My Python Learning Journey: Week 3 - Steps I Took, Problems I Faced, and How I Solved Them.

Table of contents
- Hey everyone, in this week, I learned about the following Python concepts:
- These are the steps I took to grasp the concepts:
- These are the problems that I encountered:
- This is how I solved those problems:
- 1. File I/O Error in Python: Why replace() Fails in Loops and How to Fix It:
- 2. Resolving Word Search Issues in File I/O: Finding All Occurrences in Python:
- 3. Fixing the TypeError in Python Class Methods: The Missing self Parameter:
- 4. Understanding the difference between instance and class variables:
- These are the resources that helped me learn:
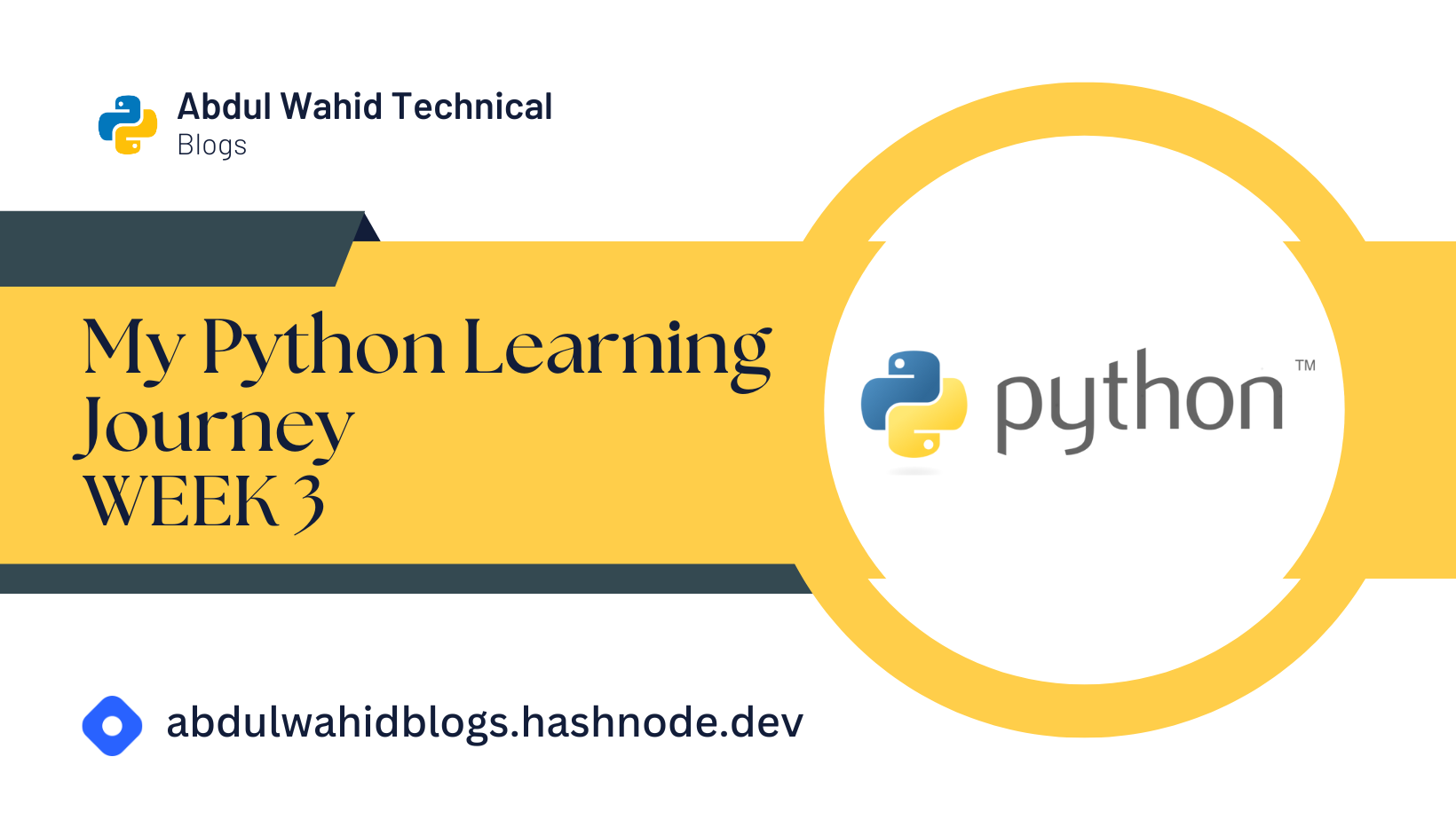
Hey everyone, in this week, I learned about the following Python concepts:
File I/O:(
r
,w
,a
) (with open
)Object-Oriented Programming (OOPS): classes, objects, attributes & methods
These are the steps I took to grasp the concepts:
Reading from and writing to files, understanding file modes (
r
,w
,a
), and using context managers (with open
).Learned about the
with open
syntax to automatically manage file closures.Created simple classes using the
class
keyword and instantiated objects from them.Defined instance variables inside the
__init__
method and accessed them usingself
.
These are the problems that I encountered:
File I/O Error in Python: Why
replace()
Fails in Loops and How to Fix It.Resolving Word Search Issues in File I/O: Finding All Occurrences in Python.
