APIs Uncovered: The Gateway to Data and Functionality
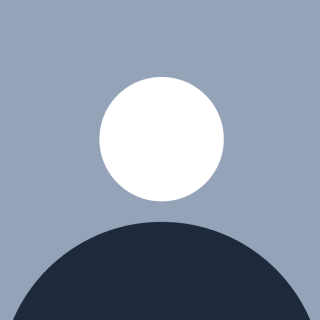
Table of contents
- APIs – The Bridge Between Systems
- What is an API? – The Digital Bridge
- How APIs Work – The Request-Response Cycle
- Basic API Request-Response Cycle
- HTTP Methods – How APIs Make Requests
- HTTP Methods in a Space Mission Context
- Understanding Status Codes – Feedback from the Server
- Building Our First Simple API – The Space Mission Info API
- Experiment with HTTP Methods
- Recap of HTTP Methods and Status Codes
- Laying the Foundation for APIs
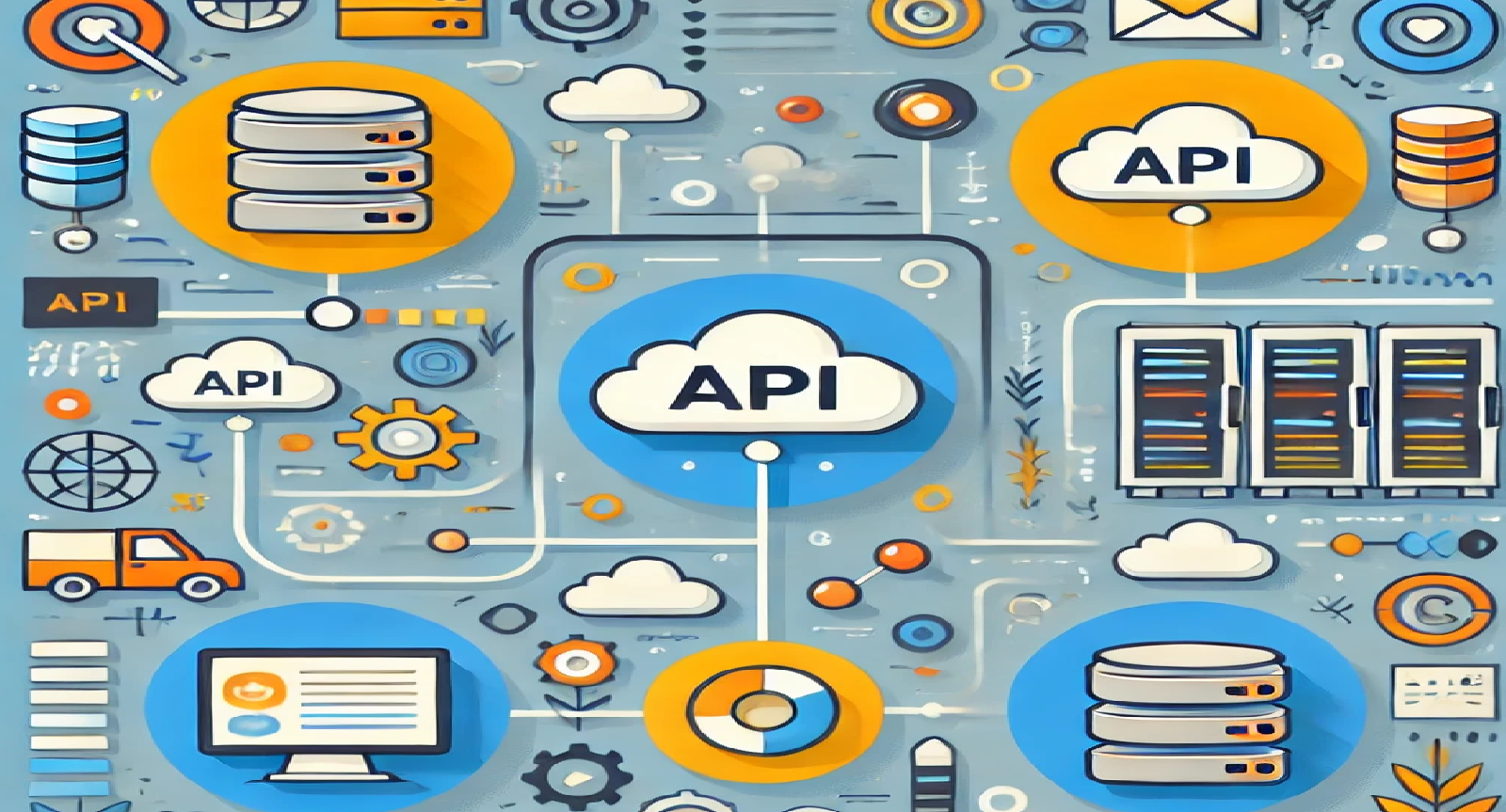
APIs – The Bridge Between Systems
Imagine you’re managing a space station and need data from various sources—weather on Mars, space mission statuses, and more. APIs (Application Programming Interfaces) act as digital translators that allow your system to communicate with others, regardless of the programming languages or platforms they use.
From checking weather forecasts to booking a flight, APIs enable applications to exchange data seamlessly. In this article, we’ll break down how APIs work, why they’re essential, and gradually build a simple Space Mission Info API using JavaScript. Let’s get started!
What is an API? – The Digital Bridge
At its core, an API is a set of rules and protocols that enables one application to access the data and functionality of another. APIs let different systems communicate, making it easy to integrate features or access data from other services.
