Managing Environment Variables Securely in Node.js Applications
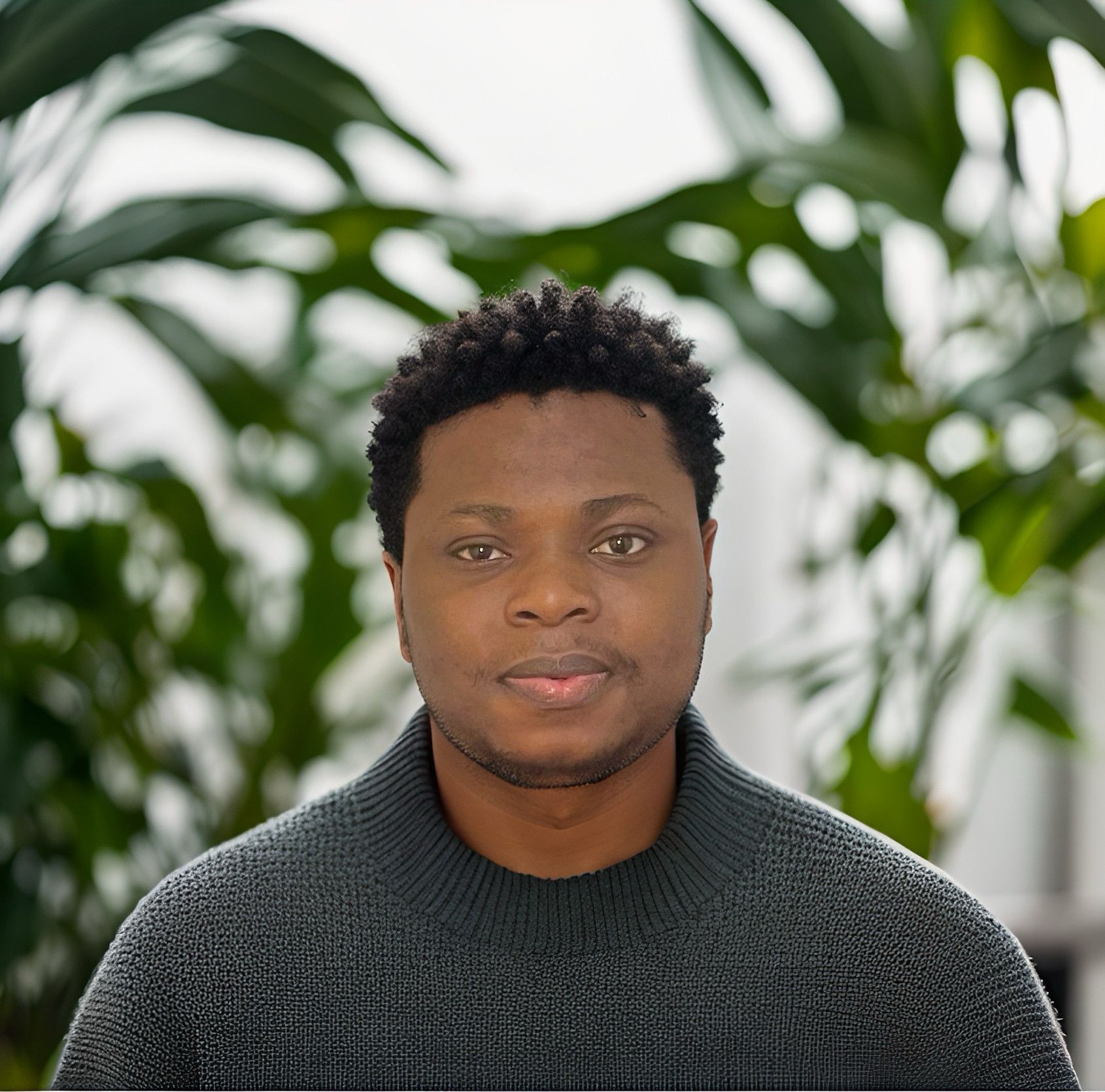
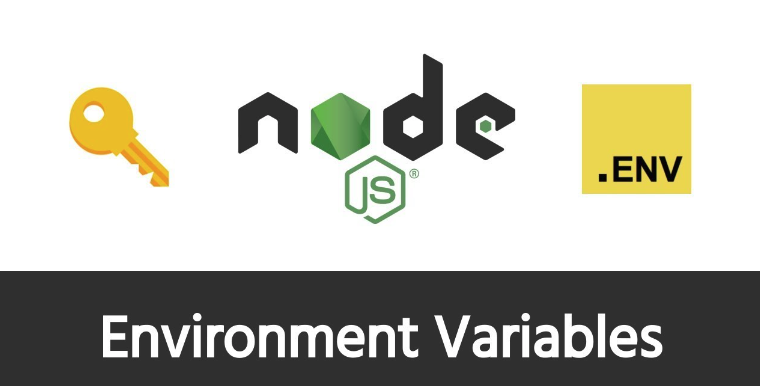
Exposing sensitive information like API keys, database credentials, and other configuration data in your code is a major security risk. For modern Node.js applications, environment variables are a common and secure way to store such sensitive data outside of the codebase. However, managing these variables securely is crucial to ensure that your application remains safe, especially in production environments.
