Building a Dynamic Book Catalog with AJAX and XML
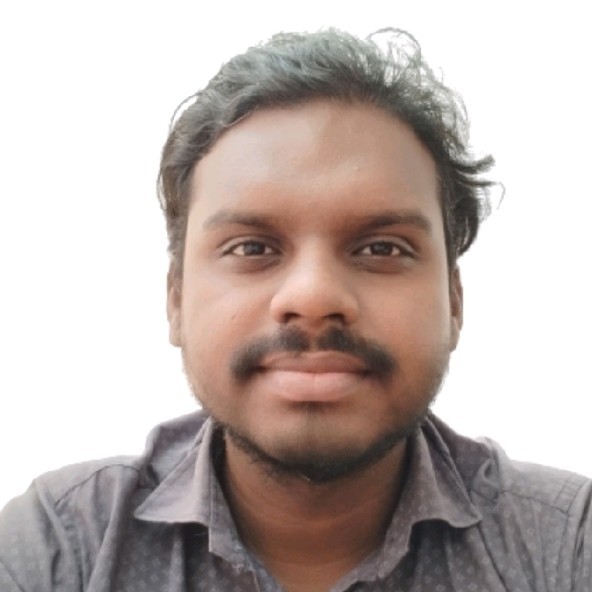
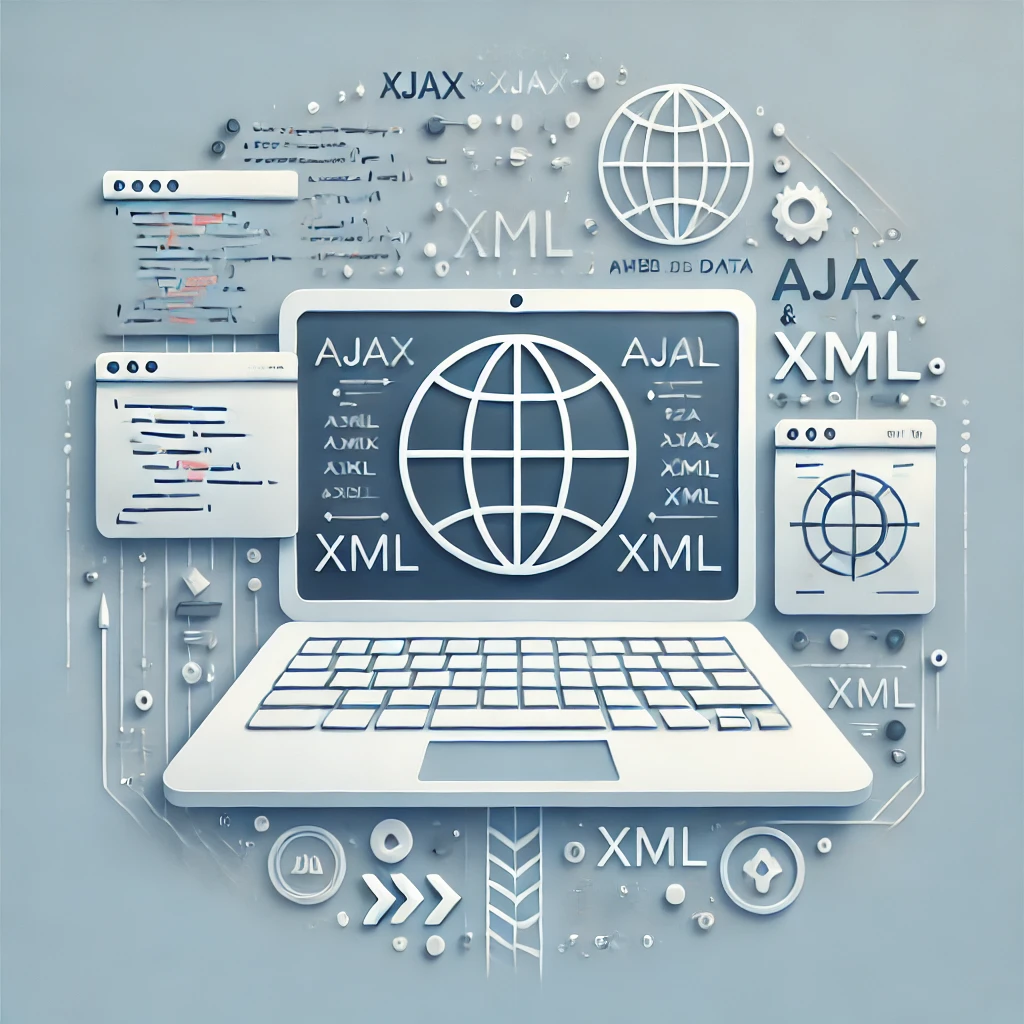
In today’s fast-paced web development world, creating smooth and responsive user experiences is key. One way to achieve this is through AJAX (Asynchronous JavaScript and XML), a powerful technique that allows web pages to communicate with servers and fetch data without reloading the page. In this blog, we'll walk through a simple project—a Dynamic Book Catalog—to demonstrate how AJAX works with XML to build a seamless user experience.
