Understanding Lombok: Simplify Your Java Code with Less Boilerplate

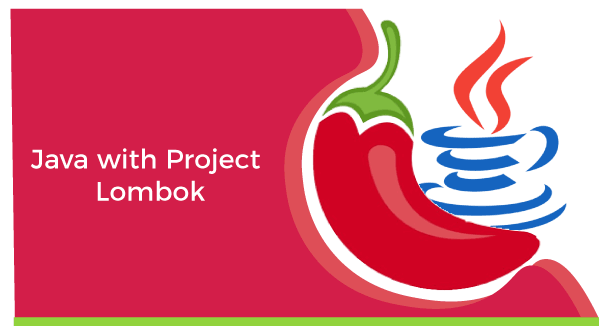
Boilerplate refers to sections of code that are repeated in multiple places with little to no variation. example- Getter and Setters
public class User {
private String name;
private String email;
private int age;
// Getters and Setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Such codes creates these problems:
Time-Consuming: Writing repetitive code takes up time that could be spent on solving actual problems.
Error-Prone: Manually adding repetitive code increases the risk of errors, such as typos or logical mistakes.
Hard to Maintain: If fields are added or modified, you must update all related boilerplate code.
To solve this problem Lombok is used, Lombok is a library that eliminates boilerplate code by using annotations.
Project Lombok: is a java library that automatically plugs into your editor and build tools, spicing up your java.
Never write another getter or equals method again, with one annotation your class has a fully featured builder, Automate your logging variables, and much more.
For example
import lombok.Data;
@Data
public class Person {
private String name;
private int age;
}
Here, the @Data annotation is used, which automatically generates Getters and Setters. This annotation also contains the toString(), equals(), and hashCode() methods and a parameterized constructor. If you want to use only getters and setters, just use @Getters and @Setters.
If you want to use Lombok, first you need to add dependency in project , in pom.xml.
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.36</version>
<scope>provided</scope>
</dependency>
After this just reload pom.xml. and add @Data annotation where you want to generate Getters and Setters and other thing automatically.
Here is the code after the use of Lombok
import lombok.Data;
@Data
public class User {
private String name;
private String email;
private int age;
}
If you'd like to contribute suggestions or point out any corrections, feel free to let me know! Your feedback is valuable and helps ensure accurate and helpful content. ๐
Subscribe to my newsletter
Read articles from Niraj Sahani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Niraj Sahani
Niraj Sahani
Iโm Niraj Sahani, a passionate software developer with a keen interest in creating Android apps. My expertise includes Java programming, Data Structures and Algorithms (DSA), and MySQL. I am dedicated to building efficient and user-friendly applications, while continually expanding my knowledge of new technologies. As a quick learner and problem solver, I am eager to contribute to innovative projects and grow in the tech industry.