How a Hacker Could Exploit Canvas Fingerprinting

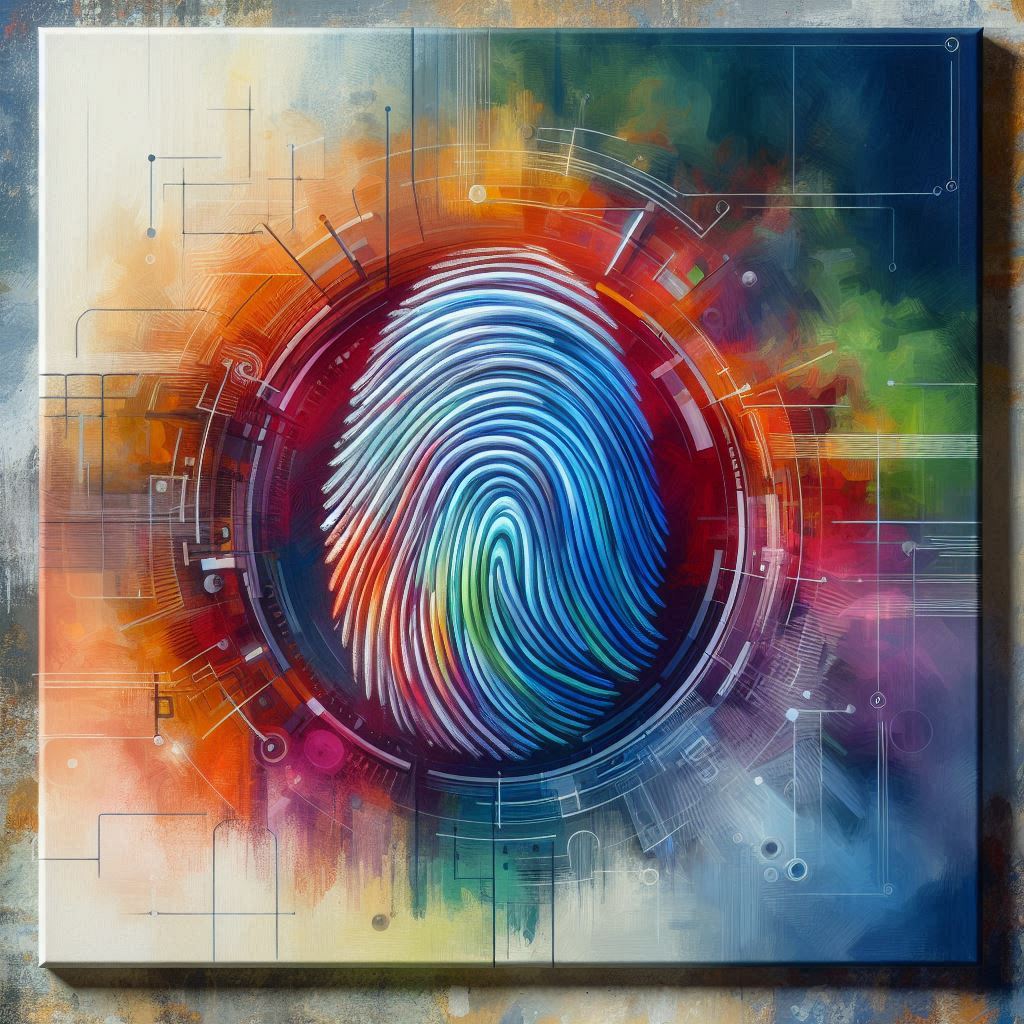
Canvas fingerprinting is a tracking technique where a unique identifier is generated based on the graphics rendering capabilities of a user's device, particularly the <canvas>
element in HTML. The process involves using the <canvas>
element to render text, images, or shapes and then extracting the image data from it to create a unique fingerprint of the device.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Canvas Fingerprinting</title>
</head>
<body>
<h1>Canvas Fingerprinting Example</h1>
<canvas id="canvas" width="200" height="200"></canvas>
<p id="fingerprint"></p>
<script>
function getCanvasFingerprint() {
// Create a canvas element and get its context
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
// Draw some text on the canvas
ctx.textBaseline = 'top';
ctx.font = '16px Arial';
ctx.fillText('Canvas Fingerprinting', 10, 10);
// Get the image data (pixel data)
var imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);
// Generate a fingerprint by converting the image data to a string
var fingerprint = imageData.data.toString();
// Return the fingerprint (this can be hashed to make it shorter)
return fingerprint;
}
// Display the fingerprint on the page
var fingerprint = getCanvasFingerprint();
document.getElementById('fingerprint').innerText = 'Fingerprint: ' + fingerprint;
</script>
</body>
</html>
How a Hacker Could Exploit Canvas Fingerprinting:
A hacker or malicious entity could use canvas fingerprinting to track users across different websites, even if they delete cookies or use incognito mode. This unique identifier is often persistent and hard to evade.
