Compiled vs. Interpreted Languages: What's the Difference?
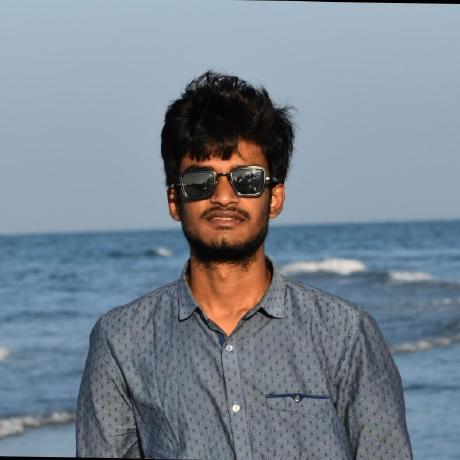
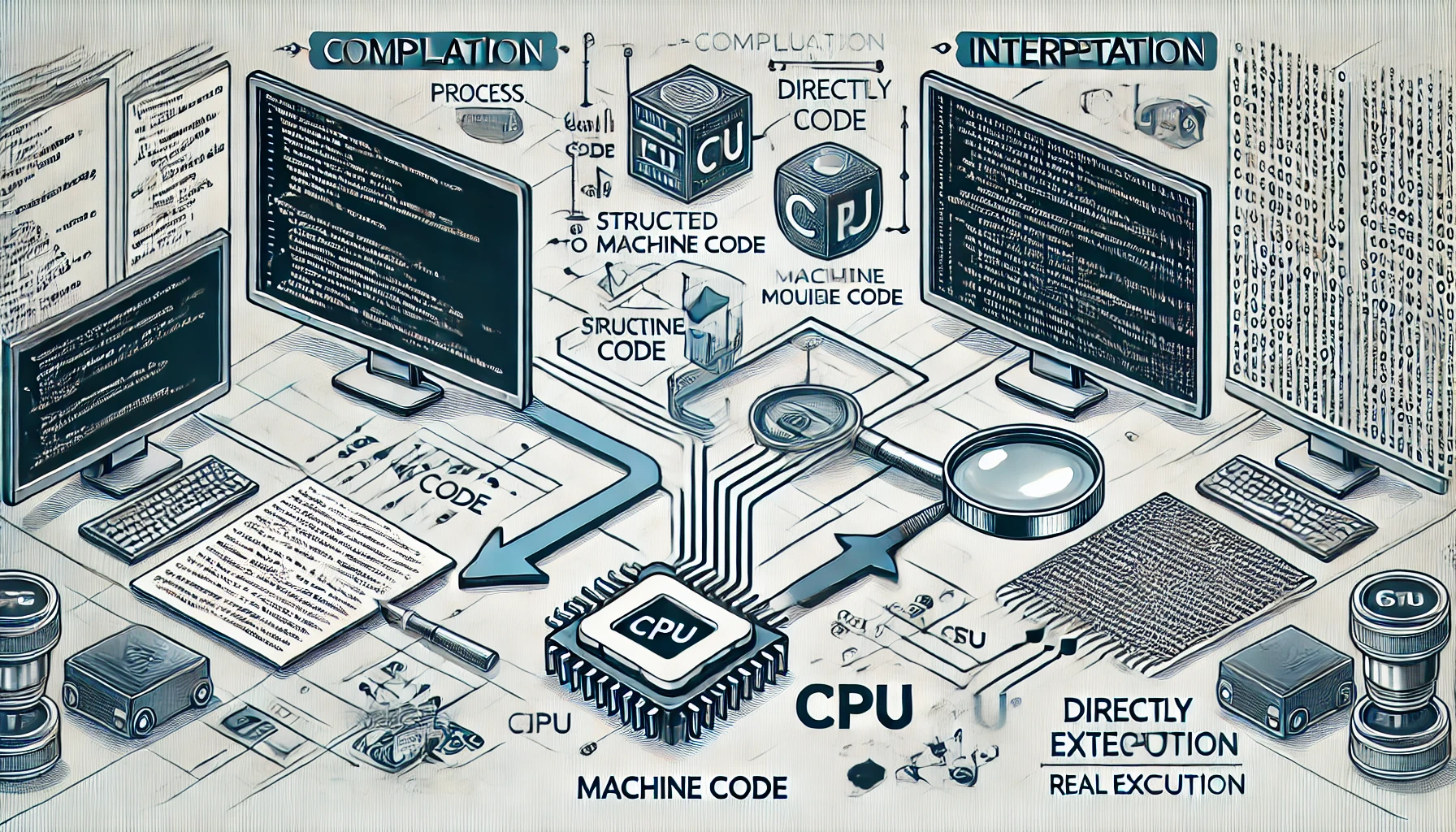
Understanding how programming languages are executed is fundamental for developers. This article explores the differences between compiled and interpreted languages, providing examples and diagrams to illustrate key concepts.
