Real-Time Applications with WebSockets and Socket.io

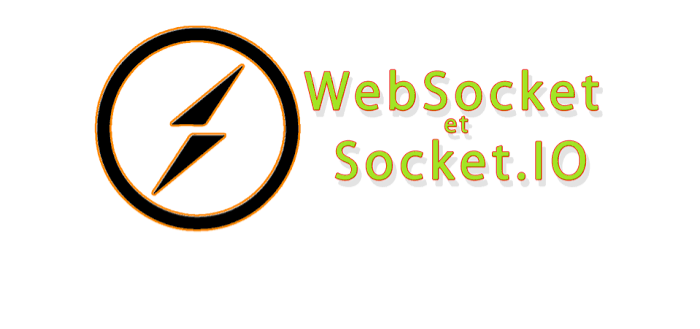
In the age of instantaneous communication and live updates, real-time applications have become an integral part of modern web development. From chat applications and collaborative tools to live sports updates and multiplayer games, real-time functionality is shaping user experiences. One of the core technologies behind these innovations is WebSockets, often used in conjunction with libraries like Socket.io for enhanced functionality and ease of use.
In this blog, we'll explore what WebSockets are, why they matter, and how Socket.io simplifies building robust real-time applications.
What Are WebSockets?
WebSockets are a communication protocol that provides full-duplex communication channels over a single TCP connection. Unlike traditional HTTP, which follows a request-response model, WebSockets enable a persistent connection between the client and the server. This means both parties can send and receive messages in real-time without the need to re-establish a connection.
How WebSockets Work
Handshake: The client sends an HTTP request to upgrade the connection to a WebSocket.
Persistent Connection: Once established, the WebSocket connection remains open, allowing for continuous communication.
Real-Time Data Exchange: Both the client and server can send data at any time, reducing latency and overhead.
Key Advantages of WebSockets
Low Latency: Real-time data exchange with minimal delay.
Efficiency: Reduces the overhead of repeated HTTP requests.
Bidirectional Communication: Both client and server can actively push data.
Socket.io: Enhancing WebSockets
While WebSockets are powerful, implementing them from scratch can be challenging. This is where Socket.io comes in. It is a JavaScript library that simplifies real-time communication by building on top of WebSockets, offering additional features such as:
Automatic reconnection
Broadcasting events
Room-based communication
Fallback to other protocols when WebSockets are unavailable (e.g., long polling)
How Socket.io Works
Socket.io uses a combination of WebSockets and other transport mechanisms to ensure compatibility across a wide range of browsers and network conditions. It consists of:
Socket.io Server: A Node.js server-side library that handles communication.
Socket.io Client: A JavaScript library for the browser that interacts with the server.
Building a Real-Time Application with WebSockets and Socket.io
Let’s walk through an example of creating a simple chat application using WebSockets and Socket.io.
Step 1: Set Up the Server
Install the necessary dependencies:
npm install express socket.io
Create a basic server (server.js
):
const express = require('express');
const http = require('http');
const { Server } = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = new Server(server);
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
// Handle connection events
io.on('connection', (socket) => {
console.log('A user connected');
// Listen for messages
socket.on('chat message', (msg) => {
io.emit('chat message', msg); // Broadcast message
});
// Handle disconnection
socket.on('disconnect', () => {
console.log('A user disconnected');
});
});
server.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Step 2: Create the Client
Create a simple HTML file (index.html
):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Chat App</title>
</head>
<body>
<ul id="messages"></ul>
<form id="form">
<input id="input" autocomplete="off" /><button>Send</button>
</form>
<script src="/socket.io/socket.io.js"></script>
<script>
const socket = io();
const form = document.getElementById('form');
const input = document.getElementById('input');
form.addEventListener('submit', (e) => {
e.preventDefault();
if (input.value) {
socket.emit('chat message', input.value);
input.value = '';
}
});
socket.on('chat message', (msg) => {
const item = document.createElement('li');
item.textContent = msg;
document.getElementById('messages').appendChild(item);
});
</script>
</body>
</html>
Step 3: Run the Application
Start the server:
node server.js
Navigate to http://localhost:3000
in your browser, and you’ll see a basic chat application in action!
Use Cases for Real-Time Applications
Chat Applications: WhatsApp, Slack, and similar platforms rely heavily on real-time messaging.
Collaborative Tools: Applications like Google Docs use WebSockets for live collaboration.
Live Feeds: Social media platforms display real-time updates using WebSockets.
Gaming: Multiplayer online games depend on low-latency, bidirectional communication.
IoT: Devices continuously communicate with servers using WebSockets to update status in real-time.
Best Practices for WebSockets and Socket.io
Authentication: Use tokens or other mechanisms to secure connections.
Scaling: Use tools like Redis to manage WebSocket connections across multiple servers.
Fallbacks: Ensure compatibility with older browsers by leveraging Socket.io’s fallback mechanisms.
Error Handling: Implement proper error-handling logic to manage disconnections and reconnections seamlessly.
Conclusion
WebSockets and Socket.io have revolutionized the way developers build real-time applications, offering low-latency and efficient communication. Whether you’re building a chat app, a live dashboard, or a collaborative tool, understanding these technologies is crucial for creating responsive, engaging user experiences.
By leveraging the power of WebSockets and the simplicity of Socket.io, developers can focus on innovation while delivering robust real-time solutions. Ready to take your applications to the next level? Start experimenting with WebSockets and Socket.io today!
Support My Work
If you found this guide on DevOps helpful and would like to support my work, consider buying me a coffee! Your support helps me continue creating beginner-friendly content and keeping it up-to-date with the latest in tech. Click the link below to show your appreciation:
I appreciate your support and happy learning! ☕
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.