Conditional Statement:
If
err == nil
, the conversion succeeded, and we print the number.Otherwise, we display an error message.
Output
Invalid number! Please enter a proper numeric value.
Example 2: Ignoring Errors
If you know the input is guaranteed to be valid, you can ignore the error using _
.
package main
import (
"fmt"
"strconv"
)
func main() {
// Guaranteed valid input
userInput := "42"
// Convert input to an integer, ignoring errors
num, _ := strconv.Atoi(userInput)
// Print the result
fmt.Printf("The number is: %d\n", num)
}
Explanation
Ignoring the Error: Instead of storing the error in a variable, we use
_
since we know the input is valid.Output: The program proceeds without error handling.
Output
The number is: 42
Example 3: Function Returning Comma-Ok
Let’s create a function to check whether a number is divisible by another. Errors matter here.
package main
import "fmt"
// Function to check divisibility
func isDivisible(num, divisor int) (bool, error) {
if divisor == 0 {
return false, fmt.Errorf("division by zero is not allowed")
}
return num%divisor == 0, nil
}
func main() {
num := 10
divisor := 0
// Using comma-ok to check divisibility
ok, err := isDivisible(num, divisor)
if err != nil {
fmt.Println(err)
} else if ok {
fmt.Printf("%d is divisible by %d\n", num, divisor)
} else {
fmt.Printf("%d is not divisible by %d\n", num, divisor)
}
}
Explanation
Function Logic: The
isDivisible
function:Returns
false
with an error ifdivisor == 0
.Returns
true
if divisible.Returns
false
otherwise.
Comma-Ok:
ok
: Indicates whether the number is divisible.err
: Captures the error, if any.
Conditional Handling:
If
err != nil
, we print the error.Otherwise, we use
ok
to determine the divisibility.
Example 4: Loop with Comma-Ok
Let’s validate multiple user inputs in a loop. We’ll ignore errors here.
package main
import (
"fmt"
"strconv"
)
func main() {
// List of user inputs
inputs := []string{"10", "abc", "25", "1x"}
for _, input := range inputs {
// Convert each input to an integer, ignoring errors
num, _ := strconv.Atoi(input)
// Print the result
fmt.Printf("Number: %d\n", num)
}
}
Explanation
Inputs: A list of strings simulates user-provided numbers.
Loop: The
for
loop iterates over each input.Conversion: For each string,
strconv.Atoi
attempts a conversion.Ignoring Errors: We use
_
to skip error handling, assuming inputs are valid.
However, skipping error handling should only be done when you're certain the input is valid.
Key Takeaways
Use
err
when error handling is critical for safe and robust programs.Use
_
when the error is irrelevant or guaranteed not to occur.Comma-Ok Syntax:
Helps safely perform operations like type conversions or function results.
Prevents runtime crashes by handling errors gracefully.
Conclusion
The comma-ok syntax is an essential feature in Go that ensures your code handles success and failure scenarios effectively. By using it correctly and thoughtfully, you can write robust and reliable Go programs.
Stay tuned for more beginner-friendly tutorials as we continue building your Go programming knowledge. 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
