A Complete Guide to Optimizing webpack with Preload, Prefetch, and Dynamic Imports
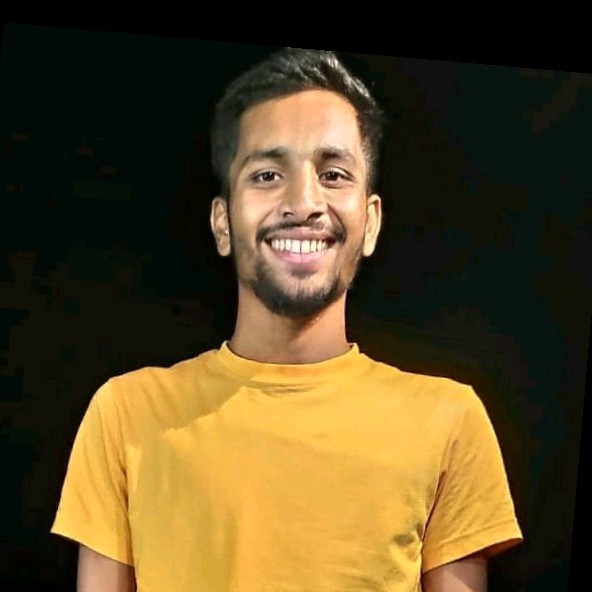
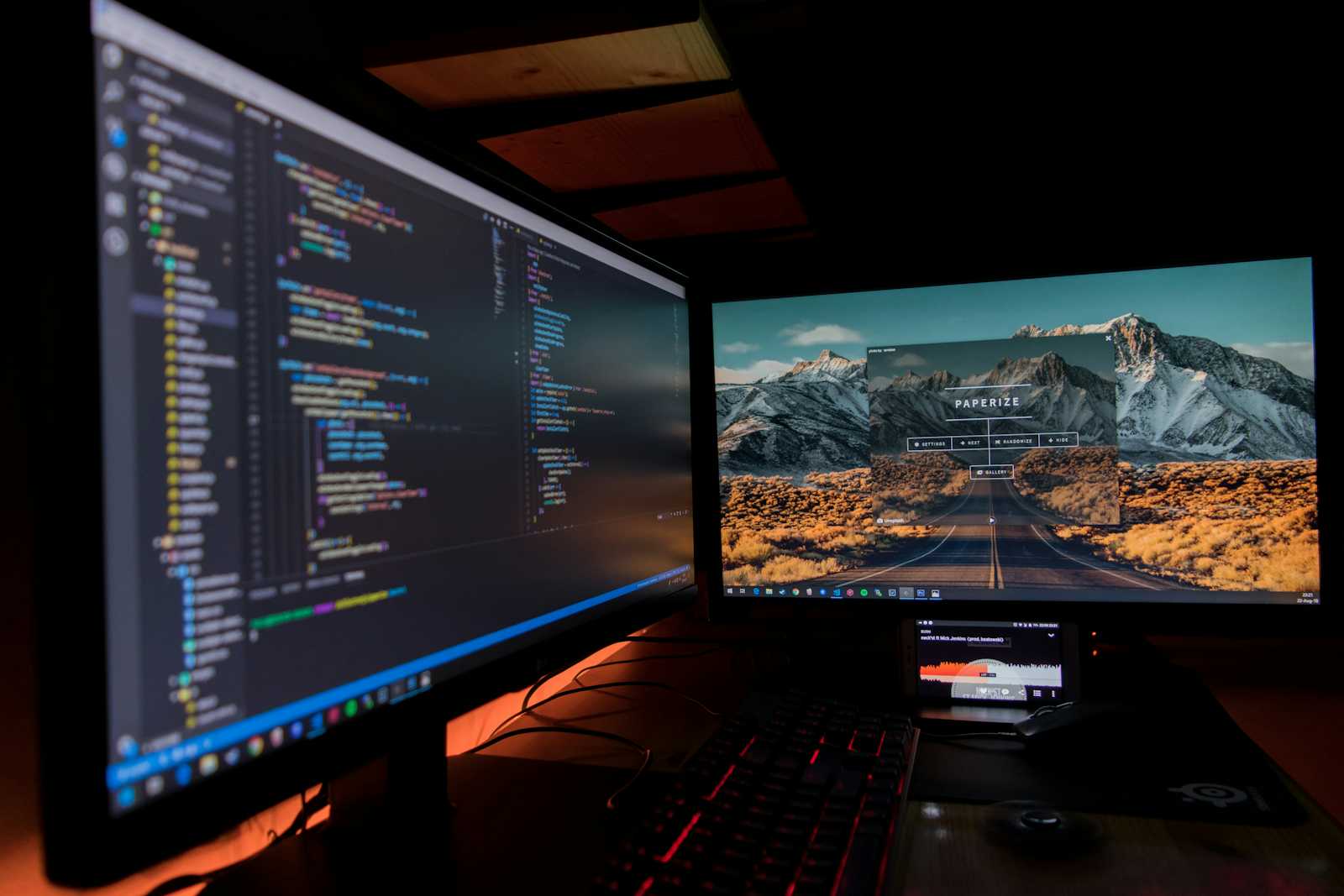
What's the Fuss About Module Loading? π€
Imagine you're building a complex e-commerce site. Your homepage needs to load quickly, but it also includes:
Product Catalog
Shopping cart
User reviews
Chat support
Analytics
Loading everything at once? That's like packing your entire wardrobe for a weekend trip! π§³
Why Do We Need Different Loading Strategies? π―
Let's look at a real problem:
// DON'T DO THIS
import HomePage from './pages/home';
import ProductCatalog from './pages/catalog';
import ShoppingCart from './components/cart';
import ChatSupport from './components/chat';
import Analytics from './utils/analytics';
function App() {
// Everything loads upfront π±
// User waits longer than necessary
}
The Problems:
Slower initial page load
Wasted bandwidth
Poor user experience
Unnecessary memory usage
How Can We Fix This? π οΈ
1. Preload: The "I Need It Now!" Strategy
What is Preload?
Think of preloading as a VIP pass to a concert. It tells Webpack, "This resource is critical; get it immediately!"
What Webpack Does?
When you use webpackPreload, Webpack automatically injects <link> tags into your HTML's <head> section. <link rel=βpreloadβ>
// Critical homepage content
const loadHomePage = async () => {
try {
const module = await import(
/* webpackPreload: true */
'./pages/home'
);
return module.default;
} catch (error) {
console.error('Failed to load homepage:', error);
}
};
When to Use:
Hero images
Above-the-fold content
Primary navigation
