Understanding Python's Platform Independence: A Deep Dive into Bytecode, AST, and Machine Code

Table of contents
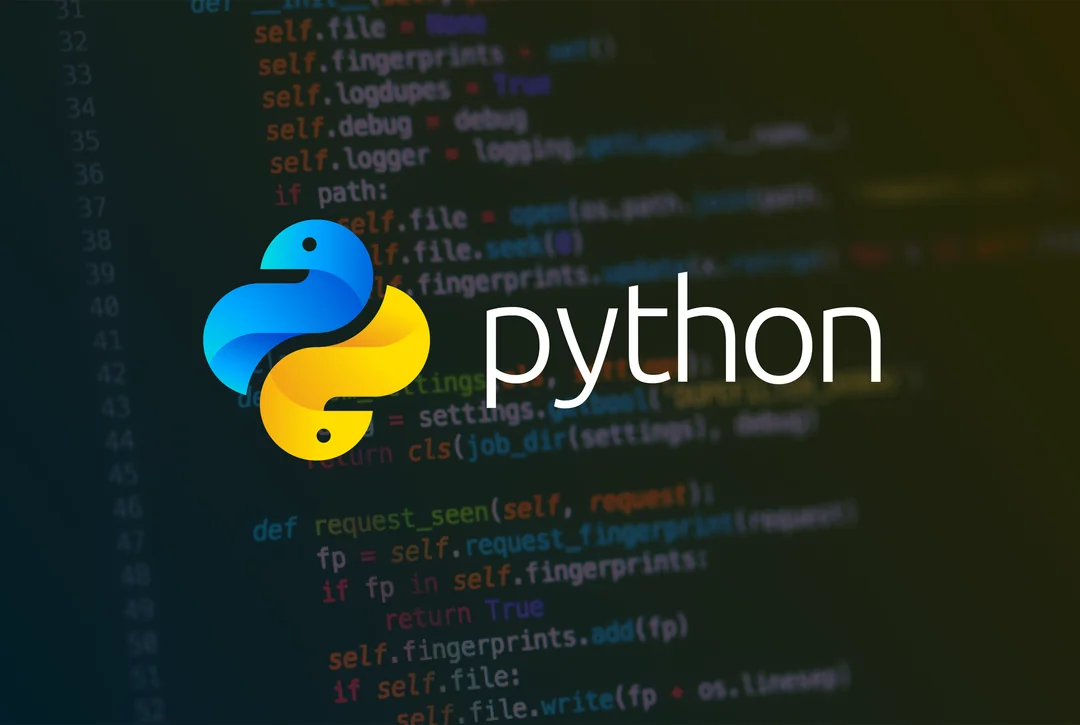
Python is one of the most popular programming languages in the world.
One of its key features is its platform independence.
What does platform independence mean?
How does Python achieve platform independence?
This blog will explore:
How Python programs are executed
The role of the Abstract Syntax Tree (AST)
The concept of bytecode
Why Python is platform-independent while languages like C++ are platform-dependent
We'll break it all down into simple terms with relatable examples.
How Python Executes Your Program
When you write a Python program and run it, a lot happens behind the scenes. Here’s a step-by-step explanation of the process:
1. Your Code as Plain Text
When you write a Python program, it’s just plain text. For example:
x = 10
if x > 5:
print("x is greater than 5")
This code is just a set of instructions written in a human-readable format. However, your computer cannot directly understand it.
2. Tokenization
The Python interpreter first breaks down the code into tokens. These are the smallest meaningful pieces of the code, like:
Keywords (
if
,print
)Variables (
x
)Operators (
=
,>
)Numbers (
10
,5
)
Think of tokens as the building blocks of your code, like bricks for a house.
3. Abstract Syntax Tree (AST)
Next, Python organizes these tokens into a structure called the Abstract Syntax Tree (AST).
The AST is like a blueprint of your code, showing how everything fits together.
Each node in the tree represents a specific construct in your program, such as an assignment, a comparison, or a function call.
For example, the AST for the above code looks something like this:
Module
├── Assign (x = 10)
├── If (x > 5)
├── Body (print("x is greater than 5"))
Why is AST Important?
