In-Depth Guide for Interview Questions on Generics in Java with Programming Examples.
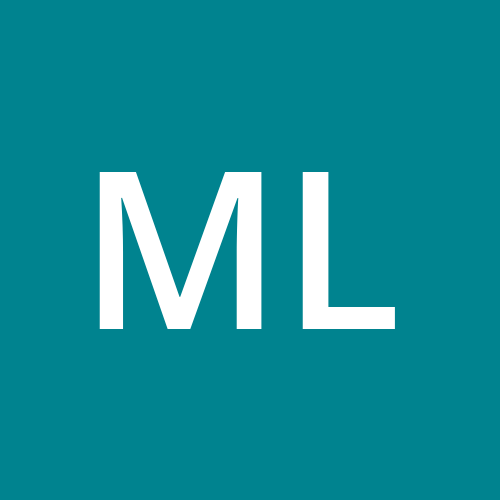
Table of contents
- 1. What Are Generics in Java?
- 2. Interview Question: What is the Purpose of Wildcards (?) in Generics?
- 3. Interview Question: What is the Difference Between T[] and List<T>?
- 4. Interview Question: What is Type Erasure in Java Generics?
- 5. Interview Question: Can You Use Primitive Types with Generics?
- 6. Interview Question: What is the Difference Between Comparable and Comparator Interfaces?
- 7. Interview Question: Can You Create a Generic Class with Multiple Type Parameters?
- Conclusion
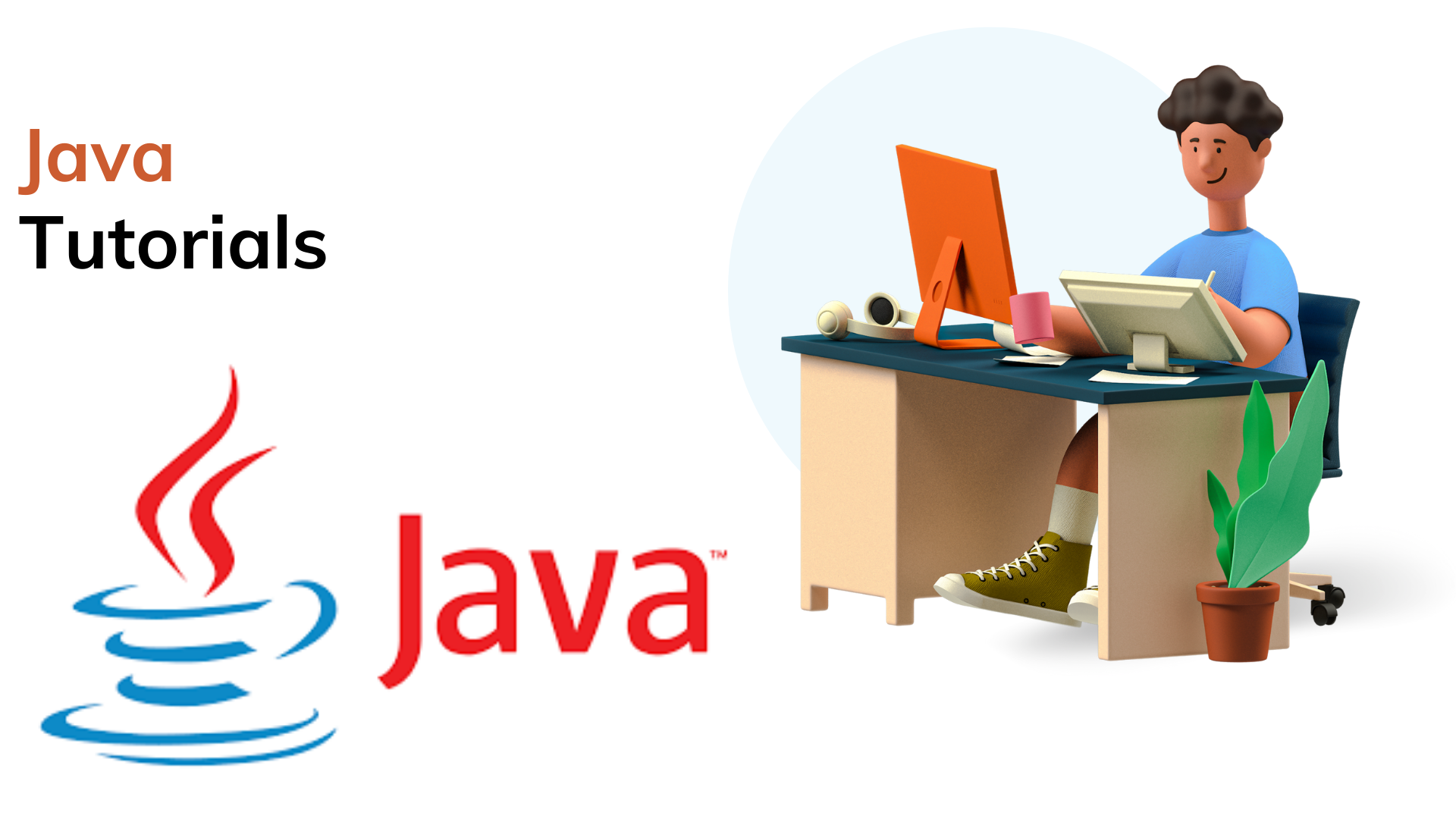
Generics in Java are a powerful feature that allows you to write more flexible, reusable, and type-safe code. Understanding generics is essential for both Java developers and for cracking interviews, as they are a common topic in technical discussions. In this guide, we'll dive deep into Java generics, explain important concepts, and provide practical examples of interview questions along with solutions.