User authentication state
Corner Cases:
// CAREFUL: Don't preload everything!
const loadMultiple = async () => {
// β BAD: Too many preloads
const [header, footer, sidebar, main] = await Promise.all([
import(/* webpackPreload: true */ './header'),
import(/* webpackPreload: true */ './footer'),
import(/* webpackPreload: true */ './sidebar'),
import(/* webpackPreload: true */ './main')
]);
// β
BETTER: Prioritize critical content
const header = await import(/* webpackPreload: true */ './header');
const [footer, sidebar] = await Promise.all([
import(/* webpackPrefetch: true */ './footer'),
import('./sidebar')
]);
};
2. Prefetch: The "I'll Need It Soon" Strategy
What is Prefetch?
Think of prefetch like ordering your coffee while you're still in line - it'll be ready when you need it.
What Webpack Does?
When you use webpackPrefetch, Webpack automatically injects <link> tags into your HTML's <head> section. <link rel=βprefetchβ>
// Product catalog that users often visit
const loadCatalog = async () => {
try {
const module = await import(
/* webpackPrefetch: true */
'./pages/catalog'
);
return module.default;
} catch (error) {
console.error('Failed to load catalog:', error);
}
};
Smart Usage Example π‘:
// Prefetch based on user behavior
function ProductList() {
useEffect(() => {
// Prefetch product details when user hovers category
const prefetchProductDetails = () => {
import(/* webpackPrefetch: true */ './ProductDetails');
};
document.querySelector('.category-list')
.addEventListener('mouseover', prefetchProductDetails);
}, []);
}
3. Dynamic Import: The "I'll Load It When I Need It" Strategy
What is Dynamic Import?