What is AJAX?
AJAX stands for Asynchronous JavaScript and XML. It’s not a programming language but a set of techniques that enable web applications to send and retrieve data from a server asynchronously. This means the user can interact with the page while the data is being fetched or updated in the background.
Key Features of AJAX:
Asynchronous Data Fetching: No page reload required.
Interactivity: Webpages feel faster and more responsive.
Data Formats: Although the "X" in AJAX stands for XML, it works equally well with JSON, plain text, or HTML.
Project Overview: Simple Book Catalog
In this project, we'll use AJAX to fetch book details stored in an XML file and dynamically display them in an HTML table. By the end, clicking a button will load the catalog without reloading the page.
Building a Dynamic Book Catalog with AJAX and XML
In today’s fast-paced web development world, creating smooth and responsive user experiences is key. One way to achieve this is through AJAX (Asynchronous JavaScript and XML), a powerful technique that allows web pages to communicate with servers and fetch data without reloading the page. In this blog, we'll walk through a simple project—a Dynamic Book Catalog—to demonstrate how AJAX works with XML to build a seamless user experience.
What is AJAX?
AJAX stands for Asynchronous JavaScript and XML. It’s not a programming language but a set of techniques that enable web applications to send and retrieve data from a server asynchronously. This means the user can interact with the page while the data is being fetched or updated in the background.
Key Features of AJAX:
Asynchronous Data Fetching: No page reload required.
Interactivity: Webpages feel faster and more responsive.
Data Formats: Although the "X" in AJAX stands for XML, it works equally well with JSON, plain text, or HTML.
Project Overview: Simple Book Catalog
In this project, we'll use AJAX to fetch book details stored in an XML file and dynamically display them in an HTML table. By the end, clicking a button will load the catalog without reloading the page.
Step 1: The XML Data File
The book data is stored in an books.xml
file. XML organizes information in a tree-like structure, making it easy to categorize and retrieve data. Here's what our XML file looks like:
<?xml version="1.0" encoding="UTF-8"?>
<catalog>
<book>
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<year>1925</year>
<genre>Fiction</genre>
</book>
<book>
<title>1984</title>
<author>George Orwell</author>
<year>1949</year>
<genre>Dystopian</genre>
</book>
<book>
<title>To Kill a Mockingbird</title>
<author>Harper Lee</author>
<year>1960</year>
<genre>Fiction</genre>
</book>
</catalog>
Each <book>
contains details like <title>
, <author>
, <year>
, and <genre>
. The <catalog>
serves as the root element that holds all the <book>
elements.
Step 2: The HTML Structure
Our webpage will have a button to trigger the AJAX request and an empty table to display the fetched data.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Book Catalog</title>
</head>
<body>
<h2>Book Catalog</h2>
<button onclick="loadBooks()">Load Books</button>
<br><br>
<table id="bookTable" border="1">
<tr>
<th>Title</th>
<th>Author</th>
<th>Year</th>
<th>Genre</th>
</tr>
</table>
</body>
</html>
Here’s what happens:
Button: When clicked, it calls the
loadBooks()
function to fetch the XML data.Table: Initially, only the headers are displayed. The book details will be added dynamically.
Step 3: The JavaScript Magic (AJAX in Action)
Now comes the fun part—writing the JavaScript to make the page dynamic using AJAX.
<script>
function loadBooks() {
// Step 1: Create a new XMLHttpRequest object
const xhttp = new XMLHttpRequest();
// Step 2: Define what happens when the file is loaded successfully
xhttp.onload = function() {
if (this.status == 200) {
displayBooks(this);
}
};
// Step 3: Open the request to fetch 'books.xml'
xhttp.open("GET", "books.xml", true);
// Step 4: Send the request
xhttp.send();
}
function displayBooks(xml) {
// Step 5: Parse the XML response
const xmlDoc = xml.responseXML;
// Step 6: Get all 'book' elements from the XML file
const books = xmlDoc.getElementsByTagName("book");
// Step 7: Build table rows with book data
let tableContent = "";
for (let i = 0; i < books.length; i++) {
const title = books[i].getElementsByTagName("title")[0].childNodes[0].nodeValue;
const author = books[i].getElementsByTagName("author")[0].childNodes[0].nodeValue;
const year = books[i].getElementsByTagName("year")[0].childNodes[0].nodeValue;
const genre = books[i].getElementsByTagName("genre")[0].childNodes[0].nodeValue;
tableContent += "<tr>" +
"<td>" + title + "</td>" +
"<td>" + author + "</td>" +
"<td>" + year + "</td>" +
"<td>" + genre + "</td>" +
"</tr>";
}
// Step 8: Update the HTML table with the new rows
document.getElementById("bookTable").innerHTML += tableContent;
}
</script>
Step 4: Understanding AJAX in the Code
Let’s break down the steps where AJAX comes into play:
1. Create XMLHttpRequest
The XMLHttpRequest
object is the heart of AJAX. It allows us to send requests to the server and receive responses.