1. What Are Generics in Java?
Generics provide a way to define classes, interfaces, and methods with a placeholder for types, enabling stronger type checks at compile-time. With generics, you can write a single class or method that works with any type, while maintaining type safety.
Example: Generic Method
public class GenericExample {
// A generic method that prints any type of array
public static <T> void printArray(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
public static void main(String[] args) {
Integer[] intArray = {1, 2, 3};
String[] strArray = {"Hello", "World"};
printArray(intArray); // prints Integer array
printArray(strArray); // prints String array
}
}
Explanation: The method printArray
is generic. The placeholder <T>
represents any type, so it works for both Integer[]
and String[]
.
2. Interview Question: What is the Purpose of Wildcards (?
) in Generics?
Wildcards in generics allow you to use unknown types in place of a type parameter. They are especially useful when you want to accept a broad range of types but don’t need to know the specific type.
Types of Wildcards:
Unbounded Wildcard (
?
): Matches any type.Upper Bounded Wildcard (
? extends T
): Matches any type that is a subtype ofT
.Lower Bounded Wildcard (
? super T
): Matches any type that is a supertype ofT
.
Example: Using Wildcards
import java.util.List;
public class WildcardExample {
// A method that accepts a list of any subtype of Number
public static void printNumbers(List<? extends Number> numbers) {
for (Number num : numbers) {
System.out.println(num);
}
}
public static void main(String[] args) {
List<Integer> integers = List.of(1, 2, 3);
List<Double> doubles = List.of(1.1, 2.2, 3.3);
printNumbers(integers); // prints Integer list
printNumbers(doubles); // prints Double list
}
}
Explanation: The method printNumbers
accepts a list of any type that extends Number
, such as Integer
, Double
, etc.
3. Interview Question: What is the Difference Between T[]
and List<T>
?
This question helps interviewers test your understanding of generics and collections in Java.
T[]
is an array of typeT
, which is a fixed-size structure that can hold elements of a single type.List<T>
is a more flexible collection that allows dynamic resizing, offers more functionality, and is part of the Java Collections Framework.
Example: Array vs List
import java.util.ArrayList;
import java.util.List;
public class ArrayListVsArray {
public static void main(String[] args) {
Integer[] intArray = new Integer[5];
List<Integer> intList = new ArrayList<>();
intArray[0] = 10; // Working with an array
intList.add(10); // Working with a List
System.out.println("Array Element: " + intArray[0]);
System.out.println("List Element: " + intList.get(0));
}
}
Explanation: Arrays have a fixed size and work with primitive types, while lists offer dynamic resizing and are part of the java.util
package.
4. Interview Question: What is Type Erasure in Java Generics?
Type erasure is a process by which the Java compiler removes all generic type information after the code is compiled, leaving only the raw types in the bytecode. This ensures backward compatibility with older versions of Java, which do not support generics.
Example: Type Erasure in Action
public class TypeErasureExample<T> {
public void printType(T value) {
System.out.println("Value: " + value);
}
public static void main(String[] args) {
TypeErasureExample<String> obj1 = new TypeErasureExample<>();
obj1.printType("Hello");
TypeErasureExample<Integer> obj2 = new TypeErasureExample<>();
obj2.printType(123);
}
}
Explanation: At runtime, the type T
is erased, and the methods work on raw types (in this case, Object
). The type parameter T
is only used during compilation for type checking.
