Article on Pytest for API Testing: Comparison with Karate and SuperTest

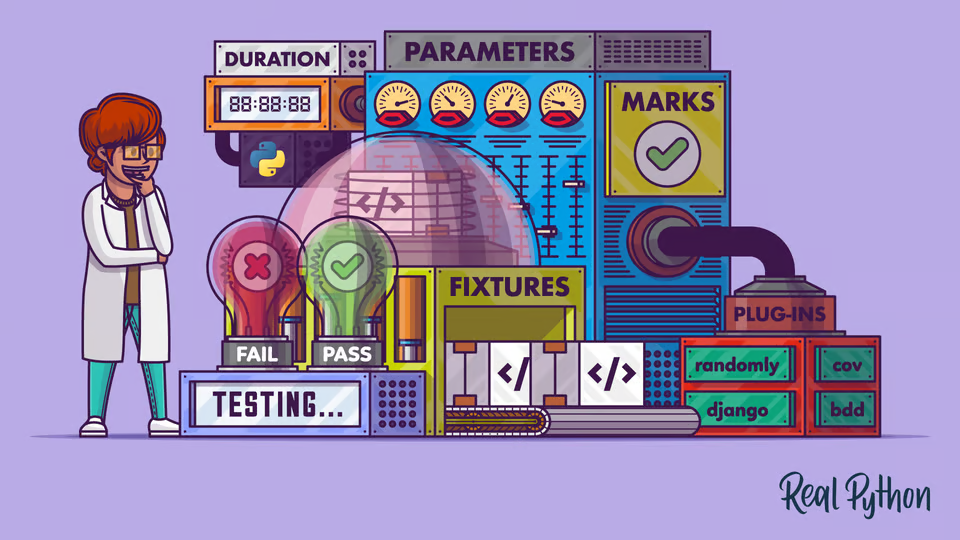
Introduction
In this article, we will explore Pytest, a popular Python testing framework, in the context of testing RESTful APIs, comparing it with other popular frameworks like Karate (for Java) and SuperTest (for Node.js). The goal is to provide a clear overview of the features, advantages, and disadvantages of each framework to help developers make informed decisions when choosing a framework for their API testing projects.
What is Pytest?
Pytest is a widely used testing framework in the Python community. It is known for its simplicity, flexibility, and ability to handle both unit and integration testing, making it an excellent choice for API testing.
Key Features of Pytest:
Simplicity in Test Writing: Pytest is easy to use, especially for Python developers. Its syntax is clean and concise, making it simple to write tests.
Extensibility: Pytest can be extended through plugins to add new functionalities, such as simulating HTTP servers, running asynchronous tests, and integrating with other tools.
Asynchronous Testing Support: With plugins like
pytest-asyncio
, Pytest allows you to write tests for APIs that make asynchronous requests, which is useful for modern APIs that use async/await.CI/CD Integration: Pytest is widely compatible with continuous integration and continuous deployment tools like Jenkins, GitLab CI, CircleCI, among others.
Installation and Setup
To get started with Pytest, simply install it using pip (Python's package manager):
pip install pytest
Once installed, you can write your tests in files prefixed with test_
, for example, test_
api.py
.
Basic Test Example with Pytest:
import requests
def test_get_api():
response = requests.get("https://api.example.com/data")
assert response.status_code == 200
assert "key" in response.json()
This is a simple example of a GET test using Pytest to validate that an API returns a 200 status code and contains the key "key"
in the response.
Advantages of Pytest for API Testing
1. Flexibility and Extensibility
Pytest is extremely flexible. There are plugins like pytest-httpserver that allow you to mock API responses during tests, which is useful for integration testing. Additionally, you can add other functionalities like performance tests or integrate with security testing services.
2. Support for Asynchronous Tests
In modern applications, APIs often require asynchronous calls. Pytest-asyncio is a plugin that allows you to easily handle asynchronous tests in Python.
Asynchronous Test Example:
import pytest
import aiohttp
import asyncio
@pytest.mark.asyncio
async def test_async_api():
async with aiohttp.ClientSession() as session:
async with session.get("https://api.example.com/data") as response:
assert response.status == 200
data = await response.json()
assert "key" in data
This example shows how to perform an asynchronous test of an API using Pytest and aiohttp.
3. Easy Integration with CI/CD
Pytest has excellent integration with CI/CD tools, making it easy to automate the execution of tests as changes are made to the code. Being based in Python, it also integrates seamlessly with Python-based CI/CD pipelines.
Disadvantages of Pytest
1. Limited to Python
Pytest is exclusive to projects written in Python. If your project isn't based on Python, you'll need to resort to other frameworks (such as RestAssured for Java or SuperTest for Node.js).
2. No Native UI Testing Support
While Pytest is excellent for API testing, it doesn't natively support UI testing. However, this can be addressed by integrating Pytest with other tools like Selenium or Playwright if necessary.
Comparison with Karate and SuperTest
Feature | Pytest (Python) | Karate (Java) | SuperTest (Node.js) |
Language | Python | Java | Node.js |
Syntax | Simple and flexible | Gherkin (similar to Cucumber) | Simple JavaScript syntax |
API Testing | REST, SOAP, GraphQL | REST, SOAP, GraphQL | REST |
Asynchronous Testing | Supported with pytest-asyncio | Not optimized for asynchronous testing | Natively supported in Node.js |
UI Testing | Needs integration with Selenium/Playwright | Built-in support for UI testing | No support for UI testing |
Ease of Use | Easy to use for Python developers | Easy for non-technical testers (with Gherkin) | Easy for Node.js developers |
CI/CD Integration | High compatibility | High compatibility | High compatibility |
Community | Large Python community | Good support in the Java community | Strong support in the Node.js community |
Conclusion
Pytest is an excellent choice for API testing in Python projects. Its flexibility, support for asynchronous testing, and easy integration with CI/CD tools make it a great framework for efficient and scalable testing.
Compared to Karate and SuperTest, Pytest excels in terms of simplicity for Python developers, while Karate is more suited for those looking for an all-in-one solution in Java with integrated API and UI testing support. SuperTest, on the other hand, is ideal for Node.js projects, but it lacks support for UI testing and is more focused on JavaScript syntax.
Ultimately, the choice of framework will depend on the language you're using and the specific needs of your project.
Subscribe to my newsletter
Read articles from JAIME ELIAS FLORES QUISPE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
