Top 40 React-based interview questions
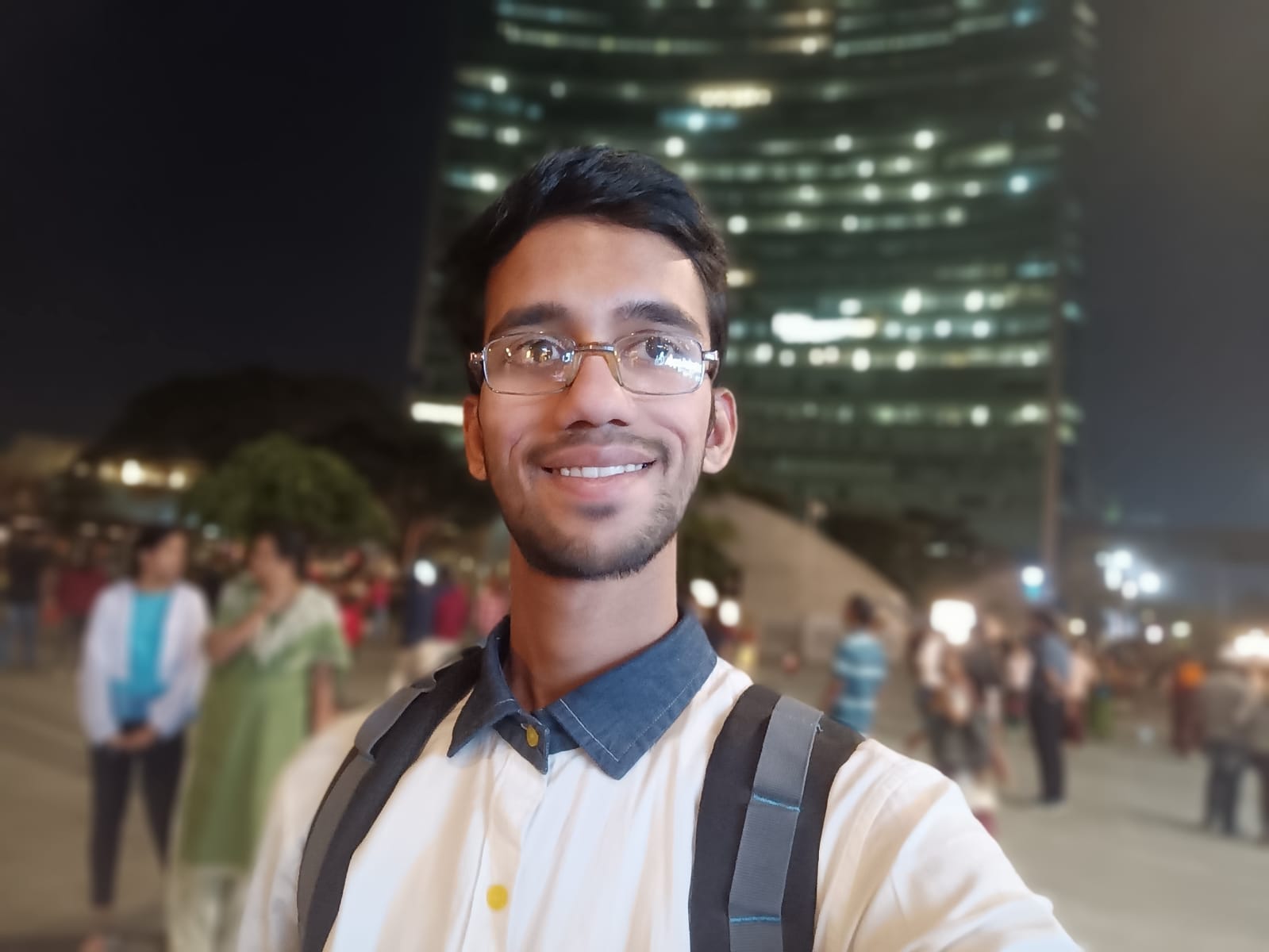
1. What is React?
React is a JavaScript library for building user interfaces, maintained by Facebook. It allows developers to create reusable UI components and manage the state of applications efficiently.
2. What is useMemo?
useMemo
is a React hook that memoizes the result of a computation to optimize performance. It recalculates the value only when the dependencies change.
3. What are the features of React?
Virtual DOM for efficient UI updates.
Component-based architecture.
Unidirectional data flow.
Declarative programming.
Support for hooks and JSX.
4. What is JSX?
JSX stands for JavaScript XML, a syntax that allows you to write HTML-like code within JavaScript, making it easier to create React elements.
