Mastering Error Handling in Go: A Step-by-Step Guide to Writing Reliable Software

Table of contents
- What is Error Handling?
- Why is Error Handling Important?
- Error Handling in Go
- Step 1: Using the error Type
- Step 2: Handling Errors Gracefully
- Step 3: Creating Custom Errors
- Step 4: The errors.Is and errors.As Functions
- Step 5: Using defer and recover for Panic Handling
- Step 6: Best Practices for Error Handling
- Conclusion
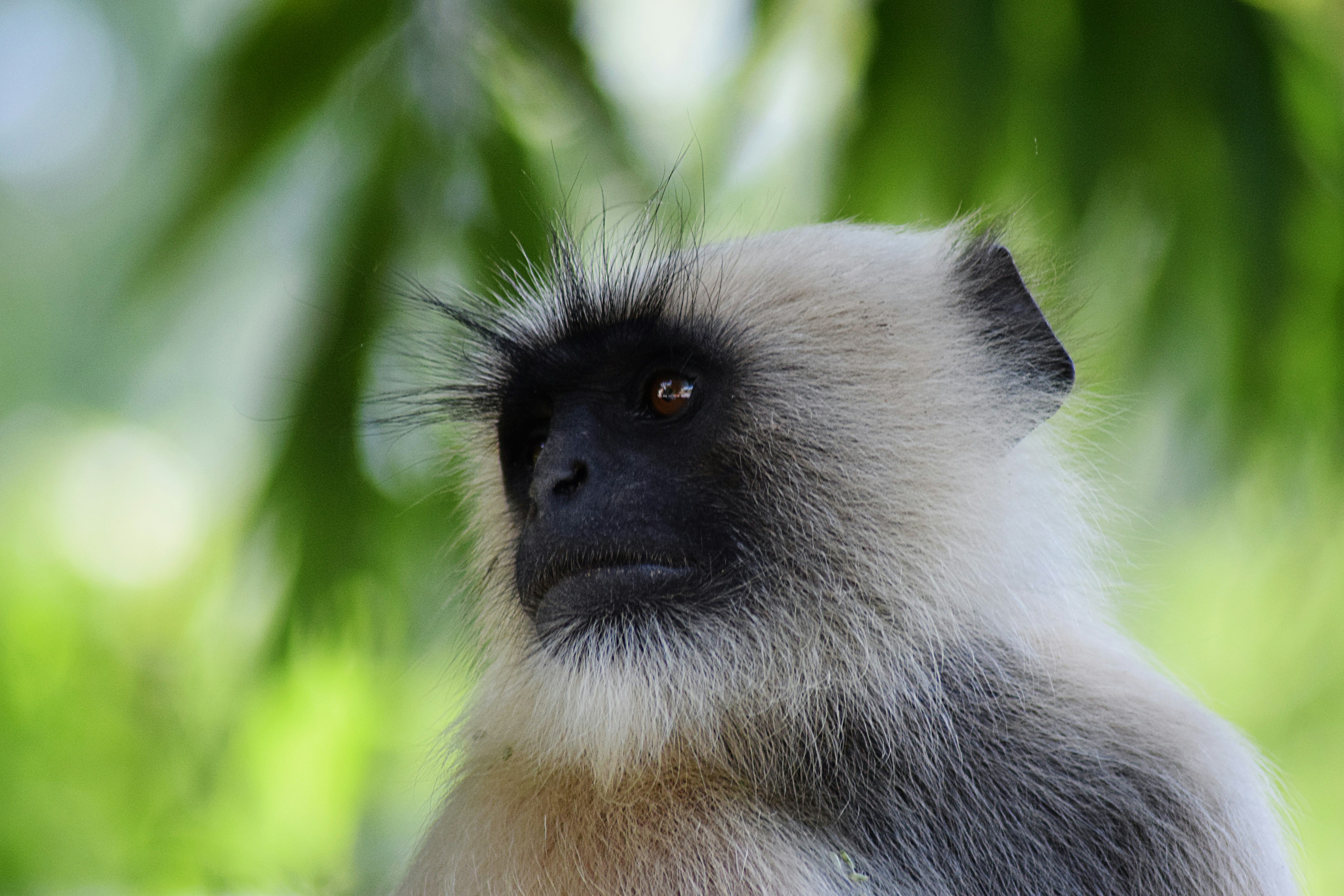
Error handling is an essential aspect of writing reliable software. It ensures that programs gracefully manage unexpected situations instead of crashing. Go has a robust and straightforward approach to error handling, which differs from many other programming languages. This guide will explain error handling step by step in an easy-to-understand way.
