Mastering Time in Go: A Beginner's Journey Through the `time` Package

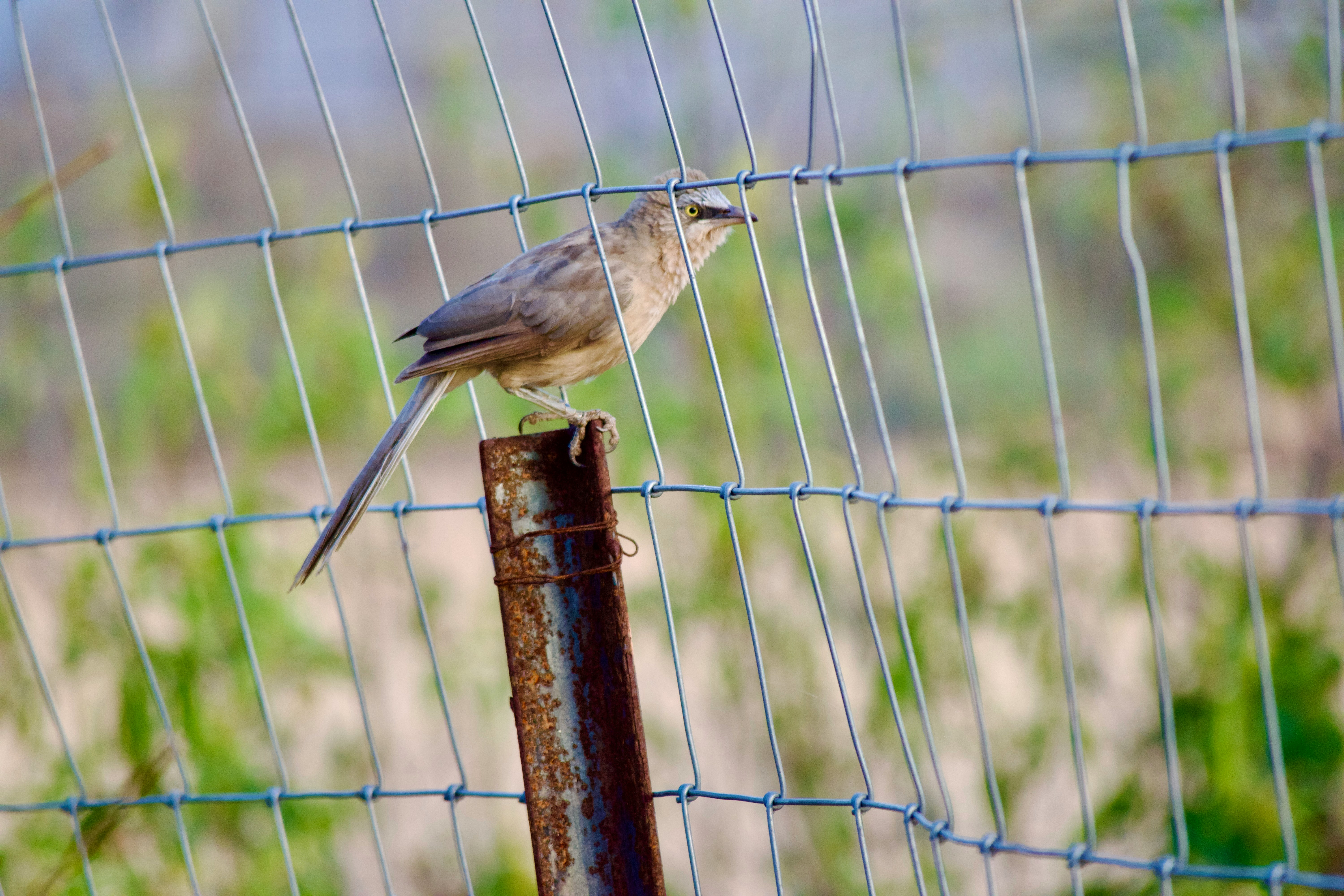
Working with dates and time is an essential part of programming. Whether you're creating schedules, logging events, or measuring durations, Go’s time
package provides all the tools you need.
This beginner-friendly guide will walk you through the basics of time handling step by step.
Why Time Handling is Important
To display current dates and times in programs.
To measure time durations (e.g., time elapsed).
To schedule and automate tasks.
To perform date calculations (e.g., adding days or months).
Getting the Current Time
The time.Now
()
function retrieves the current date and time, including the local timezone.
Example: Displaying the Current Time
package main
import (
"fmt"
"time"
)
func main() {
// Get the current time
currentTime := time.Now()
// Print the current time
fmt.Println(currentTime)
}
Explanation
time.Now
()
: Retrieves the exact time when the program is run, including:The current date.
The time (hour, minute, second).
The timezone.
Output: Prints the timestamp as a full string.
Sample Output:
2024-11-09 15:04:05.123456 +0000 UTC
Formatting Time
Go uses a specific layout reference to format time. The reference date is:
Mon Jan 2 15:04:05 MST 2006
You use parts of this reference date to define your desired format.
Example: Formatting the Current Time
package main
import (
"fmt"
"time"
)
func main() {
// Get the current time
currentTime := time.Now()
// Format the time as YYYY-MM-DD
formattedTime := currentTime.Format("2006-01-02")
// Print the formatted time
fmt.Println("Formatted Time:", formattedTime)
}
Explanation
Reference Layout:
2006
: Represents the year.01
: Represents the month.02
: Represents the day.
currentTime.Format()
: Converts the default time format into the custom format YYYY-MM-DD.
Sample Output:
Formatted Time: 2024-11-09
Parsing Time
You can convert a string into a time.Time
object using time.Parse
. This is useful for working with timestamps or user-provided date strings.
Example: Parsing a Date String
package main
import (
"fmt"
"time"
)
func main() {
// A date string
dateString := "2024-11-09"
// Parse the string into a time.Time object
parsedTime, err := time.Parse("2006-01-02", dateString)
if err != nil {
fmt.Println("Error parsing time:", err)
return
}
// Print the parsed time
fmt.Println("Parsed Time:", parsedTime)
}
Explanation
time.Parse(layout, string)
: Converts a string into atime.Time
object using the specified layout.Error Handling: Always handle errors in case the string doesn't match the expected layout.
Sample Output:
Parsed Time: 2024-11-09 00:00:00 +0000 UTC
Adding and Subtracting Time
You can manipulate dates by adding or subtracting time using the Add
or AddDate
methods.
Example: Adding Days to the Current Date
package main
import (
"fmt"
"time"
)
func main() {
// Get the current time
currentTime := time.Now()
// Add 7 days to the current time
futureTime := currentTime.AddDate(0, 0, 7)
// Print the new date
fmt.Println("Future Time:", futureTime)
}
Explanation
AddDate(years, months, days)
: Adjusts the time by the specified number of years, months, or days.Output: Displays the new date and time after adding 7 days.
Sample Output:
Future Time: 2024-11-16 15:04:05.123456 +0000 UTC
Key Takeaways
Use
time.Now
()
to get the current local time.Use
Format()
to display time in custom formats like YYYY-MM-DD.Use
time.Parse()
to convert strings into time objects.Use
AddDate()
for date manipulation (adding or subtracting days).
Conclusion
Time handling is a critical skill in programming, and Go makes it easy with the time
package. Start practicing these examples to become comfortable working with dates and times in your programs.
Stay tuned for more beginner-friendly tutorials as we explore Go's powerful features. 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