Example of Exploitation:
Tracking Across Sites:
A malicious website can use canvas fingerprinting to create a unique fingerprint of a user's device (e.g., by rendering text with slight differences that depend on the user's GPU, fonts, and device characteristics).
The website collects and stores this fingerprint, associating it with the user.
When the user visits another website that uses the same tracking method (e.g., through a third-party advertisement or analytics network), their fingerprint is sent back, revealing their identity or behavior.
Even if the user clears cookies or uses incognito mode, their fingerprint remains the same, allowing the malicious actor to track them across different sites.
Malicious Targeting:
If a hacker knows the user's fingerprint, they can monitor the user's online activities and even identify their login sessions on different websites.
They might track the user's browsing behavior, interests, and purchases to target them with phishing attacks, personalized scams, or malicious advertisements.
Data Correlation:
Once a unique fingerprint is established, malicious entities can correlate this information with other data sources (e.g., social media accounts, email addresses, and personal data breaches) to build a detailed profile of the user.
This data could be used for further exploitation, such as identity theft, fraud, or blackmail.
Risks of Being Tracked via Canvas Fingerprinting:
Here are the potential risks for users if their device is tracked through canvas fingerprinting:
1. Loss of Privacy:
The most obvious risk is the loss of privacy. Canvas fingerprinting allows websites to track users without their consent, even when they use privacy measures like incognito mode or cookie deletion.
Websites can track users across multiple sites, building a detailed history of their browsing activities.
2. Personalized Advertising and Profiling:
Tracking via canvas fingerprinting enables highly personalized advertising based on the user’s browsing habits, interests, and other behaviors. This could result in unwanted or invasive ads being served to the user.
Over time, this data can be used to create a user profile, which can include sensitive information like:
Political affiliations
Shopping habits
Health-related concerns (e.g., if the user searches for medical topics)
This profiling can lead to unwanted manipulation or targeting by advertisers and malicious parties.
3. Phishing Attacks:
If a hacker can track a user’s device across different sites, they can create highly personalized phishing attempts. For example, they may use knowledge of the user’s online behavior to craft emails or websites that appear legitimate.
The attacker may reference recent purchases or searches, which can make the phishing attempt more convincing.
4. Cross-Site Tracking (CST):
Since canvas fingerprints are persistent, users can be tracked across multiple websites. Even if they try to clear cookies or use a VPN, the unique fingerprint remains, leading to potential privacy breaches.
This could be particularly problematic in a scenario where the user accesses sensitive websites (e.g., banking, medical sites), and the attacker correlates their fingerprint with these activities.
5. Targeted Malicious Content:
Malicious actors can target users with malicious content based on their fingerprint. If a user is identified as having visited a website with known vulnerabilities or malware, they could be redirected to malicious sites or infected with malware.
Tracking the user’s interests could allow attackers to target them with social engineering tactics specific to their behaviors.
6. Unwanted Data Sharing:
Once the fingerprint is captured, the data might be sold or shared with third parties without the user’s knowledge. This data could be used for various purposes, including advertising, data brokers, or more malicious uses.
