Mastering Data Type Conversions in Go: A Beginner's Journey

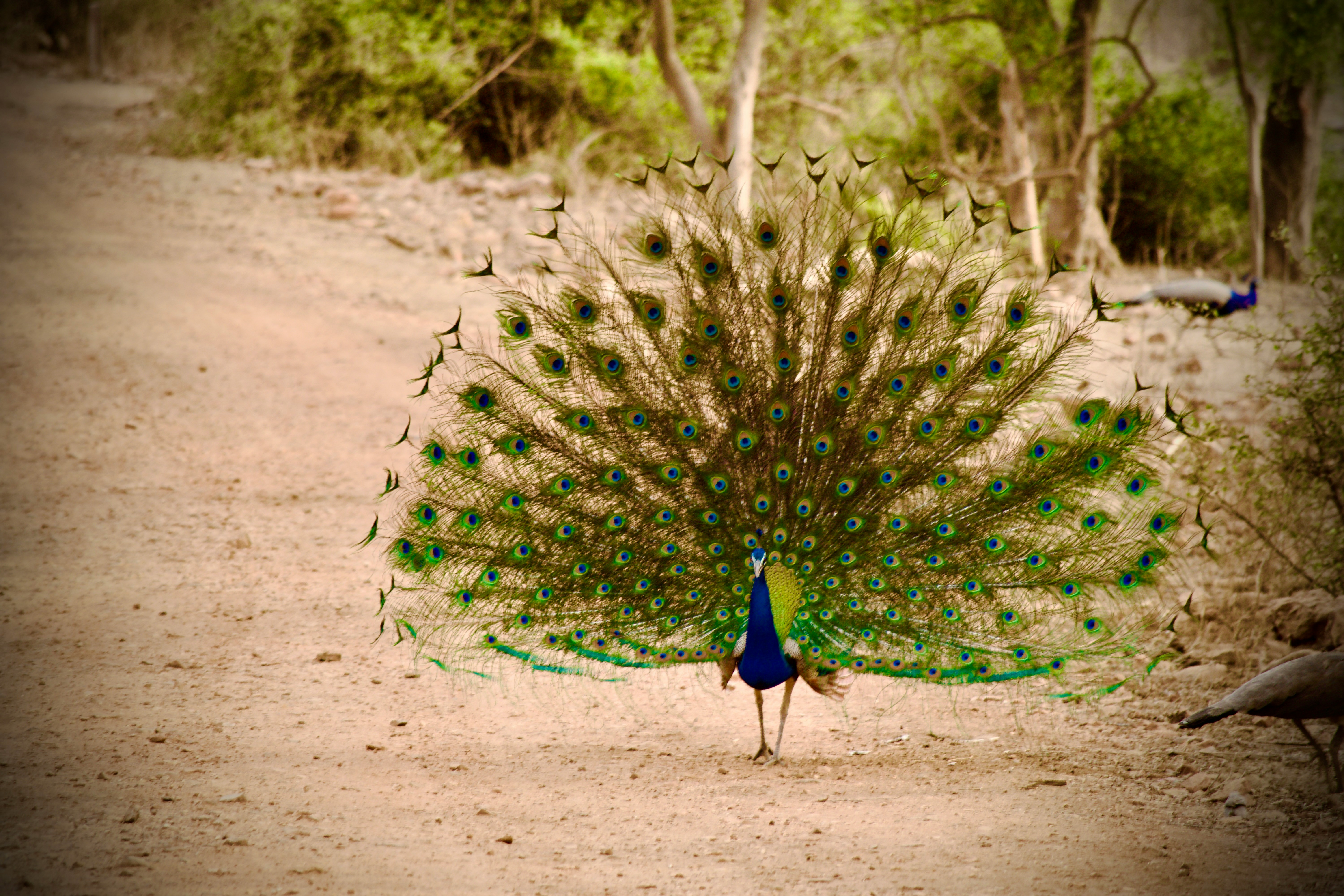
Data conversion is a fundamental aspect of programming. It allows you to transform data from one type to another, enabling seamless operations in your programs. Go, being a statically typed language, provides robust support for type conversions.
In this article, we’ll explore conversions in Go, step by step, in beginner-friendly language with examples.
