Flutter Text Widget: A Comprehensive Guide with Examples
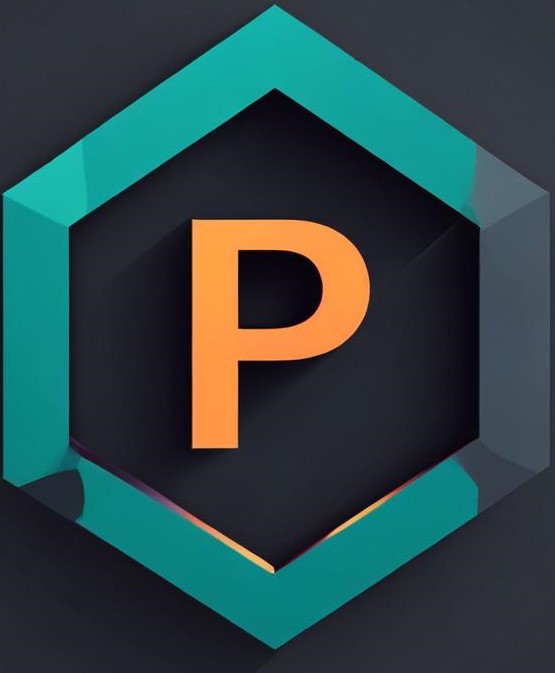
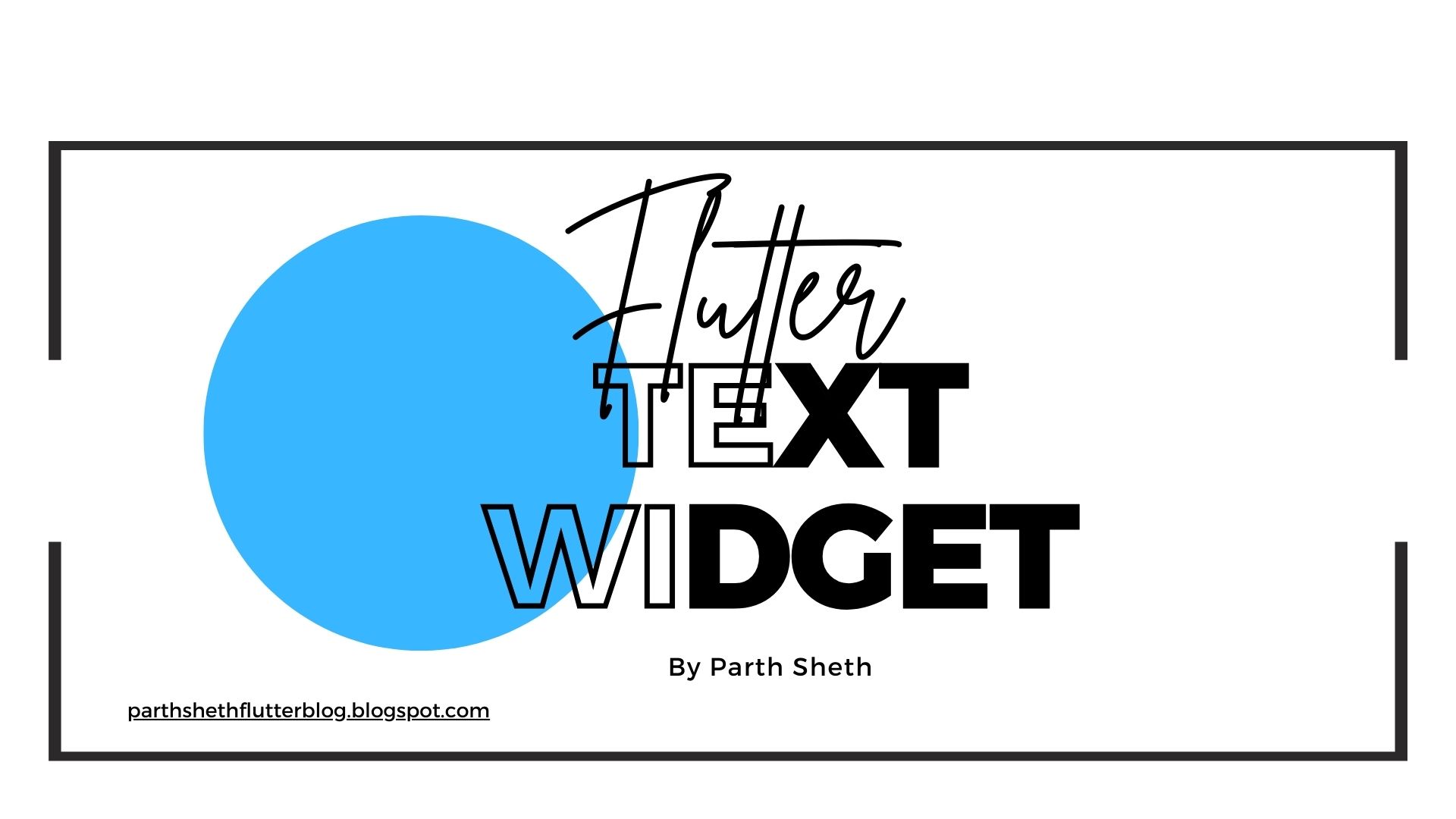
One of the most commonly used widgets in Flutter is the Text
widget. It's used to display a string of text on the screen, and it provides a wide range of customization options to suit various design needs.
