React Context vs Redux: Are They the Same?
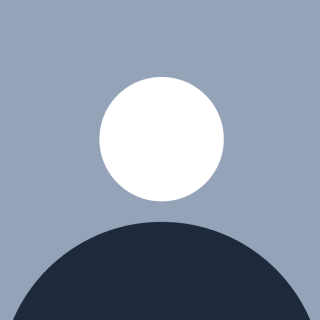
Table of contents
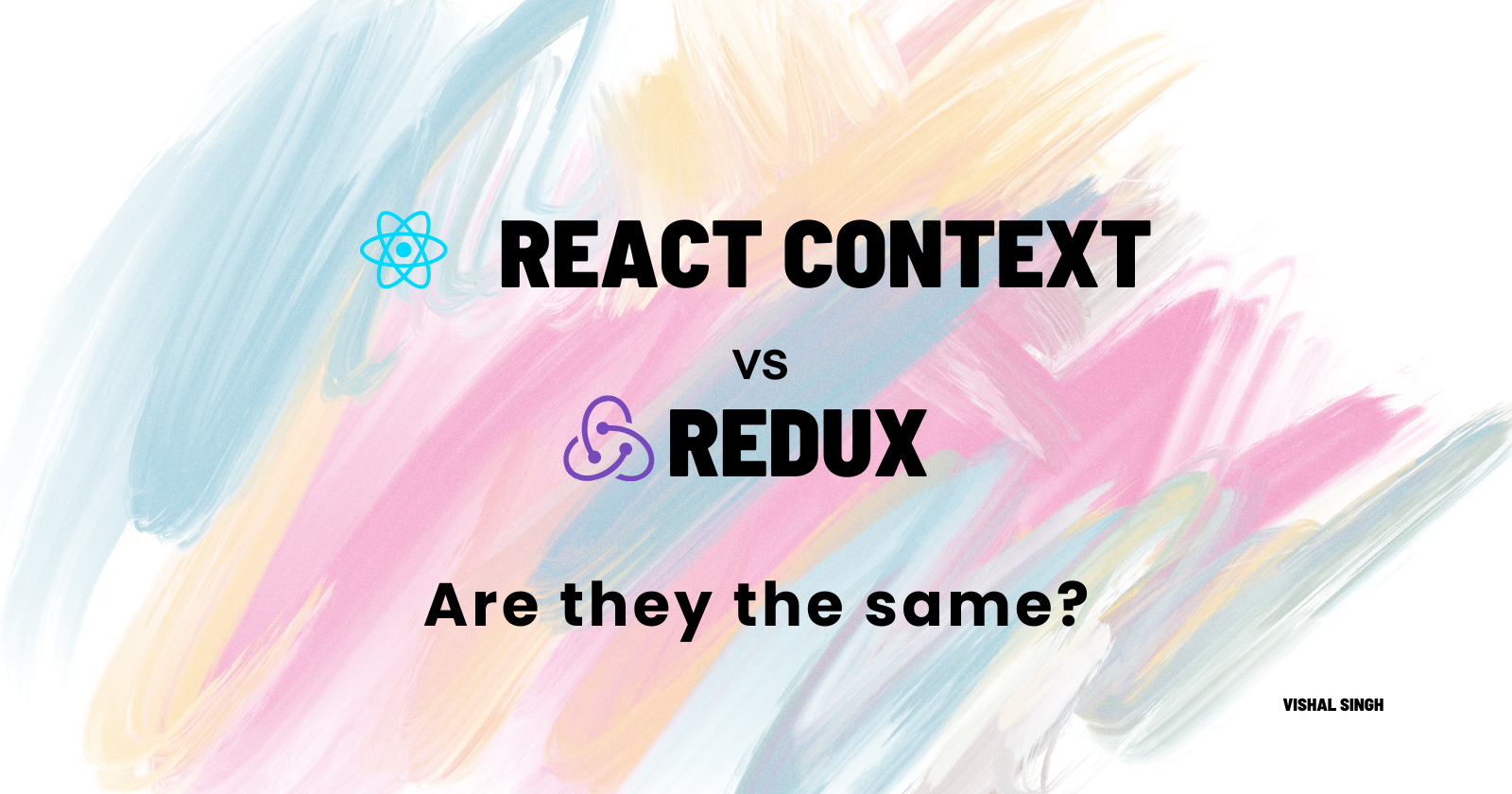
Building modern web applications, and managing and sharing state across components is a common challenge. React Context and Redux are two widely used tools for state management. At first glance, they might seem similar, but their purposes, use cases, and functionality are quite different. In this article, we’ll dive deep into both, explore their strengths and limitations, and discuss when to use each.
What is React Context?
React Context is a built-in feature of React that allows developers to share data across a component tree without passing the props manually at every level. It’s part of React's core library, introduced in version 16.3.
How React Context Works
React Context uses two main components:
Provider: Supplies the data to the components below it in the component tree.
Consumer: Retrieves and uses the provided data.
Here’s a simple example:
import React, { createContext, useContext } from 'react';
// Create a Context
const ThemeContext = createContext();
const App = () => {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
};
const Toolbar = () => {
const theme = useContext(ThemeContext);
return <div>The current theme is {theme}</div>;
};
export default App;
Advantages of React Context
Built-in: No need for additional libraries.
