Memory Management in Go: A Beginner’s Guide

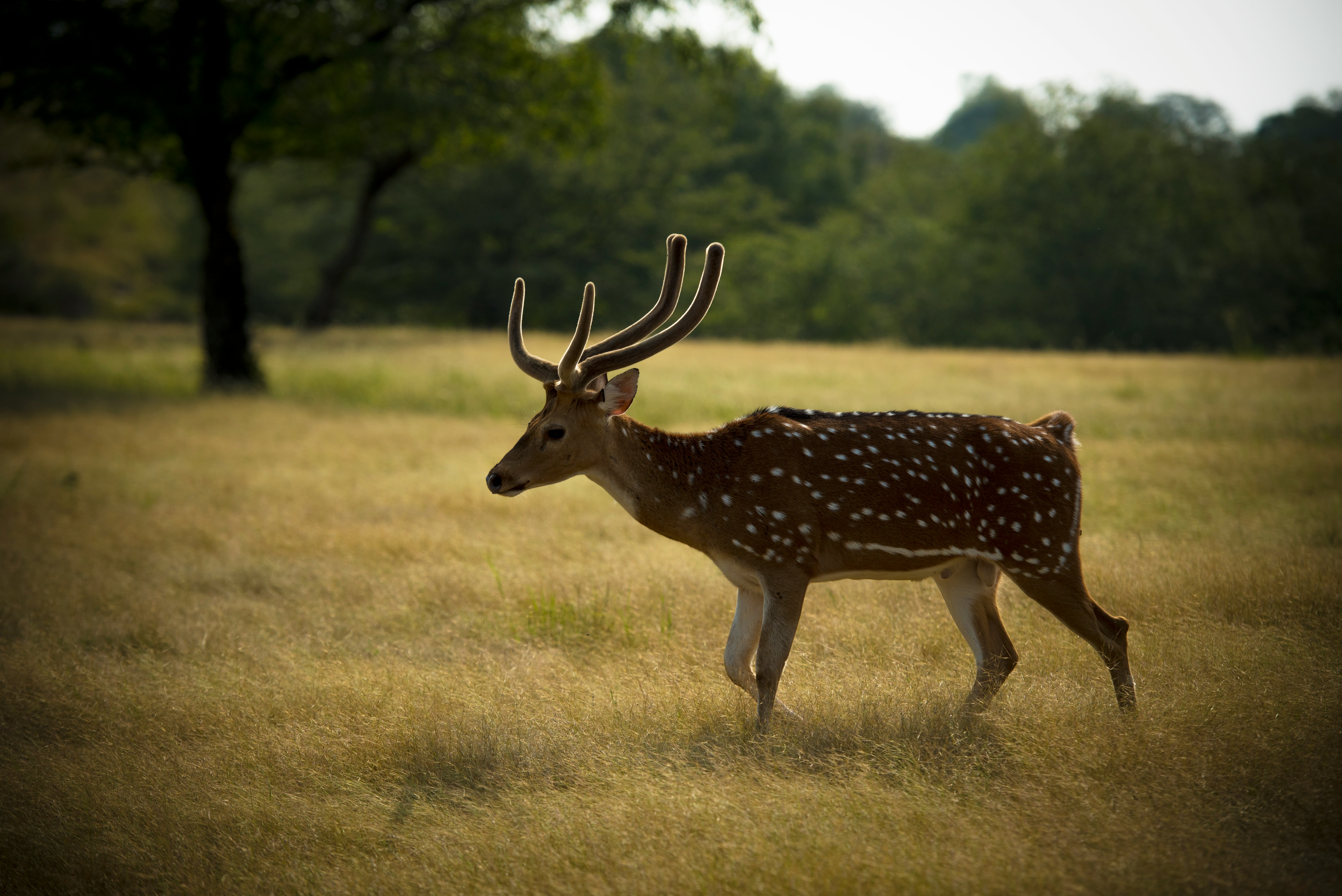
Memory management is a crucial concept in programming, and Go makes it simpler with its automatic garbage collector and efficient memory allocation mechanisms. This article explains how memory is allocated, its lifetime, and the difference between the new
and make
functions in Go—all in an easy-to-understand, beginner-friendly way.
What is Memory Management?
Memory management involves:
Allocating memory: Reserving space in your computer’s memory to store data.
Releasing memory: Freeing up unused memory to make it available for other operations.
In Go, this process is mostly handled automatically by the Garbage Collector (GC). The GC automatically reclaims memory that is no longer in use, so you don’t need to manage it manually.
Memory Allocation in Go
When you create variables, Go allocates memory for storing their values. This memory can be allocated in two places:
Stack
Used for local variables within a function.
Memory is automatically freed when the function returns.
Faster allocation but limited in size.
Heap
Used for dynamically allocated data (e.g., slices, maps).
Memory stays allocated until it’s no longer referenced.
Slower allocation but supports larger and more complex data.
Lifetime of Memory
The lifetime of a variable refers to how long it exists in memory:
Short-lived variables:
Stored on the stack.
Exist only during the execution of a function.
Long-lived variables:
Stored on the heap.
Exist until the program no longer references them (determined by the GC).
The Garbage Collector monitors and removes unused memory automatically, so you don’t need to worry about manual deallocation.
Difference Between new
and make
In Go, both new
and make
are used to allocate memory, but they serve different purposes.
Feature | new | make |
Purpose | Allocates memory for a value | Initializes complex data types (slices, maps, channels) |
Return Type | Returns a pointer to the type | Returns an initialized value of the type |
Use Case | For single values | For slices, maps, and channels |
Using new
The new
function allocates zeroed memory and returns a pointer to it.
Example: Using new
package main
import "fmt"
func main() {
// Allocate memory for an integer using new
ptr := new(int)
// The value is zero-initialized
fmt.Println("Value:", *ptr) // Prints 0
// Update the value
*ptr = 42
fmt.Println("Updated Value:", *ptr) // Prints 42
}
Explanation
new(int)
: Allocates memory for an integer and returns a pointer to it.Zero Initialization: The memory is initialized to the zero value for its type (0 for integers).
Using make
The make
function initializes and allocates memory for slices, maps, and channels.
Example: Using make
package main
import "fmt"
func main() {
// Create a slice using make
slice := make([]int, 5)
// Slice is initialized with zero values
fmt.Println("Slice:", slice) // Prints [0 0 0 0 0]
}
Explanation
make([]int, 5)
: Creates a slice with a length of 5, initialized to zero values.Usage: Only used for slices, maps, and channels.
Key Differences
Data Type:
new
works with any type (e.g., integers, structs).make
is specific to slices, maps, and channels.
Pointer vs Value:
new
returns a pointer to the allocated memory.make
returns the value itself (not a pointer).
Initialization:
new
only allocates memory and zeroes it out.make
initializes the allocated memory for immediate use.
When to Use Which?
Use
new
when you need to allocate memory for a simple type or structure.Use
make
when working with slices, maps, or channels.
Conclusion
Go’s memory management system simplifies programming by handling most of the complexities for you. Understanding how memory is allocated and the roles of new
and make
helps you write efficient and error-free code.
In upcoming tutorials, we’ll explore slices, maps, and channels in detail, where you’ll see more examples of using make
. Keep practicing, and happy coding with Go! 🚀
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