Table of Contents
Introduction
Understanding how your code is transformed into executable instructions is crucial when writing a program. Programming languages are generally classified into two categories based on their execution model:
Compiled Languages
Interpreted Languages
Each approach has its advantages and trade-offs, affecting performance, portability, and development workflow. Let's dive into the differences between these two execution models.
What is a Compiled Language?
A compiled language requires a compiler to translate the high-level source code into machine code before execution. The machine code is a binary format that the computer's processor can execute directly.
Compilation Process
The compilation process involves several steps:
Source Code: The programmer writes code in a high-level language.
Compilation: The compiler translates the source code into machine code.
Executable Program: An executable file is generated.
Execution: The executable runs on the target machine's hardware.
Diagram: Compilation Process
[Source Code] --(Compiler)--> [Machine Code] --> [Execution]
Examples of Compiled Languages
C and C++
Go
Rust
Fortran
Example: Compiling a C Program
// hello.c
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Compilation and Execution
gcc -o hello hello.c # Compiles the code into an executable named 'hello'
./hello # Executes the program
What is an Interpreted Language?
An interpreted language uses an interpreter to execute the source code line by line at runtime. There is no separate compilation step that produces machine code ahead of time.
Interpretation Process
The interpretation process includes:
Source Code: The programmer writes code in a high-level language.
Interpreter: The interpreter reads and executes the code line by line.
Execution: The code is executed on the fly.
Diagram: Interpretation Process
[Source Code] --(Interpreter)--> [Execution]
Examples of Interpreted Languages
Python
Ruby
PHP
JavaScript
Example: Running a Python Script
# hello.py
print("Hello, World!")
Execution
python hello.py # Executes the script using the Python interpreter
Key Differences Between Compiled and Interpreted Languages
Aspect | Compiled Languages | Interpreted Languages |
Translation Time | Before execution (compile time) | During execution (runtime) |
Execution Speed | Faster, as code is pre-translated to machine code | Slower, due to line-by-line interpretation |
Portability | Platform-dependent executables | Platform-independent code (requires interpreter) |
Error Detection | Compile-time errors caught before execution | Runtime errors may occur during the execution |
Source Code Exposure | Source code can remain hidden | The source code must be available to the interpreter |
Distribution | Distribute executables without source code | Source code needs to be shared with users |
Hybrid Approaches
Some languages combine compilation and interpretation to leverage their benefits.
Example: Java
Compilation to Bytecode: Java source code is compiled into an intermediate form called bytecode.
Execution by JVM: The Java Virtual Machine (JVM) interprets the bytecode or uses Just-In-Time (JIT) compilation to convert it into machine code at runtime.
Diagram: Java Execution Process
[Java Source Code] --(Compiler)--> [Bytecode] --(JVM)--> [Execution]
Example: JavaScript
Modern JavaScript engines use JIT compilation to improve performance.
- Interpretation and JIT Compilation: The engine interprets JavaScript code and compiles frequently executed code paths into machine code at runtime.
Which One Should You Choose?
The choice between a compiled or interpreted language depends on various factors:
Performance Needs: Compiled languages are generally faster due to ahead-of-time compilation.
Development Speed: Interpreted languages offer faster development cycles due to on-the-fly execution.
Portability: Interpreted languages are more portable across different platforms.
Project Complexity: Large, performance-critical applications may benefit from compiled languages.
Community and Ecosystem: Consider the libraries, frameworks, and tools available.
Conclusion
Understanding the differences between compiled and interpreted languages is essential for making informed decisions in software development. Compiled languages offer speed and efficiency, while interpreted languages provide flexibility and ease of use. Hybrid approaches aim to combine the best of both worlds.
Thank you for reading! If you have any questions or thoughts, feel free to share them in the comments below.
Subscribe to my newsletter
Read articles from Abdur Rakib directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
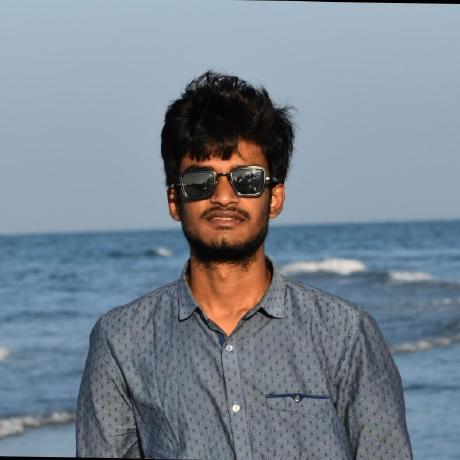