Understanding JavaScript Prototypes: A Key to Mastering OOP
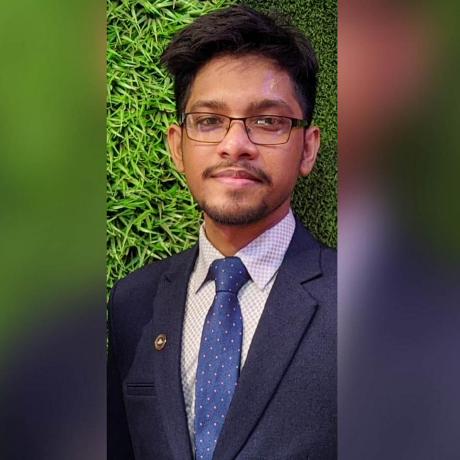
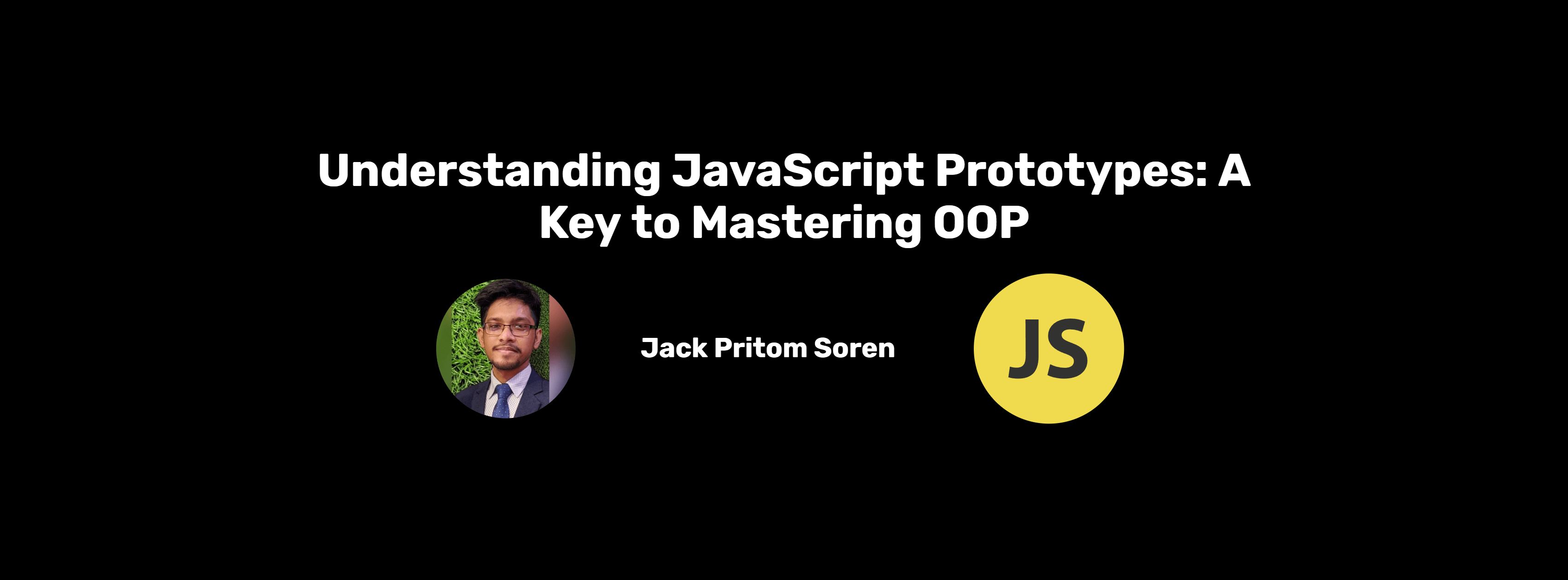
Because JavaScript is a prototype-based language, objects in the language inherit attributes and functions from prototypes. This design pattern is essential to comprehending JavaScript's object-oriented programming (OOP). Understanding prototypes and how to utilize them will be crucial if you want to learn more about JavaScript. This post will discuss the idea of prototypes, how to use them, and how they allow for more effective, reusable, and optimized code.
What is an Object in JavaScript?
Before moving on to prototypes, let's clarify what a JavaScript object is. A JavaScript object is fundamentally a collection of key-value pairs. JavaScript objects are flexible and can be made in a variety of ways, including with factory functions, constructor functions, and object literals.
Creating Objects
1. Object Literal
The simplest way to create an object is using an object literal:
let obj = {
name: 'John',
age: 30
};
2. Constructor Function
A constructor function is used to create instances of objects with similar properties:
function Person(name, age) {
this.name = name;
this.age = age;
}
let person1 = new Person('Alice', 25);
3. Factory Function
A factory function returns a new object each time it's called:
function createPerson(name, age) {
return {
name: name,
age: age
};
}
let person2 = createPerson('Bob', 40);
Returning Objects from Functions
JavaScript functions are powerful because they can return objects, which is useful for creating instances with specific properties and methods.
Example: Returning an Object from a Function
function createCar(make, model) {
return {
make: make,
model: model,
getDetails: function() {
return `${this.make} ${this.model}`;
}
};
}
let car = createCar('Toyota', 'Corolla');
console.log(car.getDetails()); // Toyota Corolla
Optimizing Object Creation with Prototypes
One of the key aspects of JavaScript's prototype-based nature is the ability to define methods on an object's prototype. This optimizes memory usage by allowing multiple objects to share the same methods, rather than defining them for each object individually.
