Promises in JavaScript: Your Key to Handling Asynchronous Operations
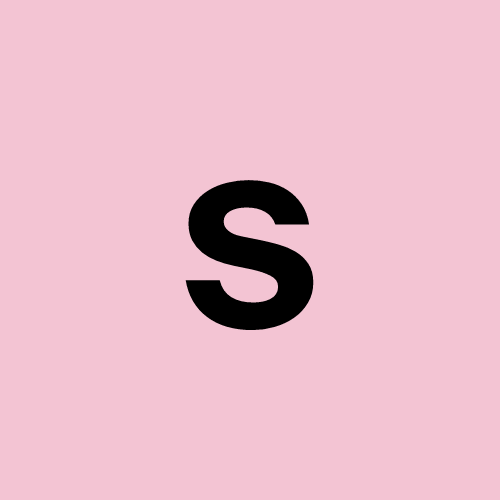
Asynchronous programming is an essential aspect of modern JavaScript, allowing developers to perform tasks like data fetching, file reading, and timer functions without blocking the execution of other code. At the heart of this paradigm are promises, which provide a cleaner and more manageable way to handle asynchronous operations compared to traditional callback methods.
What is a Promise?
A promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. When you create a promise, it can be in one of three states:
Pending: The initial state, meaning the operation has not yet completed.
Fulfilled: The operation completed successfully, resulting in a resolved value.
Rejected: The operation failed, resulting in an error.
This structure allows developers to write cleaner code that is easier to read and maintain.
How Promises Work
To create a promise, you use the Promise
constructor, which takes an executor function with two parameters: resolve
and reject
. Here’s a simple example:
const myPromise = new Promise((resolve, reject) => {
// Simulating an asynchronous operation
setTimeout(() => {
const success = true; // Change this to false to simulate an error
if (success) {
resolve("Operation succeeded!");
} else {
reject("Operation failed.");
}
}, 1000);
});
In this example, after one second, the promise will either resolve with a success message or reject with an error message.
Using Promises
To handle the result of a promise, you use the .then()
method for fulfilled promises and .catch()
for rejected ones:
myPromise
.then(result => {
console.log(result); // Logs "Operation succeeded!"
})
.catch(error => {
console.error(error); // Logs "Operation failed."
});
Chaining Promises
One of the most powerful features of promises is the ability to chain them. This allows you to perform multiple asynchronous operations in sequence without getting trapped in "callback hell." For example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
return processData(data); // Assume processData returns a promise
})
.then(finalResult => {
console.log(finalResult);
})
.catch(error => {
console.error("Error:", error);
});
Combining Multiple Promises
JavaScript also provides several utility methods for handling multiple promises:
Promise.all()
: Waits for all promises to fulfill or any to reject.Promise.race()
: Resolves or rejects as soon as one of the promises resolves or rejects.Promise.allSettled()
: Waits for all promises to settle (each may fulfill or reject).Promise.any()
: Resolves when any of the promises fulfill; rejects if all fail.
Conclusion
Promises are a fundamental part of asynchronous programming in JavaScript, providing a powerful way to manage operations that take time to complete. By using promises, developers can write more readable and maintainable code while effectively handling asynchronous tasks. Companies like Hexadecimal are leveraging these technologies to enhance their applications and streamline workflows, demonstrating the real-world impact of mastering promises in JavaScript.
Subscribe to my newsletter
Read articles from seo2 directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
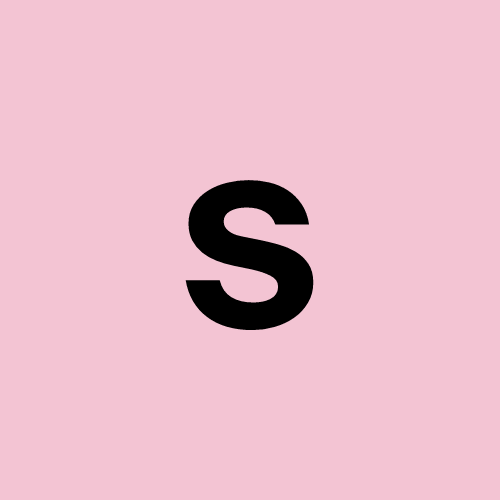