Jenkins Interview Guide: From Basic Concepts to Real-World Scenarios
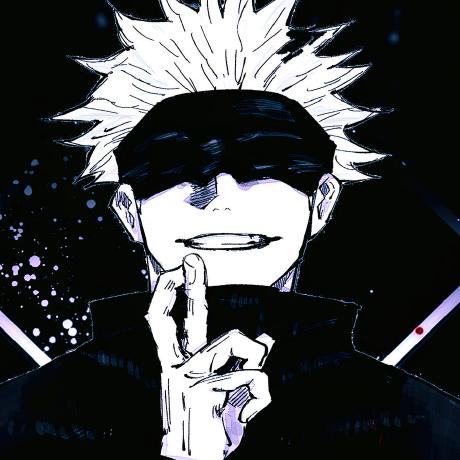
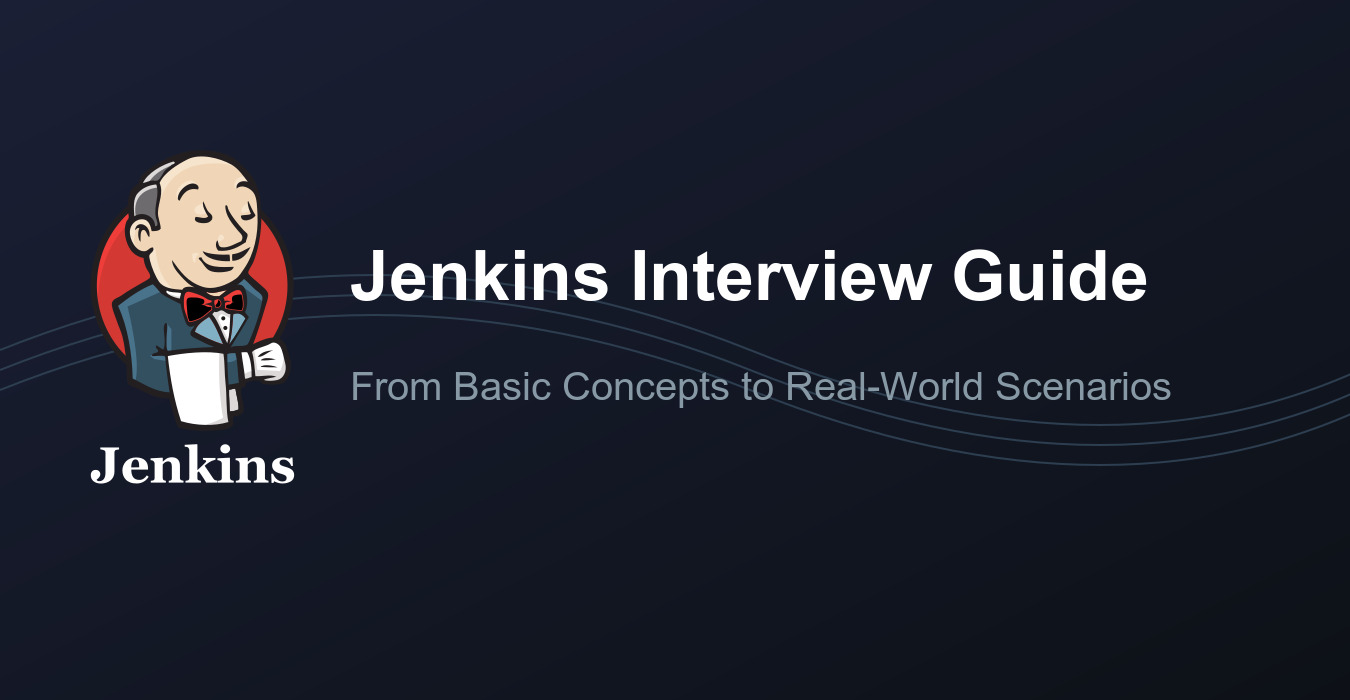
General Questions
1. What's the difference between continuous integration, continuous delivery, and continuous deployment?
Continuous Integration (CI) focuses on automatically integrating code changes from multiple developers into a shared repository. When developers commit code, automated processes build the application and run tests to verify that new changes integrate smoothly with existing code. For example, when a developer pushes code to Git, Jenkins automatically triggers a build and runs unit tests.
