What New React 19 Has Brought to The Table - Get Clear Understanding

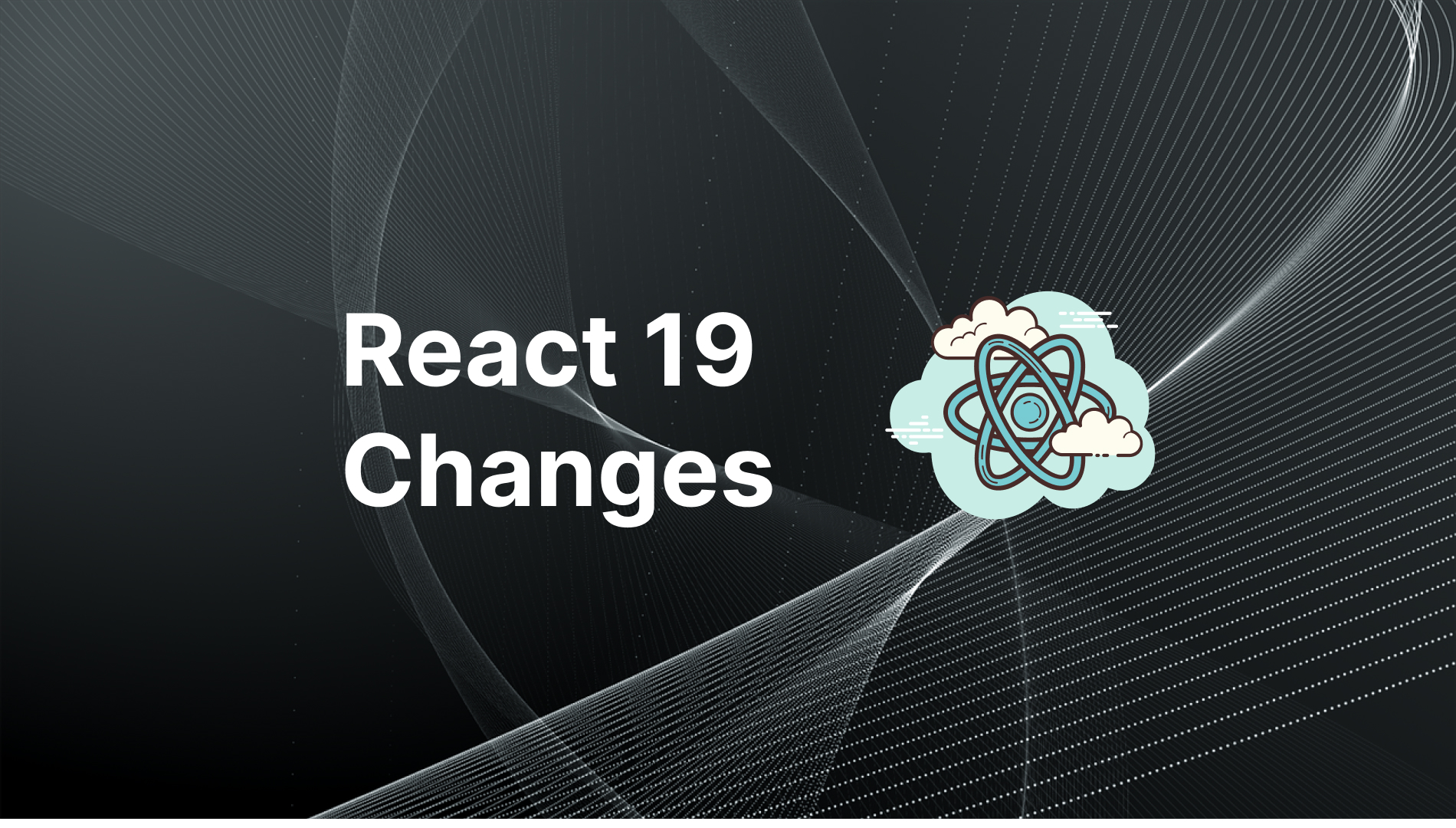
React 19 came out on April 25, 2024. The JavaScript world changes so quickly that it can sometimes feel overwhelming to keep up. But when these changes are meant to make your life as a React developer easier, it’s worth taking a look, right? Since React is such an important part of this ecosystem, staying updated is a must.