5. What is DOM?
The Document Object Model (DOM) is a programming interface for web documents, representing the structure of a webpage as a tree of objects.
6. What is Virtual DOM?
Virtual DOM is a lightweight JavaScript representation of the actual DOM. React uses it to efficiently update the UI by comparing it with the previous state (diffing).
7. What is the component lifecycle of React class components?
Mounting: constructor, getDerivedStateFromProps, render, componentDidMount.
Updating: shouldComponentUpdate, getSnapshotBeforeUpdate, componentDidUpdate.
Unmounting: componentWillUnmount.
8. What are fragments in React?
Fragments let you group multiple elements without adding extra nodes to the DOM. <React.Fragment>
or shorthand <>
is used.
9. What are props in React?
Props are inputs passed to a component to customize its behavior. They are immutable and read-only.
10. What are synthetic events in React?
Synthetic events are React's cross-browser wrapper around native browser events, providing a consistent API for handling events.
11. Difference between package.json and package-lock.json?
package.json
: Lists project dependencies and scripts.package-lock.json
: Ensures consistent dependency resolution and records exact versions.
12. Client-side vs Server-side rendering?
Client-side: Rendering occurs in the browser. Faster interaction after loading but slower initial load.
Server-side: Rendering occurs on the server. Faster initial load, better SEO, but higher server load.
13. What is state in ReactJS?
State is an object in React that holds data about a component and determines how it renders and behaves.
14. What are props?
Props (short for properties) are data passed from a parent to a child component.
15. State vs Props in React?
Aspect | State | Props |
Mutability | Mutable | Immutable |
Ownership | Owned by component | Passed from parent |
Usage | Component's data | Customize children |
16. What is props drilling?
Props drilling is the process of passing props through multiple levels of components, even when they are not needed by intermediary components.
17. Disadvantages of props drilling and how to avoid it?
Disadvantages:
Makes the code harder to maintain.
Leads to unnecessary re-renders.
Solutions:
- Use the Context API or state management libraries like Redux.
18. What are Pure Components in React?
Pure components in React are components that only re-render when their state or props change, improving performance.
19. What are refs in React?
Refs provide a way to directly access and manipulate DOM elements or React elements.
20. What is meant by forward refs?
Forward refs allow parent components to pass refs to child components using React.forwardRef
.
21. What are Error Boundaries?
Error boundaries are React components that catch JavaScript errors in their child component tree and display fallback UI.
22. What are Higher Order Components (HOCs)?
HOCs are functions that take a component as an argument and return a new component, adding extra functionality.
23. Controlled vs Uncontrolled components?
Controlled: Component state is managed by React.
Uncontrolled: State is managed by the DOM.
24. What is useCallback?
useCallback
memoizes a function, preventing unnecessary re-creation unless its dependencies change.
25. Difference between useMemo and useCallback?
useMemo
: Memoizes a computed value.useCallback
: Memoizes a function.
26. What are keys in React?
Keys are unique identifiers used to track changes to items in a list and optimize re-rendering.
27. What is lazy loading in React?
Lazy loading loads components or resources only when needed, improving performance.
28. What is suspense in React?
Suspense enables asynchronous rendering by delaying the rendering of a component until a condition is met (e.g., data fetching).
29. What are custom hooks?
Custom hooks are reusable functions that encapsulate common logic, making code more modular.
30. What is the useReducer hook?
useReducer
is an alternative to useState
, managing state with a reducer function.
31. What are portals in React?
Portals render children into a DOM node outside the parent component hierarchy.
32. What is context in React?
Context provides a way to share data between components without explicitly passing props through each level.
33. Example of Context API usage?
jsxCopy codeconst UserContext = React.createContext();
function App() {
return (
<UserContext.Provider value={{ name: 'John' }}>
<Child />
</UserContext.Provider>
);
}
function Child() {
const user = React.useContext(UserContext);
return <div>Hello, {user.name}!</div>;
}
34. Purpose of a callback function in setState()?
It ensures the state update is complete before executing additional logic.
35. Custom hook for counter increment/decrement?
jsxCopy codefunction useCounter(initialValue = 0) {
const [count, setCount] = React.useState(initialValue);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return { count, increment, decrement };
}
36. Lifecycle hooks replaced with useEffect?
componentDidMount
componentDidUpdate
componentWillUnmount
37. What is Strict Mode in React?
Strict Mode highlights potential issues in code by enabling checks and warnings during development.
38. Ways to pass data from child to parent?
Callback functions.
Using useRef in conjunction with parent components.
Context API.