Example: Suppose you’re building a dashboard that displays Mars weather data. Instead of manually entering data, your dashboard can request information from a weather service API. The API responds with the data, which you then display on your dashboard.
How APIs Work – The Request-Response Cycle
APIs operate using a request-response cycle, a simple exchange between the client (your app) and the server (the API provider). Here’s how it works:
Request: Your app (client) sends a request to the API (server), asking for specific data or to perform an action.
Response: The API processes this request and sends back a response with the requested data or a status update.
This cycle allows APIs to act as messengers between systems, enabling secure and structured communication.
Basic API Request-Response Cycle
Here’s a flowchart to visualize the request-response cycle:
[ Client (Your App) ]
|
Sends Request
|
[ API (e.g., Weather Service) ]
|
Processes Request
|
Sends Response
|
[ Client (Displays Data) ]
In this model, the client application requests specific data from the API, and the API responds with data in a format that the client understands, often JSON.
HTTP Methods – How APIs Make Requests
APIs use different HTTP methods to define the type of action being requested. Think of HTTP methods as verbs that indicate what kind of operation the client wants to perform. Here’s an overview of the most common methods:
GET: Requests data from the server without changing anything. Think of it as viewing information (e.g., retrieving weather data).
POST: Sends data to the server to create something new. This method is used when you want to add new data (e.g., creating a new mission log).
PUT: Updates existing data on the server. It’s like editing or replacing information (e.g., updating a mission status).
DELETE: Removes data from the server. It’s like deleting a record or an item (e.g., deleting a mission entry).
Each method has a specific purpose and helps structure the API in a way that makes it easy to understand and interact with.
HTTP Methods in a Space Mission Context
GET /missions: Retrieve a list of all missions.
POST /missions: Add a new mission to the list.
PUT /missions/: Update a specific mission based on its ID.
DELETE /missions/: Remove a mission based on its ID.
These methods allow clients to interact with the API in a standardized way, making it clear what each route does.
Understanding Status Codes – Feedback from the Server
When you make a request, the server responds with a status code. These codes provide feedback on the request, letting you know if it was successful or if there was an issue. Here are some of the most common status codes:
200 OK: The request was successful, and the server is responding with the requested data.
201 Created: The request was successful, and a new resource was created (usually with POST requests).
400 Bad Request: The request could not be understood due to invalid syntax.
404 Not Found: The server could not find the requested resource.
500 Internal Server Error: The server encountered an unexpected condition that prevented it from fulfilling the request.
Each status code provides valuable information about the result of the request, helping developers troubleshoot and understand what happened.
Building Our First Simple API – The Space Mission Info API
With the basics of HTTP methods and status codes covered, let’s build a simple Space Mission Info API. This API will allow clients to request information about space missions, and we’ll add functionality to create new missions as well.
Step 1: Set Up the Project
Start by setting up a new Node.js project. Open your terminal, create a new folder, and navigate into it. Then initialize npm to manage the project’s dependencies:
mkdir space-mission-api
cd space-mission-api
npm init -y
This command creates a package.json
file to track dependencies.
Step 2: Install Express
We’ll use Express to simplify routing and manage HTTP methods easily:
npm install express
Express is like the control module of our API, allowing us to define clear and structured routes.
Step 3: Write the API Code
Create a new file called index.js
and add the following code:
// Import Express
const express = require('express');
const app = express();
app.use(express.json()); // Middleware to parse JSON data
// Mission data
let missions = [
{ id: 1, name: "Apollo 11", destination: "Moon", launchDate: "1969-07-16" },
{ id: 2, name: "Voyager 1", destination: "Interstellar space", launchDate: "1977-09-05" },
{ id: 3, name: "Mars Rover", destination: "Mars", launchDate: "2003-06-10" }
];
// GET route to retrieve all missions
app.get('/missions', (req, res) => {
res.status(200).json(missions);
});
// POST route to create a new mission
app.post('/missions', (req, res) => {
const newMission = req.body;
newMission.id = missions.length + 1;
missions.push(newMission);
res.status(201).json(newMission); // Sends back the created mission
});
// Start the server on port 3000
app.listen(3000, () => {
console.log('Space Mission API is running on port 3000');
});
Explanation:
app.get('/missions'): Handles GET requests and sends back the list of missions.
app.post('/missions'): Handles POST requests, adds a new mission to the list, and returns the newly created mission.
app.use(express.json()): Middleware that parses incoming JSON data so that we can access it via
req.body
.
When you run node index.js
and visit http://localhost:3000/missions in your browser, you should see a JSON response with the mission data.
Experiment with HTTP Methods
Now that you understand GET and POST, try adding more routes to expand the Space Mission API:
GET /missions/: Allow users to retrieve a specific mission by its ID.
PUT /missions/: Update an existing mission with new information.
DELETE /missions/: Remove a mission from the list.
Recap of HTTP Methods and Status Codes
GET: Retrieve data (e.g., all missions).
POST: Create a new resource (e.g., add a new mission).
Status Codes: Indicate the outcome of the request, helping to provide feedback on what happened (e.g., 200 for success, 404 for not found).
Laying the Foundation for APIs
In this part of building a RESTful API, we’ve:
Explored what APIs are and why they’re essential for data exchange.
Learned about HTTP methods (GET, POST) and status codes.
Built a basic Space Mission Info API with routes to retrieve and add missions.
In the next part, we’ll dive deeper into REST principles, cover additional HTTP methods, and learn to structure our API for more complex interactions!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by