Effortless Authentication with Clerk in Your Next.js App 🚀

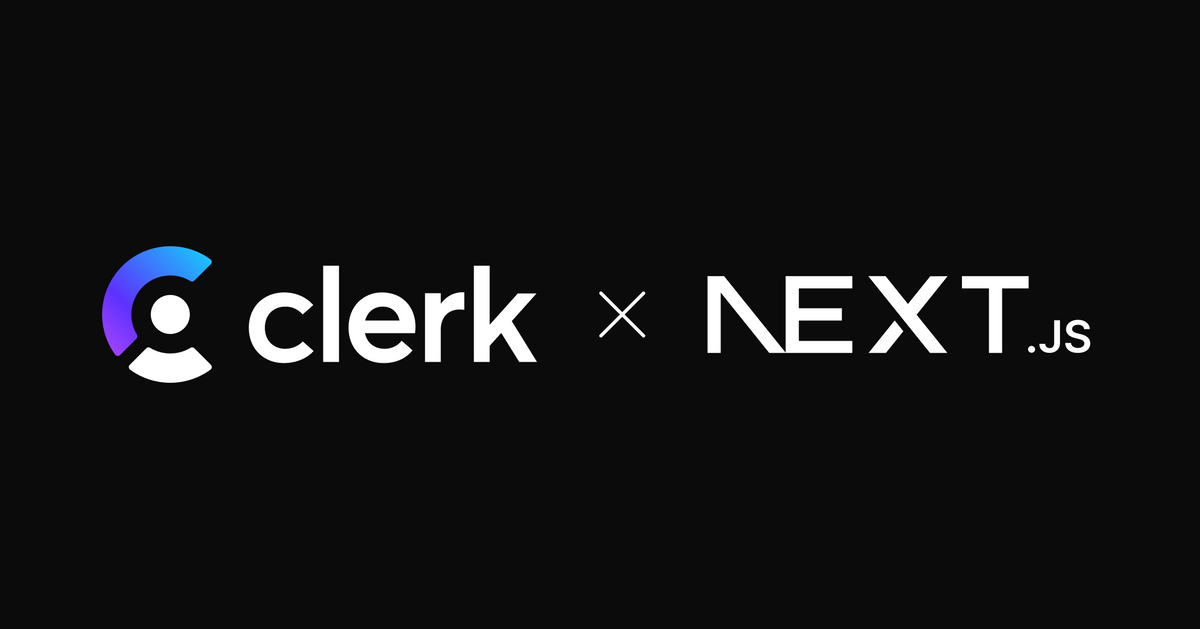
Authentication is one of the most crucial aspects of any modern application. It ensures that only authorized users can access specific parts of your app. Implementing authentication can be complex, but with Clerk, it’s seamless and developer-friendly.
In this blog, we’ll explore how to set up Clerk authentication in a Next.js application step-by-step. By the end, you’ll have a fully functional authentication system that supports login, signup, and user management.
Why Clerk?
Clerk offers:
Prebuilt UI components: No need to design login/signup forms.
Out-of-the-box integrations: Supports social logins, email/password, and more.
Developer-friendly API: Effortlessly manage users and sessions.
Secure by default: Focus on building your app without worrying about vulnerabilities.
Getting Started with Clerk in Next.js
Step 1: Install Clerk
Start by creating a Next.js app or using an existing one.
npx create-next-app@latest my-app
cd my-app
npm install @clerk/nextjs
Step 2: Register Your App on Clerk
Go to the Clerk Dashboard.
Create a new project and note down your
frontendApi
andapiKey
.
Step 3: Configure Clerk
Create a .env.local
file and add the Clerk keys:
NEXT_PUBLIC_CLERK_PUBLISHABLE_KEY=your-publishable-key
CLERK_SECRET_KEY=your-secret-key
Next, wrap your app with the Clerk Provider in layout.ts
:
import { ClerkProvider } from '@clerk/nextjs';
import '../styles/globals.css';
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<ClerkProvider>
<body className={inter.className}>{children}</body>
</ClerkProvider>
);
}
Step 4: Add Authentication Components
Clerk provides prebuilt components like <SignIn />
, <SignUp />
, and <UserButton />
.
Example for adding Sign In:
// /sign-in/page.tsx
import { SignIn } from '@clerk/nextjs';
export default function SignInPage() {
return <SignIn />;
}
Add this page under /sign-in/page.tsx
. Visit /sign-in
to test it.
Step 5: Protect Routes
Secure your app pages using Clerk’s middleware:
// /middleware.ts
import { clerkMiddleware, createRouteMatcher } from '@clerk/nextjs/server'
const isPublicRoute = createRouteMatcher(['/sign-in(.*)', '/sign-up(.*)'])
export default clerkMiddleware(async (auth, request) => {
if (!isPublicRoute(request)) {
await auth.protect()
}
})
export const config = {
matcher: [
// Skip Next.js internals and all static files, unless found in search params
'/((?!_next|[^?]*\\.(?:html?|css|js(?!on)|jpe?g|webp|png|gif|svg|ttf|woff2?|ico|csv|docx?|xlsx?|zip|webmanifest)).*)',
// Always run for API routes
'/(api|trpc)(.*)',
],
}
Alternatively, use Clerk's SignedIn
and SignedOut
components for conditional rendering.
Advanced Features
Clerk doesn’t stop at basic authentication. Explore these advanced features:
Social Login: Enable Google, GitHub, and more with a few clicks.
User Management: Add user profiles, metadata, and custom attributes.
API Authentication: Secure your API endpoints with Clerk's server-side libraries.
Final Thoughts
With Clerk, you can integrate a powerful, secure, and customizable authentication system in minutes. It saves time, offers scalability, and lets you focus on building features that matter.
Ready to get started? Check out Clerk's documentation for more.
Subscribe to my newsletter
Read articles from Mohammed Yunus Shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Yunus Shaikh
Mohammed Yunus Shaikh
I love to explore new technologies, excited to learn and grow everyday.