Beginner's Guide to Go Programming: From Basics to Advanced Concepts

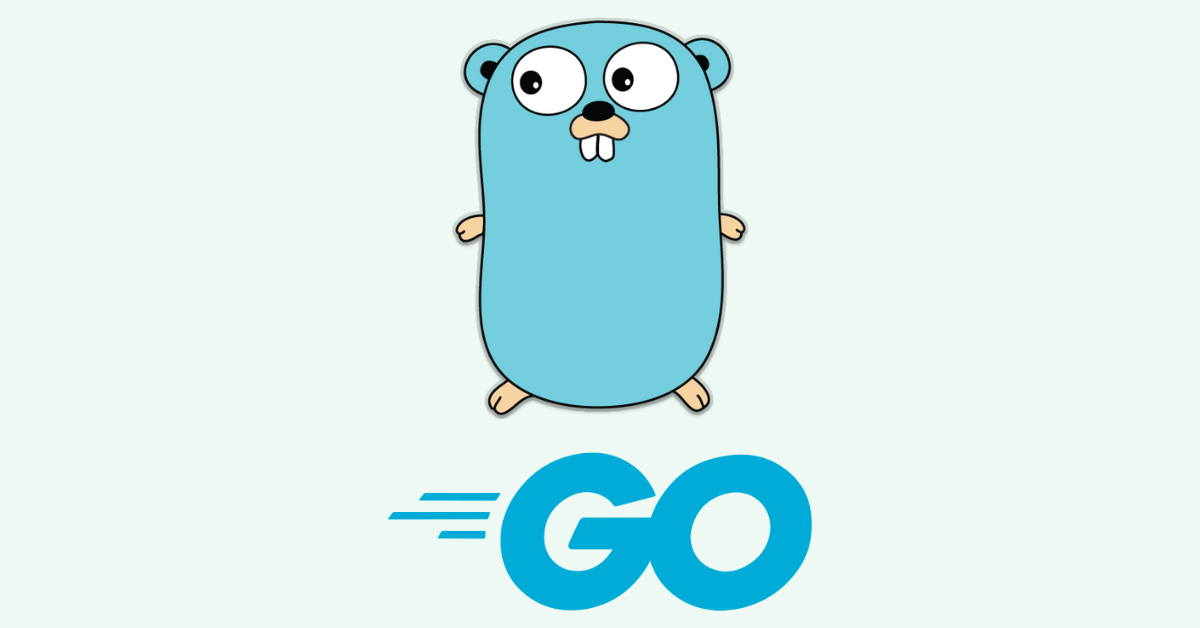
Go is a modern, fast, and efficient programming language created by Google, widely used for backend development, microservices, and cloud-based applications.
What is Go?
Go is a statically typed, compiled language designed for simplicity, efficiency, and concurrency.
Known for its easy-to-read syntax and performance comparable to C/C++.
Used by large organizations like Google, Docker, and Kubernetes.
It has garbage collection, package system, first class functions, lexical scope a system call interface, and immutable strings in which text is generally encoded in UTF-8.
It has no implicit numeric conversions, no constructors or destructors, no operator overloading, no default parameter values, no inheritance, no generics, no exceptions, no macros, no function annotations, and no thread-local storage. The language is mature and stable, and guarantees backwards compatibility: older Go programs can be compiled and run with newer versions of compilers and standard libraries.
Steps to Get Started with Go
1. Install Go
Download Go from the official website.
Follow the installation guide for your operating system.
Verify installation:
bashCopy codego version
2. Set Up Your Development Environment
Use an editor like VS Code with the Go extension for syntax highlighting, code formatting, and debugging.
Set up your Go workspace:
By default, Go uses the
$GOPATH
directory (usually~/go
).Your projects will typically be in
$GOPATH/src
.
3. Write Your First Program
Create a file called
hello.go
:goCopy codepackage main import "fmt" func main() { fmt.Println("Hello, World!") }
Run it:
bashCopy codego run hello.go
4. Learn the Basics
Variables and Types:
goCopy codevar a int = 10 b := "Go is great!" // shorthand
Functions:
goCopy codefunc add(a int, b int) int { return a + b }
Control Structures:
goCopy codefor i := 0; i < 5; i++ { fmt.Println(i) } if a > b { fmt.Println("a is greater") } else { fmt.Println("b is greater") }
Data Structures:
- Arrays, slices, maps, and structs.
5. Understand Key Concepts
Concurrency: Go's goroutines and channels make concurrent programming simple.
goCopy codego func() { fmt.Println("Running in a goroutine") }()
Error Handling: Go uses errors, not exceptions.
goCopy codeif err != nil { fmt.Println("An error occurred:", err) }
Interfaces: Enables polymorphism.
6. Learn Go's Tools
go run
: Run Go programs.go build
: Compile programs.go fmt
: Format code.go test
: Run tests.go get
: Install dependencies.
Suggested Learning Path
Basic Syntax: Learn variables, loops, functions, and control flow.
Core Features: Understand goroutines, channels, and packages.
Practice Projects:
Build a REST API.
Implement a CLI tool.
Create a simple game or calculator.
Explore Advanced Topics:
Error handling best practices.
Unit testing with
testing
package.Working with databases.
Online Resources
A Tour of Go (Interactive tutorial)
Gophercises (Practical exercises)
The best source for more information about Go is the official website, https://golang.org, which provides access to the documentation, including the Go Programming Language Specification, standard packages, and the like. There are also tutorials on how to write Go and how to write it well, and a wide variety of online text and vide o resources that will be valuable complements to this book. The Go Blog at blog.golang.org publishes some of the best writing on Go, with articles on the state of the language, plans for the future , reports on conferences, and in-depth explanation s of a wide variety of Go-related topics.
Subscribe to my newsletter
Read articles from kietHT directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

kietHT
kietHT
I am a developer who is highly interested in TypeScript. My tech stack has been full-stack TS such as Angular, React with TypeScript and NodeJS.