In this article, we will walk you through how to securely manage environment variables in Node.js applications, utilizing tools like .env
files, the dotenv
package, and best practices for handling them in production using secrets management services such as Vault or AWS Secrets Manager.
What Are Environment Variables?
Environment variables are key-value pairs used to store configuration settings outside of your application’s code. These variables can store sensitive information like:
API keys
Database credentials (username, password)
Authentication tokens
Environment-specific settings (e.g., development, production, testing configurations)
Using environment variables instead of hardcoding these secrets directly in your code provides several benefits, such as:
Security: Sensitive information is not exposed in the source code or version control.
Configurability: Easily switch configurations between different environments (development, staging, production).
Portability: Share the application code without exposing sensitive data.
Step 1: Using .env
Files in Node.js
For local development and smaller projects, environment variables can be stored in a .env
file. The .env
file contains key-value pairs that specify your environment settings.
Create a .env
File
In the root of your Node.js project, create a .env
file and add your environment variables. For example:
DATABASE_URL=postgres://user:password@localhost:5432/mydatabase
API_KEY=your_api_key_here
PORT=3000
Install the dotenv
Package
The dotenv
package is a popular Node.js library that loads environment variables from a .env
file into the process.env
object. Install it via npm:
npm install dotenv
Load the Environment Variables
To load the .env
file into your application, add the following line of code at the very top of your app.js
or server.js
file:
require('dotenv').config();
Now you can access your environment variables via process.env
. For example:
const express = require('express');
const app = express();
const port = process.env.PORT || 3000;
const apiKey = process.env.API_KEY;
app.get('/', (req, res) => {
res.send(`API Key: ${apiKey}`);
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
This way, sensitive information like API keys and database credentials are stored outside of your source code and can be easily configured for different environments.
Step 2: Best Practices for Managing .env
Files
1. Don’t Commit .env
Files to Version Control
One of the most important best practices is to never commit .env
files to your version control system (e.g., GitHub, GitLab). These files often contain sensitive data, and committing them would expose your secrets to anyone with access to your repository.
To avoid committing .env
files, add them to your .gitignore
:
# .gitignore
.env
2. Use Environment-Specific .env
Files
For different environments (development, testing, production), create separate .env
files:
.env.development
.env.test
.env.production
You can load the appropriate file based on the environment:
NODE_ENV=production node server.js
In your code, you can then load the respective .env
file based on the NODE_ENV
variable:
require('dotenv').config({ path: `.env.${process.env.NODE_ENV}` });
This way, each environment can have its own configuration, and you can avoid mixing up settings for different stages of your application’s lifecycle.
3. Minimize Secrets in .env
Files
While .env
files are useful for local development, it’s important to limit the number of sensitive variables stored in them. Use .env
files primarily for configuration data that does not require high security. For example, avoid storing secret keys or tokens directly in .env
files when possible, especially for production environments.
Step 3: Securing Environment Variables in Production
While .env
files are great for local development, they’re not sufficient for production, where the risk of exposure is higher. Here are some methods to secure environment variables in production:
1. Using Vault for Secrets Management
HashiCorp Vault is a powerful tool for managing and securing secrets in production. Vault stores and controls access to sensitive information like API keys, passwords, and certificates.
To use Vault with Node.js, you would:
Install and configure Vault on your server.
Store sensitive information securely in Vault (e.g., database credentials).
Use Vault's API to retrieve secrets in your Node.js application.
Here’s an example of retrieving a secret from Vault:
const axios = require('axios');
async function getSecret() {
const response = await axios.get('http://vault-server/v1/secret/data/myapp');
const secret = response.data.data;
console.log(secret);
}
getSecret();
This way, your Node.js application fetches secrets securely from Vault, and they are not exposed in .env
files.
2. Using AWS Secrets Manager
AWS Secrets Manager is another excellent option for securely managing environment variables, especially for applications hosted on AWS.
You can store secrets in Secrets Manager and retrieve them using the AWS SDK. Here’s an example of how to retrieve a secret using AWS SDK in Node.js:
const AWS = require('aws-sdk');
const secretsManager = new AWS.SecretsManager();
async function getSecretValue() {
const secretName = "myapp/database-credentials";
try {
const data = await secretsManager.getSecretValue({ SecretId: secretName }).promise();
if (data.SecretString) {
const secret = JSON.parse(data.SecretString);
console.log(secret);
}
} catch (error) {
console.error("Error retrieving secret: ", error);
}
}
getSecretValue();
AWS Secrets Manager provides an encrypted way to store and manage your secrets, with access control policies to ensure that only authorized entities can access them.
Step 4: General Security Practices
Use Environment Variables for Secrets Only: Keep environment variables strictly for secrets and configurations. Don’t store user data or any sensitive business data in them.