Continuous Delivery (CD) extends CI by automatically preparing code for production deployment. The code remains in a deployable state, but actual deployment requires manual approval. This gives teams control over release timing while maintaining automation benefits. For instance, after passing tests, an application is automatically deployed to staging environments but needs approval before going to production.
Continuous Deployment takes automation further by automatically deploying every change that passes tests to production without human intervention. This requires robust testing and monitoring but enables rapid feature delivery. For example, companies like Netflix use continuous deployment to push multiple updates daily without manual approvals.
2. Benefits of CI/CD
The adoption of CI/CD practices brings substantial benefits to development teams:
Speed and Efficiency: Teams can deploy changes multiple times daily instead of weekly or monthly releases. Automated processes handle repetitive tasks, allowing developers to focus on writing code. A process that might take hours manually can be completed in minutes.
Quality Assurance: Automated testing catches bugs early when they're cheaper and easier to fix. Integration issues are identified immediately rather than during final integration. For example, if a developer introduces a breaking change, they'll know within minutes rather than discovering it during release.
Risk Management: Smaller, frequent deployments mean each change carries less risk. If issues occur, teams can quickly identify and fix problematic changes or roll back to stable versions. This reduces downtime and improves system reliability.
Cost Efficiency: Early bug detection significantly reduces fix costs. Automated processes eliminate manual deployment tasks, allowing teams to focus on development.
3. What is meant by CI-CD?
CI/CD represents a comprehensive software development methodology that emphasizes automation and continuous monitoring throughout the application lifecycle. It combines:
Development Practices: Regular code integration, automated testing, and continuous feedback loops ensure code quality from the start. Automation Tools: Jenkins, Git, automated testing frameworks, and deployment tools work together to create a seamless pipeline. Cultural Philosophy: Teams embrace collaboration, shared responsibility, and continuous improvement as core values. Infrastructure Management: Automated provisioning and configuration management ensure consistent environments across stages.
4. What is Jenkins Pipeline?
Jenkins Pipeline is a suite of plugins that enables implementing continuous delivery pipelines as code. Rather than configuring jobs through the UI, you define your entire build/test/deploy process in a Jenkinsfile. This supports:
Version Control: Pipeline configurations are stored alongside application code, making changes trackable and reviewable. Code Reuse: Teams can create template pipelines and share common configurations across projects. Visualization: Jenkins provides a clear view of pipeline stages and their status. Complex Workflows: Support for parallel execution, conditional steps, and custom error handling.
5. How do you configure a job in Jenkins?
Job configuration in Jenkins involves several key steps:
Initial Setup: Create a new item, select the job type (Freestyle, Pipeline, etc.), and provide a descriptive name. Source Code Management: Configure repository details, credentials, and branch specifications. Build Triggers: Define when the job should run (commit-based, scheduled, or manual). Build Environment: Set up tools, variables, and credentials needed for the build. Build Steps: Define the actual build process, including compilation, testing, and packaging. Post-build Actions: Configure notifications, artifact archiving, and downstream job triggers.
6. Where do you find errors in Jenkins?
Jenkins provides multiple locations for error investigation:
Console Output: Provides real-time build execution logs with detailed error messages. System Logs: Located at /var/log/jenkins/jenkins.log, containing server-side issues. Build History: Shows trends and historical build results. Plugin Logs: Specific to plugin operations and configurations. Jenkins Dashboard: Offers a high-level view of job status and recent failures.
7. In Jenkins, how can you find log files?
Jenkins maintains logs in several locations:
System Logs (/var/log/jenkins/jenkins.log): Contains Jenkins server operations and errors. Build Logs (JENKINS_HOME/jobs/[job-name]/builds/[build-number]/log): Specific build execution details. Agent Logs (JENKINS_HOME/logs/slaves/): Information about distributed build agents. Plugin Logs (JENKINS_HOME/logs/): Plugin-specific logging information. Archived Logs: Past build logs stored according to retention policies.