const xhttp = new XMLHttpRequest();
2. Open the Request
We prepare the request to fetch the books.xml
file using the GET
method.
xhttp.open("GET", "books.xml", true);
GET: Retrieves data from the server.
"books.xml": The file to fetch.
true: Asynchronous mode.
3. Send the Request
This sends the prepared request to the server.
xhttp.send();
4. Handle the Response
Once the XML file is loaded, the onload
function processes the response.
xhttp.onload = function() {
if (this.status == 200) {
displayBooks(this);
}
};
this.status == 200
: Ensures the request was successful.displayBooks(this)
: Calls a function to extract and display the book data.
Step 5: Parsing and Displaying XML
The displayBooks()
function handles the parsed XML and updates the HTML table.
const books = xmlDoc.getElementsByTagName("book");
This extracts all <book>
elements from the XML file.
For each <book>
, we retrieve the values of its child elements (<title>
, <author>
, etc.):
const title = books[i].getElementsByTagName("title")[0].childNodes[0].nodeValue;
Finally, the data is added as rows to the table:
tableContent += "<tr>" +
"<td>" + title + "</td>" +
"<td>" + author + "</td>" +
"<td>" + year + "</td>" +
"<td>" + genre + "</td>" +
"</tr>";
Why AJAX Matters
AJAX enhances user experience by:
Avoiding full page reloads, keeping interactions smooth.
Fetching and updating data dynamically, reducing server load and response times.
In this project:
The books.xml file is loaded asynchronously.
Data is displayed in real-time in the HTML table.
Users enjoy a seamless experience without interruptions.
Summary
This project demonstrates how AJAX can be combined with XML to create dynamic and interactive web applications. By fetching and displaying book data asynchronously, we built a simple but effective example of modern web development practices.
Whether you’re working with XML or JSON, the principles of AJAX remain the same, and its power to create smooth, responsive web pages is unparalleled. Happy coding!
Subscribe to my newsletter
Read articles from Ahnaf Tahmid Zaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
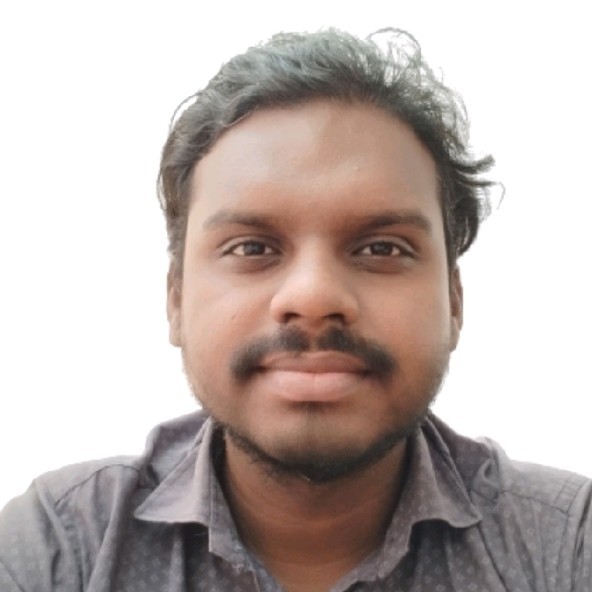
Ahnaf Tahmid Zaman
Ahnaf Tahmid Zaman
● Computer Science Undergraduate with a fervent interest in technology and innovation. ● Aspiring to become a DevOps Engineer. Currently learning key DevOps concepts including: Containerization, Orchestration, IaC, CI/CD, Monitoring and Logging, VCS, Configuration Management, Infrastructure Orchestration, Cloud Computing, Security Automation and Compliance, Identity and Access Management (IAM). ● Experienced IT Supervisor adept at guiding technology strategy and troubleshooting network performance. ● Skilled Data Collector ensuring data integrity through meticulous survey documentation. ● Strong leadership and problem-solving abilities, demonstrated through successful project supervision. ● Volunteer experience promoting cultural events and contributing to community support initiatives.