Integrating Celery with Django: A Comprehensive Guide
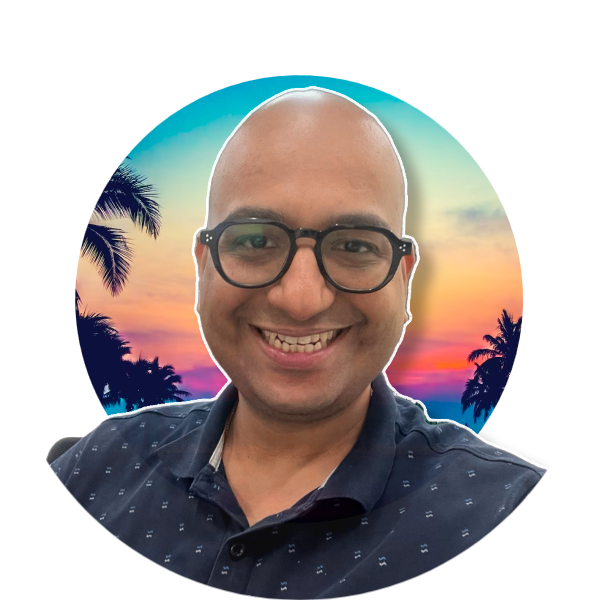
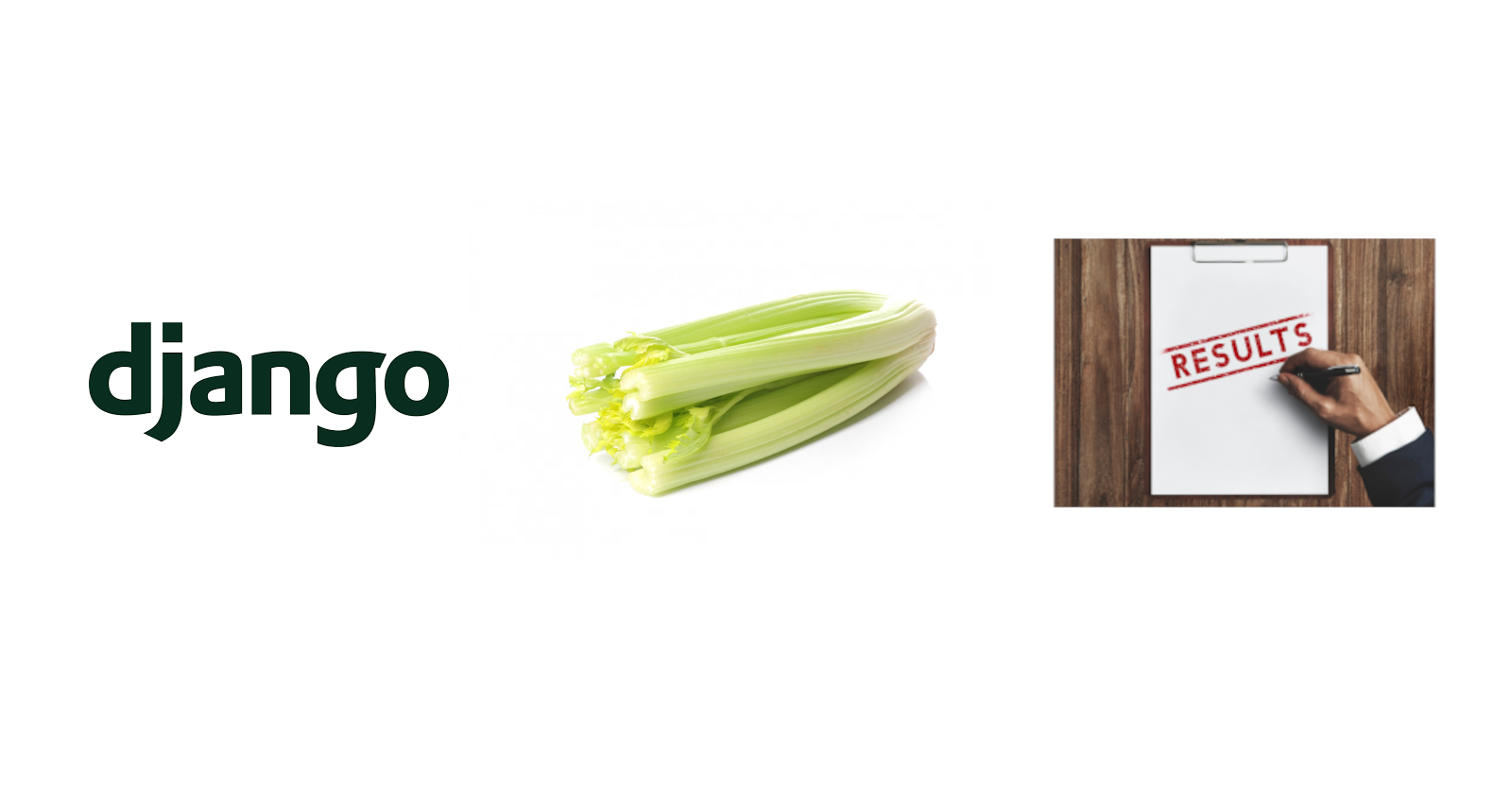
Celery is a powerful distributed task queue that can handle millions of tasks per minute. When combined with Django, it becomes an indispensable tool for handling asynchronous tasks, such as sending emails, processing images, or performing long-running computations. This guide will walk you through the process of integrating Celery with Django, covering both the basics and some advanced topics that are not typically covered in standard tutorials.