8. Jenkins workflow and script example?
A typical Jenkins workflow encompasses:
Code Checkout: Retrieving source code from version control. Build: Compiling and packaging the application. Test: Running unit and integration tests. Analysis: Code quality checks and security scans. Deployment: Moving artifacts to target environments. Notification: Informing stakeholders of build results.
9. How to create continuous deployment in Jenkins?
Implementing continuous deployment requires:
Pipeline Definition: Create a Jenkinsfile defining all stages from build to deployment. Automated Testing: Comprehensive test coverage including unit, integration, and acceptance tests. Environment Configuration: Automated environment provisioning and configuration. Deployment Scripts: Reliable deployment automation with rollback capabilities. Monitoring: Automated health checks and performance monitoring.
10. How to build a job in Jenkins?
Building a job involves:
Job Creation: Select "New Item" and choose the appropriate job type. Basic Configuration: Set up source code management and build triggers. Build Definition: Specify build steps and required tools. Testing: Configure test execution and reporting. Artifact Management: Define how build outputs are handled. Deployment: Set up deployment steps if needed.
11. Why do we use pipelines in Jenkins?
Pipelines offer significant advantages:
Code as Configuration: Pipeline definitions in version control. Visualization: Clear view of build/deploy processes. Reusability: Shared libraries and templates. Flexibility: Support for complex workflows. Reliability: Consistent, repeatable processes.
12. Is Jenkins alone sufficient for automation?
Jenkins requires integration with various tools:
Version Control: Git, SVN for code management. Build Tools: Maven, Gradle, npm for building applications. Testing Tools: JUnit, Selenium for automated testing. Deployment Tools: Docker, Kubernetes for containerization. Monitoring: Prometheus, Grafana for system monitoring.
13. How will you handle secrets in Jenkins?
Secure secret management involves: Credentials Plugin: Secure storage for passwords and keys. Environment Variables: Runtime configuration management. Vault Integration: External secret management systems. Access Controls: Role-based access to sensitive data. Secret Rotation: Regular updates to credentials.
14. Explain the different stages in a CI-CD setup
A typical CI/CD pipeline includes:
Source Stage: Code checkout and validation. Build Stage: Compilation and packaging. Test Stage: Automated testing at multiple levels. Quality Stage: Code analysis and security scanning. Deploy Stage: Automated deployment to environments. Monitor Stage: Performance and health monitoring.
15. Name some of the plugins in Jenkins
Essential Jenkins plugins include:
Git Plugin: Version control integration. Pipeline: Continuous delivery pipeline support. Docker: Container management. Credentials: Secure credential storage. Email Extension: Enhanced notification capabilities. JUnit: Test result reporting.
Scenario-Based Questions and Solutions
1. You have a Jenkins pipeline that deploys to a staging environment. Suddenly, the deployment failed due to a missing configuration file. How would you troubleshoot and resolve this issue?
When dealing with missing configuration deployment failures, start by examining the Jenkins console output to pinpoint the exact configuration file that's missing. Configuration files are crucial for deployments, and their absence can occur for various reasons.
First, check your version control system. Has the file been recently modified, deleted, or accidentally added to .gitignore? Review recent commits and branch merges - often configuration issues arise during merges or when deployment scripts are modified. Pay attention to file permissions and path changes.
Access your staging server and verify the deployment directory structure. Compare configurations across environments - is the file present in development or production? This comparison helps identify if it's an environment-specific issue or a broader problem. Check if environment variables that might affect file paths are set correctly.
For immediate resolution, restore the missing configuration from a known good state or create it from a template. Verify file permissions and ensure the Jenkins deployment user has appropriate access rights.
For long-term prevention:
Implement pre-deployment validation checks that verify all required configurations exist
Create standardized configuration templates for each environment
Use configuration management tools like Ansible or Chef
Set up monitoring alerts for critical configuration changes
Document configuration requirements and establish review processes
2. Imagine you have a Jenkins job that is taking significantly longer to complete than expected. What steps would you take to identify and mitigate the issue?
Performance degradation in Jenkins jobs requires systematic investigation and optimization. Begin by analyzing your build history to identify when the slowdown started. Was it a gradual increase or a sudden change? This timeline helps narrow down potential causes.