Think of it like ordering food - you only place the order when you're ready to eat.
// Chat support - load only when clicked
const loadChatSupport = async () => {
const button = document.getElementById('chat-button');
button.addEventListener('click', async () => {
const module = await import('./components/chat');
module.initChat();
});
};
Real-World Scenarios and Solutions π
Scenario 1: E-commerce Product Page
class ProductPage {
constructor() {
// Critical: Load immediately
this.loadProductInfo();
// Likely needed: Prefetch
this.prepareShoppingCart();
// Optional: Load on interaction
this.setupUserReviews();
}
async loadProductInfo() {
const info = await import(/* webpackPreload: true */ './product-info');
info.render();
}
async prepareShoppingCart() {
// User likely to add to cart
import(/* webpackPrefetch: true */ './shopping-cart');
}
setupUserReviews() {
const reviewTab = document.querySelector('#reviews-tab');
reviewTab.onclick = async () => {
const reviews = await import('./reviews');
reviews.show();
};
}
}
Scenario 2: Admin Dashboard
class AdminDashboard {
constructor() {
this.setupNavigation();
}
setupNavigation() {
// Preload critical analytics
import(/* webpackPreload: true */ './basic-analytics');
// Prefetch likely needed reports
import(/* webpackPrefetch: true */ './monthly-reports');
// Load heavy features on demand
document.querySelector('#export-btn').onclick = async () => {
const { exportToPDF } = await import('./pdf-exporter');
exportToPDF();
};
}
}
I have built and demo app on loading strategies here what it does:
The Home (preload) and About (prefetch) tabs show identical start times in their timing display, as both modules begin loading when the page initially loads.
The Contact tab (dynamic import) shows a different start time - specifically the moment when you click the Contact button.
If you're interested in trying it out, you can find it on my GitHub.
Conclusion π
Smart module loading isn't just about using the right webpack features - it's about understanding your application's needs and user behavior. Use these strategies wisely, monitor their impact, and always test with real-world conditions!
Remember:
Preload what you need now
Prefetch what you'll need soon
Dynamic import what you might need later
Happy Coding π§βπ»
β Basavaraj Patil
Subscribe to my newsletter
Read articles from Basavaraj Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
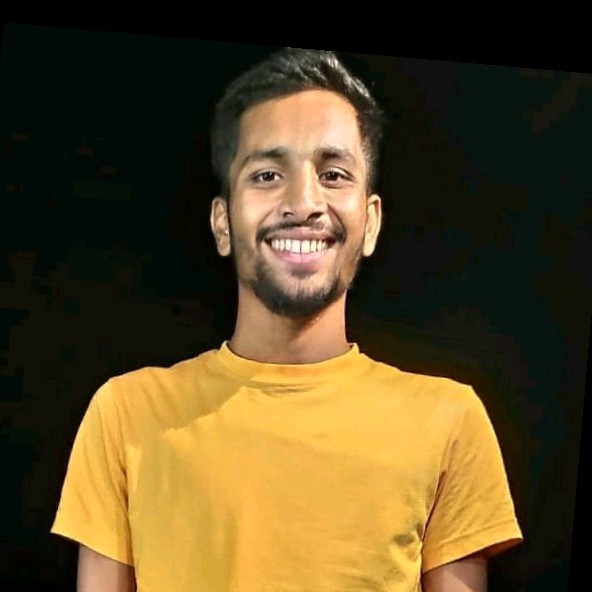
Basavaraj Patil
Basavaraj Patil
I am working as a software developer for more than 1year now, I am interested in writing code and blogs.