5. Interview Question: Can You Use Primitive Types with Generics?
No, Java generics cannot directly work with primitive types like int
, char
, etc. You have to use their corresponding wrapper classes (Integer
, Character
, etc.).
Example: Using Wrapper Classes
public class GenericPrimitiveExample {
public static <T> void printElement(T element) {
System.out.println("Element: " + element);
}
public static void main(String[] args) {
printElement(10); // using Integer as a generic argument
printElement(3.14); // using Double as a generic argument
}
}
Explanation: In this example, 10
is auto-boxed into an Integer
, and 3.14
is auto-boxed into a Double
.
6. Interview Question: What is the Difference Between Comparable
and Comparator
Interfaces?
Comparable
is used to define the natural ordering of objects of a class by implementing thecompareTo
method.Comparator
is used to define custom ordering by implementing thecompare
method, and can be passed as a parameter to methods that require sorting.
Example: Using Comparable
and Comparator
import java.util.*;
class Student implements Comparable<Student> {
String name;
int age;
Student(String name, int age) {
this.name = name;
this.age = age;
}
// Implementing compareTo method for natural ordering by age
public int compareTo(Student other) {
return Integer.compare(this.age, other.age);
}
public String toString() {
return name + ": " + age;
}
}
class AgeComparator implements Comparator<Student> {
public int compare(Student s1, Student s2) {
return Integer.compare(s1.age, s2.age);
}
}
public class ComparatorExample {
public static void main(String[] args) {
List<Student> students = Arrays.asList(new Student("Alice", 22), new Student("Bob", 20));
Collections.sort(students); // Sorting using Comparable
System.out.println("Sorted by Comparable: " + students);
students.sort(new AgeComparator()); // Sorting using Comparator
System.out.println("Sorted by Comparator: " + students);
}
}
Explanation:
Comparable
allows natural sorting based oncompareTo
(age in this case).Comparator
provides custom sorting based oncompare
(age or other criteria).
7. Interview Question: Can You Create a Generic Class with Multiple Type Parameters?
Yes, Java allows classes to have multiple type parameters. You can create generic classes that take multiple types as parameters to provide flexibility.
Example: Generic Class with Multiple Type Parameters
public class Pair<T, U> {
private T first;
private U second;
public Pair(T first, U second) {
this.first = first;
this.second = second;
}
public T getFirst() {
return first;
}
public U getSecond() {
return second;
}
public static void main(String[] args) {
Pair<String, Integer> pair = new Pair<>("Age", 25);
System.out.println(pair.getFirst() + ": " + pair.getSecond());
}
}
Explanation: The Pair
class is generic and accepts two types T
and U
. This allows it to hold a pair of values, each with different types.
Conclusion
Generics in Java provide a powerful tool to write type-safe and reusable code. Understanding and mastering generics is essential for acing Java interview questions. In this article, we've covered key concepts such as wildcards, type erasure, the difference between Comparable
and Comparator
, and how to use generics with collections and custom classes. By practicing these examples, you'll be well-prepared for generics-related questions in your next Java interview.
More such articles:
https://www.youtube.com/@maheshwarligade
Subscribe to my newsletter
Read articles from Maheshwar Ligade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
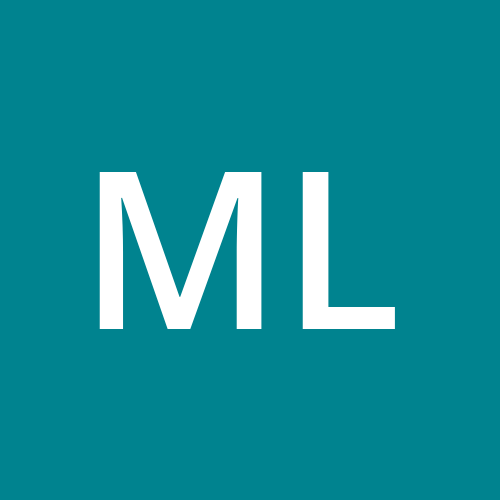
Maheshwar Ligade
Maheshwar Ligade
Learner, Love to make things simple, Full Stack Developer, StackOverflower, Passionate about using machine learning, deep learning and AI