Ownable And Ownable2step: Better Choice?
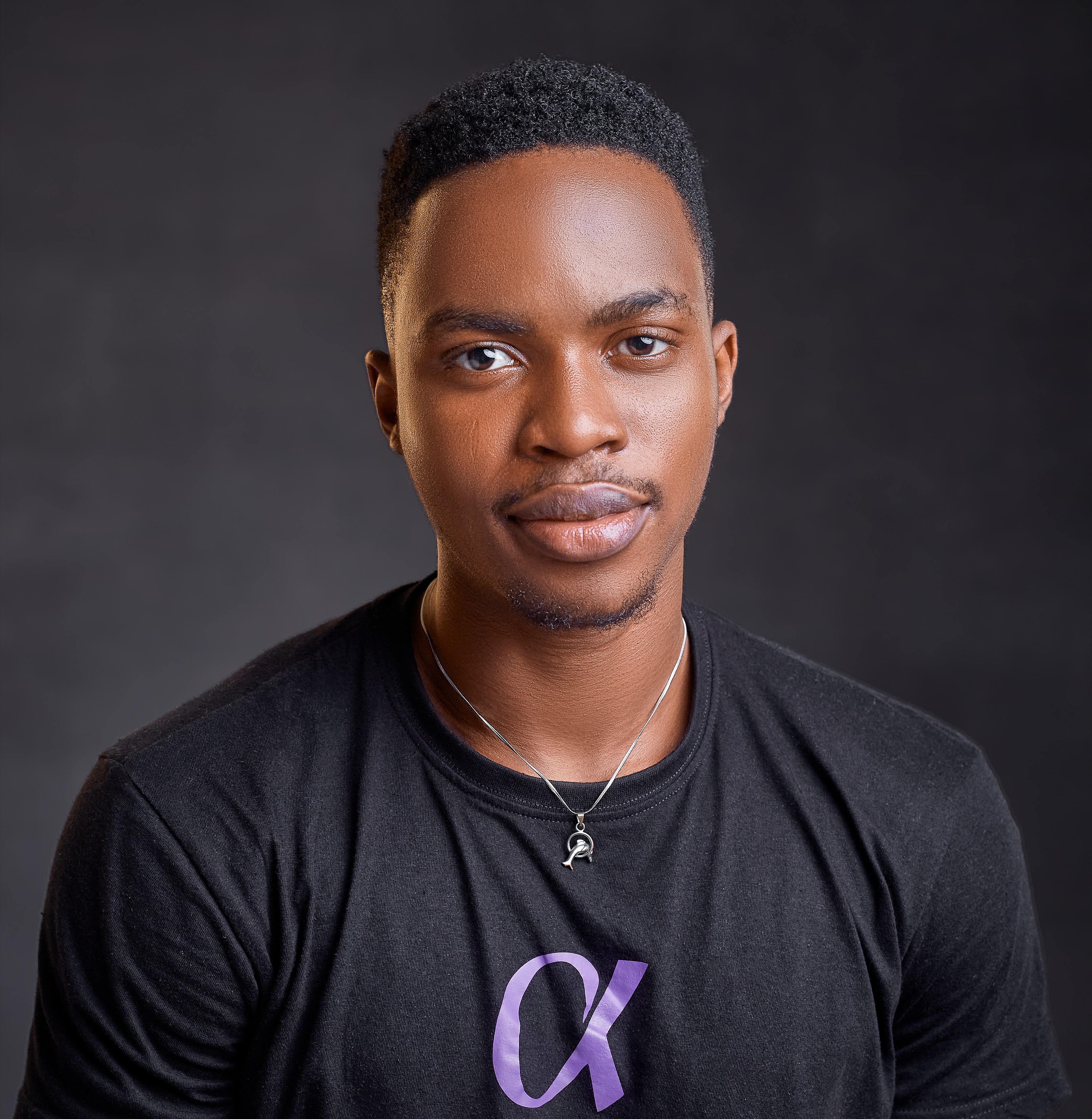
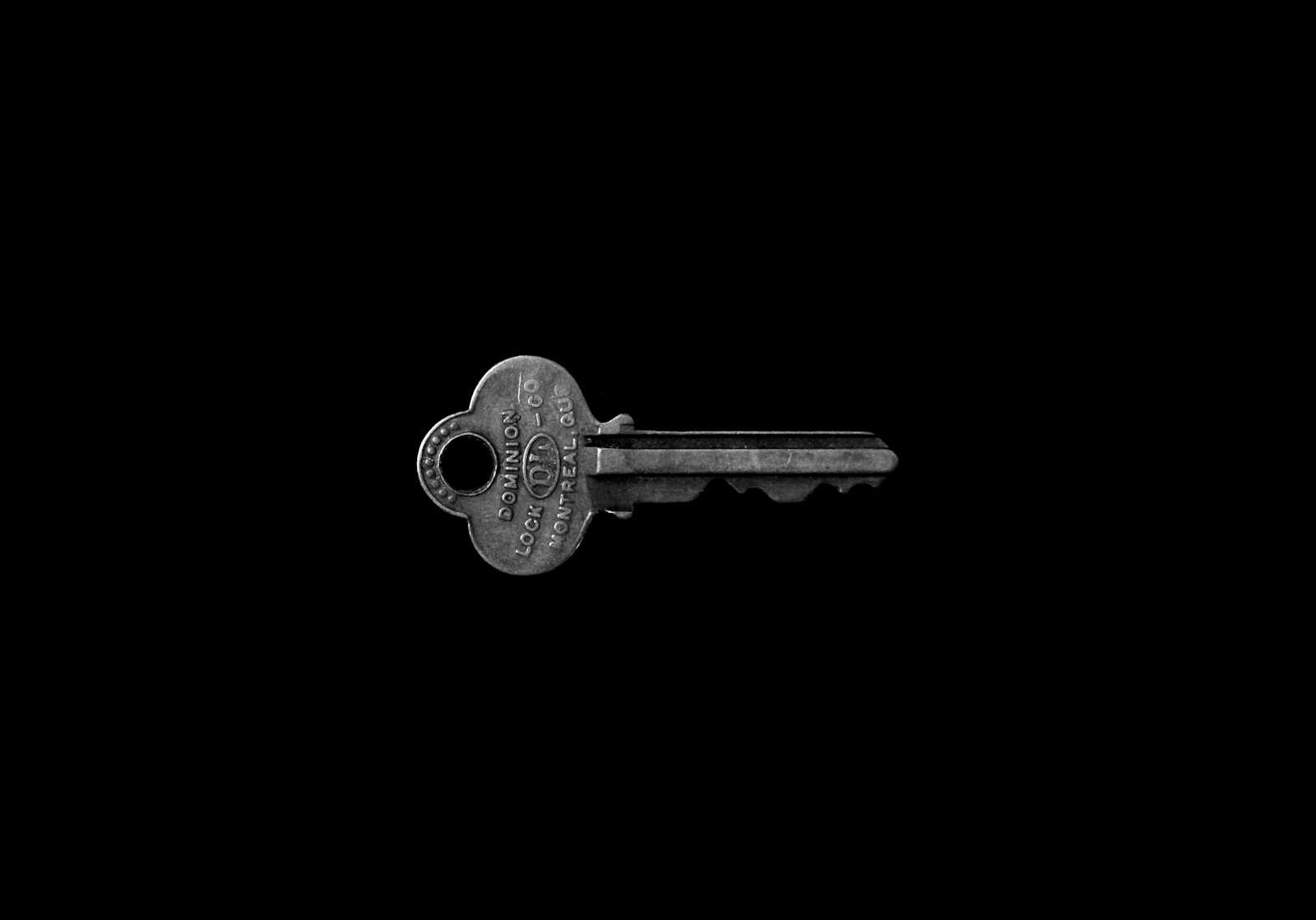
Access control is a crucial aspect of any smart contract; no matter how secure the logic of a contract is, a weak access control will make it appear on Rekt sooner or later.
The OpenZeppelin team came up with the Ownable
library for this reason. Therefore, many Solidity engineers or founding teams do not see the reason to invent the wheel by building their ownership contract from scratch. Moreso, the OZ libraries are widely known to have been well-audited.