What is Error Handling?
Error handling is the process of identifying and responding to errors in a program. For example, if a program tries to read a file that doesn’t exist, it should handle this situation and inform the user instead of stopping unexpectedly.
Why is Error Handling Important?
Prevents Crashes: Your program doesn’t abruptly stop running.
Improves User Experience: Users get meaningful messages instead of cryptic errors.
Ensures Reliability: Helps the program continue functioning in other areas.
Error Handling in Go
In Go, errors are treated as values. They can be created, returned, and passed around like any other data. The standard way of handling errors is by using Go’s built-in error
type.
Step 1: Using the error
Type
The error
type is used to represent errors in Go. A function that can result in an error usually returns two values:
The expected result.
An
error
value indicating if something went wrong.
Example
package main
import (
"errors"
"fmt"
)
// Function that returns an error if input is negative
func checkNumber(n int) (string, error) {
if n < 0 {
return "", errors.New("negative number is not allowed")
}
return "Number is valid", nil
}
func main() {
message, err := checkNumber(-5)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Success:", message)
}
}
Explanation
Line 8: We define a function
checkNumber
that returns two values: a string and an error.Line 10: If the number is negative, we create an error using
errors.New
and return it.Line 15: In
main
, we check iferr
is notnil
. If it’s notnil
, an error occurred, and we print it.
Output
Error: negative number is not allowed
Step 2: Handling Errors Gracefully
Errors should be handled where they occur, allowing the program to continue working if possible.
Example
package main
import (
"fmt"
"strconv"
)
func main() {
num, err := strconv.Atoi("123abc") // Convert string to integer
if err != nil {
fmt.Println("Error:", err) // Handle the error
return
}
fmt.Println("Converted number:", num)
}
Explanation
Line 7: We use
strconv.Atoi
to convert a string to an integer.Line 8: If
err
is notnil
, we print the error and return to stop further execution.
Output
Error: strconv.Atoi: parsing "123abc": invalid syntax
Step 3: Creating Custom Errors
Go allows you to create custom errors for more descriptive and specific error messages.
Example
package main
import (
"fmt"
)
// Custom error function
func divide(a, b int) (int, error) {
if b == 0 {
return 0, fmt.Errorf("cannot divide %d by zero", a)
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
Explanation
Line 7: We use
fmt.Errorf
to format the error message dynamically.Line 13: If
err
is notnil
, the error message is displayed.
Output
Error: cannot divide 10 by zero
Step 4: The errors.Is
and errors.As
Functions
Go provides utilities to check and unwrap errors, especially when working with nested or wrapped errors.
Example: Using errors.Is
package main
import (
"errors"
"fmt"
)
var ErrNotFound = errors.New("item not found")
func findItem(id int) error {
if id != 1 {
return ErrNotFound
}
return nil
}
func main() {
err := findItem(2)
if errors.Is(err, ErrNotFound) {
fmt.Println("Error: The item was not found.")
} else {
fmt.Println("Item found successfully!")
}
}
Explanation
Line 5: We define a custom error
ErrNotFound
.Line 14: We use
errors.Is
to check if the error matchesErrNotFound
.
Output
Error: The item was not found.
Step 5: Using defer
and recover
for Panic Handling
Sometimes, programs encounter severe errors (panics) that can stop execution. Use defer
and recover
to gracefully handle panics.
Example
package main
import "fmt"
func safeDivide(a, b int) {
defer func() {
if r := recover(); r != nil {
fmt.Println("Recovered from panic:", r)
}
}()
fmt.Println("Result:", a/b)
}
func main() {
safeDivide(10, 0)
fmt.Println("Program continues...")
}
Explanation
Line 7: The
defer
function runs at the end ofsafeDivide
, even if there’s a panic.Line 8:
recover
catches the panic and prevents the program from crashing.
Output
Recovered from panic: runtime error: integer divide by zero
Program continues...
Step 6: Best Practices for Error Handling
Check Errors Immediately: Always check for
err
right after a function call.Avoid Panic for Regular Errors: Use
panic
only for unrecoverable situations.Use Meaningful Error Messages: Help users understand what went wrong.
Group Related Errors: Use constants or variables for reusable error messages.
Conclusion
In this article, we covered:
The basics of error handling in Go.
How to handle and create custom errors.
Tools like
errors.Is
,errors.As
, andrecover
for advanced error management.
Error handling ensures that your programs are robust and user-friendly. By practicing these techniques, you’ll write more reliable and professional Go programs.
Stay tuned for more beginner-friendly Go tutorials. Happy coding! 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