The best part about React 19 is that it focuses on making things simpler. The updates are designed to make learning React easier and let you spend more time creating instead of dealing with tricky setups. Some of the new features are really game-changers and could make a big difference in how you work, so you definitely don’t want to miss them.
I always try to explain things in a way that’s easy to understand, without throwing in complicated words. This article is no exception. My goal is to make sure you get everything clearly, so let’s explore the awesome updates in React 19 together!
Remember, React 19 is not quite stable yet. Currently it’s called React Canary. So, keep in mind that it’s not actually recommended for production.
React Compiler
To optimize our React applications, we use some inbuilt methods like useMemo
, useCallback
or memo
. This tells React not to compile the code again if the inputs don’t change. But if you forget to apply memoization, it results in wasting React resources and computational power. To deal with this, React 19 introduced React Compiler. React’s new compiler is the eyeball of the 19th version’s new release. The new compiler optimizes your code behind the scenes, so you can drop these hooks and focus on writing beautiful, clean React components.
In short, you don’t need to wrap your functions with useMemo
or useCallback
for optimized performance, and you also don’t need to wrap your component with memo
to prevent re-rendering your components.
Actions (useTransition
hook)
Let’s talk some gibberish 😀!! Have you noticed how the useTransition
hook was barely mentioned before React 19 came out? Or is it just me? Well, at least that’s what I noticed, especially among Junior Developers. Anyway, let me give you an idea of how it worked in the previous version and then we’ll see why it’s such an important feature now.
useTransition
returns an array with two elements, startTransition
function and isPending
boolean. You can wrap your state updates inside the startTransition
function to mark them as transitions (less priority code). Which means the part wrapped with startTransition
starts to work after the other continuous tasks get completed.
In React 18, the startTransition
function did not support async functions directly. This was a limitation because startTransition
was designed to mark updates as low-priority but couldn't handle asynchronous logic natively.
In React 19, this limitation has been addressed. Now, startTransition
supports async functions, meaning you can perform asynchronous tasks inside it (e.g., data fetching) while keeping those updates marked as transitions. This enhancement allows for more flexible and intuitive usage of startTransition
, making it feel like a "new" feature even though it's technically an improvement to an existing one.
For example, you could handle the pending and error state in useState
:
// Before Actions
function UpdateName({}) {
const [name, setName] = useState("");
const [error, setError] = useState(null);
const [isPending, setIsPending] = useState(false);
const handleSubmit = async () => {
setIsPending(true);
const error = await updateName(name);
setIsPending(false);
if (error) {
setError(error);
return;
}
redirect("/path");
};
return (
<div>
<input value={name} onChange={(event) => setName(event.target.value)} />
<button onClick={handleSubmit} disabled={isPending}>
Update
</button>
{error && <p>{error}</p>}
</div>
);
}
React 19 supports using async functions in transitions to handle pending states, errors, forms, and optimistic updates automatically. For example, you can use useTransition
to handle the pending state for you:
// Using pending state from Actions
function UpdateName({}) {
const [name, setName] = useState("");
const [error, setError] = useState(null);
const [isPending, startTransition] = useTransition();
const handleSubmit = () => {
startTransition(async () => {
const error = await updateName(name);
if (error) {
setError(error);
return;
}
redirect("/path");
})
};
return (
<div>
<input value={name} onChange={(event) => setName(event.target.value)} />
<button onClick={handleSubmit} disabled={isPending}>
Update
</button>
{error && <p>{error}</p>}
</div>
);
}
The async transition will immediately set the isPending
state to true, make the async request(s), and switch isPending
to false after any transitions. This allows you to keep the current UI responsive and interactive while the data is changing.
<form>
Actions
The React team added support for passing functions as the action
.
export default function App() {
const [name, setName] = useState(
() => JSON.parse(localStorage.getItem("name")) || "Anonymous user"
)
async function formAction(formData){
try {
const newName = await updateNameInDB(formData.get("name"))
setName(newName)
}
}
return (
<>
<p className="username">
Current user: <span>{name}</span>
</p>
<form action={formAction}>
<input
type="text"
name="name"
required
/>
<button type="submit">Update</button>
</form>
</>
)
}
The formAction
function (you can name anything) gets you the form data within its parameter. Each field denoted by name attribute and so you got to be careful in naming inputs. the formData
parameter is actually the native FormData Web API object. You can find it out on mdn web docs. Another good thing is you don’t need to apply event.preventDefault()
as it is handled by React.