But in January 2023, the OZ team announced an improved version of Ownable
called Ownable2Step
. Currently, Solidity engineers are encouraged to use Ownable2Step
over its other counterpart.
This blog post is for both practising Solidity engineers and new ones as it will go explain these two libraries, recommend a better one, and even go ahead to build sizeable contracts to implement them.
What is Ownable
? Explanation and Codebase Walkthrough
Without controversy, the address that deploys a contract is the owner. Practically, there are times when the ownership of a contract will have to be changed.
For instance, if the current owner of the deploying address is leaving the team and someone else needs to be in charge, or similar scenarios.
In such cases, two things need to be done:
previous owner must transfer ownership to the new entity in charge
previous owner must renounce ownership and check out from admin responsibilities
Implementing is simple, only that there may be security nuances that can break the protocol. This is where Ownable
becomes very helpful. It helps with transfer and renunciation of contract ownership.
The codebase must be reviewed for proper insights.
// SPDX-License-Identifier: MIT
// OpenZeppelin Contracts (last updated v5.0.0) (access/Ownable.sol)
pragma solidity ^0.8.20;
import {Context} from "../utils/Context.sol";
/**
* @dev Contract module which provides a basic access control mechanism, where
* there is an account (an owner) that can be granted exclusive access to
* specific functions.
*
* The initial owner is set to the address provided by the deployer. This can
* later be changed with {transferOwnership}.
*
* This module is used through inheritance. It will make available the modifier
* `onlyOwner`, which can be applied to your functions to restrict their use to
* the owner.
*/
abstract contract Ownable is Context {
address private _owner;
/**
* @dev The caller account is not authorized to perform an operation.
*/
error OwnableUnauthorizedAccount(address account);
/**
* @dev The owner is not a valid owner account. (eg. `address(0)`)
*/
error OwnableInvalidOwner(address owner);
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
/**
* @dev Initializes the contract setting the address provided by the deployer as the initial owner.
*/
constructor(address initialOwner) {
if (initialOwner == address(0)) {
revert OwnableInvalidOwner(address(0));
}
_transferOwnership(initialOwner);
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
_checkOwner();
_;
}
/**
* @dev Returns the address of the current owner.
*/
function owner() public view virtual returns (address) {
return _owner;
}
/**
* @dev Throws if the sender is not the owner.
*/
function _checkOwner() internal view virtual {
if (owner() != _msgSender()) {
revert OwnableUnauthorizedAccount(_msgSender());
}
}
/**
* @dev Leaves the contract without owner. It will not be possible to call
* `onlyOwner` functions. Can only be called by the current owner.
*
* NOTE: Renouncing ownership will leave the contract without an owner,
* thereby disabling any functionality that is only available to the owner.
*/
function renounceOwnership() public virtual onlyOwner {
_transferOwnership(address(0));
}
/**
* @dev Transfers ownership of the contract to a new account (`newOwner`).
* Can only be called by the current owner.
*/
function transferOwnership(address newOwner) public virtual onlyOwner {
if (newOwner == address(0)) {
revert OwnableInvalidOwner(address(0));
}
_transferOwnership(newOwner);
}
/**
* @dev Transfers ownership of the contract to a new account (`newOwner`).
* Internal function without access restriction.
*/
function _transferOwnership(address newOwner) internal virtual {
address oldOwner = _owner;
_owner = newOwner;
emit OwnershipTransferred(oldOwner, newOwner);
}
}
It is an abstract contract, which means it cannot run by itself, but must be executed with another smart contract.
In the constructor
, it is the case that at the first instance, the ownership of the contract is transferred to the deploying address; known as the initialOwner
.
transferOwnership
is a public function that enables the ownership of the contract transferred to someone else called newOwner
. Note that it has a check if (newOwner == address(0))
that will bounce an old owner from having ownership access sequel to a successful transfer.
Moving on to renounceOwnership
, it is implemented as the transfer of the ownership to a zero address; which is a dead address.
What is Ownable2Step? How is it different from Ownable?
Ownable2Step
was built as a fix to an assumption in Ownable
. What if a wrong address is mistakenly passed as a parameter for ownership transfer?
Even though this is human error, it should not be taken lightly. Hence, the need for Ownable2Step
.
