10 Bash scripts that every beginner DevOps engineer can use to automate workflows

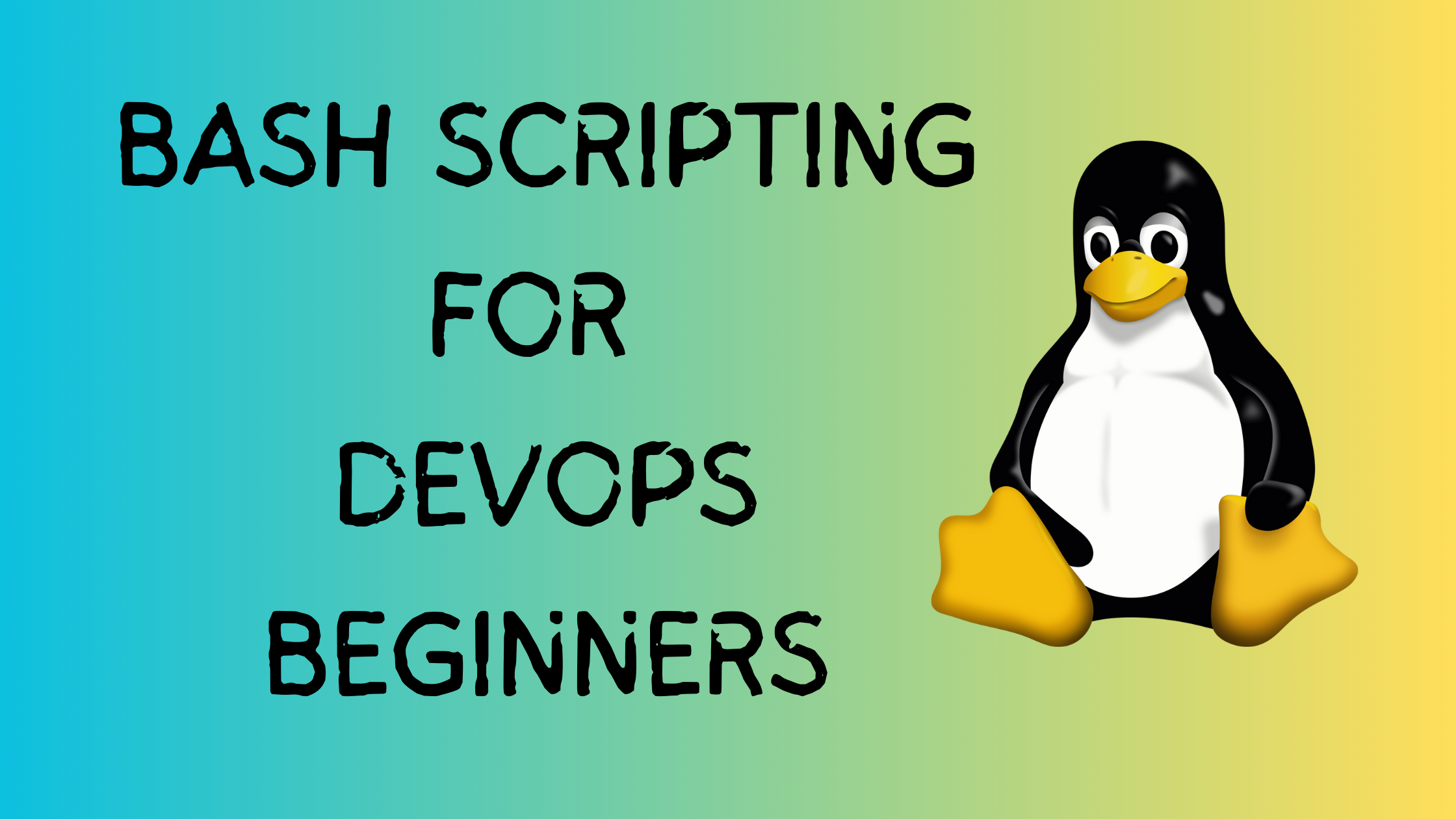
1. Update and Upgrade System Packages
Scenario: Your server needs to be updated with the latest security patches.
Script:
#!/bin/bash
echo "Updating system packages..."
sudo apt update && sudo apt upgrade -y
echo "System updated successfully!"
What it does: Ensures your server is updated.
Steps:
sudo apt update
: Checks for new updates.sudo apt upgrade -y
: Installs updates automatically.