Error Checking: The AST helps Python catch syntax errors (e.g., missing
:
after anif
statement).Optimization: Python can analyze and optimize the AST before proceeding to the next step.
Foundation for Bytecode: The AST is converted into bytecode, which is what the Python Virtual Machine (PVM) understands.
To Read More About AST, Read My Dedicated Blog on AST.
4. Bytecode Generation
Python compiles the AST into bytecode. Bytecode is a lower-level representation of your code, but it’s still not specific to any operating system or hardware.
Example of bytecode:
1 0 LOAD_CONST 1 (10)
2 STORE_NAME 0 (x)
2 4 LOAD_NAME 0 (x)
6 LOAD_CONST 2 (5)
8 COMPARE_OP 4 (>)
10 POP_JUMP_IF_FALSE 20
3 12 LOAD_NAME 1 (print)
14 LOAD_CONST 3 ('x is greater than 5')
16 CALL_FUNCTION 1
18 POP_TOP
5. Python Virtual Machine (PVM)
The PVM executes the bytecode. It translates the bytecode into machine code that your computer’s processor can understand.
Platform Independence in Python
Here’s the key: Bytecode is platform-independent, meaning it can run on any operating system as long as Python is installed. When you share a Python program or its bytecode (.pyc
file) with someone else, they don’t need to recompile it—they just need the PVM.
How to Run a .pyc
File
Write your Python program (e.g.,
example.py
).Generate the bytecode:
python -m py_compile example.py
This creates a
.pyc
file in the__pycache__
folder.Share the
.pyc
file with your friend.Your friend can run it:
python example.pyc
C++ vs. Python: Why C++ is Platform-Dependent
C++ works differently from Python:
In C++, the code is compiled directly into machine code specific to your system.
Machine code is platform-dependent because it’s tailored to a specific operating system and processor architecture.
If you share your compiled C++ program with a friend who has a different system, it won’t work unless you recompile it on their machine.
Real-Life Analogy
Python: Imagine writing instructions in a common language (like English). Anyone with a dictionary (the Python interpreter) can understand and execute the instructions.
C++: Imagine writing instructions in a local dialect. If someone doesn’t speak that dialect (a different operating system), they can’t understand it.
Summary of Key Concepts
Concept | Role |
Tokenization | Breaks your code into smaller components (e.g., keywords, variables). |
AST | Organizes tokens into a structured, tree-like representation of your code. |
Bytecode | Platform-independent, low-level code that the Python Virtual Machine (PVM) can execute. |
PVM | Executes the bytecode by translating it into machine code specific to your system. |
C++ Compilation | Directly converts code into platform-dependent machine code, requiring recompilation for different systems. |
Conclusion
Python’s platform independence is achieved through its two-step process: compiling to bytecode and then interpreting it with the PVM. The AST ensures your code is error-free and well-structured, while bytecode makes your program portable across platforms. In contrast, languages like C++ are platform-dependent because they generate machine code specific to the system they’re compiled on.
Understanding these concepts not only demystifies how Python works but also highlights why it’s so powerful and versatile. Whether you're sharing Python code or .pyc
files, Python ensures smooth execution on any platform!
Additional Resources:
Check out these links to learn more:
- Python AST Documentation
Learn more about the AST module in Python.
Let's Stay Connected!
I’d love to connect with you! Follow me on:
Stay Updated!
Subscribe to my newsletter for the latest Python tutorials and updates!
Thank You for Reading!
Feel free to reach out with any questions. Happy coding! 🚀
Subscribe to my newsletter
Read articles from Deepak Kumar Mohanty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Deepak Kumar Mohanty
Deepak Kumar Mohanty
Hi there! I'm Deepak Mohanty, a BCA graduate from Bhadrak Autonomous College, affiliated with Fakir Mohan University in Balasore, Odisha, India. Currently, I'm diving deep into the world of Data Science. I'm passionate about understanding the various techniques, algorithms, and applications of data science. My goal is to build a solid foundation in this field and share my learning journey through my blog. I'm eager to explore how data science is used in different industries and contribute to solving real-world problems with data.