Ownable2Step
is different from Ownable
due to how ownership transfer is broken into 2 major steps: the ownership must be transferred and will not become effective till the new owner accepts it.
Here is how it gets interesting: only the new owner can accept the ownership invitation.
Having explained that, let’s dive into the actual codebase:
// SPDX-License-Identifier: MIT
// OpenZeppelin Contracts (last updated v5.1.0) (access/Ownable2Step.sol)
pragma solidity ^0.8.20;
import {Ownable} from "./Ownable.sol";
/**
* @dev Contract module which provides access control mechanism, where
* there is an account (an owner) that can be granted exclusive access to
* specific functions.
*
* This extension of the {Ownable} contract includes a two-step mechanism to transfer
* ownership, where the new owner must call {acceptOwnership} in order to replace the
* old one. This can help prevent common mistakes, such as transfers of ownership to
* incorrect accounts, or to contracts that are unable to interact with the
* permission system.
*
* The initial owner is specified at deployment time in the constructor for `Ownable`. This
* can later be changed with {transferOwnership} and {acceptOwnership}.
*
* This module is used through inheritance. It will make available all functions
* from parent (Ownable).
*/
abstract contract Ownable2Step is Ownable {
address private _pendingOwner;
event OwnershipTransferStarted(address indexed previousOwner, address indexed newOwner);
/**
* @dev Returns the address of the pending owner.
*/
function pendingOwner() public view virtual returns (address) {
return _pendingOwner;
}
/**
* @dev Starts the ownership transfer of the contract to a new account. Replaces the pending transfer if there is one.
* Can only be called by the current owner.
*
* Setting `newOwner` to the zero address is allowed; this can be used to cancel an initiated ownership transfer.
*/
function transferOwnership(address newOwner) public virtual override onlyOwner {
_pendingOwner = newOwner;
emit OwnershipTransferStarted(owner(), newOwner);
}
/**
* @dev Transfers ownership of the contract to a new account (`newOwner`) and deletes any pending owner.
* Internal function without access restriction.
*/
function _transferOwnership(address newOwner) internal virtual override {
delete _pendingOwner;
super._transferOwnership(newOwner);
}
/**
* @dev The new owner accepts the ownership transfer.
*/
function acceptOwnership() public virtual {
address sender = _msgSender();
if (pendingOwner() != sender) {
revert OwnableUnauthorizedAccount(sender);
}
_transferOwnership(sender);
}
}
First of all, you can notice that there is no function like renounceOwnership
. This is simply because Ownable2Step
is an improvement on—and still uses—the Ownable
codebase.
That said, here is the flow:
the
initialOwner
transfers ownership to thenewOwner
the
newOwner
, at this point, becomes thependingOwner
the
pendingOwner
becomes a bona fidenewOwner
after interacting with theacceptOwnership
function
How to Implement Ownable and Ownable2Step in a Contract
Implementing these libraries is simple, and once you know how to implement Ownable2Step
, you can implement the other one. Here is an example:
// SPDX-License-Identifier: UNLICENSED
pragma solidity ^0.8.13;
import "@openzeppelin/contracts/access/Ownable2Step.sol";
contract Counter is Ownable2Step{
address public commander;
constructor() Ownable(msg.sender) {
_transferOwnership(msg.sender);
}
uint256 public number;
function setNumber(uint256 newNumber) public {
number = newNumber;
}
function increment() public {
number++;
}
function transferOwnership (address newOwner) public override onlyOwner {
_transferOwnership(newOwner);
}
function acceptOwnership() public override{
address accepter = _msgSender();
_transferOwnership(accepter);
}
}
Generally, I think the only place you might want to have issue is the constructor
if you don’t do it properly. But if you follow it as implemented above, you are good to go.
Which one is a better choice between Ownable
and Ownable2Step
?
Clearly, Ownable2Step
is a better access control library than Ownable
as it has an authentication method to ensure that:
only the intended
newOwner
accepts the ownershipwhen mistakenly done to an unintended address, the initial owner can quickly create new one.
To a large extent, Ownable2Step eliminates the human errors that can occur during contract ownership transfer.
If you enjoyed reading this, I’ll encourage you to follow me on Twitter!