When a form
Action succeeds, React automatically resets the form. But if you want to reset the <form>
manually, you can call the new requestFormReset
React DOM API.
New Hook: useActionState
React.useActionState
was previously called ReactDOM.useFormState
in the Canary releases, but it has been renamed and deprecated useFormState
.useActionState
tracks component state, pending status and provides a wrapped action function for use in form or any other place we might want to perform a mutation.
Here is an example to break it down more descriptively:
import { useActionState } from "react"
import { updateNameInDB } from "../api/user"
export default function App() {
const [user, formAction, isPending] = useActionState(insertName, {
name: "John Doe",
error: null
})
async function insertName(prevState, formData){
try {
const response = await updateNameInDB(formData.get("name"))
return {name: response.name}
}catch(error){
return {...prevState, error: error.error}
}
}
return (
<>
{user?.name && (
<p style={{ color: "green" }}>
Current user: <span>{user.name}</span>
</p>
)}
{user?.error && <p style={{ color: "red" }}>{user.error}</p>}
<form action={formAction}>
<input
type="text"
name="name"
required
/>
<button type="submit">Update</button>
</form>
</>
)
}
How this hook works is described with reference to the example:
The first argument of the
useActionState
hook is the "Action" function, which is defined here asinsertName
.The second argument is the initial state, which is accessible through the first element of the result array. In this example, the initial state includes
name
anderror
, and the state is represented asuser
in the component.The
insertName
function returns the updated state. If the operation is successful, it updates thename
property. If an error occurs, it updates theerror
property while preserving the rest of the previous state.The result of the
useActionState
hook is an array with three elements:The current state (
user
): Reflects the latest state of the data.A dispatchable function (
formAction
): Triggers the action when called, as seen in theform
element'saction
attribute.A pending state (
isPending
): Tracks whether the action is currently in progress, useful for managing transitions or loading indicators.
New Hook: useOptimistic
When it’s performing a data mutation and to show the final state right after the user instructs (could be a tap on a button) or you could say optimistically while the async request is underway, you need to use this hook. Here is a demonstration how you can do this:
function ChangeName({currentName, onUpdateName}) {
const [optimisticName, setOptimisticName] = useOptimistic(currentName);
const submitAction = async (formData) => {
const newName = formData.get("name");
setOptimisticName(newName);
const updatedName = await updateName(newName);
onUpdateName(updatedName);
};
return (
<form action={submitAction}>
<p>Your name is: {optimisticName}</p>
<p>
<label>Change Name:</label>
<input
type="text"
name="name"
disabled={currentName !== optimisticName}
/>
</p>
</form>
);
}
The useOptimistic
hook will immediately render the optimisticName
while the updateName
request is in progress. When the update finishes React will insert the updated value in currentName
or if the update gets errors, React will automatically switch back to the currentName
value.
New Hook: useFormStatus
useFormStatus
hook helps you keep track of your form submissions. Wait a minute 🤔! is it another hook to track the async transition? well the answer is ‘yes’ and ‘no’ both. As you have already learnt the useActionState
hook, you could say this is another hook for tracking the async transition. But The useFormStatus
doesn’t cause any action to happen, rather it provides status information of the last form submission.
import { useFormStatus } from "react-dom";
import action from './actions';
function Submit() {
const status = useFormStatus();
return <button disabled={status.pending}>Submit</button>
}
export default function App() {
return (
<form action={action}>
<Submit />
</form>
);
}
Well, I would say the most important thing to notice here is useFormStatus
hook actually comes from react-dom
, not react
.
useFormStatus
reads the status of the parent <form>
as if the form was a Context provider. To get status information, the Submit
component must be rendered within a <form>
. The Hook returns information like the pending
property which tells you if the form is actively submitting.
In the above example, Submit
uses this information to disable <button>
presses while the form is submitting.