What are Conversions?
Conversions are operations that change a value from one data type to another.
For example:
Converting an integer to a float.
Converting a string to an integer.
Why Are Conversions Necessary?
Data compatibility: Ensures that operations between different types work correctly.
Precision control: Allows you to handle floating-point numbers, integers, and strings appropriately.
Program correctness: Prevents type mismatches that could lead to runtime errors.
Types of Conversions in Go
Numeric conversions: Converting numbers between different types (e.g., int to float).
String conversions: Converting other types to strings and vice versa.
Numeric Conversions
Go does not allow implicit type conversions. This means you must explicitly convert one type to another.
Example: Converting an int
to a float
package main
import (
"fmt"
)
func main() {
// Declare an integer variable
var myInt int = 42
// Convert the integer to a float
myFloat := float64(myInt)
// Print both values with explicit formatting for the float
fmt.Println("Integer:", myInt)
fmt.Printf("Float: %.1f\n", myFloat) // Forces one decimal place
}
Explanation
float64(myInt)
: Converts the integermyInt
to a floating-point number of typefloat64
.Output:
Integer: 42 Float: 42.0
Important Note
Go is strict about conversions. An int
cannot automatically be used as a float
, so you must explicitly convert it.
Example: Converting a float
to an int
package main
import (
"fmt"
)
func main() {
// Declare a float variable
var myFloat float64 = 42.99
// Convert the float to an integer
myInt := int(myFloat)
// Print both values
fmt.Println("Float:", myFloat)
fmt.Println("Integer:", myInt)
}
Explanation
int(myFloat)
: Converts the floating-point numbermyFloat
to an integer by truncating the decimal part.Output:
Float: 42.99 Integer: 42
Key Point: Truncation
When converting a float to an int, the fractional part is discarded (not rounded).
String Conversions
Go provides built-in functions to convert between strings and other types using the strconv
package.
Example: Converting an int
to a string
package main
import (
"fmt"
"strconv"
)
func main() {
// Declare an integer
var myInt int = 123
// Convert the integer to a string
myString := strconv.Itoa(myInt)
// Print the string
fmt.Println("String:", myString)
}
Explanation
strconv.Itoa()
: Converts an integer to a string.Output:
String: 123
Example: Converting a string
to an int
package main
import (
"fmt"
"strconv"
)
func main() {
// Declare a string
var myString string = "456"
// Convert the string to an integer
myInt, err := strconv.Atoi(myString)
if err != nil {
fmt.Println("Error:", err)
return
}
// Print the integer
fmt.Println("Integer:", myInt)
}
Explanation
strconv.Atoi()
: Converts a string to an integer.Error Handling: Always check for errors when converting strings to numbers because the string might not represent a valid number.
Output:
Integer: 456
Conversion Between Strings and Bytes
Go treats strings and byte slices ([]byte
) differently. You can convert between them for efficient handling of textual data.
Example: Converting a string
to a []byte
package main
import (
"fmt"
)
func main() {
// Declare a string
myString := "Hello, Go!"
// Convert the string to a byte slice
byteSlice := []byte(myString)
// Print the byte slice
fmt.Println("Byte Slice:", byteSlice)
}
Explanation
[]byte(myString)
: Converts the string into a slice of bytes.Output: Displays the ASCII values of the characters in the string.
Byte Slice: [72 101 108 108 111 44 32 71 111 33]
Example: Converting a []byte
to a string
package main
import (
"fmt"
)
func main() {
// Declare a byte slice
byteSlice := []byte{72, 101, 108, 108, 111}
// Convert the byte slice to a string
myString := string(byteSlice)
// Print the string
fmt.Println("String:", myString)
}
Explanation
string(byteSlice)
: Converts a byte slice back into a string.Output: Displays the reconstructed string.
String: Hello
Key Rules for Conversions
Explicit Conversions Only: Go does not allow implicit type conversions.
Loss of Precision: Converting
float
toint
discards the decimal part.Error Handling: Always handle errors when converting strings to numbers.
Conclusion
Conversions in Go are explicit and straightforward. Understanding how to work with numeric, string, and byte conversions is essential for building robust Go applications. Practice these examples to build a strong foundation, and stay tuned for more beginner-friendly tutorials!
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