Memory Optimization Example
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.getDetails = function() {
return `${this.name} is ${this.age} years old`;
};
let person1 = new Person('Alice', 25);
let person2 = new Person('Bob', 30);
console.log(person1.getDetails()); // Alice is 25 years old
console.log(person2.getDetails()); // Bob is 30 years old
In the example above, both person1
and person2
share the same getDetails
method, which is defined on the prototype. This means they do not each have their own copy of the method, saving memory.
Object.create() and Prototypes
The Object.create()
method allows you to create a new object that inherits from a specific prototype.
Example: Using Object.create()
let personProto = {
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
let person = Object.create(personProto);
person.name = 'Charlie';
person.greet(); // Hello, my name is Charlie
In this case, person
inherits from personProto
, meaning it has access to the greet
method.
Understanding Prototypes in Depth
Every object in JavaScript has a prototype, which is also an object. This prototype object can have its own properties and methods, which are inherited by the object. This chain of inheritance is known as the prototype chain.
Example: Prototypes in Action
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(`${this.name} makes a sound`);
};
let dog = new Animal('Dog');
dog.speak(); // Dog makes a sound
In this example, dog
is an instance of Animal
, and it inherits the speak
method from Animal.prototype
.
Constructor Functions and Prototypes
Constructor functions are a common way to create multiple instances of the same object type. These functions work in conjunction with prototypes to share methods across instances.
Example: Constructor Function with Prototype Methods
function Book(title, author) {
this.title = title;
this.author = author;
}
Book.prototype.getDetails = function() {
return `${this.title} by ${this.author}`;
};
let book1 = new Book('1984', 'George Orwell');
let book2 = new Book('To Kill a Mockingbird', 'Harper Lee');
console.log(book1.getDetails()); // 1984 by George Orwell
console.log(book2.getDetails()); // To Kill a Mockingbird by Harper Lee
Here, both book1
and book2
share the getDetails
method, thanks to prototype inheritance.
The new
Keyword and the this
Keyword
When you use the new
keyword with a constructor function, it creates a new instance of the object and binds the this
keyword to the new instance.
Example: Using the new
Keyword
function Laptop(brand, model) {
this.brand = brand;
this.model = model;
}
let myLaptop = new Laptop('Dell', 'XPS 13');
console.log(myLaptop.brand); // Dell
In this case, the new
keyword creates a new instance of Laptop
, and this
refers to that instance within the constructor.
ES6 Class Syntax
In ES6, JavaScript introduced the class
syntax, which provides a more convenient and familiar way to define constructor functions and prototypes. However, it's important to note that classes in JavaScript are just syntactic sugar over the prototype-based inheritance model.
Example: Using Classes
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
getDetails() {
return `${this.name} is ${this.age} years old`;
}
}
let person1 = new Person('Alice', 25);
console.log(person1.getDetails()); // Alice is 25 years old
Here, the Person
class behaves similarly to the constructor function and prototype method example, but with a more concise syntax.
Arrays and Prototypes
JavaScript arrays are objects, and like all objects, they inherit properties and methods from their prototype, Array.prototype
. This is why you can call array-specific methods like push
, pop
, and reduce
on arrays.
Example: Working with Arrays and Prototypes
let arr = [1, 2, 3];
console.log(arr.__proto__ === Array.prototype); // true
Array.prototype.sum = function() {
return this.reduce((acc, curr) => acc + curr, 0);
};
console.log(arr.sum()); // 6
In this example, we extend Array.prototype
to include a sum
method, which is shared by all array instances.
Conclusion
The foundation of JavaScript's object-oriented programming paradigm is prototypes. You can write more effective and reusable code if you understand how they operate. The following are the main conclusions:
Objects are key-value pairs, created using literals, constructors, or factory functions.
Prototypes allow objects to share methods and properties, improving memory efficiency.
Use Object.create() to create objects with a specified prototype.
Constructor functions and classes help create multiple instances of an object type, with methods defined on the prototype.
The new keyword creates instances, and this refers to the instance within the constructor.
Arrays inherit methods from
Array.prototype
, which is an object itself.
You may fully utilize JavaScript's object-oriented features and write reusable, effective code by becoming proficient with prototypes. Have fun with your coding!
Subscribe to my newsletter
Read articles from Jack Pritom Soren directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
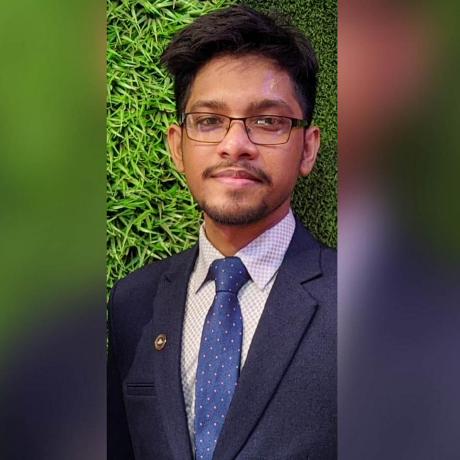
Jack Pritom Soren
Jack Pritom Soren
Software Engineer (SWE) specializing in Frontend development, proficient in JavaScript, React, Next.js, Angular, and a variety of frontend tools. Also skilled in MERN Stack. Committed to crafting clean, efficient code and driving innovation in every project. Passionate about collaborating with dynamic teams to create impactful solutions and continuously advance in the field of frontend development.