Understanding the Flutter Container Widget
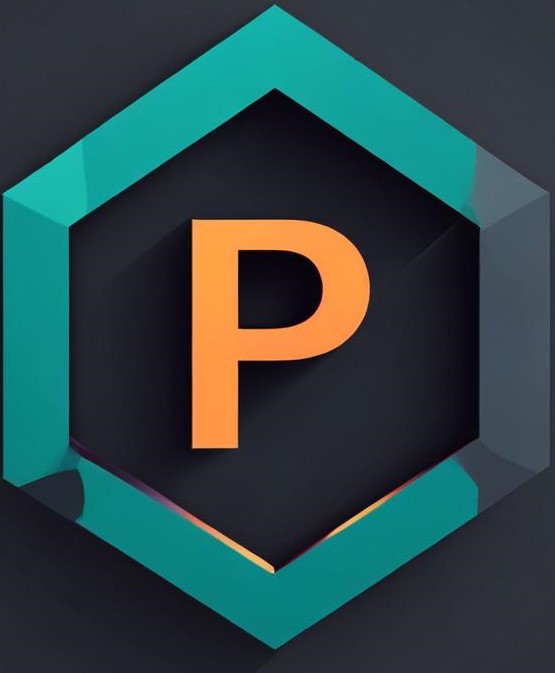
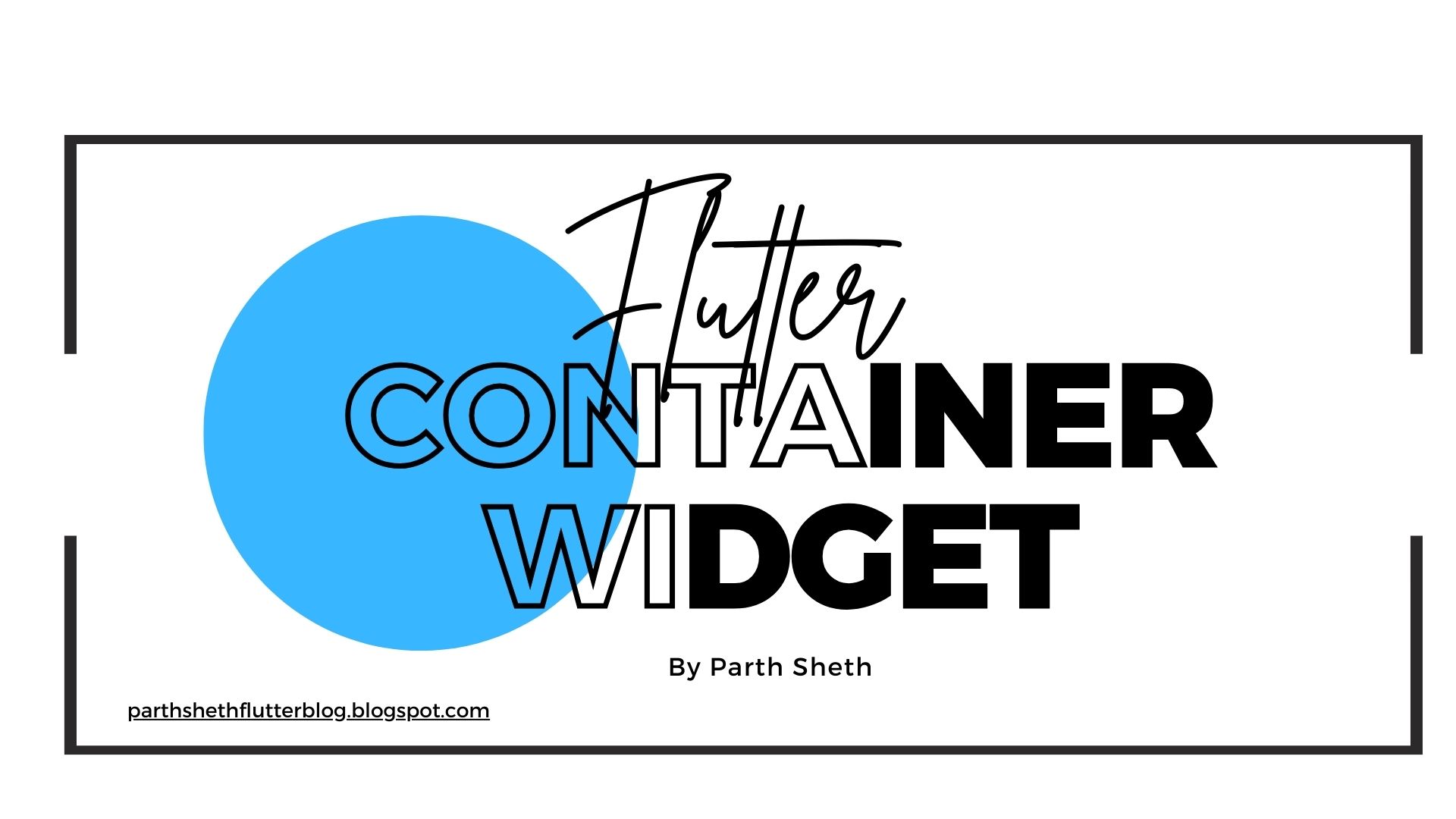
In Flutter, the Container
widget is one of the most commonly used and versatile widgets. It allows you to create a box model in your app's layout. You can use it to apply padding, margins, borders, backgrounds, and much more. If you're just starting with Flutter, the Container
widget is an essential concept to master, as it plays a significant role in building flexible and customized UIs.