Setting Up Celery in a Django Project
Basic Setup
Install Celery: First, ensure that Celery is installed in your Django project. You can do this using pip
pip install celery
Create a Celery Instance: In your Django project, create a new file named
celery.py
in the same directory as yoursettings.py
. This file will define your Celery application instance.# proj/proj/celery.py import os from celery import Celery os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'proj.settings') app = Celery('proj') app.config_from_object('django.conf:settings', namespace='CELERY') app.autodiscover_tasks()
Initialize Celery in Django: Ensure that your Celery app is loaded when Django starts by importing it in your
__init__.py
file.# proj/proj/__init__.py from .celery import app as celery_app __all__ = ('celery_app',)
Advanced Configuration
Custom Task Classes: If your application requires custom behavior for tasks, consider creating custom task classes. For instance, you might want to log additional information or handle retries differently.
from celery import Task class CustomTask(Task): def on_failure(self, exc, task_id, args, kwargs, einfo): # Custom error handling pass
Using Django's ORM as a Result Backend: The
django-celery-results
extension allows you to store task results using Django's ORM. This can be particularly useful for tracking task states and results.pip install django-celery-results
Add
django_celery_results
to yourINSTALLED_APPS
and run migrations:INSTALLED_APPS = ( ..., 'django_celery_results', )
bash
python manage.py migrate django_celery_results
Configure the result backend in your
settings.py
:CELERY_RESULT_BACKEND = 'django-db'
Advanced Topics
Handling Transactions with Celery
A common issue when using Celery with Django is ensuring that tasks are triggered only after a database transaction is committed. Celery 5.4 introduces the delay_on_commit()
method, which simplifies this process.
from django.db import transaction
from myapp.tasks import send_email
def create_user(request):
user = User.objects.create(username=request.POST['username'])
transaction.on_commit(lambda: send_email.delay(user.pk))
Monitoring and Managing Celery
Flower: Flower is a real-time web-based monitoring tool for Celery. It provides insights into task progress, history, and statistics.
pip install flower celery -A proj flower
Prometheus and Grafana: For more advanced monitoring, consider integrating Celery with Prometheus and Grafana. This setup allows you to visualize task metrics and set up alerts.
Optimizing Celery Performance
Task Batching: If you have tasks that can be processed in batches, consider implementing batch processing to reduce overhead and improve throughput.
Prefetch Limits: Adjust the
worker_prefetch_multiplier
setting to control how many tasks a worker prefetches. This can help balance load and reduce memory usage.CELERY_WORKER_PREFETCH_MULTIPLIER = 1
Security Considerations
Task Signing: Enable task signing to ensure that tasks are not tampered with. This adds an extra layer of security, especially in distributed environments.
CELERY_TASK_SERIALIZER = 'json' CELERY_ACCEPT_CONTENT = ['json'] CELERY_RESULT_SERIALIZER = 'json' CELERY_TASK_SIGNING = True
Conclusion
Integrating Celery with Django can significantly enhance your application's ability to handle asynchronous tasks efficiently. By following this guide, you should have a solid foundation for setting up and optimizing Celery in your Django projects. For further exploration, consider diving into Celery's advanced features, such as chord and canvas, to orchestrate complex workflows.
Other topics to look out for -
Using Celery with Django Channels: Explore how Celery can be used alongside Django Channels for real-time applications.
Implementing Retry Strategies: Discuss advanced retry strategies for handling transient errors in tasks.
Celery with Docker and Kubernetes: Guide on deploying Celery in containerized environments for scalability and resilience.
By exploring these topics, you can further enhance your understanding and utilization of Celery in Django projects.
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
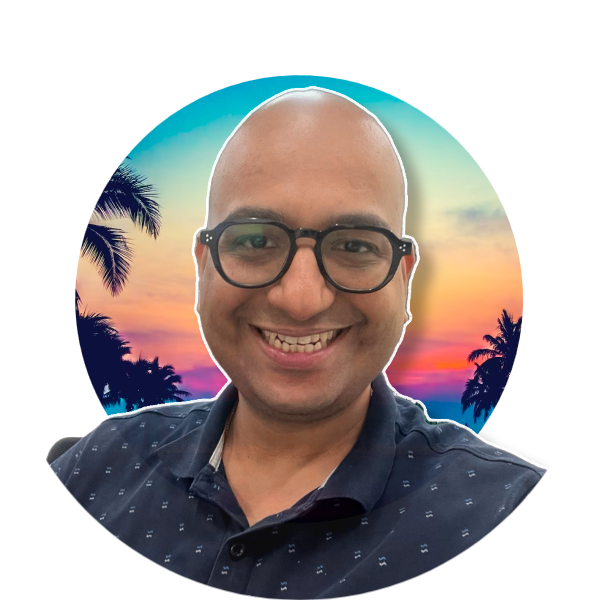
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.