Control Mutability in Your TypeScript Applications

1 min read
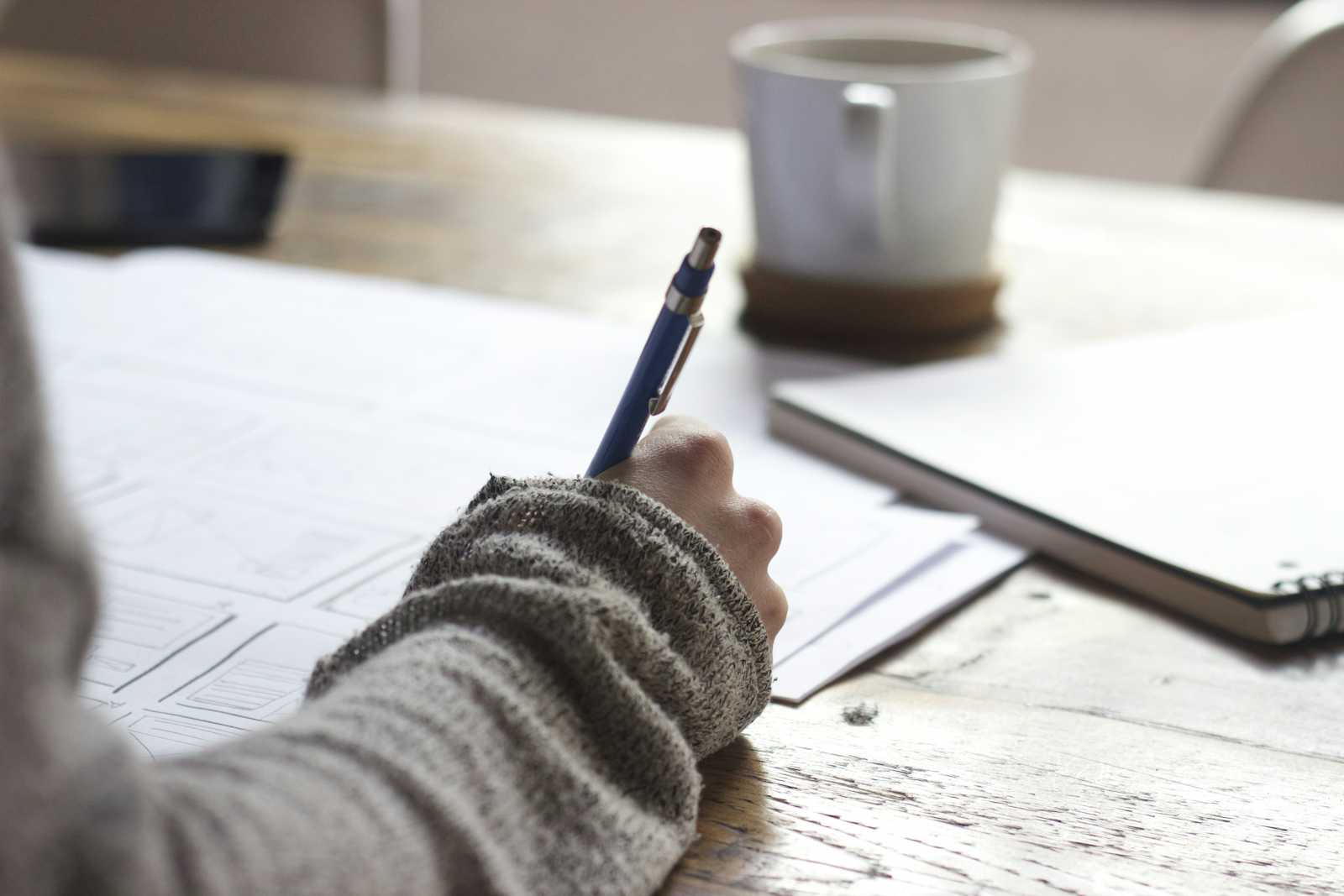
In TypeScript, mutability can be controlled using keywords like readonly
the as const
assertion. These tools allow you to control whether a variable, property, or value can be reassigned or modified, ensuring immutability where needed.
These keywords can prevent accidental mutations to any properties on an object.
type UserSchema = {
readonly id: number;
name: string;
phone: string;
...
}
const user: UserSchema = {
id: 10,
name:"John",
phone:"999"
}
user.name = "Doe"; // you can change name
user.id = 1; // TS error since id is readonly
You can also use Readonly<T>
type helper to make all properties on an object read-only.
type Config = {
url: string;
port: number;
env: string
}
type ReadonlyConfig = Readonly<Config>; // hover on ReadonlyConfig to see that
// all properties are readonly.
When you want to make literal values immutable, you can use as const
assertion.
// making array literal as immutable
const roles = ["admin","user","guest"] as const;
roles[0] = "xyz"; // TS error
// making object literal as immutable
const theme = {
primary:"#000",
onPrimary:"#FFF"
} as const;
theme.primary = "#CCC"; // TS error: Cannot assign to 'primary' because it is a read-only property.
These were some of the examples of how you could utilize read-only and as const
assertion in your TypeScript app.
0
Subscribe to my newsletter
Read articles from Jawad Vajeeh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