What is the Container Widget?
A Container
in Flutter is a box model widget that can contain a single child widget and is used to customize and style the layout. You can think of it as a "wrapper" that gives you the ability to control the size, position, decoration, and many other aspects of its child widget. The Container
widget doesn’t have a visual representation on its own but instead applies the customizations to its child widget.
Common Use Cases for Container
Adding padding or margin around a widget.
Setting a fixed height, width, or both.
Adding background color or image to the widget.
Applying borders or rounded corners.
Positioning elements on the screen using alignment.
Transforming widgets with rotation, scaling, etc.
Properties of the Container
Widget
The Container
widget comes with several important properties that allow us to modify its appearance and behavior. Here are the most commonly used ones:
child
This is the widget that is wrapped by theContainer
. You can only have one child widget in aContainer
.width and height
These properties allow you to set a fixed width and height for theContainer
. If you don't provide these values, theContainer
will size itself based on its child widget.padding
Padding adds space inside theContainer
, between its border and its child. It's useful for ensuring that the child widget doesn’t touch the edges of the container.margin
Margin is the space outside theContainer
. It’s useful for creating space between the container and other widgets.decoration
Thedecoration
property allows you to style theContainer
with things like a background color, gradient, borders, and rounded corners.alignment
Thealignment
property allows you to position the child widget inside theContainer
. By default, the child widget is aligned at the top-left corner.constraints
You can use this to apply additional size constraints to theContainer
, such as a minimum or maximum width/height.transform
Thetransform
property allows you to apply a 2D transformation to theContainer
, like scaling, rotating, or translating.color
This is a shortcut for setting a solid background color for theContainer
.
Example 1: Basic Container with Padding and Color
Let's start with a simple example that demonstrates how to create a Container
with padding and a background color:
Container(
padding: EdgeInsets.all(20),
color: Colors.blue,
child: Text(
'Hello, Flutter!',
style: TextStyle(color: Colors.white),
),
)
In this example:
padding: EdgeInsets.all(20)
gives the text 20 pixels of space around it within the container.color:
Colors.blue
sets the background color of theContainer
to blue.The
Text
widget is the child of theContainer
and will be displayed with white text.
Example 2: Container with Margin, Border, and Rounded Corners
Next, let's explore a more advanced example where we apply margin, border, and rounded corners:
Container(
margin: EdgeInsets.all(20),
padding: EdgeInsets.all(10),
decoration: BoxDecoration(
color: Colors.green,
border: Border.all(color: Colors.black, width: 2),
borderRadius: BorderRadius.circular(10),
),
child: Text(
'Styled Container',
style: TextStyle(color: Colors.white),
),
)
In this example:
margin: EdgeInsets.all(20)
creates 20 pixels of space outside theContainer
.padding: EdgeInsets.all(10)
adds 10 pixels of space inside theContainer
, around theText
.decoration: BoxDecoration
is used to style theContainer
:The background color is set to green.
A black border with 2-pixel width is added.
The corners are rounded with a radius of 10 pixels.
Example 3: Container with Constraints and Alignment
Sometimes, you may want to control the size of the Container
and align its child inside. You can do this using the constraints
and alignment
properties.
Container(
constraints: BoxConstraints(
maxWidth: 200,
maxHeight: 100,
),
alignment: Alignment.center,
color: Colors.orange,
child: Text(
'Centered Text',
style: TextStyle(color: Colors.white),
),
)
Here:
The
constraints
property is used to set the maximum width to 200 pixels and the maximum height to 100 pixels.alignment:
Alignment.center
centers theText
widget inside theContainer
.