What is the Flutter Text Widget?
The Text
widget is a simple yet essential widget in Flutter that allows developers to display text content in their applications. It is highly customizable, enabling you to adjust text style, alignment, overflow handling, and much more.
Here’s the basic syntax of the Text
widget:
Text(
'Your text goes here',
style: TextStyle(fontSize: 20, color: Colors.black),
)
In this simple example, the Text
widget is displaying the string "Your text goes here" with a font size of 20 and a color of black.
Basic Example: Displaying a Simple Text
Let's look at a basic example where we display a simple string using the Text
widget.
Text(
'Hello, Flutter!',
style: TextStyle(
fontSize: 30,
color: Colors.blue,
fontWeight: FontWeight.bold,
),
),
Explanation:
Text
: This is the widget that will display the text.style
: TheTextStyle
property allows us to customize the text (font size, color, weight, etc.).fontSize: 30
: Sets the size of the text.color:
Colors.blue
: Changes the color of the text to blue.fontWeight: FontWeight.bold
: Makes the text bold.
Styling the Text Widget
Flutter provides a powerful TextStyle
class to style text. You can modify the text in a variety of ways such as changing the font size, color, weight, letter spacing, line height, and even apply custom fonts.
Example: Customizing Text Style
Text(
'Flutter is Awesome!',
style: TextStyle(
fontSize: 25,
fontWeight: FontWeight.w600,
color: Colors.green,
letterSpacing: 1.5,
height: 1.5,
),
)
Explanation:
fontSize: Controls the size of the text.
fontWeight: Changes the weight of the text.
FontWeight.w600
is a medium-bold weight.color: Specifies the color of the text. In this case, it’s set to green.
letterSpacing: Adds space between the characters. In this example, the spacing is set to 1.5.
height: Sets the height of each line of text, effectively controlling the line spacing.
Handling Text Overflow
When text exceeds the space available, it can be clipped, ellipsized, or wrapped. Flutter provides several properties to handle text overflow:
- overflow: This property determines how the text should behave when it doesn't fit within the available space. You can choose from
TextOverflow.ellipsis
,TextOverflow.fade
, orTextOverflow.clip
.
Example: Text Overflow Handling
Text(
'This is a very long text that might overflow in a limited space',
overflow: TextOverflow.ellipsis,
style: TextStyle(fontSize: 18),
)
Explanation:
TextOverflow.ellipsis
: Adds "..." at the end of the text when it overflows.TextOverflow.fade
: Fades the text when it overflows.TextOverflow.clip
: Simply clips the text at the boundary.
Aligning the Text
Flutter allows you to align the text in different ways using the textAlign
property. You can align the text to the left, center, right, or justify it within the widget.
Example: Text Alignment
Text(
'Aligned Text Example',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 22),
)
Explanation:
In this example, the text will be centered within the available space. Other options for textAlign
include:
TextAlign.left
: Aligns text to the left.TextAlign.right
: Aligns text to the right.TextAlign.justify
: Justifies the text, meaning it stretches the lines to fill the space.
