Building a Credit Card Validator with Golang

Table of contents
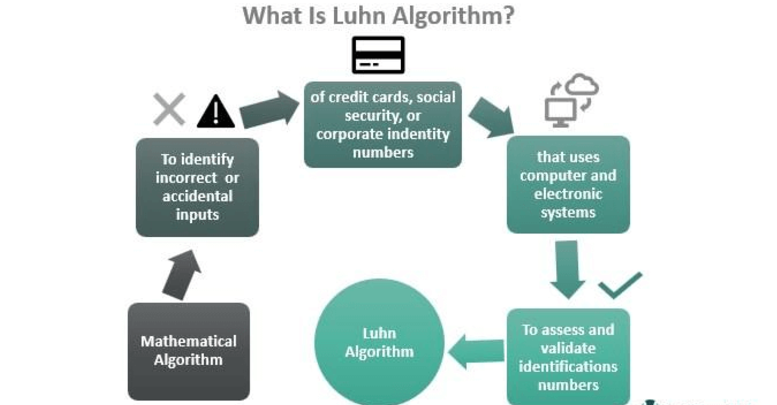
Nowadays, fraudsters are all around us due to the development of new technologies and the Internet. Each time you shop online there is always a possibility that somebody somewhere is trying to use a credit card that has been stolen. And guess what? They are still quite aware of it.
That is why, when designing secure applications, one of the first activities to be implemented is to determine if a credit card number entered is a valid number. But how do you do that? Oh with something called the Luhn Algorithm of course! And so today I am going to explain to you about how you can code a hyper duper basic Credit Card Validator in Go — AKA Golang — who uses this algorithm.. Let’s dive in, shall we?
The Sneaky World of Fraud
Picture this: for instance, if you are browsing the internet, eager to purchase a new gadget, and then, you click on the “Pay Now” button, the web gets paid. But wait… what if that credit card number you’ve entered is not valid at all? Not to mention the time that the article was written by a professional but it got lost in the cyber space or worse, taken by a hacker?
Other times, tricksters pinch the credit card details and run tests by electronic systems to know the numbers that work, and make away with the correct ones for giant buys. The sad part? This happens every day, to thousands of ordinary consumers who have no idea that they are being watched.
So, how do we fight back? Oh, that’s simple – we check out credit card numbers before they get used in frauds. And this brings us to the Luhn Algorithm as its potential here shall be considered.
What is Luhn Algorithm and why does it matter?
This may be termed as the Luhn Algorithm, which is actually a sophisticated way of asking the question, “Is this credit card number valid”? It does not identify the fraud or stolen cards itself, but it checks that the card number is correct. If it is not, then it will not pass, well…
Here’s how it works:
Double the numbers in the second column starting from the right column.
If a digit when doubled exceed 9, then the digit should be reduced by 9.
Sum up all the individual digits and if figures summed up is divisible by 10 then
the said credit card number is legitimate.
It is fast, effective and is your first line of security against incidences of fraud on the cyber space.
Building the Credit Card Validator in Go
This is a Credit Card Validator that I’ve created in Go or as I like to call it a piece of cake! The full code is in my GitHub repo. and you can feel free to mess with it as I wished. Oh well, let me make some analization so here we are.
What’s Inside the Project? The project is pretty simple and consists of three parts:
luhn.go
– The Luhn algorithm is a magical abbreviation occurs at this point.main.go
– This file shows how we can start a server in order to respond to HTTP requests./static/index.html
– A static HTML page for the user input using with a very basic front end form.
/credit-card-validator
│
├── main.go
├── luhn.go
└── static/
└── index.html
luhn.go
: Where the Magic Happens
In luhn.go
, you can see this program checking if the card number is valid following the Luhn Algorithm. Oh yes it is! it might sound complicated but its just few lines of codes! Basically, we:
Iterate through each digit in the card number.
Double every second digit beginning from the digit in the rightmost column. When the doubled number is 10 or more, then one should subtract 9.
Sum of everything and check whether the total is a multiple of 10 or not.
It is similar to an instant mental computation of the number to see whether it conforms to certain pattern. You can also find the complete code here.
main.go
: The Heart of the Server
However, we need to inform our application to start listening to the requests and validate card numbers now. That is where main.go
comes in. This file creates a server that answers to POST requests on the /validate endpoint. Whenever the user enters his/her card number, the server checks that number with the help of Luhn Algorithm and then returns the results.
Here’s how it works:
The server listens for a POST request at
http://localhost:8080/validate.
The card number needs to be checked for its validity with the help of Luhn Algorithm and therefore it will be passed to Luhn Algorithm function.
The output is then packaged in a neat JSON response to be sent back to the user.
It’s that simple! The code for this part can be found here.
static/index.html:
The Frontend (Keep It Simple)
On the frontend I went all out simple as shown above. All one has to do is to enter his card number and send it. The form then, submits the card number to the server, and once the next page is rendered the result (valid / invalid entry) is presented on that page only.
Here’s how it works:
Their HTML form is used to capture the card number of the user.
JavaScript has the responsibility of sending the card number to the server.
After getting the response from the server, JavaScript changes the page informing the user whether the card is valid or not.
Simple, right? This is where it is Given the above mentioned equations the code for this part can be seen here.
How to Run the Project
Wish to use it on your local system? Here’s how:
Clone the repo:
git clone https://github.com/yourusername/credit-card-validator.git cd credit-card-validator
Install Go if you haven’t already: Install Go.
Run the app:
go run main.go luhn.go
Open
index.html
: Go to thestatic
folder and openindex.html
in your browser.Test it: Enter a card number in the form and see if it’s valid.
Conclusion: It is just a simple Check, but it Counts
Unfortunately, fraud is all around us – but that doesn't mean that we have to sit idly and watch the scammers ruin it for everyone. Creating the basic credit card validator with Go, and the Luhn Algorithm is a good start to make sure that no wrong card numbers will get into the system.
You can find the full code on my GitHub repo, but here is a glimpse you can modify it or add your own elements to it as you wish. In other words the more one understands security the more secure their users will be!
Be safe, continue coding, and do not forget to check those card numbers!
Also, if you enjoyed this content, please leave a like ❤️! Your feedback is invaluable and encourages me to keep creating more valuable content.
Subscribe to my newsletter
Read articles from Denish directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