Example 4: Container with Transformation (Rotation)
You can also apply transformations to your Container
to achieve effects like rotation, scaling, or translating the widget.
Container(
color: Colors.purple,
width: 150,
height: 150,
child: Transform.rotate(
angle: 0.5, // Rotates the container by 0.5 radians
child: Center(
child: Text(
'Rotated Text',
style: TextStyle(color: Colors.white),
),
),
),
)
In this example:
The
Container
has a fixed width and height of 150 pixels.The
Transform.rotate
widget is used to rotate theContainer
by 0.5 radians.
Example 5: Using Container
for Layout (Flex)
Container
is also useful for layout purposes. Here's an example where we use Container
within a Row
widget to create a flexible layout.
Row(
children: [
Container(
color: Colors.red,
width: 100,
height: 100,
child: Center(child: Text('Box 1')),
),
Container(
color: Colors.green,
width: 100,
height: 100,
child: Center(child: Text('Box 2')),
),
Container(
color: Colors.blue,
width: 100,
height: 100,
child: Center(child: Text('Box 3')),
),
],
)
In this example:
Three
Container
widgets are placed inside aRow
.Each
Container
has a fixed size, and the text inside is centered.
Conclusion
The Container
widget in Flutter is incredibly powerful and can be used in a variety of ways to style and position elements in your app. Whether you're adjusting size, adding padding, or applying complex transformations, Container
is an essential widget in every Flutter developer's toolkit.
By combining different properties like decoration
, alignment
, and padding
, you can achieve complex UI designs and layouts. We hope this blog has helped you understand the versatility of the Container
widget and how to use it effectively in your Flutter apps.
Happy Coding!! 🙂
Subscribe to my newsletter
Read articles from Parth Sheth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
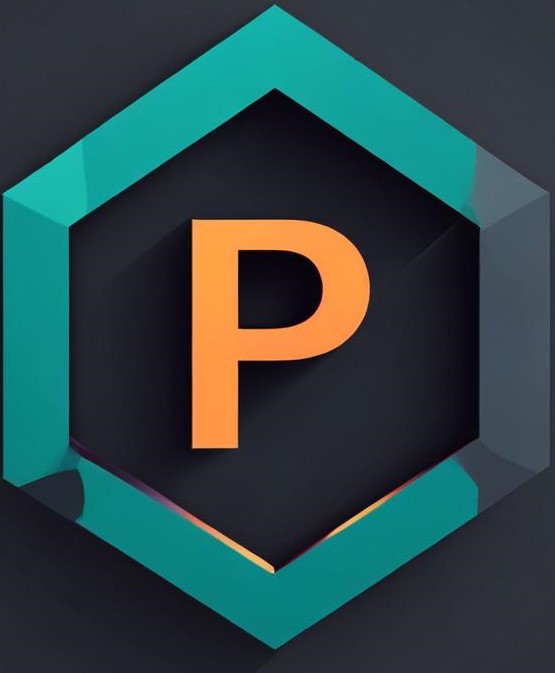
Parth Sheth
Parth Sheth
"🚀 Fluttering through the digital cosmos, I am Parth Sheth, a passionate architect of interactive marvels in the realm of Flutter development. With a fervent love for coding and an insatiable curiosity for the ever-evolving tech landscape, I embark on a perpetual quest to redefine the boundaries of innovation. From sleek UI designs to seamless user experiences, I orchestrate symphonies of code that resonate with elegance and functionality. 💻✨ My journey in Flutter is more than just a profession; it's a thrilling odyssey where creativity meets craftsmanship. Armed with a keen eye for detail and an arsenal of cutting-edge tools, I navigate the complexities of app development with finesse, crafting solutions that not only meet but exceed expectations. 🎨🔧 But beyond the lines of code lies a deeper commitment—to inspire, empower, and elevate. Through my words, my work, and my unwavering dedication to excellence, I strive to ignite the spark of imagination in others, fostering a community where innovation knows no bounds. 🌟💡 So come, fellow adventurers, and join me on this exhilarating expedition into the unknown. Together, let's sculpt the future, one pixel at a time, and unleash the full potential of Flutter's boundless possibilities. Connect with me, and let's embark on this extraordinary voyage—together, we shall chart new horizons and redefine what's possible in the digital landscape. 🌍🔍"