Multiline Text
By default, the Text
widget handles multiline text automatically, breaking the text into lines as needed. If you have long text, you may want to make sure it wraps correctly.
Example: Multiline Text
Text(
'This is a long text that will wrap into multiple lines if necessary.',
style: TextStyle(fontSize: 18),
softWrap: true, // This ensures the text wraps to the next line.
)
Explanation:
softWrap
: When set totrue
, text will wrap to the next line if it exceeds the container width.softWrap
: When set totrue
, text will wrap to the next line if it exceeds the container width.
Rich Text and Multiple Styles
Flutter allows you to use RichText
for displaying text with multiple styles in the same widget. This is useful if you want to style parts of your text differently.
Example: Rich Text with Multiple Styles
RichText(
text: TextSpan(
text: 'Hello, ',
style: TextStyle(color: Colors.black, fontSize: 20),
children: <TextSpan>[
TextSpan(
text: 'Flutter!',
style: TextStyle(color: Colors.blue, fontSize: 25, fontWeight: FontWeight.bold),
),
],
),
)
In this example, "Hello" will be displayed in black, and "Flutter!" will be displayed in blue and bold.
Conclusion
The Text
widget in Flutter is an incredibly versatile tool for displaying and customizing text. From simple static text to rich, multiline, and overflow-handled text, you can easily tweak the properties to achieve the desired result.
Flutter's extensive TextStyle
class and properties like textAlign
, overflow
, and softWrap
give developers full control over how text looks and behaves. With the ability to apply different fonts, colors, and styles, you can create a polished and user-friendly UI that suits your app's needs.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Parth Sheth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
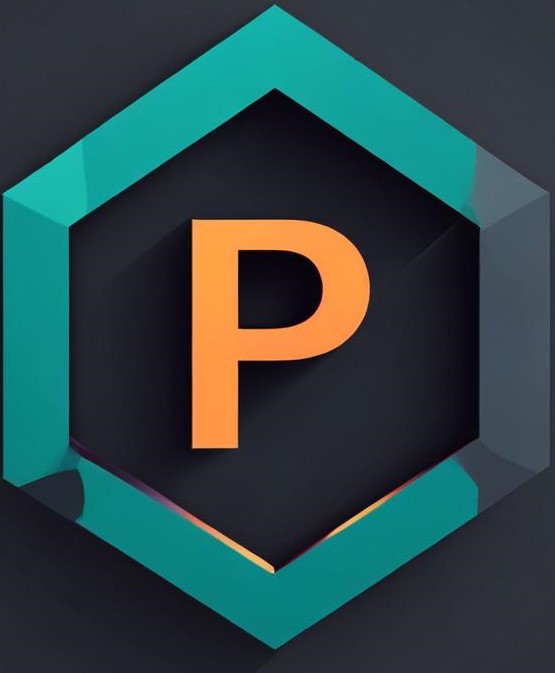
Parth Sheth
Parth Sheth
"🚀 Fluttering through the digital cosmos, I am Parth Sheth, a passionate architect of interactive marvels in the realm of Flutter development. With a fervent love for coding and an insatiable curiosity for the ever-evolving tech landscape, I embark on a perpetual quest to redefine the boundaries of innovation. From sleek UI designs to seamless user experiences, I orchestrate symphonies of code that resonate with elegance and functionality. 💻✨ My journey in Flutter is more than just a profession; it's a thrilling odyssey where creativity meets craftsmanship. Armed with a keen eye for detail and an arsenal of cutting-edge tools, I navigate the complexities of app development with finesse, crafting solutions that not only meet but exceed expectations. 🎨🔧 But beyond the lines of code lies a deeper commitment—to inspire, empower, and elevate. Through my words, my work, and my unwavering dedication to excellence, I strive to ignite the spark of imagination in others, fostering a community where innovation knows no bounds. 🌟💡 So come, fellow adventurers, and join me on this exhilarating expedition into the unknown. Together, let's sculpt the future, one pixel at a time, and unleash the full potential of Flutter's boundless possibilities. Connect with me, and let's embark on this extraordinary voyage—together, we shall chart new horizons and redefine what's possible in the digital landscape. 🌍🔍"