Fixing the TypeError in Python Class Methods: The Missing
self
Parameter.Understanding the difference between instance and class variables.
This is how I solved those problems:
1. File I/O Error in Python: Why replace()
Fails in Loops and How to Fix It:
Problem:
When using the
replace()
function inside a loop, it only replaced the last word in the list, not all the words. This happened because I wasn’t updating the text after each replacement.The variable
newtext
is updated in each loop iteration, but it always starts with the original content oftext
. As a result, only the last word is replaced in the final file.
words = ["Donkey", "Animal", "bad"]
with open("file.txt") as f: # Open the file and read the content
text = f.read()
for word in words:
newtext = text.replace(word, "#" * len(word)) # Replacing using the original text each time
with open("file.txt", "w") as f: # Write the updated text back to the file
f.write(newtext) # Only the last word gets replaced
'''
Output:
Donkey is a herbivores animal.
Donkey is not a ### animal.
Donkey carries load. '''
Solution:
To fix the issue, I updated the
text
variable after replacing each word. This way, thereplace()
function works on the updated text after each iteration.The
text
variable is updated during each loop iteration and each call toreplace()
works on the latest version oftext
, so all the replacements are applied.
words = ["Donkey", "Animal", "bad"]
with open("file.txt") as f: # Open the file and read the content
text = f.read()
for word in words:
text = text.replace(word, "#" * len(word)) # Update text with the new replacement
with open("file.txt", "w") as f: # Write the fully updated text back to the file
f.write(text) # All words are replaced properly
'''
Output:
###### is a herbivores animal.
###### is not a ### animal.
###### carries load. '''
2. Resolving Word Search Issues in File I/O: Finding All Occurrences in Python:
Problem:
The
break
statement stops the loop as soon as the first occurrence of the word "python" is found.The
break
statement exits the loop after the first match is found, so the program doesn’t continue checking the remaining lines.
with open("log.txt") as f: # Open the file "log.txt" in read mode
lines = f.readlines() # Read all lines from the file into a list
line_no = 1 # Initialize line number to 1
for line in lines: # Loop through each line in the file
if "python" in line: # Check if the word "python" is present
print(f"Yes, python is present in line number: {line_no}")
# ERROR: The 'break' here stops the loop after finding the first occurrence,
# so subsequent occurrences are not checked or printed.
break
line_no += 1 # Increment the line number for the next iteration
else: # If no occurrence was found, print a message
print("No, python is not present")
Solution:
To find all occurrences of the word "python," remove the
break
statement and let the loop continue.Without the
break
statement, the loop processes every line in the file. Each time it finds the word "python," it prints the line number where it’s found.
with open("log.txt") as f: # Open the file "log.txt" in read mode
lines = f.readlines() # Read all lines from the file into a list
line_no = 1 # Initialize line number to 1
# Initialize a flag to track if 'python' is found in any line
found = False
for line in lines: # Loop through each line in the file
if "python" in line:
print(f"Yes, python is present in line number: {line_no}")
found = True # Set the flag to True when 'python' is found
line_no += 1 # Increment the line number for the next iteration
# If the flag remains False, it means 'python' wasn't found in any line
if not found:
print("No, python is not present")
3. Fixing the TypeError in Python Class Methods: The Missing self
Parameter:
Problem:
When defining methods inside a class, one common mistake is forgetting to include
self
as the first parameter in the method.This causes Python to throw a
TypeError
when you try to call the method on an instance of the class.
class Employee:
language = "Python"
salary = 1200000
def getInfo(): # Missing 'self' as the first parameter
print(f"The language selected is {language} and the salary is {salary}")
abdul = Employee() # Create an instance of Employee
abdul.getInfo() # Call the getInfo method on the instance
# TypeError: Employee.getInfo() takes 0 positional arguments but 1 was given
Solution:
To fix this error, add
self
as the first parameter of thegetInfo()
method.This allows the method to access instance attributes such as
language
andsalary
class Employee:
language = "Python"
salary = 1200000
def getInfo(self): # Correct method definition with 'self'
print(f"The language selected is {self.language} and the salary is {self.salary}")
abdul = Employee() # Create an instance of Employee
abdul.getInfo() # Call the getInfo method on the instance
# Output: The language selected is Python and the salary is 1200000
4. Understanding the difference between instance and class variables:
Problem: Understanding the difference between instance and class variables.
Solution:
Instance variables belong to a specific object (instance) of a class. This means that each object can have its own unique values for these variables.
Class variables are shared across all objects (instances) of a class. This means that if we change a class variable, all objects of that class will reflect the change.
class Car: # Class variable (shared by all instances)
wheels = 4 # All cars have 4 wheels by default
def __init__(self, make, model): # Instance variables (specific to each car)
self.make = make
self.model = model
# Create two Car objects
car1 = Car("Toyota", "Corolla")
car2 = Car("Honda", "Civic")
# Accessing class variable (same for all cars)
print(car1.wheels) # Output: 4
print(car2.wheels) # Output: 4
# Accessing instance variables (unique for each car)
print(car1.make) # Output: Toyota
print(car2.make) # Output: Honda
These are the resources that helped me learn:
Some valuable resources I'd like to share:
Subscribe to my newsletter
Read articles from Sheikh Abdul Wahid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
