Building Go Applications for macOS, Linux, and Windows: A Beginner’s Guide

Table of contents
- Why Build for Multiple Platforms?
- Step 1: Prepare Your Environment
- Step 2: Write Your Go Program
- Step 3: Build for Your Current Platform
- Step 4: Build for Other Platforms
- Example: Build for macOS, Linux, and Windows
- Step 5: Verify Your Builds
- Step 6: Automate Cross-Platform Builds (Optional)
- Common GOOS and GOARCH Values
- Key Points to Remember
- Conclusion
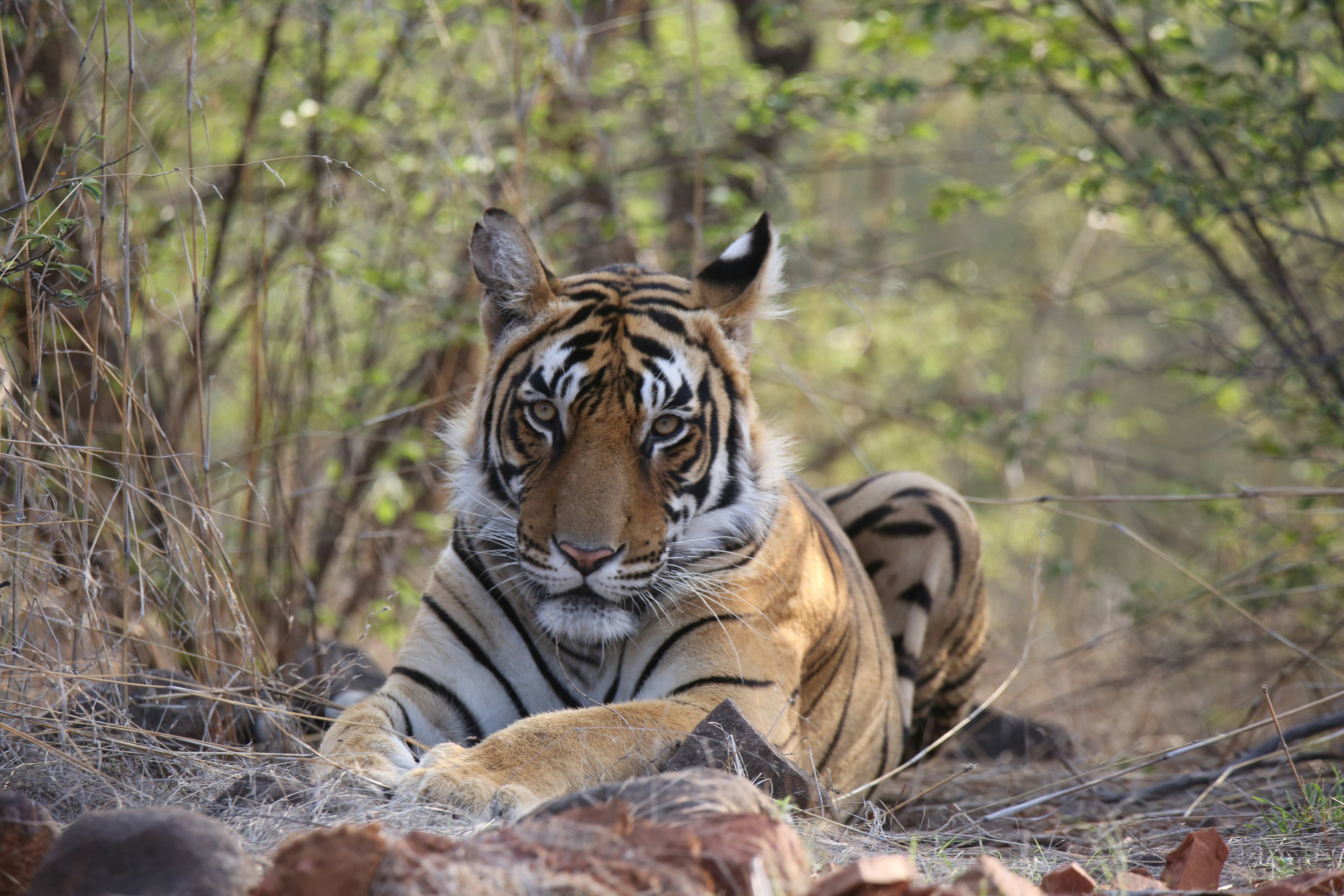
Go (Golang) makes it easy to create cross-platform applications. With Go’s built-in tools, you can compile your program to run on different operating systems like macOS, Linux, and Windows—all from a single codebase.