Review the Jenkins console output and build logs thoroughly. Which specific stages or steps are taking longer? Is it during compilation, testing, or deployment? Sometimes certain stages become bottlenecks while others maintain normal performance.
Monitor system resources on both Jenkins master and build agents:
CPU utilization patterns
Memory consumption and potential leaks
Disk I/O operations and potential bottlenecks
Network bandwidth usage and latency
Build queue length and executor utilization
Common issues that cause slowdowns include:
Insufficient cleanup between builds
Sequential execution of tasks that could run in parallel
Inefficient test execution strategies
Resource contention between jobs
Growing workspace size
Inadequate hardware resources
Implement solutions based on findings:
Enable parallel execution where possible
Configure proper workspace cleanup
Optimize test execution and build scripts
Implement efficient caching mechanisms
Upgrade hardware resources if necessary
Set up build time monitoring and alerts
3. You need to implement a secure method to manage environment-specific secrets for different stages (development, staging, production) in your Jenkins pipeline. How would you approach this?
Secret management in Jenkins requires a comprehensive security strategy. Begin by assessing what types of secrets you need to manage: API keys, database credentials, certificates, or other sensitive data.
Implement the Jenkins Credentials Plugin as your foundation. This provides encrypted storage and secure access to credentials. Create separate credential stores for each environment to maintain isolation between development, staging, and production secrets.
For enhanced security:
Integrate with external secret management services (HashiCorp Vault, AWS Secrets Manager)
Implement role-based access control (RBAC)
Set up audit logging for all secret access
Enable automatic secret rotation
Use encryption for secrets at rest and in transit
In your pipeline, use the withCredentials block to securely inject secrets into build steps. Never expose secrets in build logs or environment variables. Implement proper error handling to prevent accidental secret exposure in stack traces.
Establish processes for:
Regular secret rotation
Access review and revocation
Emergency secret replacement
Audit log monitoring
Security incident response
4. Suppose your Jenkins master node is under heavy load and build times are increasing. What strategies can you use to distribute the load and ensure efficient build processing?
Load distribution for Jenkins requires both architectural changes and optimization strategies. Start by understanding your current load patterns - are certain times of day particularly busy? Are specific job types causing the most load?
Implement a distributed builds architecture:
Set up multiple build agents across different machines
Configure agent labels for specialized builds
Use cloud-based agents for dynamic scaling
Keep the master node focused on orchestration
Configure intelligent job distribution:
Prioritize critical builds
Implement queue management
Set up resource throttling
Use load balancing plugins
For optimal performance:
Monitor agent health and performance
Implement proper cleanup routines
Configure resource limits
Use caching effectively
Optimize build scripts
5. A developer commits a code change that breaks the build. How would you set up Jenkins to automatically handle such scenarios and notify the relevant team members?
Build failure management requires automated detection and response systems. Implement pre-commit hooks that run basic tests before allowing commits. Configure your Jenkins pipeline to run comprehensive tests on all changes.
Set up notifications:
Email alerts to the commit author and team
Slack/Teams integration for immediate notification
Dashboard displays for build status
SMS alerts for critical failures
Implement automatic responses:
Block merging of failing code
Trigger automated rollback if necessary
Create Jira/GitHub issues automatically
Generate detailed failure reports
For better tracking:
Record build failure patterns
Maintain build health statistics
Track mean time to recovery
Monitor test coverage trends
6. You are tasked with setting up a Jenkins pipeline for a multi-branch project. How would you handle different configurations and build steps for different branches?
Multi-branch pipeline management requires careful configuration and organization. Use Jenkinsfile to define branch-specific behaviors. Implement branch discovery to automatically create pipelines for new branches.
For configuration management:
Use conditional logic based on branch names
Maintain environment-specific variables
Configure different deployment targets
Set up appropriate security controls
Implement branch-specific behaviors:
Different test suites for feature branches
Specialized deployment procedures
Varying resource allocations
Custom notification settings
7. How would you implement a rollback strategy in a Jenkins pipeline to revert to a previous stable version if the deployment fails?