39. How to send data from child to parent using callbacks?
jsxCopy codefunction Parent() {
const handleData = (data) => console.log(data);
return <Child onSend={handleData} />;
}
function Child({ onSend }) {
return <button onClick={() => onSend("Hello, Parent!")}>Send</button>;
}
40. How to send data from child to parent using useRef?
jsxCopy codefunction Parent() {
const childRef = React.useRef();
const handleClick = () => alert(childRef.current.value);
return (
<>
<Child ref={childRef} />
<button onClick={handleClick}>Get Value</button>
</>
);
}
const Child = React.forwardRef((props, ref) => (
<input ref={ref} type="text" placeholder="Enter text" />
));
Subscribe to my newsletter
Read articles from ANIRUDH HEGDE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
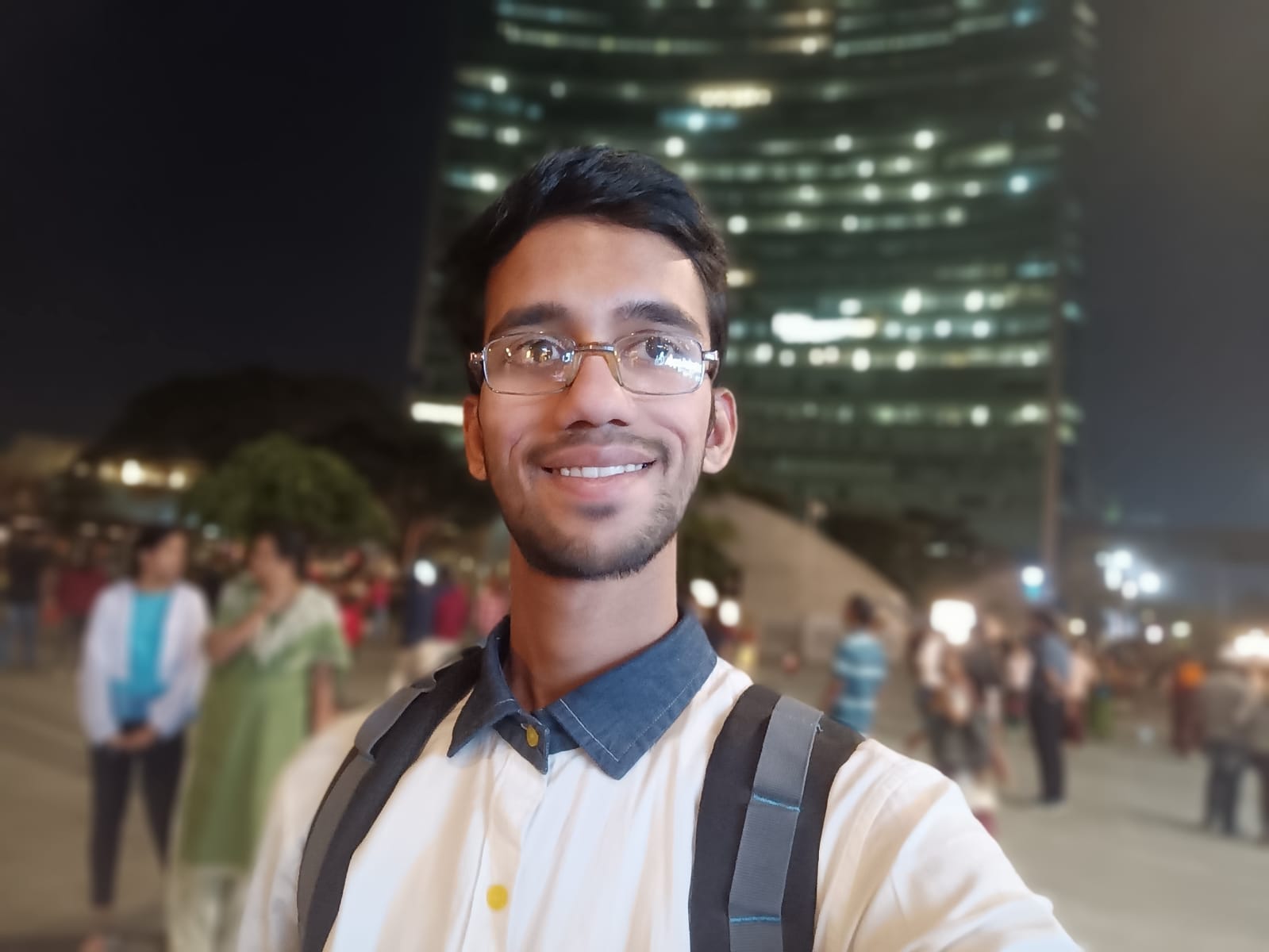
ANIRUDH HEGDE
ANIRUDH HEGDE
Pythonista