Understanding Jenkins: A Comprehensive Guide
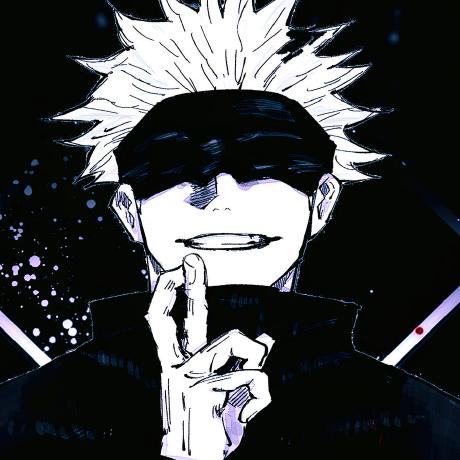
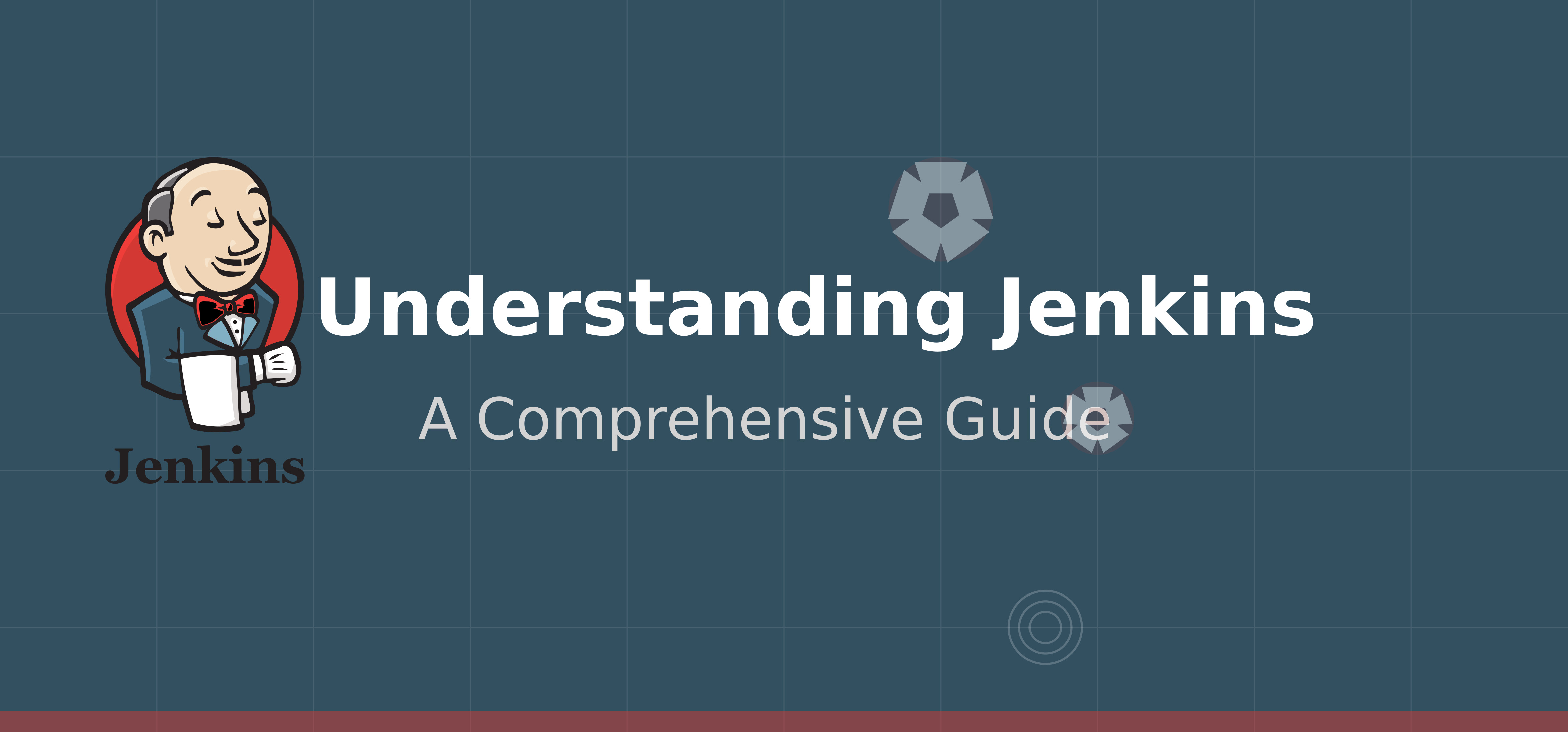
What is Jenkins?