Subscribe to my newsletter
Read articles from John Fáwọlé directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
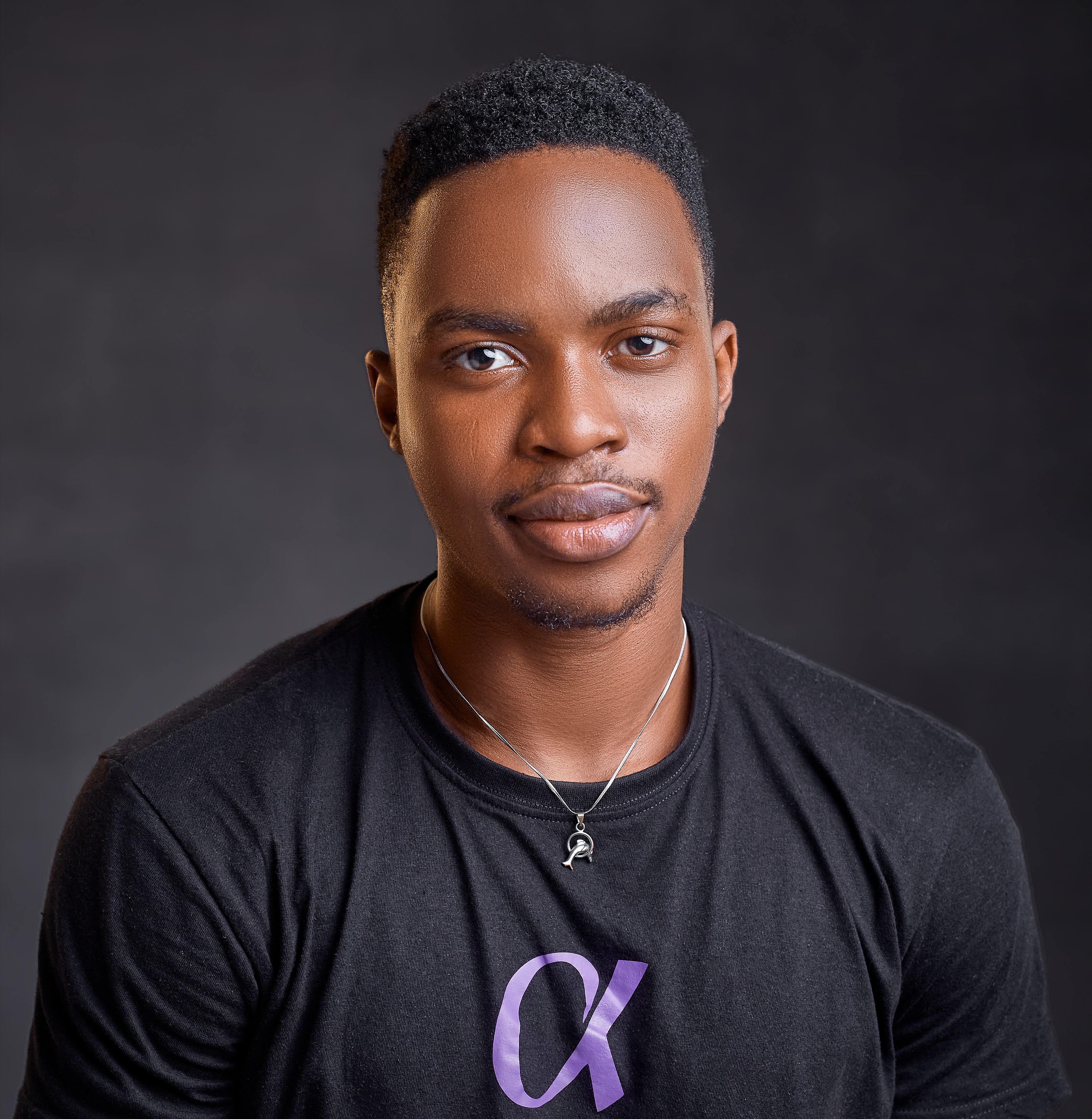
John Fáwọlé
John Fáwọlé
Web3 Technical Writer | Content Lead | Marketing Strategist | For Devs