Companies could collect fingerprints to resell or create databases of user profiles, which could then be sold or traded.
7. Erosion of Anonymity:
Users may not be able to maintain their anonymity on the internet if their device can be uniquely identified across different websites.
This could be particularly risky for people who want to browse anonymously for personal, political, or professional reasons (e.g., journalists, activists, whistleblowers).
8. Difficult to Detect or Block:
Unlike cookies or traditional tracking mechanisms, canvas fingerprinting does not require any data to be stored on the user's device. This makes it harder to detect or block using common privacy tools.
Even with privacy-focused browser extensions or tools like Privacy Badger or uBlock Origin, canvas fingerprinting can sometimes bypass detection because it's based on how the device behaves, not how it stores information.
9. Increased Risk in Shared Devices:
If multiple people use the same device (e.g., a family computer or public terminal), canvas fingerprinting could wrongly associate a user’s activities with another person who uses the same machine, leading to false identification.
This could cause privacy issues, especially if one of the users engages in sensitive activities or uses the device for high-risk online behavior (e.g., accessing personal accounts or medical information).
How to Mitigate Risks:
1. Use Anti-Tracking Tools:
Privacy Badger and uBlock Origin can block canvas fingerprinting and other tracking methods.
Extensions like NoScript can block JavaScript from running, preventing the creation of a fingerprint.
2. Use Privacy-Focused Browsers:
Brave browser has built-in protections against fingerprinting and tracking.
Firefox has advanced privacy settings, including the ability to block canvas fingerprinting.
3. Disable JavaScript (Advanced):
- Disabling JavaScript will prevent the majority of canvas fingerprinting scripts from running, but this can break many websites.
4. Use VPNs or Proxy Services:
- Although canvas fingerprints are tied to device characteristics and not IP addresses, using a VPN can add an extra layer of anonymity by hiding your location and IP address.
5. Browser Fingerprint Spoofing:
- Tools like CanvasBlocker (for Firefox) can spoof your canvas fingerprint, making it harder for trackers to uniquely identify your device.
6. Incognito or Private Browsing Mode:
- While this won’t fully protect against canvas fingerprinting, it does block cookies and localStorage tracking, making it a useful privacy measure.
Example: Detecting Canvas Fingerprinting
Step-by-Step Instructions:
Open the Browser's Developer Tools:
In Google Chrome, Firefox, or Edge, press
F12
orCtrl + Shift + I
(Windows/Linux) orCmd + Option + I
(Mac) to open the Developer Tools.Go to the Console tab.
Copy the Detection Code:
- Copy the detection code (for canvas fingerprinting, cookies, etc.) into your clipboard. Here’s an example for canvas fingerprinting detection:
(function() {
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
ctx.textBaseline = 'top';
ctx.font = '16px Arial';
ctx.fillText('Canvas Fingerprinting Test', 10, 10);
try {
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);
const fingerprint = imageData.data.toString();
alert('Canvas fingerprinting detected!');
console.log('Fingerprint:', fingerprint);
} catch (e) {
console.log('Canvas fingerprinting not detected.');
}
})();
- Paste the Code in the Console:
Paste the code into the console and press Enter.
If the site is performing canvas fingerprinting, you will see an alert, and the fingerprint string will be logged to the console. If no fingerprinting is detected, it will log "Canvas fingerprinting not detected."
Conclusion:
Canvas fingerprinting is a significant privacy risk because it allows persistent tracking of users across different websites without relying on cookies. Hackers or malicious websites can exploit this method for phishing, malicious content delivery, and even profiling. The risks of being tracked by canvas fingerprinting include loss of privacy, targeted advertising, and vulnerability to social engineering attacks.
To protect against canvas fingerprinting, users should use anti-tracking tools, privacy-focused browsers, and take other privacy precautions, such as using VPNs and disabling JavaScript where necessary.
Subscribe to my newsletter
Read articles from Nguyen Thi Thao directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