A robust rollback strategy is essential for production stability. First, establish a comprehensive versioning system for your applications, keeping complete copies of each successful deployment including application code, configuration files, database schemas, and environment settings in an artifact repository like Artifactory or Nexus. Consider maintaining a "last known good" tag that's updated only after thorough validation of a new deployment.
Create automated health checks that run immediately after deployment. These should verify critical application functions, database connectivity, external service integrations, and key business operations. Define clear metrics for deployment success - for instance, error rates below 0.1%, response times under 200ms, and all critical services responding.
When a deployment fails these health checks, your rollback procedure should automatically:
Stop incoming traffic to the failing deployment
Restore the previous application version from your artifact repository
Apply the corresponding database state (through snapshots or reverse migrations)
Restore any environment-specific configurations
Run health checks again to verify the rollback succeeded
Most importantly, test your rollback procedures regularly. Include rollback testing in your deployment pipeline, perhaps during off-hours, to ensure the process works when needed. Maintain detailed logs of all deployments and rollbacks for troubleshooting and compliance purposes.
8. In a scenario where you have multiple teams working on different projects, how would you structure Jenkins jobs and pipelines to ensure efficient resource utilization and manage permissions?
Managing multiple teams requires careful organization and access control. Structure your Jenkins environment like a well-organized company with clear boundaries and shared resources. Create a hierarchical folder structure that mirrors your organization - for example, separate folders for frontend, backend, and infrastructure teams, with subfolders for specific projects or applications.
Implement granular role-based access control (RBAC) that gives teams autonomy within their space while protecting shared resources. For instance, developers might have full access to their team's jobs but read-only access to other teams' build results. Use Project-based Matrix Authorization Strategy plugin to manage permissions at the folder level.
Resource management becomes critical in multi-team setups. Each team should have dedicated build agents with appropriate tools and configurations for their specific needs. Shared resources like deployment environments or specialized testing tools should be managed through a resource scheduling system. For example, use the Lockable Resources plugin to prevent multiple teams from deploying to staging simultaneously.
9. Your Jenkins agents are running in a cloud environment, and you notice that build times fluctuate due to varying resource availability. How would you optimize the performance and cost of these agents?
Cloud-based Jenkins agents require sophisticated optimization for both performance and cost. Start by implementing detailed monitoring of your build processes - track build times, resource utilization, queue times, and costs. Use this data to identify patterns and optimize accordingly.
Create distinct agent configurations based on workload types. Compile-heavy Java builds might need compute-optimized instances, while JavaScript builds might run fine on smaller instances. Use spot instances for non-time-critical builds to reduce costs, but maintain a base level of on-demand instances for critical jobs.
Implement intelligent caching strategies. Use artifact caching to avoid repeatedly downloading dependencies. Configure workspace cleanup policies based on instance storage costs versus build time impact. For example, keep workspaces for frequently built branches but clean up feature branch workspaces after merging.
Set up auto-scaling policies that consider both queue length and cost constraints. Scale up quickly during peak hours but scale down aggressively during off-hours. Monitor instance lifecycle - terminate instances that have been idle too long or show degraded performance.
Containerize your build environments using Docker to ensure consistency and faster startup times. Maintain optimized base images with commonly used tools and dependencies pre-installed. Use multi-stage builds to keep final images small and efficient.
Regular optimization reviews are crucial. Analyze cost and performance metrics monthly, identifying opportunities for improvement. Consider factors like instance family selection, region placement, and reserved instance purchases for predictable workloads.
Subscribe to my newsletter
Read articles from Kanav Gathe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
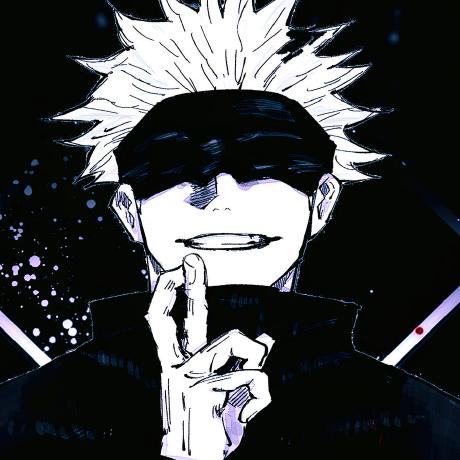