2. Monitor Disk Space
Scenario: You want to avoid your server running out of storage space.
Script:
#!/bin/bash
THRESHOLD=80
echo "Checking disk usage..."
USAGE=$(df / | tail -1 | awk '{print $5}' | sed 's/%//')
if [ "$USAGE" -gt "$THRESHOLD" ]; then
echo "Warning! Disk usage is above $THRESHOLD%. Current usage: $USAGE%"
else
echo "Disk usage is fine. Current usage: $USAGE%"
fi
What it does: Alerts you if the disk usage exceeds 80%.
3. Automate File Backups
Scenario: You need daily backups for a critical directory.
Script:
#!/bin/bash
SOURCE="/path/to/source"
DEST="/path/to/backup"
DATE=$(date +%Y-%m-%d)
echo "Backing up files from $SOURCE to $DEST/$DATE..."
mkdir -p "$DEST/$DATE"
cp -r "$SOURCE"/* "$DEST/$DATE"
echo "Backup completed!"
What it does: Creates a backup folder with the current date and copies files into it.
4. Deploy a Simple Web Server
Scenario: Quickly set up a basic web server for testing.
Script:
#!/bin/bash
echo "Installing Nginx..."
sudo apt update
sudo apt install -y nginx
echo "Starting Nginx service..."
sudo systemctl start nginx
sudo systemctl enable nginx
echo "Nginx web server is ready!"
What it does: Installs and starts Nginx, a lightweight web server.
5. Add and Manage Users
Scenario: You need to create a new user with admin rights.
Script:
#!/bin/bash
USERNAME="newuser"
PASSWORD="password123"
GROUP="sudo"
echo "Creating user $USERNAME..."
sudo useradd -m -s /bin/bash "$USERNAME"
echo "$USERNAME:$PASSWORD" | sudo chpasswd
echo "Adding $USERNAME to $GROUP group..."
sudo usermod -aG "$GROUP" "$USERNAME"
echo "User $USERNAME created with admin privileges!"
What it does: Creates a user, sets a password, and grants admin access.
6. Check Server Uptime
Scenario: You want to know how long your server has been running without restarting.
Script:
#!/bin/bash
echo "Server uptime:"
uptime
What it does: Displays the server’s uptime and system load.
7. Check Active Processes
Scenario: Your server is slow, and you want to find resource-heavy processes.
Script:
#!/bin/bash
echo "Top 5 processes by CPU usage:"
ps -eo pid,ppid,cmd,%mem,%cpu --sort=-%cpu | head -6
What it does: Lists the top 5 processes using the most CPU.
8. Automate SSH Key Generation
Scenario: You need a secure key for connecting to another server without a password.
Script:
#!/bin/bash
KEY_NAME="id_rsa"
echo "Generating SSH key..."
ssh-keygen -t rsa -b 4096 -f "$HOME/.ssh/$KEY_NAME" -N ""
echo "SSH key generated at $HOME/.ssh/$KEY_NAME"
What it does: Creates an SSH key pair for secure connections.
9. Restart a Service
Scenario: Your web server stops unexpectedly, and you want to restart it automatically.
Script:
#!/bin/bash
KEY_NAME="id_rsa"
echo "Generating SSH key..."
ssh-keygen -t rsa -b 4096 -f "$HOME/.ssh/$KEY_NAME" -N ""
echo "SSH key generated at $HOME/.ssh/$KEY_NAME"
What it does: Checks if a service (e.g., Nginx) is running and restarts it if it’s not.
10. Schedule a Cron Job
Scenario: You need a script to run automatically every day at 2 AM.
Script:
#!/bin/bash
JOB="0 2 * * * /path/to/script.sh"
CRON_FILE="/tmp/mycron"
echo "Adding cron job..."
(crontab -l 2>/dev/null; echo "$JOB") | crontab -
echo "Cron job added successfully!"
What it does: Schedules a script (/path/to/
script.sh
) to run at 2 AM daily.
Tips for Running Scripts:
Save the Script: Use a
.sh
file, e.g.,script.sh
.Make it Executable: Run
chmod +x
script.sh
.Run It: Use
./
script.sh
to execute the script.Debugging: Use
bash -x
script.sh
if you face errors.
With these scripts, you can automate many repetitive tasks, saving time and ensuring consistency in your DevOps workflow!
Subscribe to my newsletter
Read articles from Shaik Mustafa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