Jenkins is an open-source automation server that has become the de facto standard for continuous integration and continuous delivery (CI/CD) in software development. It acts as a crucial middleman between your development process and deployment, automating the building, testing, and deployment of applications.
Key Features
Extensibility: Supports over 1500 plugins
Easy Configuration: Web interface for setup and management
Distributed Builds: Supports distributed build environments
Platform Agnostic: Runs on Windows, Linux, macOS
Pipeline Support: Code-as-configuration approach
Community Support: Large, active community
Why Use Jenkins?
Automation of Repetitive Tasks
Build automation
Test execution
Deployment processes
Code quality checks
Integration Capabilities
Version control systems (Git, SVN)
Build tools (Maven, Gradle)
Container platforms (Docker, Kubernetes)
Cloud providers (AWS, Azure, GCP)
Cost Efficiency
Open-source
Reduces manual intervention
Minimizes deployment errors
Speeds up development cycle
Installation Guide
Prerequisites
JDK 11 or newer
256MB+ RAM (1GB+ recommended)
10GB+ disk space
Installation Steps for Different Platforms
Ubuntu/Debian
# Add Jenkins repository key
wget -q -O - https://pkg.jenkins.io/debian-stable/jenkins.io.key | sudo apt-key add -
# Add Jenkins repository
sudo sh -c 'echo deb https://pkg.jenkins.io/debian-stable binary/ > /etc/apt/sources.list.d/jenkins.list'
# Update package index and install Jenkins
sudo apt update
sudo apt install jenkins
# Start Jenkins service
sudo systemctl start jenkins
sudo systemctl enable jenkins
CentOS/RHEL
# Add Jenkins repository
sudo wget -O /etc/yum.repos.d/jenkins.repo https://pkg.jenkins.io/redhat-stable/jenkins.repo
sudo rpm --import https://pkg.jenkins.io/redhat-stable/jenkins.io.key
# Install Jenkins
sudo yum install jenkins
# Start Jenkins service
sudo systemctl start jenkins
sudo systemctl enable jenkins
Docker Installation
# Pull Jenkins image
docker pull jenkins/jenkins:lts
# Run Jenkins container
docker run -d -p 8080:8080 -p 50000:50000 \
-v jenkins_home:/var/jenkins_home \
--name jenkins jenkins/jenkins:lts
Initial Setup
Access Jenkins at
http://localhost:8080
Retrieve initial admin password:
sudo cat /var/lib/jenkins/secrets/initialAdminPassword
Install suggested plugins
Create admin user
Configure Jenkins URL
Jenkins Architecture
Components Overview
Jenkins Master
Handles scheduling
Manages slave nodes
Serves web interface
Stores configurations
Jenkins Agents/Slaves
Execute build jobs
Provide different environments
Distribute workload
Executors
Represent concurrent build capacity
Configurable per node
Prevent resource overload
Job Types
Freestyle Projects
Simple, single tasks
GUI-based configuration
Limited complexity support
Pipeline Projects
Complex workflows
Code-based configuration
Advanced features support
Multi-branch Pipelines
Automatic branch discovery
Different configurations per branch
Automated workflow per branch
Pipeline and Freestyle Jobs
Freestyle Jobs
Configuration
<?xml version='1.0' encoding='UTF-8'?>
<project>
<description>Example Freestyle Job</description>
<builders>
<hudson.tasks.Shell>
<command>echo "Hello, Jenkins!"</command>
</hudson.tasks.Shell>
</builders>
<publishers>
<hudson.tasks.Mailer>
<recipients>team@example.com</recipients>
</hudson.tasks.Mailer>
</publishers>
</project>
Pipeline Jobs
Declarative Pipeline Example
pipeline {
agent any
environment {
MAVEN_HOME = tool 'M3'
}
stages {
stage('Checkout') {
steps {
git 'https://github.com/username/repository.git'
}
}
stage('Build') {
steps {
sh "${MAVEN_HOME}/bin/mvn clean install"
}
}
stage('Test') {
parallel {
stage('Unit Tests') {
steps {
sh "${MAVEN_HOME}/bin/mvn test"
}
}
stage('Integration Tests') {
steps {
sh "${MAVEN_HOME}/bin/mvn verify"
}
}
}
}
stage('Deploy') {
steps {
sh './deploy.sh'
}
}
}
post {
always {
junit '**/target/surefire-reports/*.xml'
cleanWs()
}
success {
echo 'Build successful!'