Simple API: Works well for straightforward data sharing like themes, language, or user preferences.
Scoped State: The state is scoped to a specific tree, making it easy to manage contextually.
Limitations of React Context
Performance Issues: Updating context triggers a re-render of the all-consuming components, even if they don’t depend on the updated value.
No Middleware: Lacks built-in support for handling side effects like API calls or logging.
Manual State Management: Developers need to use hooks like
useState
oruseReducer
to handle state, which can get complex as the app grows.
What is Redux?
Redux is a library for state management that helps predictably manage the global state. It provides a structured approach with a central store, actions, and reducers. Redux is often used in large-scale applications with complex state interactions.
How Redux Works
Redux relies on three core principles:
Single Source of Truth: The application's state is stored in a single object called the store.
State is Read-Only: The only way to change the state is by dispatching an action.
Pure Functions for Updates: Reducers specify how the state is updated based on the dispatched action.
Here’s an example of Redux in action:
import { createStore } from 'redux';
// Initial State
const initialState = {
count: 0,
};
// Reducer
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
};
// Create Store
const store = createStore(counterReducer);
// Dispatch Actions
store.dispatch({ type: 'INCREMENT' });
console.log(store.getState()); // { count: 1 }
Advantages of Redux
Centralized State: All states are managed in one place, making it easier to debug and maintain.
Predictable State Updates: Reducers ensure state updates are consistent and predictable.
Middleware Support: Tools like
redux-thunk
andredux-saga
handle side effects effectively.Rich Developer Tools: Redux DevTools allows developers to inspect state changes, time travel, and debug efficiently.
Limitations of Redux
Boilerplate Code: Setting up Redux requires writing actions, reducers, and sometimes middleware, which can feel verbose for smaller applications.
Learning Curve: Understanding concepts like middleware, selectors, and reducers can be challenging for beginners.
Overhead: Using Redux for simple state management might be overkill.
For more simplicity you can use Reduxjs/toolkit
Are They the Same?
React Context and Redux are not the same, although they may appear similar in certain use cases. Both can be used for state management and passing data throughout an application, but they differ in philosophy, use cases, and functionality. Here's a detailed explanation:
1. Purpose and Philosophy
React Context:
Primarily designed for dependency injection and sharing data across a component tree without passing props manually at every level.
Suitable for simpler state-sharing needs like themes, language, or small-scale state management.
It's not inherently a state management library but a tool for avoiding prop drilling.
Redux:
A state management library with a strict unidirectional data flow.
Designed to handle complex, large-scale application states with reliable and predictable state updates.
Provides features like time-travel debugging, middleware, and fine-grained control over state management.
2. State Management and Updates
React Context:
Uses a
Provider
to pass a value to its descendant components.Updates trigger a re-render of all-consuming components when the value changes, which can lead to performance bottlenecks in large applications.
The built-in mechanism for handling the state, so you manage the state yourself (e.g., using
useState
oruseReducer
).
Redux:
Centralized global store where the state is managed in a single object or a collection of reducers.
Updates are handled through dispatching actions and reducers that define how the state changes.
Offers optimized state updates by connecting only the components that need access to specific slices of the state (
mapStateToProps
oruseSelector
).
3. Middleware and Side Effects
React Context:
- Built-in support for middleware or handling asynchronous operations like API calls. Developers need to rely on
useEffect
or custom patterns.
- Built-in support for middleware or handling asynchronous operations like API calls. Developers need to rely on
Redux:
Has robust middleware support (
redux-thunk
,redux-saga
, etc.) for handling side effects such as API calls, logging, or complex asynchronous workflows.Middleware simplifies debugging and decouples side-effect logic from UI components.
4. Debugging and Developer Tools
React Context:
Limited debugging tools. You rely on React Developer Tools and custom logs.
The built-in mechanism for inspecting changes or tracking the flow of data.
Redux:
Redux comes with powerful debugging tools like the Redux DevTools, which allows you to inspect state changes, time travel, and replay actions.
Better suited for debugging complex state updates.
5. Performance
React Context:
Context updates can cause unnecessary re-renders of components that consume the context, even if they don't use the updated value.
Requires optimization techniques like memoization (
React.memo
,useMemo
, or splitting contexts).
Redux:
Optimized updates through
connect
(HOC) oruseSelector
, which allows components to subscribe to specific slices of state, reducing unnecessary re-renders.Built-in mechanisms to manage state more efficiently.
6. Use Cases
React Context:
It is ideal for situations where prop drilling is the main issue.
Examples:
Theme toggles
Localization (language settings)
Authenticated user info (small scale)
Redux:
These are suitable ideals for large-scale applications that involve complex interactions of state.
Examples:
E-commerce applications (cart, user info, product catalog)
Dashboards with data fetched from multiple sources
Applications needing advanced state debugging
Can React Context Replace Redux?
React Context combined with useReducer
might seem like a simpler alternative to Redux for small-scale applications. However:
It lacks middleware support, debugging tools, and performance optimizations.
Redux is designed for structured state management and scales better for complex apps.
Conclusion
React Context and Redux serve different purposes and are not interchangeable in most scenarios. React Context is great for solving the problem of prop drilling, while Redux is a full-fledged state management library tailored for larger applications. Choose the one that fits your application's complexity and requirements. Happy coding! 💻
Subscribe to my newsletter
Read articles from Vishal Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by