This guide will walk you through building Go applications for macOS, Linux, and Windows step by step.
Why Build for Multiple Platforms?
If you want your program to reach a broader audience, you’ll often need to provide versions for various operating systems. For example:
macOS users might need a
.dmg
or a simple executable.Linux users usually prefer binary files or
.deb
packages.Windows users often require
.exe
files.
Step 1: Prepare Your Environment
Before building for multiple platforms, ensure you have Go installed and set up correctly on your system.
Check Go Installation:
Run this command to verify your Go installation:go version
You should see output like:
go version go1.20.5 linux/amd64
Set Your Go Workspace:
Ensure yourGOPATH
andGOROOT
are set up if you're using older Go versions. Newer Go versions handle this automatically.
Step 2: Write Your Go Program
Create a simple Go program that you want to build. For this example, let’s write a basic "Hello, World!" program.
Example Code
package main
import (
"fmt"
)
func main() {
fmt.Println("Hello, World!")
}
Save this file as main.go
.
Step 3: Build for Your Current Platform
Before diving into cross-platform builds, compile your program for your current operating system.
Command
go build main.go
Result
For macOS/Linux: Creates a binary file named
main
(no extension).For Windows: Creates a binary file named
main.exe
.
Run the Program
./main # macOS/Linux
main.exe # Windows
Step 4: Build for Other Platforms
Go’s compiler supports cross-compilation, meaning you can build executables for any supported operating system and architecture from your current system.
Cross-Compilation Syntax
Use the GOOS
and GOARCH
environment variables to specify the target platform and architecture.
GOOS=<target-os> GOARCH=<target-arch> go build main.go
Example: Build for macOS, Linux, and Windows
Here’s how you can build for each platform:
Build for macOS
GOOS=darwin GOARCH=amd64 go build -o hello_mac main.go
GOOS=darwin
: Specifies macOS.GOARCH=amd64
: Specifies a 64-bit architecture.-o hello_mac
: Names the output file ashello_mac
.
Build for Linux
GOOS=linux GOARCH=amd64 go build -o hello_linux main.go
GOOS=linux
: Specifies Linux.Output: Creates a binary file
hello_linux
.
Build for Windows
GOOS=windows GOARCH=amd64 go build -o hello_windows.exe main.go
GOOS=windows
: Specifies Windows.Output: Creates an
.exe
file namedhello_windows.exe
.
Step 5: Verify Your Builds
Once you’ve built the executables, you can test them on their respective platforms:
macOS/Linux: Copy the binary and run it using the terminal:
./hello_mac # macOS ./hello_linux # Linux
Windows: Transfer the
.exe
file to a Windows machine and double-click it to execute.
Step 6: Automate Cross-Platform Builds (Optional)
If you frequently build for multiple platforms, you can automate the process using a script.
Bash Script for Automation
Create a file named build.sh
:
#!/bin/bash
echo "Building for macOS..."
GOOS=darwin GOARCH=amd64 go build -o hello_mac main.go
echo "Building for Linux..."
GOOS=linux GOARCH=amd64 go build -o hello_linux main.go
echo "Building for Windows..."
GOOS=windows GOARCH=amd64 go build -o hello_windows.exe main.go
echo "All builds completed!"
Make the script executable:
chmod +x build.sh
Run the script:
./build.sh
Common GOOS and GOARCH Values
Platform | GOOS | GOARCH |
macOS | darwin | amd64 |
Linux | linux | amd64 |
Windows | windows | amd64 |
ARM | linux | arm |
Key Points to Remember
Cross-compilation is built-in: Go makes it simple to build for other platforms using
GOOS
andGOARCH
.Output naming: Use the
-o
flag to specify meaningful file names for each platform.Testing: Always test your builds on the target platform to ensure compatibility.
File permissions: On Linux/macOS, set executable permissions with
chmod +x
.
Conclusion
Go’s ability to cross-compile for multiple platforms is a powerful feature that helps developers create applications for diverse users. By following this guide, you can confidently build Go programs for macOS, Linux, and Windows.
Stay tuned for more tutorials to help you master Go! 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