Restrict Access to Secrets: Always use role-based access control (RBAC) and policies to limit who can access secrets in your environment.
Regularly Rotate Secrets: Change your secrets (API keys, credentials) periodically. Automate the rotation process if possible to minimize human error and reduce the impact of potential leaks.
Audit Access to Secrets: Implement logging and auditing to track access to your secrets. Tools like Vault and AWS Secrets Manager provide access logs, which you can monitor for suspicious activity.
Conclusion
Managing environment variables securely is crucial to keeping your Node.js application safe from data breaches and security risks. By using tools like .env
files with the dotenv
package, you can easily manage environment-specific configurations during development. However, for production environments, tools like Vault and AWS Secrets Manager provide more robust solutions for securely managing secrets and configurations.
By following the best practices outlined in this article, you can ensure that your sensitive data is stored and accessed in a secure, manageable way, reducing the risk of exposure and keeping your Node.js application safe.
Happy coding!
Subscribe to my newsletter
Read articles from Nicholas Diamond directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
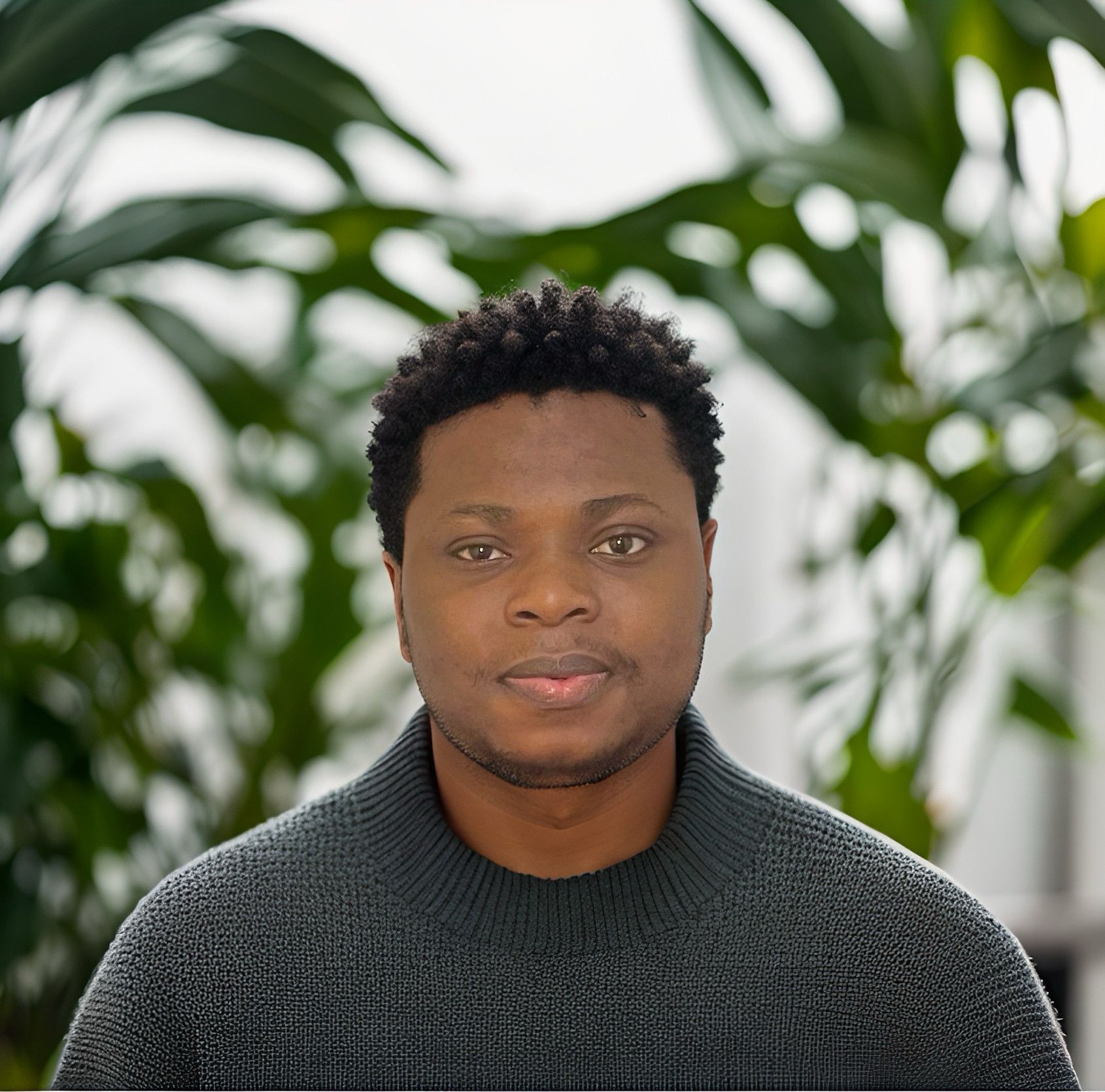