emailext subject: 'Build Successful',
body: 'Your build has completed successfully.',
to: 'team@example.com'
}
failure {
echo 'Build failed!'
emailext subject: 'Build Failed',
body: 'Your build has failed. Please check the logs.',
to: 'team@example.com'
}
}
}
Nodes and Agents
Setting Up a Node
Via Web Interface
Navigate to "Manage Jenkins" → "Manage Nodes"
Click "New Node"
Configure:
Name
Number of executors
Remote root directory
Labels
Launch method
Availability
Via Configuration Script
import jenkins.model.*
import hudson.model.*
import hudson.slaves.*
import hudson.plugins.sshslaves.*
Jenkins.instance.addNode(
new DumbSlave(
"agent1",
"Agent description",
"/home/jenkins",
"2",
Node.Mode.NORMAL,
"label1",
new SSHLauncher(
"agent.example.com",
22,
SSHLauncher.lookupSystemCredentials("agent-ssh-key"),
null,
null,
null,
null,
null,
null,
null,
null
),
RetentionStrategy.INSTANCE,
new LinkedList()
)
)
Groovy Pipeline Syntax
Basic Structure
// Declarative Pipeline
pipeline {
agent any
stages {
stage('Example') {
steps {
echo 'Hello World'
}
}
}
}
// Scripted Pipeline
node {
stage('Example') {
echo 'Hello World'
}
}
Advanced Features
Parallel Execution
stage('Parallel Steps') {
parallel {
stage('Branch A') {
steps {
echo "On Branch A"
}
}
stage('Branch B') {
steps {
echo "On Branch B"
}
}
}
}
Conditional Execution
stage('Conditional') {
when {
branch 'master'
environment name: 'DEPLOY_TO', value: 'production'
}
steps {
echo 'Deploying to production'
}
}
Webhooks Configuration
Setting Up Webhooks
In Jenkins
Navigate to job configuration
Check "GitHub hook trigger for GITScm polling"
Save configuration
In GitHub
# Webhook URL format http://jenkins-url/github-webhook/
Configuration Steps
Go to repository settings
Add webhook
Set content type to application/json
Select events to trigger webhook
Webhook Security
// Pipeline with webhook security
pipeline {
triggers {
githubPush()
}
stages {
stage('Webhook Build') {
when {
expression {
return env.GIT_BRANCH ==~ /.*main/
}
}
steps {
echo 'Building after webhook trigger'
}
}
}
}
Master-Slave Architecture
Master Configuration
jenkins {
systemMessage = 'Jenkins Master Configuration'
numExecutors = 2
mode = Node.Mode.NORMAL
labelString = 'master'
securityRealm {
local {
allowsSignup = false
users {
user {
id = 'admin'
password = 'admin123'
}
}
}
}
}
Slave Configuration
// Slave node configuration
node('slave-label') {
stage('Build on Slave') {
ws('/path/to/workspace') {
checkout scm
sh 'mvn clean install'
}
}
}
Load Balancing
pipeline {
agent {
label 'linux && java'
}
stages {
stage('Distributed Build') {
parallel {
stage('Build 1') {
agent {
label 'agent1'
}
steps {
sh './build.sh'
}
}
stage('Build 2') {
agent {
label 'agent2'
}
steps {
sh './build.sh'
}
}
}
}
}
}
Best Practices
Security
Use HTTPS/SSH for communication
Implement proper authentication
Regular security updates
Access control lists
Performance
Distribute load appropriately
Monitor resource usage
Regular maintenance
Proper scaling
Maintenance
Regular backups
Log rotation
Plugin updates
Configuration management
Troubleshooting
Monitor build logs
Check system logs
Network connectivity
Resource allocation
This guide covers the fundamental aspects of Jenkins implementation and management. Regular updates and maintenance ensure optimal performance and security of your Jenkins installation.
Subscribe to my newsletter
Read articles from Kanav Gathe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
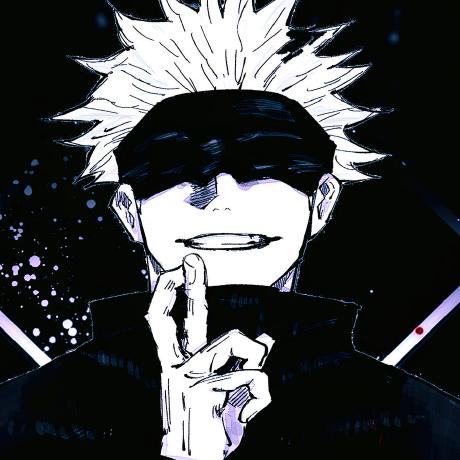