New API: use
you can read a promise with use
, and React will Suspend the Component until the promise resolves:
import {use} from 'react';
function Comments({commentsPromise}) {
// `use` will suspend until the promise resolves.
const comments = use(commentsPromise);
return comments.map(comment => <p key={comment.id}>{comment}</p>);
}
function Page({commentsPromise}) {
// When `use` suspends in Comments,
// this Suspense boundary will be shown.
return (
<Suspense fallback={<div>Loading...</div>}>
<Comments commentsPromise={commentsPromise} />
</Suspense>
)
}
The example above demonstrates the use case of the use
API. ‘Comments’ is a component that consumes a promise called ‘commentsPromise’. The promise is consumed by the new use
API which makes it suspend the component. By the way, you have to wrap the component with Suspense
fallback.
The use
API has a limitation which you must be aware of. The limitation is every time the use
API containing component re-renders it creates another promise which lead to bad performance. So, basically it doesn’t have caching mechanism. Here is their cautious note from the release blog:
use
API reads data from context as well. For instance, to read context values, we simply pass the context to use()
, and the function traverses the component tree to find the closest context provider.
Unlike the useContext()
Hook to read context, the use()
function can be used within conditionals and loops in our components!
import { use, createContext } from 'react';
const Context = createContext({ data: "Data from context" });
// nested component
function NestedChildComponent({ value }) {
if (value) {
const context = use(Context);
}
}
ref
as a prop (No forwardRef
)
In React 19, you can pass refs just like any other prop which leads to deprecation of forwardRef . This streamlines your component code and makes ref handling a breeze. 🧹
function MyInput({placeholder, ref}) {
return <input placeholder={placeholder} ref={ref} />
}
//...
<MyInput ref={ref} />
The React team has decided to make forwardRef
deprecated in the upcoming versions.
React Server Components
By the way, know that React Server Components (RSCs) are a React feature that supports server-side rendering, but frameworks like Next.js have embraced RSCs and integrated them seamlessly into their workflow. If you are new-comer to the ecosystem, get clarified this thing beforehand jumping into studying its mechanism.
React Server Components are a new capability being introduced in React 19 that allows us to create stateless React components that run on the server.
Since React Server Components are able to run on a web server, they can be used to access the data layer without having to interact with an API!
import db from "./database";
// React Server Component
async function BlogPost({ postId }) {
// Load blog post data from database
const post = await db.posts.get(postId);
// Load comments for the post from database
const comments = await db.comments.getByPostId(postId);
return (
<div>
<h2>{post.title}</h2>
<p>{post.content}</p>
<h3>Comments</h3>
<ul>
{comments.map((comment) => (
<li key={comment.id}>
<Comment {...comment} />
</li>
))}
</ul>
</div>
);
}
With this, we don’t have to expose an API endpoint or use additional client-side fetching logic to load data directly into our components. All the data handling is done on the server.
Keep in mind that Server Components are run on the server and not the browser. As a result, they’re unable to use traditional React component APIs like useState
. To introduce interactivity to a React Server Component setting, we’ll need to leverage Client Components that complement the Server Components for handling interactivity.
When working with React Server Components, “use client” denotes that the component is a Client Component, which means it can manage state, handle user interactions, and use browser-specific APIs. This directive explicitly tells the React framework and bundler to treat this component differently from Server Components, which are stateless and run on the server.
// React Client Component
"use client"
export function Comment({ id, text }) {
const [likes, setLikes] = useState(0);
function handleLike() {
setLikes(likes + 1);
}
return (
<div>
<p>{text}</p>
<button onClick={handleLike}>Like ({likes})</button>
</div>
);
}
On the flip-side, React Server Components are the default so we don’t state “use server” at the top of Server Component files. Instead, “use server” should only be used to mark server-side functions that can be called from Client Components.
"use client"
export default function FormComponent() {
async function handleSubmit(formData) {
"use server"
const name = formData.get("name")
console.log(`Form submitted with name: ${name}`)
return { success: true, message: `Hello, ${name}!` }
}
return (
<form action={handleSubmit}>
<label>
Name:
<input type="text" name="name" required />
</label>
<button type="submit">Submit</button>
</form>
)
}
I think this example clarifies it well.
Conclusion
React 19 is still called React Canary. So, it will not be a good idea to use it for production. But embrace the future with React 19 and make your development experience smoother and more enjoyable.
Subscribe to my newsletter
Read articles from Abeer Abdul Ahad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abeer Abdul Ahad
Abeer Abdul Ahad
I am a Full stack developer. Currently focusing on Next.js and Backend.